Bridge Pattern简单随笔
2010-03-16 11:13
169 查看
Bridge模式就是把抽象部分和它的实现部分分离开来,让两者可独立变化。这里的抽象部分指的是一个概念层次上的东西,它的实现部分指的是实现这个东西的(功能)部分,分离就把实现部分从它要实现的抽象部分独立出来,自我封装成对象。
桥梁模式:将抽象化与实现化脱耦,使得二者可以独立的变化,也就是说将他们之间的强关联变成弱关联,也就是指在一个软件系统的抽象化和实现化之间使用组合/聚合关系而不是继承关系,从而使两者可以独立的变化。Bridge模式使用“对象间的组合关系”解耦了抽象和实现之间固有的绑定关系,使得抽象和实现可以沿着各自的维度来变化。
Bridge模式很好的符合了开放-封闭原则和优先使用对象,而不是继承这两个面向对象原则,理解好这两个原则,有助于形成正确的设计思想和培养良好的设计风格。。Bridge模式有时候类似于多继承方案,但是多继承方案往往违背了类的单一职责原则(即一个类只有一个变化的原因),复用性比较差。Bridge模式是比多继承方案更好的解决方法。Bridge模式的应用一般在“两个非常强的变化维度”,有时候即使有两个变化的维度,但是某个方向的变化维度并不剧烈——换言之两个变化不会导致纵横交错的结果,并不一定要使用Bridge模式。
开放封闭原则(OCP,Open Closed Principle)是所有面向对象原则的核心。软件设计本身所追求的目标就是封装变化、降低耦合,而开放封闭原则正是对这一目标的最直接体现。其他的设计原则,很多时候是为实现这一目标服务的。
关于开放封闭原则,其核心的思想是:
软件实体应该是可扩展,而不可修改的。也就是说,对扩展是开放的,而对修改是封闭的。
因此,开放封闭原则主要体现在两个方面:
对扩展开放,意味着有新的需求或变化时,可以对现有代码进行扩展,以适应新的情况。
对修改封闭,意味着类一旦设计完成,就可以独立完成其工作,而不要对类进行任何修改。
桥梁模式的用意
桥梁模式的用意是"将抽象化(Abstraction)与实现化(Implementation)脱耦,使得二者可以独立地变化"。这句话有三个关键词,也就是抽象化、实现化和脱耦。
抽象化
存在于多个实体中的共同的概念性联系,就是抽象化。作为一个过程,抽象化就是忽略一些信息,从而把不同的实体当做同样的实体对待。
实现化
抽象化给出的具体实现,就是实现化。
脱耦
所谓耦合,就是两个实体的行为的某种强关联。而将它们的强关联去掉,就是耦合的解脱,或称脱耦。在这里,脱耦是指将抽象化和实现化之间的耦合解脱开,或者说是将它们之间的强关联改换成弱关联。将两个角色之间的继承关系改为聚合关系,就是将它们之间的强关联改换成为弱关联。因此,桥梁模式中的所谓脱耦,就是指在一个软件系统的抽象化和实现化之间使用组合/聚合关系而不是继承关系,从而使两者可以相对独立地变化。这就是桥梁模式的用意。
下图所示就是一个实现了桥梁模式的示意性系统的结构图。
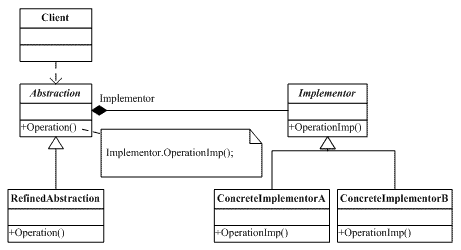
可以看出,这个系统含有两个等级结构,也就是:
由抽象化角色和修正抽象化角色组成的抽象化等级结构。
由实现化角色和两个具体实现化角色所组成的实现化等级结构。
抽象化角色为设计的重点,采用组合模式来解耦强关联:
1 using System;
2 using System.Collections.Generic;
3 using System.Text;
4
5 namespace BridgePatternSam
6 {
7 public abstract class Operater
8 {
9 //对象组合进行操作 关键点
10
11 protected Implement _implementer;
12 public Implement Implementer
13 {
14 set
15 {
16 _implementer = value;
17 }
18 }
19
20 public virtual void Work(string str)
21 {//必须用VIRTUAL 用ABSTRACT 无法声明主体
22 _implementer.Execute(str);
23 }
24 }
25 }
26
27 using System;
28 using System.Collections.Generic;
29 using System.Text;
30
31 namespace BridgePatternSam
32 {
33 public class OperaterA:Operater
34 {
35 public override void Work(string str)
36 {//
37 _implementer.Execute(str);
38 }
39 }
40 }
41
42 using System;
43 using System.Collections.Generic;
44 using System.Text;
45
46 namespace BridgePatternSam
47 {
48 public class OperaterB:Operater
49 {
50 public override void Work(string str)
51 {
52 //throw new Exception("The method or operation is not implemented.");
53 _implementer.Execute(str);
54 }
55 }
56 }
57
具体操作类为:
1 using System;
2 using System.Collections.Generic;
3 using System.Text;
4
5 namespace BridgePatternSam
6 {
7 public abstract class Implement
8 {//具体操作类
9 public abstract void Execute(string str);
10 }
11 }
12
13
14 using System;
15 using System.Collections.Generic;
16 using System.Text;
17
18 namespace BridgePatternSam
19 {
20 public class JavaImplement:Implement
21 {
22 public override void Execute(string str)
23 {
24 //throw new Exception("The method or operation is not implemented.");
25 Console.WriteLine("----------------------------------------------");
26 Console.WriteLine("It is carried out in the platform of java!!");
27 Console.WriteLine("input information : "+str);
28 Console.WriteLine("----------------------------------------------");
29 }
30 }
31 }
32
33 using System;
34 using System.Collections.Generic;
35 using System.Text;
36
37 namespace BridgePatternSam
38 {
39 public class NetImplement:Implement
40 {
41 public override void Execute(string str)
42 {
43 Console.WriteLine("----------------------------------------------");
44 Console.WriteLine("It is carried out in the platform of net!!");
45 Console.WriteLine("input information :"+str);
46 Console.WriteLine("----------------------------------------------");
47 }
48 }
49 }
50
客户端就得相应的组合对象,实现相应的功能:
1 using System;
2 using System.Collections.Generic;
3 using System.Text;
4
5 namespace BridgePatternSam
6 {
7 class Program
8 {
9 static void Main(string[] args)
10 {
11 Operater oper = new OperaterA();
12 oper.Implementer = new JavaImplement();
13 oper.Work("A java");
14 Console.ReadLine();
15
16 oper = new OperaterB();
17 oper.Implementer = new NetImplement();
18 oper.Work("B net");
19 Console.ReadLine();
20
21 oper = new OperaterB();
22 oper.Implementer = new JavaImplement();
23 oper.Work("B JAVA");
24 Console.ReadLine();
25 }
26 }
27 }
28
写了3种方式的,当然这个是很简单的操作,只是最基础的操作而已,具体情况复杂多了
显示结果如下:
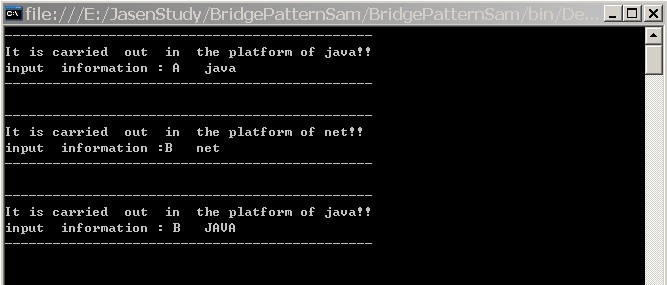
************************************************************
源代码下载:/Files/jasenkin/BridgePatternSam.rar
桥梁模式:将抽象化与实现化脱耦,使得二者可以独立的变化,也就是说将他们之间的强关联变成弱关联,也就是指在一个软件系统的抽象化和实现化之间使用组合/聚合关系而不是继承关系,从而使两者可以独立的变化。Bridge模式使用“对象间的组合关系”解耦了抽象和实现之间固有的绑定关系,使得抽象和实现可以沿着各自的维度来变化。
Bridge模式很好的符合了开放-封闭原则和优先使用对象,而不是继承这两个面向对象原则,理解好这两个原则,有助于形成正确的设计思想和培养良好的设计风格。。Bridge模式有时候类似于多继承方案,但是多继承方案往往违背了类的单一职责原则(即一个类只有一个变化的原因),复用性比较差。Bridge模式是比多继承方案更好的解决方法。Bridge模式的应用一般在“两个非常强的变化维度”,有时候即使有两个变化的维度,但是某个方向的变化维度并不剧烈——换言之两个变化不会导致纵横交错的结果,并不一定要使用Bridge模式。
开放封闭原则(OCP,Open Closed Principle)是所有面向对象原则的核心。软件设计本身所追求的目标就是封装变化、降低耦合,而开放封闭原则正是对这一目标的最直接体现。其他的设计原则,很多时候是为实现这一目标服务的。
关于开放封闭原则,其核心的思想是:
软件实体应该是可扩展,而不可修改的。也就是说,对扩展是开放的,而对修改是封闭的。
因此,开放封闭原则主要体现在两个方面:
对扩展开放,意味着有新的需求或变化时,可以对现有代码进行扩展,以适应新的情况。
对修改封闭,意味着类一旦设计完成,就可以独立完成其工作,而不要对类进行任何修改。
桥梁模式的用意
桥梁模式的用意是"将抽象化(Abstraction)与实现化(Implementation)脱耦,使得二者可以独立地变化"。这句话有三个关键词,也就是抽象化、实现化和脱耦。
抽象化
存在于多个实体中的共同的概念性联系,就是抽象化。作为一个过程,抽象化就是忽略一些信息,从而把不同的实体当做同样的实体对待。
实现化
抽象化给出的具体实现,就是实现化。
脱耦
所谓耦合,就是两个实体的行为的某种强关联。而将它们的强关联去掉,就是耦合的解脱,或称脱耦。在这里,脱耦是指将抽象化和实现化之间的耦合解脱开,或者说是将它们之间的强关联改换成弱关联。将两个角色之间的继承关系改为聚合关系,就是将它们之间的强关联改换成为弱关联。因此,桥梁模式中的所谓脱耦,就是指在一个软件系统的抽象化和实现化之间使用组合/聚合关系而不是继承关系,从而使两者可以相对独立地变化。这就是桥梁模式的用意。
二、 桥梁模式的结构
下图所示就是一个实现了桥梁模式的示意性系统的结构图。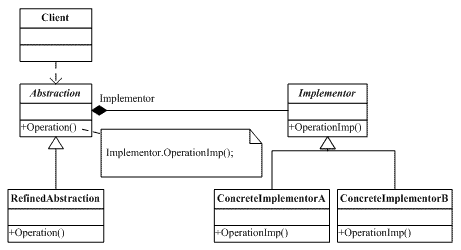
可以看出,这个系统含有两个等级结构,也就是:
由抽象化角色和修正抽象化角色组成的抽象化等级结构。
由实现化角色和两个具体实现化角色所组成的实现化等级结构。
抽象化角色为设计的重点,采用组合模式来解耦强关联:
1 using System;
2 using System.Collections.Generic;
3 using System.Text;
4
5 namespace BridgePatternSam
6 {
7 public abstract class Operater
8 {
9 //对象组合进行操作 关键点
10
11 protected Implement _implementer;
12 public Implement Implementer
13 {
14 set
15 {
16 _implementer = value;
17 }
18 }
19
20 public virtual void Work(string str)
21 {//必须用VIRTUAL 用ABSTRACT 无法声明主体
22 _implementer.Execute(str);
23 }
24 }
25 }
26
27 using System;
28 using System.Collections.Generic;
29 using System.Text;
30
31 namespace BridgePatternSam
32 {
33 public class OperaterA:Operater
34 {
35 public override void Work(string str)
36 {//
37 _implementer.Execute(str);
38 }
39 }
40 }
41
42 using System;
43 using System.Collections.Generic;
44 using System.Text;
45
46 namespace BridgePatternSam
47 {
48 public class OperaterB:Operater
49 {
50 public override void Work(string str)
51 {
52 //throw new Exception("The method or operation is not implemented.");
53 _implementer.Execute(str);
54 }
55 }
56 }
57
具体操作类为:
1 using System;
2 using System.Collections.Generic;
3 using System.Text;
4
5 namespace BridgePatternSam
6 {
7 public abstract class Implement
8 {//具体操作类
9 public abstract void Execute(string str);
10 }
11 }
12
13
14 using System;
15 using System.Collections.Generic;
16 using System.Text;
17
18 namespace BridgePatternSam
19 {
20 public class JavaImplement:Implement
21 {
22 public override void Execute(string str)
23 {
24 //throw new Exception("The method or operation is not implemented.");
25 Console.WriteLine("----------------------------------------------");
26 Console.WriteLine("It is carried out in the platform of java!!");
27 Console.WriteLine("input information : "+str);
28 Console.WriteLine("----------------------------------------------");
29 }
30 }
31 }
32
33 using System;
34 using System.Collections.Generic;
35 using System.Text;
36
37 namespace BridgePatternSam
38 {
39 public class NetImplement:Implement
40 {
41 public override void Execute(string str)
42 {
43 Console.WriteLine("----------------------------------------------");
44 Console.WriteLine("It is carried out in the platform of net!!");
45 Console.WriteLine("input information :"+str);
46 Console.WriteLine("----------------------------------------------");
47 }
48 }
49 }
50
客户端就得相应的组合对象,实现相应的功能:
1 using System;
2 using System.Collections.Generic;
3 using System.Text;
4
5 namespace BridgePatternSam
6 {
7 class Program
8 {
9 static void Main(string[] args)
10 {
11 Operater oper = new OperaterA();
12 oper.Implementer = new JavaImplement();
13 oper.Work("A java");
14 Console.ReadLine();
15
16 oper = new OperaterB();
17 oper.Implementer = new NetImplement();
18 oper.Work("B net");
19 Console.ReadLine();
20
21 oper = new OperaterB();
22 oper.Implementer = new JavaImplement();
23 oper.Work("B JAVA");
24 Console.ReadLine();
25 }
26 }
27 }
28
写了3种方式的,当然这个是很简单的操作,只是最基础的操作而已,具体情况复杂多了
显示结果如下:
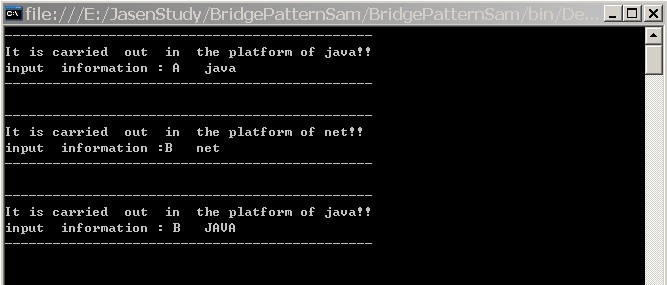
************************************************************
源代码下载:/Files/jasenkin/BridgePatternSam.rar
相关文章推荐
- 随笔--帧动画的几种简单实现方式
- PhotoShop简单随笔
- 鸡尾酒排序简单随笔
- [Object-C语言随笔之四]创建视图并绘制简单图形
- 随笔之生成简单的验证码
- 自定义委托,事件,参数的简单随笔
- 建造者模式(Builder Pattern)简单随笔
- Adapter Patterm简单随笔
- Composite Pattern简单随笔
- Decorator Pattern简单随笔
- Android随笔之布局属性简单用法
- jquery学习随笔(简单选择器)
- python读写csv文件的心得(随笔一:简单的读写csv文件)
- Java Web 随笔(2):简单的DOM操作
- android Mediaplayer各种属性和方法简单介绍(copy自随笔)
- 自定义特性Attribute简单随笔
- Proxy Pattern设计模式简单随笔
- cc随笔:简单的自定义图片加密
- 写一个简单的静态商城页面注意点(个人随笔)
- 第一篇随笔,讲讲简单的js基本功【prototype初探】