Asp.Net 开发之(1) ---如何自动加载数据到页面或更新数据到数据容器中(基础控件)
2009-09-04 10:48
1061 查看
此文章只代表作者在某一段时间内的看法与观点。
对于一个程序员来说,他们最希望看到了就是如何可以减少他们的工作,也就是说使用最少的code,实现最多的function。在Asp.Net开发的过程中,对data controls的操作是不必不可少,最基本的功能就是对Controls data的加载及Controls选择或输入的值存储起来,也就是所谓的Load 和Update方法。而我们在平时的软件开发的过程中又是怎样来实现这些功能的呢?一般情况下我相信大家都和我之前一样,通过对页面中的每一个Controls做更新或加载,如下代码所示。
一.传统的操作模式
HTML 代码:

1

<%@ Page Language="C#" AutoEventWireup="true" CodeBehind="ControlsOpr.aspx.cs" Inherits="Adrienne.WCF.WebUI.ControlsOpr" %>
2

<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
3

<html xmlns="http://www.w3.org/1999/xhtml">
4

<head runat="server">
5

<title>Untitled Page</title>
6

</head>
7

<body>
8

<form id="form1" runat="server">
9

<div>
10

<dir>
11

用户名:<asp:TextBox ID="UserName" runat="server"></asp:TextBox>
12

</dir>
13

<dir>
14

性别:<asp:RadioButtonList ID="Sex" runat="server" RepeatColumns="2">
15

<asp:ListItem>男</asp:ListItem>
16

<asp:ListItem>女</asp:ListItem>
17

</asp:RadioButtonList>
18

<asp:Button ID="Upload" runat="server" Text="加载" onclick="Upload_Click" />
19

<asp:Button ID="Update" runat="server" Text="更新" onclick="Update_Click" />
20

</dir>
21

</div>
22

<asp:GridView ID="GridView1" runat="server">
23

</asp:GridView>
24

</form>
25

</body>
26

</html>
CS代码:

1

using System;
2

using System.Collections;
3

using System.Configuration;
4

using System.Data;
5

using System.Linq;
6

using System.Web;
7

using System.Web.Security;
8

using System.Web.UI;
9

using System.Web.UI.HtmlControls;
10

using System.Web.UI.WebControls;
11

using System.Web.UI.WebControls.WebParts;
12

using System.Xml.Linq;
13

14

namespace Adrienne.WCF.WebUI
15

{
16

public partial class ControlsOpr : System.Web.UI.Page
17

{
18

protected void Page_Load(object sender, EventArgs e)
19

{
20

if (!IsPostBack)
21

{
22

//Create the Date
23

DataTable dt = new DataTable();
24

dt.Columns.Add("USER_NAME");
25

dt.Columns.Add("SEX");
26

DataRow row = dt.NewRow();
27

row["USER_NAME"] = "Xiong Wei";
28

row["SEX"] = "男";
29

dt.Rows.Add(row);
30

ViewState["dt"] = dt;
31

}
32

}
33

34

protected void Upload_Click(object sender, EventArgs e)
35

{
36

//Original method
37

if (ViewState["dt"] != null)
38

{
39

DataTable dt = ViewState["dt"] as DataTable;
40

////Should be check each column before
41

this.UserName.Text = dt.Rows[0]["USER_NAME"] as string;
42

this.Sex.SelectedValue = dt.Rows[0]["SEX"] as string;
43

44

this.GridView1.DataSource = dt;
45

this.DataBind();
46

}
47

}
48

49

protected void Update_Click(object sender, EventArgs e)
50

{
51

if (ViewState["dt"] != null)
52

{
53

DataTable dt = ViewState["dt"] as DataTable;
54

//Should be check each column before
55

dt.Rows[0]["USER_NAME"]=this.UserName.Text;
56

dt.Rows[0]["SEX"]=this.Sex.SelectedValue;
57

58

this.GridView1.DataSource = dt;
59

this.DataBind();
60

}
61

}
62

}
63

}
界面如下:
点击Upload_Click
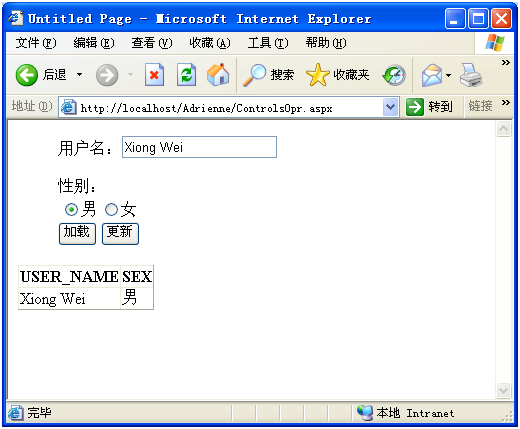
点击Update_Click
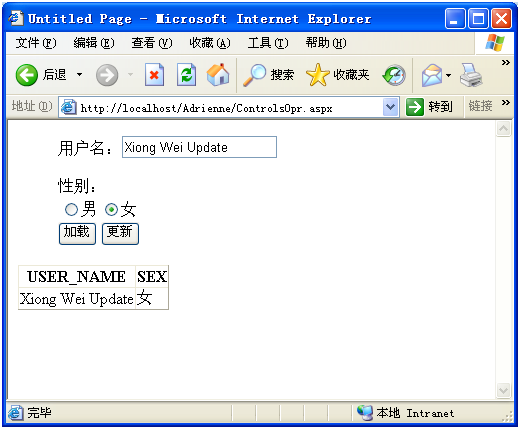
传统模式就是这样对页面中的数据进行操作的。
Upload_Click事件完成了对面页中控件值的加载;
Update_Click事件完成了把页面中控件的值更新到相应的数据控件中去。
我们可以来假设一下,如果存在这样一个项目,项目中存在大概有50个Page,每个Page当中大概有50个Control,这时就存在一个问题,我们如何对这些控件进行加载和保存数据呢,可想而知,上面的方法将存在巨大的工作量,针对上面方法的一个缺点,我们就可以考虑写一些Common的方法来实现上面的操作,从而优化操作模式,下面本人就自己的观点写了一段代码来优化上面的不足。
二.优化的操作模式
在本例中Controls比较少,假设每一个Page中有50个Controls,一个Project中有几十个Pages,那么请问,这个时候我们怎么做,难道还是每一个写一次吧,毋庸置疑,答案是否定的,那么我们又应该怎么了做呢?为此本人写了一个简单的类,这个类主要用来完成对控件值的加载以及对数据容器的更新。
操作类代码:

1

using System;
2

using System.Data;
3

using System.Configuration;
4

using System.Linq;
5

using System.Web;
6

using System.Web.Security;
7

using System.Web.UI;
8

using System.Web.UI.HtmlControls;
9

using System.Web.UI.WebControls;
10

using System.Web.UI.WebControls.WebParts;
11

using System.Xml.Linq;
12

using Microsoft.Practices.EnterpriseLibrary.Logging;
13

14

namespace Adrienne.WCF.WebUI
15

{
16

/// <summary>
17

/// Provide the operation for the controls of the pages
18

/// </summary>
19

public class PageHelper : PageBase
20

{
21

/// <summary>
22

/// Fill the data information to the controls of the web page
23

/// </summary>
24

/// <param name="row"></param>
25

/// <param name="control"></param>
26

public static void FillPage(DataRow row, Control control)
27

{
28

foreach (DataColumn col in row.Table.Columns)
29

{
30

string colName = col.ColumnName.Replace("_", "");
31

Control findControl;
32

findControl = control.FindControl(colName);
33

if (findControl != null)
34

{
35

if (row[col.ColumnName] == null)
36

{
37

continue;
38

}
39

if (findControl is TextBox)
40

{
41

TextBox textbox = (TextBox)findControl;
42

textbox.Text = row[col.ColumnName].ToString();
43

}
44

else if (findControl is RadioButton)
45

{
46

RadioButton radiobutton = (RadioButton)findControl;
47

radiobutton.Checked = bool.Parse(row[col.ColumnName].ToString());
48

}
49

else if (findControl is RadioButtonList)
50

{
51

RadioButtonList radiobuttonlist = (RadioButtonList)findControl;
52

radiobuttonlist.SelectedValue = row[col.ColumnName].ToString();
53

}
54

else if (findControl is CheckBox)
55

{
56

CheckBox checkbox = (CheckBox)findControl;
57

checkbox.Checked = bool.Parse(row[col.ColumnName].ToString());
58

}
59

else if (findControl is CheckBoxList)
60

{
61

CheckBoxList checkboxlist = (CheckBoxList)findControl;
62

checkboxlist.SelectedValue = row[col.ColumnName].ToString();
63

}
64

else if (findControl is DropDownList)
65

{
66

DropDownList dropdownlist = (DropDownList)findControl;
67

dropdownlist.SelectedValue = row[col.ColumnName].ToString();
68

}
69

else if (findControl is Label)
70

{
71

Label label = (Label)findControl;
72

label.Text = row[col.ColumnName].ToString();
73

}
74

75

}
76

}
77

}
78

79

/// <summary>
80

/// Upload the value of the control to a datarow
81

/// </summary>
82

/// <param name="row"></param>
83

/// <param name="control"></param>
84

public static void UpdateData(DataRow row, Control control)
85

{
86

foreach (DataColumn col in row.Table.Columns)
87

{
88

string colName = col.ColumnName.Replace("_", "");
89

Control findControl;
90

findControl = control.FindControl(colName);
91

if (findControl != null)
92

{
93

if (findControl is TextBox)
94

{
95

TextBox textbox = (TextBox)findControl;
96

row[col.ColumnName] = textbox.Text;
97

}
98

else if (findControl is RadioButton)
99

{
100

RadioButton radiobutton = (RadioButton)findControl;
101

row[col.ColumnName] = radiobutton.Checked;
102

}
103

else if (findControl is RadioButtonList)
104

{
105

RadioButtonList radiobuttonlist = (RadioButtonList)findControl;
106

row[col.ColumnName] = radiobuttonlist.SelectedValue;
107

}
108

else if (findControl is CheckBox)
109

{
110

CheckBox checkbox = (CheckBox)findControl;
111

row[col.ColumnName] = checkbox.Checked;
112

}
113

else if (findControl is CheckBoxList)
114

{
115

CheckBoxList checkboxlist = (CheckBoxList)findControl;
116

row[col.ColumnName] = checkboxlist.SelectedValue;
117

}
118

else if (findControl is DropDownList)
119

{
120

DropDownList dropdownlist = (DropDownList)findControl;
121

row[col.ColumnName] = dropdownlist.SelectedValue;
122

}
123

else if (findControl is Label)
124

{
125

Label label = (Label)findControl;
126

row[col.ColumnName] = label.Text;
127

}
128

129

}
130

}
131

}
132

}
133

}
134

调用此方法:

1

using System;
2

using System.Collections;
3

using System.Configuration;
4

using System.Data;
5

using System.Linq;
6

using System.Web;
7

using System.Web.Security;
8

using System.Web.UI;
9

using System.Web.UI.HtmlControls;
10

using System.Web.UI.WebControls;
11

using System.Web.UI.WebControls.WebParts;
12

using System.Xml.Linq;
13

14

namespace Adrienne.WCF.WebUI
15

{
16

public partial class ControlsOpr : System.Web.UI.Page
17

{
18

protected void Page_Load(object sender, EventArgs e)
19

{
20

if (!IsPostBack)
21

{
22

//Create the Date
23

DataTable dt = new DataTable();
24

dt.Columns.Add("USER_NAME");
25

dt.Columns.Add("SEX");
26

DataRow row = dt.NewRow();
27

row["USER_NAME"] = "Xiong Wei";
28

row["SEX"] = "男";
29

dt.Rows.Add(row);
30

ViewState["dt"] = dt;
31

}
32

}
33

34

protected void Upload_Click(object sender, EventArgs e)
35

{
36

//Original method
37

if (ViewState["dt"] != null)
38

{
39

DataTable dt = ViewState["dt"] as DataTable;
40

////Should be check each column before
41

//this.UserName.Text = dt.Rows[0]["USER_NAME"] as string;
42

//this.Sex.SelectedValue = dt.Rows[0]["SEX"] as string;
43

PageHelper.FillPage(dt.Rows[0],this);
44

45

this.GridView1.DataSource = dt;
46

this.DataBind();
47

}
48

}
49

50

protected void Update_Click(object sender, EventArgs e)
51

{
52

if (ViewState["dt"] != null)
53

{
54

DataTable dt = ViewState["dt"] as DataTable;
55

//Should be check each column before
56

//dt.Rows[0]["USER_NAME"]=this.UserName.Text;
57

//dt.Rows[0]["SEX"]=this.Sex.SelectedValue;
58

PageHelper.UpdateData(dt.Rows[0], this);
59

60

this.GridView1.DataSource = dt;
61

this.DataBind();
62

}
63

}
64

}
65

}
66

通过上面的例子,我们知道,如果页面上有50个Control来做加载或更新数据的操作,只要用上面的两句话搞定。
其实我们在软件开发的过程中,如果发现一直在重复的写一定的代码,一样模式的代码,那么说明我的开发方式或都思想上有问题,或者说需要更新。就本例而言,其实这一个基础的操作,只是对一些基础控件的操作,并且操作的方式比较简单,在以后的时间里,我将写一些关于其它控件的操作。
对于一个程序员来说,他们最希望看到了就是如何可以减少他们的工作,也就是说使用最少的code,实现最多的function。在Asp.Net开发的过程中,对data controls的操作是不必不可少,最基本的功能就是对Controls data的加载及Controls选择或输入的值存储起来,也就是所谓的Load 和Update方法。而我们在平时的软件开发的过程中又是怎样来实现这些功能的呢?一般情况下我相信大家都和我之前一样,通过对页面中的每一个Controls做更新或加载,如下代码所示。
一.传统的操作模式
HTML 代码:

1

<%@ Page Language="C#" AutoEventWireup="true" CodeBehind="ControlsOpr.aspx.cs" Inherits="Adrienne.WCF.WebUI.ControlsOpr" %>
2

<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
3

<html xmlns="http://www.w3.org/1999/xhtml">
4

<head runat="server">
5

<title>Untitled Page</title>
6

</head>
7

<body>
8

<form id="form1" runat="server">
9

<div>
10

<dir>
11

用户名:<asp:TextBox ID="UserName" runat="server"></asp:TextBox>
12

</dir>
13

<dir>
14

性别:<asp:RadioButtonList ID="Sex" runat="server" RepeatColumns="2">
15

<asp:ListItem>男</asp:ListItem>
16

<asp:ListItem>女</asp:ListItem>
17

</asp:RadioButtonList>
18

<asp:Button ID="Upload" runat="server" Text="加载" onclick="Upload_Click" />
19

<asp:Button ID="Update" runat="server" Text="更新" onclick="Update_Click" />
20

</dir>
21

</div>
22

<asp:GridView ID="GridView1" runat="server">
23

</asp:GridView>
24

</form>
25

</body>
26

</html>
CS代码:

1

using System;
2

using System.Collections;
3

using System.Configuration;
4

using System.Data;
5

using System.Linq;
6

using System.Web;
7

using System.Web.Security;
8

using System.Web.UI;
9

using System.Web.UI.HtmlControls;
10

using System.Web.UI.WebControls;
11

using System.Web.UI.WebControls.WebParts;
12

using System.Xml.Linq;
13

14

namespace Adrienne.WCF.WebUI
15

{
16

public partial class ControlsOpr : System.Web.UI.Page
17

{
18

protected void Page_Load(object sender, EventArgs e)
19

{
20

if (!IsPostBack)
21

{
22

//Create the Date
23

DataTable dt = new DataTable();
24

dt.Columns.Add("USER_NAME");
25

dt.Columns.Add("SEX");
26

DataRow row = dt.NewRow();
27

row["USER_NAME"] = "Xiong Wei";
28

row["SEX"] = "男";
29

dt.Rows.Add(row);
30

ViewState["dt"] = dt;
31

}
32

}
33

34

protected void Upload_Click(object sender, EventArgs e)
35

{
36

//Original method
37

if (ViewState["dt"] != null)
38

{
39

DataTable dt = ViewState["dt"] as DataTable;
40

////Should be check each column before
41

this.UserName.Text = dt.Rows[0]["USER_NAME"] as string;
42

this.Sex.SelectedValue = dt.Rows[0]["SEX"] as string;
43

44

this.GridView1.DataSource = dt;
45

this.DataBind();
46

}
47

}
48

49

protected void Update_Click(object sender, EventArgs e)
50

{
51

if (ViewState["dt"] != null)
52

{
53

DataTable dt = ViewState["dt"] as DataTable;
54

//Should be check each column before
55

dt.Rows[0]["USER_NAME"]=this.UserName.Text;
56

dt.Rows[0]["SEX"]=this.Sex.SelectedValue;
57

58

this.GridView1.DataSource = dt;
59

this.DataBind();
60

}
61

}
62

}
63

}
界面如下:
点击Upload_Click
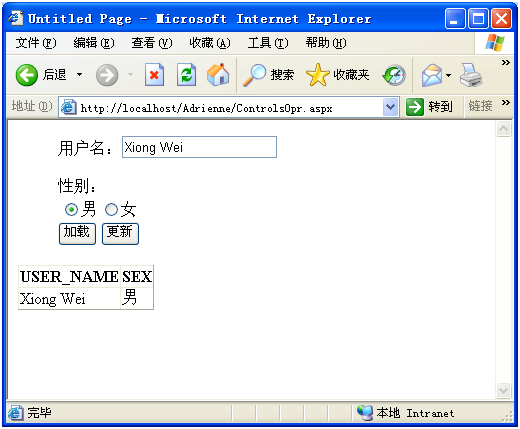
点击Update_Click
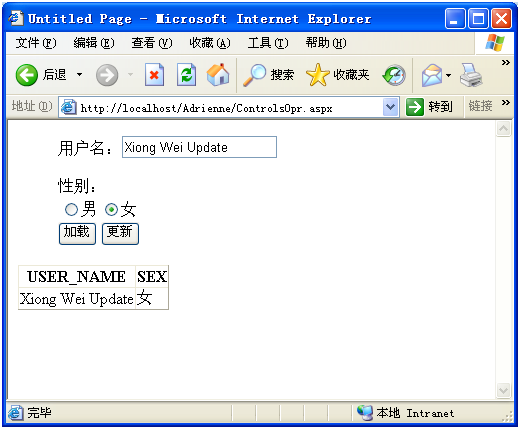
传统模式就是这样对页面中的数据进行操作的。
Upload_Click事件完成了对面页中控件值的加载;
Update_Click事件完成了把页面中控件的值更新到相应的数据控件中去。
我们可以来假设一下,如果存在这样一个项目,项目中存在大概有50个Page,每个Page当中大概有50个Control,这时就存在一个问题,我们如何对这些控件进行加载和保存数据呢,可想而知,上面的方法将存在巨大的工作量,针对上面方法的一个缺点,我们就可以考虑写一些Common的方法来实现上面的操作,从而优化操作模式,下面本人就自己的观点写了一段代码来优化上面的不足。
二.优化的操作模式
在本例中Controls比较少,假设每一个Page中有50个Controls,一个Project中有几十个Pages,那么请问,这个时候我们怎么做,难道还是每一个写一次吧,毋庸置疑,答案是否定的,那么我们又应该怎么了做呢?为此本人写了一个简单的类,这个类主要用来完成对控件值的加载以及对数据容器的更新。
操作类代码:

1

using System;
2

using System.Data;
3

using System.Configuration;
4

using System.Linq;
5

using System.Web;
6

using System.Web.Security;
7

using System.Web.UI;
8

using System.Web.UI.HtmlControls;
9

using System.Web.UI.WebControls;
10

using System.Web.UI.WebControls.WebParts;
11

using System.Xml.Linq;
12

using Microsoft.Practices.EnterpriseLibrary.Logging;
13

14

namespace Adrienne.WCF.WebUI
15

{
16

/// <summary>
17

/// Provide the operation for the controls of the pages
18

/// </summary>
19

public class PageHelper : PageBase
20

{
21

/// <summary>
22

/// Fill the data information to the controls of the web page
23

/// </summary>
24

/// <param name="row"></param>
25

/// <param name="control"></param>
26

public static void FillPage(DataRow row, Control control)
27

{
28

foreach (DataColumn col in row.Table.Columns)
29

{
30

string colName = col.ColumnName.Replace("_", "");
31

Control findControl;
32

findControl = control.FindControl(colName);
33

if (findControl != null)
34

{
35

if (row[col.ColumnName] == null)
36

{
37

continue;
38

}
39

if (findControl is TextBox)
40

{
41

TextBox textbox = (TextBox)findControl;
42

textbox.Text = row[col.ColumnName].ToString();
43

}
44

else if (findControl is RadioButton)
45

{
46

RadioButton radiobutton = (RadioButton)findControl;
47

radiobutton.Checked = bool.Parse(row[col.ColumnName].ToString());
48

}
49

else if (findControl is RadioButtonList)
50

{
51

RadioButtonList radiobuttonlist = (RadioButtonList)findControl;
52

radiobuttonlist.SelectedValue = row[col.ColumnName].ToString();
53

}
54

else if (findControl is CheckBox)
55

{
56

CheckBox checkbox = (CheckBox)findControl;
57

checkbox.Checked = bool.Parse(row[col.ColumnName].ToString());
58

}
59

else if (findControl is CheckBoxList)
60

{
61

CheckBoxList checkboxlist = (CheckBoxList)findControl;
62

checkboxlist.SelectedValue = row[col.ColumnName].ToString();
63

}
64

else if (findControl is DropDownList)
65

{
66

DropDownList dropdownlist = (DropDownList)findControl;
67

dropdownlist.SelectedValue = row[col.ColumnName].ToString();
68

}
69

else if (findControl is Label)
70

{
71

Label label = (Label)findControl;
72

label.Text = row[col.ColumnName].ToString();
73

}
74

75

}
76

}
77

}
78

79

/// <summary>
80

/// Upload the value of the control to a datarow
81

/// </summary>
82

/// <param name="row"></param>
83

/// <param name="control"></param>
84

public static void UpdateData(DataRow row, Control control)
85

{
86

foreach (DataColumn col in row.Table.Columns)
87

{
88

string colName = col.ColumnName.Replace("_", "");
89

Control findControl;
90

findControl = control.FindControl(colName);
91

if (findControl != null)
92

{
93

if (findControl is TextBox)
94

{
95

TextBox textbox = (TextBox)findControl;
96

row[col.ColumnName] = textbox.Text;
97

}
98

else if (findControl is RadioButton)
99

{
100

RadioButton radiobutton = (RadioButton)findControl;
101

row[col.ColumnName] = radiobutton.Checked;
102

}
103

else if (findControl is RadioButtonList)
104

{
105

RadioButtonList radiobuttonlist = (RadioButtonList)findControl;
106

row[col.ColumnName] = radiobuttonlist.SelectedValue;
107

}
108

else if (findControl is CheckBox)
109

{
110

CheckBox checkbox = (CheckBox)findControl;
111

row[col.ColumnName] = checkbox.Checked;
112

}
113

else if (findControl is CheckBoxList)
114

{
115

CheckBoxList checkboxlist = (CheckBoxList)findControl;
116

row[col.ColumnName] = checkboxlist.SelectedValue;
117

}
118

else if (findControl is DropDownList)
119

{
120

DropDownList dropdownlist = (DropDownList)findControl;
121

row[col.ColumnName] = dropdownlist.SelectedValue;
122

}
123

else if (findControl is Label)
124

{
125

Label label = (Label)findControl;
126

row[col.ColumnName] = label.Text;
127

}
128

129

}
130

}
131

}
132

}
133

}
134

调用此方法:

1

using System;
2

using System.Collections;
3

using System.Configuration;
4

using System.Data;
5

using System.Linq;
6

using System.Web;
7

using System.Web.Security;
8

using System.Web.UI;
9

using System.Web.UI.HtmlControls;
10

using System.Web.UI.WebControls;
11

using System.Web.UI.WebControls.WebParts;
12

using System.Xml.Linq;
13

14

namespace Adrienne.WCF.WebUI
15

{
16

public partial class ControlsOpr : System.Web.UI.Page
17

{
18

protected void Page_Load(object sender, EventArgs e)
19

{
20

if (!IsPostBack)
21

{
22

//Create the Date
23

DataTable dt = new DataTable();
24

dt.Columns.Add("USER_NAME");
25

dt.Columns.Add("SEX");
26

DataRow row = dt.NewRow();
27

row["USER_NAME"] = "Xiong Wei";
28

row["SEX"] = "男";
29

dt.Rows.Add(row);
30

ViewState["dt"] = dt;
31

}
32

}
33

34

protected void Upload_Click(object sender, EventArgs e)
35

{
36

//Original method
37

if (ViewState["dt"] != null)
38

{
39

DataTable dt = ViewState["dt"] as DataTable;
40

////Should be check each column before
41

//this.UserName.Text = dt.Rows[0]["USER_NAME"] as string;
42

//this.Sex.SelectedValue = dt.Rows[0]["SEX"] as string;
43

PageHelper.FillPage(dt.Rows[0],this);
44

45

this.GridView1.DataSource = dt;
46

this.DataBind();
47

}
48

}
49

50

protected void Update_Click(object sender, EventArgs e)
51

{
52

if (ViewState["dt"] != null)
53

{
54

DataTable dt = ViewState["dt"] as DataTable;
55

//Should be check each column before
56

//dt.Rows[0]["USER_NAME"]=this.UserName.Text;
57

//dt.Rows[0]["SEX"]=this.Sex.SelectedValue;
58

PageHelper.UpdateData(dt.Rows[0], this);
59

60

this.GridView1.DataSource = dt;
61

this.DataBind();
62

}
63

}
64

}
65

}
66

通过上面的例子,我们知道,如果页面上有50个Control来做加载或更新数据的操作,只要用上面的两句话搞定。
其实我们在软件开发的过程中,如果发现一直在重复的写一定的代码,一样模式的代码,那么说明我的开发方式或都思想上有问题,或者说需要更新。就本例而言,其实这一个基础的操作,只是对一些基础控件的操作,并且操作的方式比较简单,在以后的时间里,我将写一些关于其它控件的操作。
相关文章推荐
- Skyline软件二次开发初级——1如何在web页面中添加控件和加载三维地图数据
- Asp.net开发心得点滴[动态加载的用户控件使用事件委托,交给页面处理的事件无效问题]
- ASP.NET基础教程-使用CommandBuilder对象自动生成SQL语句对数据进行批量更新
- ASP.Net MVC开发基础学习笔记(8):新建数据页面
- ASP.Net MVC开发基础学习笔记(8):新建数据页面
- SolpartMenu的使用:(二)、在ASP.NET页面中使用SolpartMenu控件之动态的加载数据库中的数据来生成菜单
- asp.net控件开发基础(17) --------初识数据绑定控件
- asp.net的服务器控件客户端空件的区别以及如何刷新页面不靠数据库数据停留在页面
- SolpartMenu的使用:(二)、在ASP.NET页面中使用SolpartMenu控件之动态的加载数据库中的数据来生成菜单
- asp.net控件开发基础(19) --------数据列表绑定控件
- VS2008 在进行ASP.NET 开发时 如何避免加载设计页面时假死及减少保存所耗的时间。
- asp.net如何将页面Table控件中的数据写到excel中总结
- Asp.net自定义服务器控件开发小技巧: 如何正确获得回传数据
- asp.net控件开发基础(17) --------初识数据绑定控件
- asp.net控件开发基础(17) --------初识数据绑定控件
- ASP.Net MVC开发基础学习笔记(7):数据查询页面
- Asp.net自定义服务器控件开发小技巧: 如何正确获得回传数据
- asp.net控件开发基础(1)
- HTML控件ID和NAME属性的区别,以及如何在asp.net页面的.CS文件中获得.ASPX页面中HTML控件的值
- 页面使用asp.net验证控件在提交数据时无响应