how to integrate ASP.NET codes inside SharePoint
2008-04-18 14:07
316 查看
You definitely won't be able to do code-behind in SharePoint. However, here is a workaround to get inline code to work:
- "An error occurred during the processing of xxx.aspx. Code blocks are not allowed in this file."
- "This page has encountered a critical error. contact your system administrator if this problem persist."
Example inline code
==================================
Open up SharePoint Designer and the masterpage you want to edit. Switch to the code view and anywhere from the first <head> tag down you can add some inline c# code:
<script runat="server">
string myVar = "hello world!";
void Page_Load(object sender, System.EventArgs e)
{
Response.Write(myVar);
SPSite siteCollection = new SPSite("http://[sharepoint]");
SPWeb site = siteCollection.OpenWeb("/[subsite]/");
SPList list = site.Lists["Announcements"];
SPListItemCollection items = list.Items;
foreach(SPListItem item in items)
{
Response.Write(item["Title"].ToString());
Response.Write("<br/>");
}
}
</script>
This shows how you can use the SharePoint object model in your inline code. If you saved the master page now and opened the site in your browser you would get an error about not being able to run code blocks (listed above). There’s an extra line you need to put into your web.config file first to allow this inline c# to execute.
Modify the web.config file
==================================
1. Open the web.config file and make the following change:
<PageParserPaths>
<PageParserPath VirtualPath="/_catalogs/masterpage/*" CompilationMode="Always" AllowServerSideScript="true" IncludeSubFolders="true" />
</PageParserPaths>
The PageParserPaths xml tags will be there already you just need to add the line in between.
2. Save web.config .
3. Start -> Run -> iisreset /noforce , click OK.
Note: I would like to point out that making this change is possibly not good practice. The fact that CompilationMode being set to "Always" points out that there may be a performance hit and caching won’t exactly work well.
http://forums.microsoft.com/TechNet/ShowPost.aspx?PostID=2779241&SiteID=17&mode=1
我们往往会碰到这样的需求:
我们可以创建aspx页面,将其部署到12\TEMPLATE\LAYOUTS目录下,并通过feature的方式来安装(如果有DLL的话,拷贝到对应的SharePoint站点的\bin目录中)。
这样做没有什么问题,但是_layouts目录是所有站点都可以访问的。我需要达到这样的效果: http://mossdemo/onesite/customapp/custompage1.aspx
而现在的实现,却是:
http://mossdemo/onesite/_layouts/custompage1.apsx
而且,更严重的是,如果我这么试:
http://mossdemo/anothersite/_layouts/custompage1.aspx
同样起作用。但事实上我只在onesite上安装的该feature。
解决办法:
假设我们的feature目录叫做customapp,完整的路径为 C:\Program Files\Common Files\Microsoft Shared\web server extensions\12\TEMPLATE\FEATURES\customapp
下面有如下的内容:
2个文件
feature.xml
elements.xml
1个文件夹,叫custompages。用来存放我们的aspx页面。其中有两个页面文件:
custompage1.aspx
custompage2.aspx
我们可以这样组织feature.xml内容:
<?xml version="1.0" encoding="utf-8" ?>
<feature id="{AAA4124D-2A89-43df-8427-F820D7B20CC9}" title="FileUploadDemo" xmlns="http://schemas.microsoft.com/sharepoint/" hidden="FALSE" scope="Web" version="1.0.0.0" description="Loads a file into sharepoint site" creator="">
<elementmanifests>
<elementmanifest location="elements.xml" />
<elementfile location="custompages\custompage1.aspx" />
<elementfile location="custompages\custompage2.aspx" />
</elementmanifests>
</feature>
elements.xml内容如下:
<?xml version="1.0" encoding="utf-8" ?>
<elements xmlns="http://schemas.microsoft.com/sharepoint/">
<module path="custompages" name="CustomPages">
<file path="custompage1.aspx" name="" type="Ghostable" url="custompages/custompage1.aspx" />
<file path="custompage2.aspx" name="" type="Ghostable" url="custompages/custompage2.aspx" />
</module>
</elements>
为了测试,custompage1.aspx的内容很简单,只是显示SPWeb的Title。内容如下:
<%@ Assembly Name="Microsoft.SharePoint.ApplicationPages, Version=12.0.0.0, Culture=neutral, PublicKeyToken=71e9bce111e9429c" %>
<%@ Page Language="C#"
MasterPageFile="~masterurl/default.master" %>
<%@ Import Namespace="Microsoft.SharePoint.ApplicationPages" %>
<%@ Register TagPrefix="SharePoint" Namespace="Microsoft.SharePoint.WebControls"
Assembly="Microsoft.SharePoint, Version=12.0.0.0, Culture=neutral, PublicKeyToken=71e9bce111e9429c" %>
<%@ Register TagPrefix="Utilities" Namespace="Microsoft.SharePoint.Utilities" Assembly="Microsoft.SharePoint, Version=12.0.0.0, Culture=neutral, PublicKeyToken=71e9bce111e9429c" %>
<%@ Import Namespace="Microsoft.SharePoint" %>
<%@ Register TagPrefix="SharePoint" Namespace="Microsoft.SharePoint.WebControls"
Assembly="Microsoft.SharePoint, Version=12.0.0.0, Culture=neutral, PublicKeyToken=71e9bce111e9429c" %>
<%@ Register TagPrefix="Utilities" Namespace="Microsoft.SharePoint.Utilities" Assembly="Microsoft.SharePoint, Version=12.0.0.0, Culture=neutral, PublicKeyToken=71e9bce111e9429c" %>
<%@ Import Namespace="Microsoft.SharePoint" %>
<script runat="server">
protected void Page_Load(object sender, EventArgs e)
{
this.lbtest.Text = SPContext.Current.Web.Title;
}
</script>
<asp:Content ID="Content1" ContentPlaceHolderID="PlaceHolderPageTitle" runat="server">
<SharePoint:EncodedLiteral ID="EncodedLiteral1" runat="server" Text="自定义页1"
EncodeMethod='HtmlEncode' />
</asp:Content>
<asp:Content ID="Content2" ContentPlaceHolderID="PlaceHolderPageTitleInTitleArea"
runat="server">
<SharePoint:EncodedLiteral ID="EncodedLiteral2" runat="server" Text="定制内容"
EncodeMethod='HtmlEncode' />
</asp:Content>
<asp:Content ID="Content3" ContentPlaceHolderID="PlaceHolderAdditionalPageHead" runat="server">
<meta name="Robots" content="NOINDEX " />
<meta name="SharePointError" content="" />
</asp:Content>
<asp:Content ID="Content4" ContentPlaceHolderID="PlaceHolderMain" runat="server">
<table width="100%" border="0" class="ms-titleareaframe" cellpadding="0">
<tr>
<td valign="top" width="100%" style="padding-top: 10px" class="ms-descriptiontext">
<span class="ms-descriptiontext">
<asp:Label ID="lbtest" runat="server" Text=""></asp:Label>
</span>
</td>
</tr>
</table>
</asp:Content>
一切就绪后,安装feature:
stsadm -o installfeature -name customapp
然后到某个SharePoint站点(如http://mossdemo/onesite)上,激活该网站功能(名为FileUploadDemo的就是)。
现在,就可以通过如下的方式访问我们的aspx页面了:
http://mossdemo/onesite/custompages/custompage1.aspx
发个图
- "An error occurred during the processing of xxx.aspx. Code blocks are not allowed in this file."
- "This page has encountered a critical error. contact your system administrator if this problem persist."
Example inline code
==================================
Open up SharePoint Designer and the masterpage you want to edit. Switch to the code view and anywhere from the first <head> tag down you can add some inline c# code:
<script runat="server">
string myVar = "hello world!";
void Page_Load(object sender, System.EventArgs e)
{
Response.Write(myVar);
SPSite siteCollection = new SPSite("http://[sharepoint]");
SPWeb site = siteCollection.OpenWeb("/[subsite]/");
SPList list = site.Lists["Announcements"];
SPListItemCollection items = list.Items;
foreach(SPListItem item in items)
{
Response.Write(item["Title"].ToString());
Response.Write("<br/>");
}
}
</script>
This shows how you can use the SharePoint object model in your inline code. If you saved the master page now and opened the site in your browser you would get an error about not being able to run code blocks (listed above). There’s an extra line you need to put into your web.config file first to allow this inline c# to execute.
Modify the web.config file
==================================
1. Open the web.config file and make the following change:
<PageParserPaths>
<PageParserPath VirtualPath="/_catalogs/masterpage/*" CompilationMode="Always" AllowServerSideScript="true" IncludeSubFolders="true" />
</PageParserPaths>
The PageParserPaths xml tags will be there already you just need to add the line in between.
2. Save web.config .
3. Start -> Run -> iisreset /noforce , click OK.
Note: I would like to point out that making this change is possibly not good practice. The fact that CompilationMode being set to "Always" points out that there may be a performance hit and caching won’t exactly work well.
http://forums.microsoft.com/TechNet/ShowPost.aspx?PostID=2779241&SiteID=17&mode=1
我们往往会碰到这样的需求:
我们可以创建aspx页面,将其部署到12\TEMPLATE\LAYOUTS目录下,并通过feature的方式来安装(如果有DLL的话,拷贝到对应的SharePoint站点的\bin目录中)。
这样做没有什么问题,但是_layouts目录是所有站点都可以访问的。我需要达到这样的效果: http://mossdemo/onesite/customapp/custompage1.aspx
而现在的实现,却是:
http://mossdemo/onesite/_layouts/custompage1.apsx
而且,更严重的是,如果我这么试:
http://mossdemo/anothersite/_layouts/custompage1.aspx
同样起作用。但事实上我只在onesite上安装的该feature。
解决办法:
假设我们的feature目录叫做customapp,完整的路径为 C:\Program Files\Common Files\Microsoft Shared\web server extensions\12\TEMPLATE\FEATURES\customapp
下面有如下的内容:
2个文件
feature.xml
elements.xml
1个文件夹,叫custompages。用来存放我们的aspx页面。其中有两个页面文件:
custompage1.aspx
custompage2.aspx
我们可以这样组织feature.xml内容:
<?xml version="1.0" encoding="utf-8" ?>
<feature id="{AAA4124D-2A89-43df-8427-F820D7B20CC9}" title="FileUploadDemo" xmlns="http://schemas.microsoft.com/sharepoint/" hidden="FALSE" scope="Web" version="1.0.0.0" description="Loads a file into sharepoint site" creator="">
<elementmanifests>
<elementmanifest location="elements.xml" />
<elementfile location="custompages\custompage1.aspx" />
<elementfile location="custompages\custompage2.aspx" />
</elementmanifests>
</feature>
elements.xml内容如下:
<?xml version="1.0" encoding="utf-8" ?>
<elements xmlns="http://schemas.microsoft.com/sharepoint/">
<module path="custompages" name="CustomPages">
<file path="custompage1.aspx" name="" type="Ghostable" url="custompages/custompage1.aspx" />
<file path="custompage2.aspx" name="" type="Ghostable" url="custompages/custompage2.aspx" />
</module>
</elements>
为了测试,custompage1.aspx的内容很简单,只是显示SPWeb的Title。内容如下:
<%@ Assembly Name="Microsoft.SharePoint.ApplicationPages, Version=12.0.0.0, Culture=neutral, PublicKeyToken=71e9bce111e9429c" %>
<%@ Page Language="C#"
MasterPageFile="~masterurl/default.master" %>
<%@ Import Namespace="Microsoft.SharePoint.ApplicationPages" %>
<%@ Register TagPrefix="SharePoint" Namespace="Microsoft.SharePoint.WebControls"
Assembly="Microsoft.SharePoint, Version=12.0.0.0, Culture=neutral, PublicKeyToken=71e9bce111e9429c" %>
<%@ Register TagPrefix="Utilities" Namespace="Microsoft.SharePoint.Utilities" Assembly="Microsoft.SharePoint, Version=12.0.0.0, Culture=neutral, PublicKeyToken=71e9bce111e9429c" %>
<%@ Import Namespace="Microsoft.SharePoint" %>
<%@ Register TagPrefix="SharePoint" Namespace="Microsoft.SharePoint.WebControls"
Assembly="Microsoft.SharePoint, Version=12.0.0.0, Culture=neutral, PublicKeyToken=71e9bce111e9429c" %>
<%@ Register TagPrefix="Utilities" Namespace="Microsoft.SharePoint.Utilities" Assembly="Microsoft.SharePoint, Version=12.0.0.0, Culture=neutral, PublicKeyToken=71e9bce111e9429c" %>
<%@ Import Namespace="Microsoft.SharePoint" %>
<script runat="server">
protected void Page_Load(object sender, EventArgs e)
{
this.lbtest.Text = SPContext.Current.Web.Title;
}
</script>
<asp:Content ID="Content1" ContentPlaceHolderID="PlaceHolderPageTitle" runat="server">
<SharePoint:EncodedLiteral ID="EncodedLiteral1" runat="server" Text="自定义页1"
EncodeMethod='HtmlEncode' />
</asp:Content>
<asp:Content ID="Content2" ContentPlaceHolderID="PlaceHolderPageTitleInTitleArea"
runat="server">
<SharePoint:EncodedLiteral ID="EncodedLiteral2" runat="server" Text="定制内容"
EncodeMethod='HtmlEncode' />
</asp:Content>
<asp:Content ID="Content3" ContentPlaceHolderID="PlaceHolderAdditionalPageHead" runat="server">
<meta name="Robots" content="NOINDEX " />
<meta name="SharePointError" content="" />
</asp:Content>
<asp:Content ID="Content4" ContentPlaceHolderID="PlaceHolderMain" runat="server">
<table width="100%" border="0" class="ms-titleareaframe" cellpadding="0">
<tr>
<td valign="top" width="100%" style="padding-top: 10px" class="ms-descriptiontext">
<span class="ms-descriptiontext">
<asp:Label ID="lbtest" runat="server" Text=""></asp:Label>
</span>
</td>
</tr>
</table>
</asp:Content>
一切就绪后,安装feature:
stsadm -o installfeature -name customapp
然后到某个SharePoint站点(如http://mossdemo/onesite)上,激活该网站功能(名为FileUploadDemo的就是)。
现在,就可以通过如下的方式访问我们的aspx页面了:
http://mossdemo/onesite/custompages/custompage1.aspx
发个图
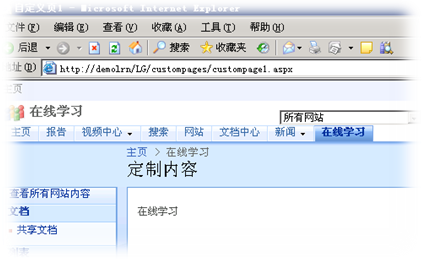
相关文章推荐
- How to enable an ASP.Net application to run on a SharePoint virtual server
- How to put asp.net file to sharepoint
- How to Share Session State Between Classic ASP and ASP.NET
- Deploying an ASP.NET HttpHandler to SharePoint 2010
- Web Parts: From SharePoint to ASP.NET 2.0
- [转]How to Integrate SharePoint 2010 with Reporting Services
- How to Share Session/Application State Across Different ASP.NET Web Applications
- ASP.NET WebUserControl to SharePoint WebPart Best Practice (vs2008)
- How to Integrate SAP Business Data Into SharePoint 2010 Using Business Connectivity Services and LINQ to SAP
- How to integrate custom security policy with Windows domain authentication in ASP.NET
- How to Configure Workflow Infrastructure 2013 in Sharepoint 2013
- How to Export & Import Web Parts in SharePoint(转)
- How to install ASP.NET 1.1 with IIS7 on Vista and Windows 2008
- How to use Asp.Net Mvc ActionFilterAttribute for form authentication
- How to install SharePoint 2010 on Windows 7
- How to Back up and restore SharePoint 2013 using a VSS requestor
- How to Host ASP.Net Web API on IIS Server
- How to install sharepoint server 2010 sp2 in window 7 x64
- How to solve second button click download problem on SharePoint 2010
- How to fix intellisense issues after upgrading to ASP.NET Ajax 1.0 RC