Ajax实现二级联动下拉框!
2007-01-23 11:20
399 查看
这是一个Ajax的经典示例,也是Ajax的长处所在。不多说了,下面来看代码。
项目结构图:
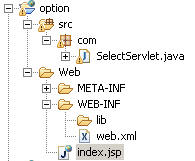
index.jsp:
<%@ page language="java" contentType="text/html; charset=utf-8"%>
<html>
<head>
<title>My JSP 'index.jsp' starting page</title>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8">
<SCRIPT type="text/javascript">
var _xmlHttpRequestObj;
window.onload=function(){
}
function Change_Select()
{
var zhi=document.getElementById('hero').value;
var url="servlet/SelectServlet?id="+encodeURIComponent(escape(zhi));
_xmlHttpRequestObj=true;
try {
_xmlHttpRequestObj = new ActiveXObject("Msxml2.XMLHTTP");
} catch (e) {
try {
_xmlHttpRequestObj = new ActiveXObject("Microsoft.XMLHTTP");
} catch (E) {
_xmlHttpRequestObj = false;
}
}
if (!_xmlHttpRequestObj && typeof XMLHttpRequest!='undefined') {
try {
_xmlHttpRequestObj = new XMLHttpRequest();
} catch (e) {
_xmlHttpRequestObj=false;
}
}
if (!_xmlHttpRequestObj && window.createRequest) {
try {
_xmlHttpRequestObj = window.createRequest();
_xmlHttpRequestObj.overrideMimeType('text/xml');//设置MiME类别.
} catch (e) {
_xmlHttpRequestObj=false;
}
}
if(_xmlHttpRequestObj)
{
_xmlHttpRequestObj.open("POST",url,true);
_xmlHttpRequestObj.onreadystatechange=callback;
_xmlHttpRequestObj.send();
}
}
function callback()
{
if(_xmlHttpRequestObj.readyState == 4)
{
if(_xmlHttpRequestObj.status == 200)
{
parseMessage();
}else{
alert("Not able to retrieve description"+req.statusText);
}
}
}
function parseMessage()
{
var xmlDoc=_xmlHttpRequestObj.responseXML.documentElement;
var xSel=xmlDoc.getElementsByTagName('select');
var select_root=document.getElementById('skill');
select_root.options.length=0;
for(var i=0;i<xSel.length;i++)
{
var xValue=xSel[i].childNodes[0].firstChild.nodeValue;
var xText=xSel[i].childNodes[1].firstChild.nodeValue;
var option=new Option(xText,xValue);
try{
select_root.add(option);
}catch(e){
}
}
}
</SCRIPT>
</head>
<body>
<div align="center">
<form name="form1" method="post" action="">
<TABLE width="70%" boder="0" cellspacing="0">
<TR>
<TD align="center">Double Select Box</TD>
</TR>
<TR>
<TD>
<SELECT name="hero" id="hero" onChange="Change_Select()">
<OPTION value="0">Unbounded</OPTION>
<OPTION value="1">D.K.</OPTION>
<OPTION value="2">NEC.</OPTION>
<OPTION value="3">BOSS</OPTION>
</SELECT>
<SELECT name="skill" id="skill">
<OPTION value="0">Unbounded</OPTION>
</SELECT>
</TD>
</TR>
<TR><td> </td></TR>
</TABLE>
</form>
</div>
</body>
</html>
SelectServlet.java:
package com;
import java.io.IOException;
import java.io.PrintWriter;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
public class SelectServlet extends HttpServlet {
/**
* Constructor of the object.
*/
public SelectServlet() {
super();
}
/**
* Destruction of the servlet. <br>
*/
public void destroy() {
super.destroy(); // Just puts "destroy" string in log
// Put your code here
}
/**
* The doGet method of the servlet. <br>
*
* This method is called when a form has its tag value method equals to get.
*
* @param request the request send by the client to the server
* @param response the response send by the server to the client
* @throws ServletException if an error occurred
* @throws IOException if an error occurred
*/
public void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
doPost(request,response);
}
/**
* The doPost method of the servlet. <br>
*
* This method is called when a form has its tag value method equals to post.
*
* @param request the request send by the client to the server
* @param response the response send by the server to the client
* @throws ServletException if an error occurred
* @throws IOException if an error occurred
*/
public void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
response.setContentType("text/xml");
response.setHeader("Cache-Control","no-cache");
String targetId=request.getParameter("id").toString();
String xml_start="<selects>";
String xml_end="</selects>";
String xml="";
if(targetId.equalsIgnoreCase("0")){
xml="<select><value>0</value><text>Unbounded</text></select>";
}else if(targetId.equalsIgnoreCase("1")){
xml="<select><value>1</value><text>Mana Burn</text></select>";
xml +="<select><value>2</value><text>Death Coil</text></select>";
xml +="<select><value>3</value><text>Unholy Aura</text></select>";
xml +="<select><value>4</value><text>Unholy Fire</text></select>";
}else if(targetId.equalsIgnoreCase("2")){
xml="<select><value>1</value><text>Corprxplode</text></select>";
xml +="<select><value>2</value><text>Raise Dead</text></select>";
xml +="<select><value>3</value><text>Brilliance Aura</text></select>";
xml +="<select><value>4</value><text>Aim Aura</text></select>";
}else{
xml="<select><value>1</value><text>Rain of Chaos</text></select>";
xml +="<select><value>2</value><text>Finger of Death</text></select>";
xml +="<select><value>3</value><text>Bash</text></select>";
xml +="<select><value>4</value><text>Summon Doom</text></select>";
}
String last_xml=xml_start+xml+xml_end;
response.getWriter().write(last_xml);
}
/**
* Initialization of the servlet. <br>
*
* @throws ServletException if an error occure
*/
public void init() throws ServletException {
// Put your code here
}
}
web.xml:
<?xml version="1.0" encoding="UTF-8"?>
<web-app version="2.4"
xmlns="http://java.sun.com/xml/ns/j2ee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://java.sun.com/xml/ns/j2ee http://java.sun.com/xml/ns/j2ee/web-app_2_4.xsd"> <servlet>
<servlet-name>SelectServlet</servlet-name>
<servlet-class>com.SelectServlet</servlet-class>
</servlet>
<servlet-mapping>
<servlet-name>SelectServlet</servlet-name>
<url-pattern>/servlet/SelectServlet</url-pattern>
</servlet-mapping>
<welcome-file-list>
<welcome-file>index.jsp</welcome-file>
</welcome-file-list>
</web-app>
运行结果图:
项目结构图:
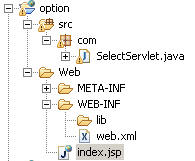
index.jsp:
<%@ page language="java" contentType="text/html; charset=utf-8"%>
<html>
<head>
<title>My JSP 'index.jsp' starting page</title>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8">
<SCRIPT type="text/javascript">
var _xmlHttpRequestObj;
window.onload=function(){
}
function Change_Select()
{
var zhi=document.getElementById('hero').value;
var url="servlet/SelectServlet?id="+encodeURIComponent(escape(zhi));
_xmlHttpRequestObj=true;
try {
_xmlHttpRequestObj = new ActiveXObject("Msxml2.XMLHTTP");
} catch (e) {
try {
_xmlHttpRequestObj = new ActiveXObject("Microsoft.XMLHTTP");
} catch (E) {
_xmlHttpRequestObj = false;
}
}
if (!_xmlHttpRequestObj && typeof XMLHttpRequest!='undefined') {
try {
_xmlHttpRequestObj = new XMLHttpRequest();
} catch (e) {
_xmlHttpRequestObj=false;
}
}
if (!_xmlHttpRequestObj && window.createRequest) {
try {
_xmlHttpRequestObj = window.createRequest();
_xmlHttpRequestObj.overrideMimeType('text/xml');//设置MiME类别.
} catch (e) {
_xmlHttpRequestObj=false;
}
}
if(_xmlHttpRequestObj)
{
_xmlHttpRequestObj.open("POST",url,true);
_xmlHttpRequestObj.onreadystatechange=callback;
_xmlHttpRequestObj.send();
}
}
function callback()
{
if(_xmlHttpRequestObj.readyState == 4)
{
if(_xmlHttpRequestObj.status == 200)
{
parseMessage();
}else{
alert("Not able to retrieve description"+req.statusText);
}
}
}
function parseMessage()
{
var xmlDoc=_xmlHttpRequestObj.responseXML.documentElement;
var xSel=xmlDoc.getElementsByTagName('select');
var select_root=document.getElementById('skill');
select_root.options.length=0;
for(var i=0;i<xSel.length;i++)
{
var xValue=xSel[i].childNodes[0].firstChild.nodeValue;
var xText=xSel[i].childNodes[1].firstChild.nodeValue;
var option=new Option(xText,xValue);
try{
select_root.add(option);
}catch(e){
}
}
}
</SCRIPT>
</head>
<body>
<div align="center">
<form name="form1" method="post" action="">
<TABLE width="70%" boder="0" cellspacing="0">
<TR>
<TD align="center">Double Select Box</TD>
</TR>
<TR>
<TD>
<SELECT name="hero" id="hero" onChange="Change_Select()">
<OPTION value="0">Unbounded</OPTION>
<OPTION value="1">D.K.</OPTION>
<OPTION value="2">NEC.</OPTION>
<OPTION value="3">BOSS</OPTION>
</SELECT>
<SELECT name="skill" id="skill">
<OPTION value="0">Unbounded</OPTION>
</SELECT>
</TD>
</TR>
<TR><td> </td></TR>
</TABLE>
</form>
</div>
</body>
</html>
SelectServlet.java:
package com;
import java.io.IOException;
import java.io.PrintWriter;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
public class SelectServlet extends HttpServlet {
/**
* Constructor of the object.
*/
public SelectServlet() {
super();
}
/**
* Destruction of the servlet. <br>
*/
public void destroy() {
super.destroy(); // Just puts "destroy" string in log
// Put your code here
}
/**
* The doGet method of the servlet. <br>
*
* This method is called when a form has its tag value method equals to get.
*
* @param request the request send by the client to the server
* @param response the response send by the server to the client
* @throws ServletException if an error occurred
* @throws IOException if an error occurred
*/
public void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
doPost(request,response);
}
/**
* The doPost method of the servlet. <br>
*
* This method is called when a form has its tag value method equals to post.
*
* @param request the request send by the client to the server
* @param response the response send by the server to the client
* @throws ServletException if an error occurred
* @throws IOException if an error occurred
*/
public void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
response.setContentType("text/xml");
response.setHeader("Cache-Control","no-cache");
String targetId=request.getParameter("id").toString();
String xml_start="<selects>";
String xml_end="</selects>";
String xml="";
if(targetId.equalsIgnoreCase("0")){
xml="<select><value>0</value><text>Unbounded</text></select>";
}else if(targetId.equalsIgnoreCase("1")){
xml="<select><value>1</value><text>Mana Burn</text></select>";
xml +="<select><value>2</value><text>Death Coil</text></select>";
xml +="<select><value>3</value><text>Unholy Aura</text></select>";
xml +="<select><value>4</value><text>Unholy Fire</text></select>";
}else if(targetId.equalsIgnoreCase("2")){
xml="<select><value>1</value><text>Corprxplode</text></select>";
xml +="<select><value>2</value><text>Raise Dead</text></select>";
xml +="<select><value>3</value><text>Brilliance Aura</text></select>";
xml +="<select><value>4</value><text>Aim Aura</text></select>";
}else{
xml="<select><value>1</value><text>Rain of Chaos</text></select>";
xml +="<select><value>2</value><text>Finger of Death</text></select>";
xml +="<select><value>3</value><text>Bash</text></select>";
xml +="<select><value>4</value><text>Summon Doom</text></select>";
}
String last_xml=xml_start+xml+xml_end;
response.getWriter().write(last_xml);
}
/**
* Initialization of the servlet. <br>
*
* @throws ServletException if an error occure
*/
public void init() throws ServletException {
// Put your code here
}
}
web.xml:
<?xml version="1.0" encoding="UTF-8"?>
<web-app version="2.4"
xmlns="http://java.sun.com/xml/ns/j2ee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://java.sun.com/xml/ns/j2ee http://java.sun.com/xml/ns/j2ee/web-app_2_4.xsd"> <servlet>
<servlet-name>SelectServlet</servlet-name>
<servlet-class>com.SelectServlet</servlet-class>
</servlet>
<servlet-mapping>
<servlet-name>SelectServlet</servlet-name>
<url-pattern>/servlet/SelectServlet</url-pattern>
</servlet-mapping>
<welcome-file-list>
<welcome-file>index.jsp</welcome-file>
</welcome-file-list>
</web-app>
运行结果图:
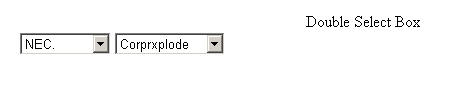
相关文章推荐
- Ajax实现二级联动下拉框!
- Ajax实现二级联动下拉框
- 利用ajax实现二级联动下拉框
- Ajax实现二级联动下拉框
- Ajax实现二级联动下拉框
- 使用ajax+json+struts实现省份下拉框二级联动
- Ajax实现的城市二级联动二
- Ajax实现的城市二级联动三
- AjaxPro.2.dll实现省市的二级无刷新联动
- Ajax实现省市二级联动(源代码)
- Rails——下拉框二级联动实现省、市选择
- Ajax联动下拉框的实现例子
- Ajax实现二级联动菜单
- 用AjaxPro实现二级联动
- 在laravel中使用ajax实现二级联动
- asp.net DropDownList无刷新ajax二级联动实现详细过程
- 初学ajax,实现用户名重复提示、二级/三级联动下拉框
- javascript实现二级联动下拉框
- ajax与json实现省市二级联动
- 用ajax(vb.net) 实现dropdownlist二级无刷新联动~!