单链表的基础实现
2006-03-23 09:36
267 查看
单链表的基础实现
时间:2006/03/23
测试环境:TC2.0
运行后结果:
时间:2006/03/23
测试环境:TC2.0
#include <stdio.h> #define LEN sizeof(struct LNode) #define NULL 0 typedef int ElemType; struct LNode { ElemType data; struct LNode *next; }; /*创建一个带空头结点的单链表*/ struct LNode *CreatList() { struct LNode *head,*p,*temp; head = (struct LNode *)malloc(LEN); p = temp = (struct LNode *)malloc(LEN); printf("input the number:"); scanf("%d",&p->data); head->next = p; while(p->data!=0) { p = (struct LNode *)malloc(LEN); printf("input the number:"); scanf("%d",&p->data); temp->next = p; temp = p; } temp->next = NULL; return head; } /*打印出单链表*/ void Print(struct LNode *head) { struct LNode *p; p = head->next; while(p->next!=NULL) { printf("%d ",p->data); p = p->next; } } /*销毁这个线性表*/ void DestroyList(struct LNode *head) { struct LNode *p,*temp; p = temp = head->next; while(head->next!=NULL) { head->next = p->next; temp = p; free(temp); p = head->next; } } /*在第i个位置前插入新结点*/ void InsertList(struct LNode *head,int i) { struct LNode *p,*insert; int count = 0; p = head; while(count<i-1 && p) { count++; p = p->next; } if(count>i-1 || !p) return; printf("input a number that you want to insert:"); insert = (struct LNode *)malloc(LEN); scanf("%d",&insert->data); insert->next = p->next; p->next = insert; } /*删除第i个结点*/ void DeleteList(struct LNode *head,int i) { struct LNode *p,*temp; int count = 0; p = head; while(count<i-1 && p) { count++; p = p->next; } if(count>i-1 || !p) return; temp = p->next; p->next = temp->next; free(temp); } void main() { struct LNode *L; /*创建链表并打印*/ L = CreatList(); printf("your list is:/n"); Print(L); printf("/n"); /*在第3个结点前插入新结点,并打印*/ InsertList(L,3); printf("now,your list is:/n"); Print(L); printf("/n"); /*删除第4个结点,并打印*/ printf("delete the 4th member,"); printf("now,your list is:/n"); /*销毁链表,并验证是否成功*/ DeleteList(L,4); Print(L); DestroyList(L); if(!L->next) printf("/nyour list has destroyed!"); }
运行后结果:
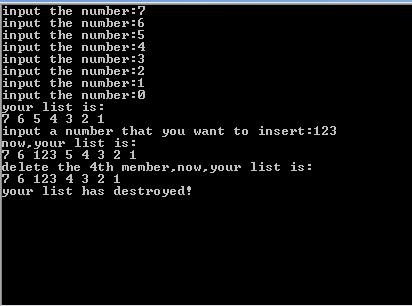
相关文章推荐
- 基础数据结构:顺序表、链表——Python实现
- 数据结构基础(3)---C语言实现单链表
- 算法--基础算法--栈、队列、背包(链表实现)
- Hrbust-1546-基础数据结构——单链表(1)【链表实现】
- 数据结构基础(10) --单链表迭代器的设计与实现
- 数据结构基础(3)---C语言实现单链表
- 数据结构基础(11) --循环链表的设计与实现
- 数据结构基础(12) --双向循环链表的设计与实现
- 【c基础练习】c语言实现链表
- 数据结构基础 之 双链表 与 循环链表 各类操作、思想与实现
- 基础数据结构:顺序表、链表——Python实现
- 二叉树【链表实现】基础练习
- 数据结构基础(12) --双向循环链表的设计与实现
- 链表基础2(实现链表的逆序、将新结点插入到特定位置、边插结点边排序)
- 链表基础以及约瑟夫环的实现
- 数据结构学习笔记2(链表 上 单链表基础操作&实现多项式相乘)
- 数据结构基础(12) --双向循环链表的设计与实现
- C#基础之温习--使用列表和链表实现优一个先级队列
- 链表基础(C语言实现)
- 数据结构基础(12) --双向循环链表的设计与实现