shell中的函数,shell中的数组,告警系统需求分析,
2018-08-01 16:42
941 查看
shell中的函数
函数就是把一段代码整理到了一个小单元中,并给这个小单元起一个名字,当用到这段代码时直接调用这个小单元的名字即可。格式
function f_name() { command }
函数必须要放在最前面
函数名字不可以是数字
示例1
#! bin/bash function inp () { echo $# $2 $3 $0 $# } inp 1 a 2 [root@localhost ~]# sh fun1.sh 3 a 2 fun1.sh 3
$0 是脚本的文件名
$# 是代表参数个数
还有 $@ 是表示所有的参数
#! bin/bash function inp () { echo "The first par is $1" echo "The second par is $2" echo "The third par is $3" echo "The scritp name is $0" echo "The number of par is $#" } inp b a 2 3 adff [root@localhost ~]# sh fun1.sh The first par is b The second par is a The third par is 2 The scritp name is fun1.sh The number of par is 5
示例二 ,定义加法函数
函数得第一个数与第二个数相加,然后打印出数值#!/bin/bash sum() { s=$[$1+$2] echo $s } sum 1 2
示例三,输入网卡名字,显示IP
#!/bin/bash ip() { ifconfig |grep -A1 "$1 " |tail -1 |awk '{print $2}'|awk -F':' '{print $2}' } read -p "Please input the eth name: " e myip=`ip $e` echo "$e address is $myip"
如果输出内容不是自己想要得,需要一段一段得调试,看看是哪里出现了问题。
shell中得数组
定义数组,一串字符,可以是数字,也可以是字母
表示一个数组得格式 有大括号里面还有中括号里面是@[root@localhost ~]# b=(1 2 3) [root@localhost ~]# echo $b 1 [root@localhost ~]# echo ${b[@]} 1 2 3
也可以用*表示
[root@localhost ~]# echo ${b[@]} 1 2 3 [root@localhost ~]# echo ${b[*]} 1 2 3
查看数组其中某一个元素得值
[root@localhost ~]# echo ${b[0]} 1 [root@localhost ~]# echo ${b[1]} 2 [root@localhost ~]# echo ${b[2]} 3
echo ${#a[@]} 获取数组的元素个数
[root@localhost ~]# echo ${#b[@]} 3
数组赋值
更改数组里面得内容[root@localhost ~]# b[3]=a [root@localhost ~]# echo ${b[*]} 1 2 3 a
[root@localhost ~]# b[3]=a [root@localhost ~]# echo ${b[*]} 1 2 3 a [root@localhost ~]# b[3]=acdsdcsa [root@localhost ~]# echo ${b[*]} 1 2 3 acdsdcsa
删除元素
[root@localhost ~]# unset b[3] [root@localhost ~]# echo ${b[*]} 1 2 3
数组分片
定义变量 a=(seq 1 10)
[root@localhost ~]# a=(`seq 1 10`) [root@localhost ~]# echo ${a[@]} 1 2 3 4 5 6 7 8 9 10
echo ${a[@]:0:3} 从第一个元素开始,截取3个
echo ${a[@]:1:4} 从第二个元素开始,截取4个
echo ${a[@]:0-3:2} 从倒数第3个元素开始,截取2个
[root@localhost ~]# echo ${a[@]:0:3} 1 2 3 [root@localhost ~]# echo ${a[@]:1:4} 2 3 4 5 [root@localhost ~]# echo ${a[@]:0-3:2} 8 9
数组的替换
echo ${a[@]/3/100} a=(${a[@]/3/100})
告警系统需求分析
需求:使用shell定制各种个性化告警工具,但需要统一化管理、规范化管理。思路:指定一个脚本包,包含主程序、子程序、配置文件、邮件引擎、输出日志等。
主程序:作为整个脚本的入口,是整个系统的命脉。
配置文件:是一个控制中心,用它来开关各个子程序,指定各个相关联的日志文件。
子程序:这个才是真正的监控脚本,用来监控各个指标。
邮件引擎:是由一个python程序来实现,它可以定义发邮件的服务器、发邮件人以及发件人密码
输出日志:整个监控系统要有日志输出。
要求:我们的机器角色多种多样,但是所有机器上都要部署同样的监控系统,也就说所有机器不管什么角色,整个程序框架都是一致的,不同的地方在于根据不同的角色,定制不同的配置文件。
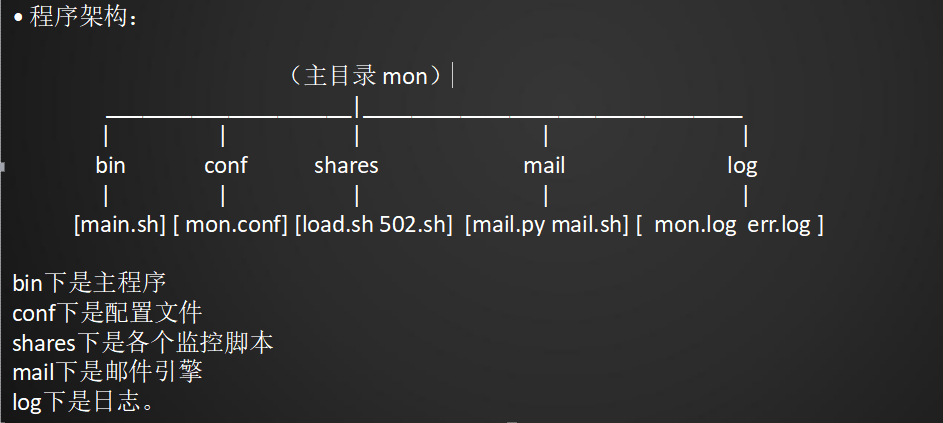
告警系统main.sh
#!/bin/bash #Written by aming. # 是否发送邮件的开关 export send=1 # 过滤ip地址 export addr=`/sbin/ifconfig |grep -A1 "ens33: "|awk '/inet/ {print $2}'` dir=`pwd` # 只需要最后一级目录名 last_dir=`echo $dir|awk -F'/' '{print $NF}'` # 下面的判断目的是,保证执行脚本的时候,我们在bin目录里,不然监控脚本、邮件和日志很有可能找不到 if [ $last_dir == "bin" ] || [ $last_dir == "bin/" ]; then conf_file="../conf/mon.conf" else echo "you shoud cd bin dir" exit fi exec 1>>../log/mon.log 2>>../log/err.log echo "`date +"%F %T"` load average" /bin/bash ../shares/load.sh #先检查配置文件中是否需要监控502 if grep -q 'to_mon_502=1' $conf_file; then export log=`grep 'logfile=' $conf_file |awk -F '=' '{print $2}' |sed 's/ //g'` /bin/bash ../shares/502.sh fi
告警系统mon.conf
## to config the options if to monitor ## 定义mysql的服务器地址、端口以及user、password to_mon_cdb=0 ##0 or 1, default 0,0 not monitor, 1 monitor db_ip=10.20.3.13 db_port=3315 db_user=username db_pass=passwd ## httpd 如果是1则监控,为0不监控 to_mon_httpd=0 ## php 如果是1则监控,为0不监控 to_mon_php_socket=0 ## http_code_502 需要定义访问日志的路径 to_mon_502=1 logfile=/data/log/xxx.xxx.com/access.log ## request_count 定义日志路径以及域名 to_mon_request_count=0 req_log=/data/log/www.discuz.net/access.log domainname=www.discuz.net
告警系统load.sh
#! /bin/bash ##Writen by aming## load=`uptime |awk -F 'average:' '{print $2}'|cut -d',' -f1|sed 's/ //g' |cut -d. -f1` if [ $load -gt 10 ] && [ $send -eq "1" ] then echo "$addr `date +%T` load is $load" >../log/load.tmp /bin/bash ../mail/mail.sh aming_test@163.com "$addr\_load:$load" `cat ../log/load.tmp` fi echo "`date +%T` load is $load"
告警系统502.sh
#! /bin/bash d=`date -d "-1 min" +%H:%M` c_502=`grep :$d: $log |grep ' 502 '|wc -l` if [ $c_502 -gt 10 ] && [ $send == 1 ]; then echo "$addr $d 502 count is $c_502">../log/502.tmp /bin/bash ../mail/mail.sh $addr\_502 $c_502 ../log/502.tmp fi echo "`date +%T` 502 $c_502"
告警系统mail.sh
log=$1 t_s=`date +%s` t_s2=`date -d "2 hours ago" +%s` if [ ! -f /tmp/$log ] then echo $t_s2 > /tmp/$log fi t_s2=`tail -1 /tmp/$log|awk '{print $1}'` echo $t_s>>/tmp/$log v=$[$t_s-$t_s2] echo $v if [ $v -gt 3600 ] then ./mail.py $1 $2 $3 echo "0" > /tmp/$log.txt else if [ ! -f /tmp/$log.txt ] then echo "0" > /tmp/$log.txt fi nu=`cat /tmp/$log.txt` nu2=$[$nu+1] echo $nu2>/tmp/$log.txt if [ $nu2 -gt 10 ] then ./mail.py $1 "trouble continue 10 min $2" "$3" echo "0" > /tmp/$log.txt fi fi
mail.sh内容 //其中mail.py内容到这里下载https://coding.net/u/aminglinux/p/aminglinux-book/git/blob/master/D22Z/mail.py
相关文章推荐
- shell中的函数,shell中的数组,告警系统需求分析
- shell中的函数 shell中的数组 告警系统需求分析
- shell中的函数、数组及告警系统需求分析
- linux学习第六十六篇:shell中的函数,shell中的数组,告警系统需求分析
- shell中的函数、shell中的数组、告警系统需求分析
- 20.16 20.17shell中的函数(上下);20.18 shell中的数组;20.19 告警系统需求分析
- 十六周四次课 2018.02.08 shell中的函数、shell中的数组、告警系统需求分析
- 【shell编程】shell中的函数、shell中的数组和告警系统需求分析
- shell中的函数、shell的数组、告警系统需求分析
- 20.16/20.17 shell中的函数 20.18 shell中的数组20.19 告警系统需求分析
- shell中的函数、数组、告警系统分析
- shell中的函数(function)、数组、告警系统分析
- 20.16/20.17 shell中的函数 20.18 shell中的数组 20.19 告警系统需求
- Shell告警系统(需求分析,告警系统主脚本,告警系统配置文件,告警系统监控项目)
- 告警系统需求分析 告警系统主脚本 告警系统配置文件 告警系统监控项目
- 邮件告警系统需求分析
- 警告系统需求分析 告警系统配置文件 告警系统监控项目load.sh
- 【Linux】中的shell脚本语法篇之函数和数组
- 需求分析挑战之旅——疯狂的订餐系统
- 基于UML的Blog系统分析与设计之一------用户需求篇