Java设计模式之原型模式
2018-04-01 19:27
302 查看
原型模式(Prototype)
一、概述
二、结构
三、浅度克隆和深度克隆
浅度克隆
深度克隆一、概述
定义:原型模式属于对象的创建模式。通过给出一个原型对象来指明所有创建的对象的类型,然后用复制这个原型对象的办法创建出更多同类型的对象。简言之:就是复制粘贴。这就是原型模式的用意。
二、结构原型模式主要用于对象的复制,它的核心是就是类图中的原型类Prototype。Prototype类需要具备以下两个条件:1、实现Cloneable接口。在java语言有一个Cloneable接口,它的作用只有一个,就是在运行时通知虚拟机可以安全地在实现了此接口的类上使用clone方法。在java虚拟机中,只有实现了这个接口的类才可以被拷贝,否则在运行时会抛出 CloneNotSupportedException异常。2、重写Object类中的clone方法。Java中,所有类的父类都是 Object类,Object类中有一个clone方法,作用是返回对象的一个拷贝,但是其作用域protected类型的,一般的类无法调用,因 此,Prototype类需要将clone方法的作用域修改为public类型。三、浅度克隆和深度克隆
浅度克隆
只负责克隆按值传递的数据(比如基本数据类型、String类型),而不复制它所引用的对象,换言之,所有的对其他对象的引用都仍然指向原来的对象。
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
结果:
前后地址相同吗: false
输出p1:汤高 20
输出p2:汤高 20
输出p1:汤高 20
输出p2:周思远 19通过上诉测试可知对于基本类型和String类型的数据前后都是指向不同的地址空间,改变一个不会影响其他的对象浅度克隆图如下
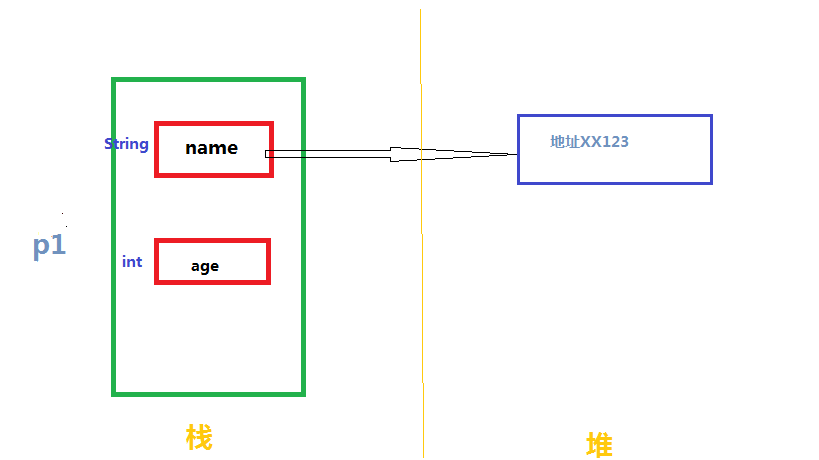
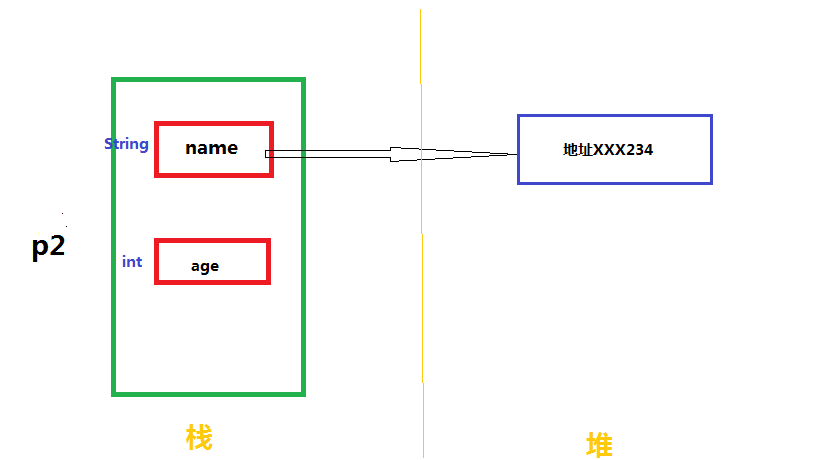
但是如果包含引用类型比如对象、数组、集合等,就只会克隆引用,结果指向同一个引用地址
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
结果:
[汤小高, 周小思]
[汤小高, 周小思]
[汤小高, 周小思, TSY]
[汤小高, 周小思, TSY]
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
结果:
true
学校名: 清华
学校名: 清华
学校名: 北大
学校名: 北大我只改变了p1 结果p2也变了 原因如下图,共享一个引用浅度克隆图如下
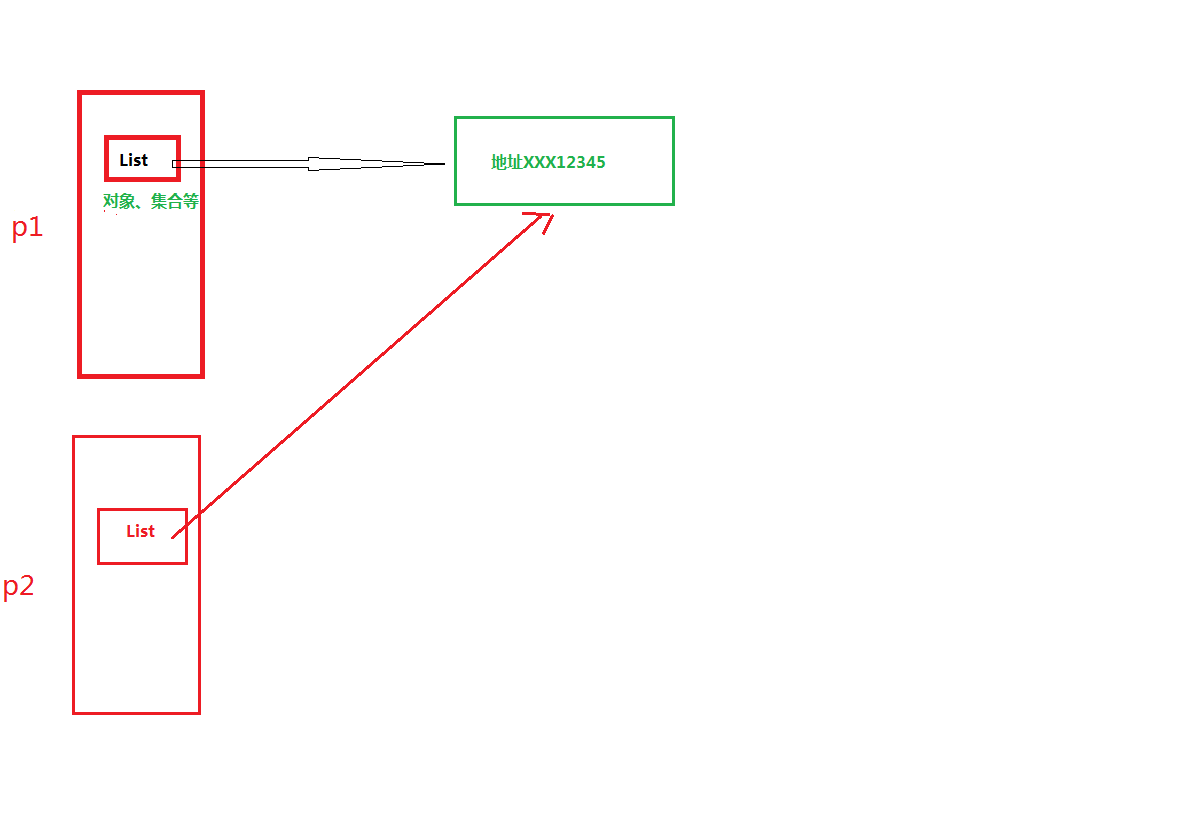
这时候就需要使用深度克隆了!
深度克隆
除了浅度克隆要克隆的值外,还负责克隆引用类型的数据。那些引用其他对象的变量将指向被复制过的新对象,而不再是原有的那些被引用的对象。换言之,深度克隆把要复制的对象所引用的对象都复制了一遍,而这种对被引用到的对象的复制叫做间接复制。
深度克隆要深入到多少层,是一个不易确定的问题。在决定以深度克隆的方式复制一个对象的时候,必须决定对间接复制的对象时采取浅度克隆还是继续 采用深度克隆。因此,在采取深度克隆时,需要决定多深才算深。此外,在深度克隆的过程中,很可能会出现循环引用的问题,必须小心处理。要实现深度克隆 必须修改clone()方法
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
测试类同上结果:
[汤小高, 周小思]
[汤小高, 周小思]
[汤小高, 周小思, TSY]结果:
false
学校名: 清华
学校名: 清华
学校名: 北大
学校名: 清华
我修改了p1,p2的值没有改变 原因如下深度克隆图如下
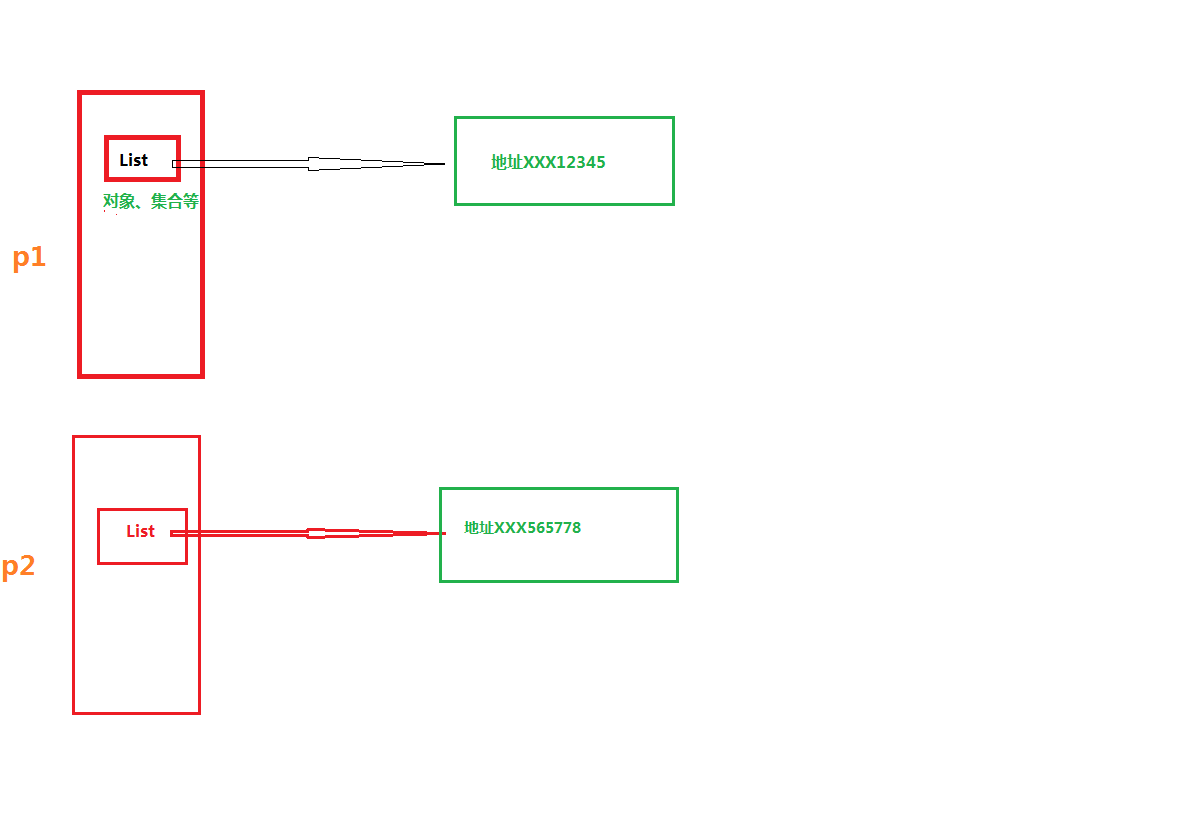
博客转载于:https://blog.csdn.net/tanggao1314/article/details/50596042
一、概述
二、结构
三、浅度克隆和深度克隆
浅度克隆
深度克隆一、概述
定义:原型模式属于对象的创建模式。通过给出一个原型对象来指明所有创建的对象的类型,然后用复制这个原型对象的办法创建出更多同类型的对象。简言之:就是复制粘贴。这就是原型模式的用意。
二、结构原型模式主要用于对象的复制,它的核心是就是类图中的原型类Prototype。Prototype类需要具备以下两个条件:1、实现Cloneable接口。在java语言有一个Cloneable接口,它的作用只有一个,就是在运行时通知虚拟机可以安全地在实现了此接口的类上使用clone方法。在java虚拟机中,只有实现了这个接口的类才可以被拷贝,否则在运行时会抛出 CloneNotSupportedException异常。2、重写Object类中的clone方法。Java中,所有类的父类都是 Object类,Object类中有一个clone方法,作用是返回对象的一个拷贝,但是其作用域protected类型的,一般的类无法调用,因 此,Prototype类需要将clone方法的作用域修改为public类型。三、浅度克隆和深度克隆
浅度克隆
只负责克隆按值传递的数据(比如基本数据类型、String类型),而不复制它所引用的对象,换言之,所有的对其他对象的引用都仍然指向原来的对象。
public class Person1 implements Cloneable{ //基本数据类型 private int age; //String引用类型 private String name; public int getAge() { return age; } public void setAge(int age) { this.age = age; } public String getName() { return name; } public void setName(String name) { this.name = name; } public Person1 clone() { Person1 person =null; try { person = (Person1) super.clone(); return person; } catch (CloneNotSupportedException e) { e.printStackTrace(); return null; } } } public class Client { public static void main(String[] args) { Person1 p1=new Person1(); p1.setName("汤高"); p1.setAge(20); // Person p2=p1;//地址相同 只是把引用给了p2 指向同一个地址 // System.out.println(p1==p2);//true Person1 p2=p1.clone(); //拷贝 地址不同了 指向不同的地址 System.out.println("前后地址相同吗: "+(p2==p1)); System.out.println("输出p1:" +p1.getName()+"\t"+p1.getAge()); System.out.println("输出p2:" +p2.getName()+"\t"+p2.getAge()); //修改拷贝后的对象的属性值 p2.setName("周思远"); p2.setAge(19); System.out.println("输出p1:" +p1.getName()+"\t"+p1.getAge()); System.out.println("输出p2:" +p2.getName()+"\t"+p2.getAge()); } }1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
结果:
前后地址相同吗: false
输出p1:汤高 20
输出p2:汤高 20
输出p1:汤高 20
输出p2:周思远 19通过上诉测试可知对于基本类型和String类型的数据前后都是指向不同的地址空间,改变一个不会影响其他的对象浅度克隆图如下
但是如果包含引用类型比如对象、数组、集合等,就只会克隆引用,结果指向同一个引用地址
public class Person2 implements Cloneable{ //基本数据类型 private int age; //String引用类型 private String name; //引用类型 private List<String> friends=new ArrayList<String>(); //对象 private School school; public School getSchool() { return school; } public void setSchool(School school) { this.school = school; } public int getAge() { return age; } public void setAge(int age) { this.age = age; } public String getName() { return name; } public void setName(String name) { this.name = name; } public List<String> getFriends() { return friends; } public void setFriends(List<String> friends) { this.friends = friends; } public Person2 clone() { Person2 person =null; try { person = (Person2) super.clone(); return person; } catch (CloneNotSupportedException e) { e.printStackTrace(); return null; } } } public class Client2 { public static void main(String[] args) { Person2 p1=new Person2(); List<String> friends=new ArrayList<String>(); friends.add("汤小高"); friends.add("周小思"); p1.setFriends(friends); Person2 p2=p1.clone(); System.out.println(p1.getFriends()); System.out.println(p2.getFriends()); friends.add("TSY"); p1.setFriends(friends); System.out.println(p1.getFriends()); System.out.println(p2.getFriends()); School school=new School(); school.setName("清华"); } }1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
结果:
[汤小高, 周小思]
[汤小高, 周小思]
[汤小高, 周小思, TSY]
[汤小高, 周小思, TSY]
public class Client3 { public static void main(String[] args) { Person2 p1=new Person2(); School school=new School(); school.setName("清华"); p1.setSchool(school); Person2 p2=p1.clone(); System.out.println(p1.getSchool()==p2.getSchool()); System.out.println(p1.getSchool()); System.out.println(p2.getSchool()); school.setName("北大"); p1.setSchool(school); System.out.println(p1.getSchool());//北大 System.out.println(p2.getSchool());//北大 } }1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
结果:
true
学校名: 清华
学校名: 清华
学校名: 北大
学校名: 北大我只改变了p1 结果p2也变了 原因如下图,共享一个引用浅度克隆图如下
这时候就需要使用深度克隆了!
深度克隆
除了浅度克隆要克隆的值外,还负责克隆引用类型的数据。那些引用其他对象的变量将指向被复制过的新对象,而不再是原有的那些被引用的对象。换言之,深度克隆把要复制的对象所引用的对象都复制了一遍,而这种对被引用到的对象的复制叫做间接复制。
深度克隆要深入到多少层,是一个不易确定的问题。在决定以深度克隆的方式复制一个对象的时候,必须决定对间接复制的对象时采取浅度克隆还是继续 采用深度克隆。因此,在采取深度克隆时,需要决定多深才算深。此外,在深度克隆的过程中,很可能会出现循环引用的问题,必须小心处理。要实现深度克隆 必须修改clone()方法
public class Person2 implements Cloneable{ //基本数据类型 private int age; //String引用类型 private String name; //引用类型 private List<String> friends=new ArrayList<String>(); //对象 private School school; public School getSchool() { return school; } public void setSchool(School school) { this.school = school; } public int getAge() { return age; } public void setAge(int age) { this.age = age; } public String getName() { return name; } public void setName(String name) { this.name = name; } public List<String> getFriends() { return friends; } public void setFriends(List<String> friends) { this.friends = friends; } //修改后的clone方法 public Person2 clone() { try { Person2 person = (Person2) super.clone(); if(this.getFriends()!=null){ List<String> friends=new ArrayList<String>(); for(String friend:this.getFriends()){ friends.add(friend); } person.setFriends(friends); } if(this.getSchool()!=null){ School school=new School(); school.setName(this.getSchool().getName()); person.setSchool(school); } return person; } catch (CloneNotSupportedException e) { e.printStackTrace(); return null; } } }1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
测试类同上结果:
[汤小高, 周小思]
[汤小高, 周小思]
[汤小高, 周小思, TSY]结果:
false
学校名: 清华
学校名: 清华
学校名: 北大
学校名: 清华
我修改了p1,p2的值没有改变 原因如下深度克隆图如下
博客转载于:https://blog.csdn.net/tanggao1314/article/details/50596042
相关文章推荐
- 7.java设计模式(读书笔记)原型模式
- Java进阶篇设计模式之三 ----- 建造者模式和原型模式
- java设计模式(2)——原型模式
- java设计模式第13弹--原型模式
- java设计模式--原型设计模式 Prototype
- 《学习笔记》之JAVA设计模式--原型模式
- java设计模式之原型模式
- Java设计模式——原型模式
- JAVA设计模式之原型模式
- Java设计模式---原型模式(Prototype)
- java设计模式学习笔记-原型模式
- 【java】java设计模式(5):原型模式(Prototype)
- 设计模式之第9章-原型模式(Java实现)
- Java设计模式之原型模式(Prototype模式)介绍
- java设计模式之原型模式
- java 设计模式学习笔记(8) - 原型模式
- java设计模式之原型模式
- java设计模式之原型设计模式
- Java设计模式(七):原型模式
- java设计模式--原型设计模式 Prototype