JavaWeb基础——JSP自定义标签
2018-03-23 10:15
323 查看
1、什么是自定义标签
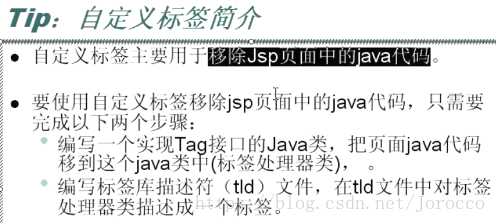
2、自定义标签原理
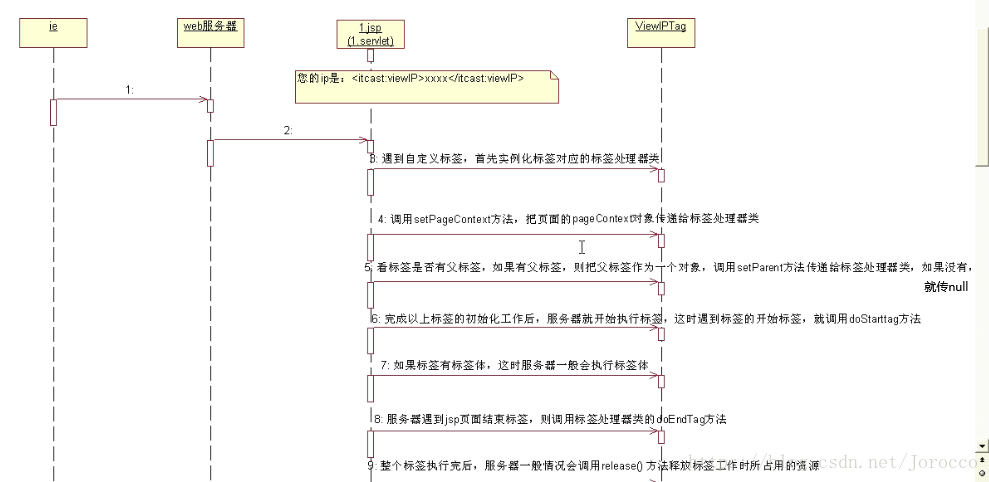
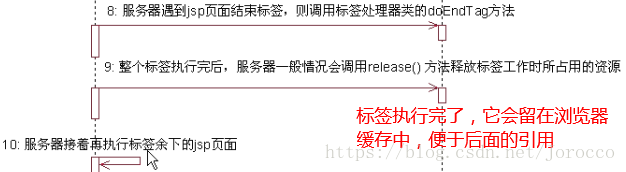
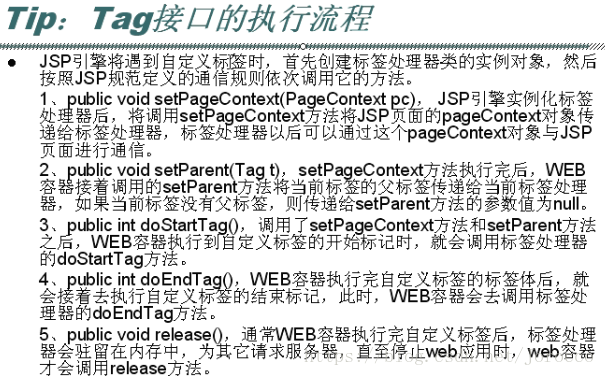
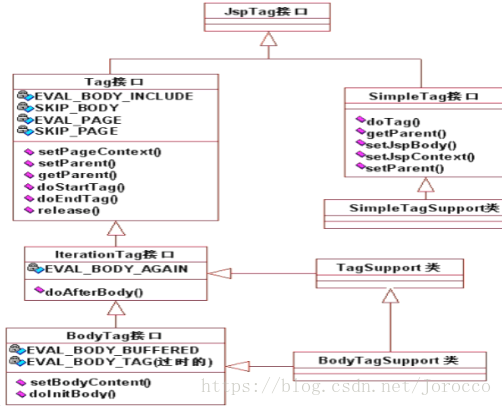
3、自定义标签开发过程
1、编写一个实现tag接口的java类
public class ViewIPTag extends TagSupport {
}
2、在tld文件中对标签处理器类进行描述(tld文件的位置:WEB-INF下)
3、在jsp页面中导入和使用自定义标签
4、自定义标签案例
itcast.tld
ViewIPTag.java
Tag1.jsp
TagDemo1.java
Tag2.jsp
TagDemo3.java
Tag3.jsp
TagDemo4.java
Tag4.jsp
TagDemo5.java
Tag5.jsp
4、简单自定义标签
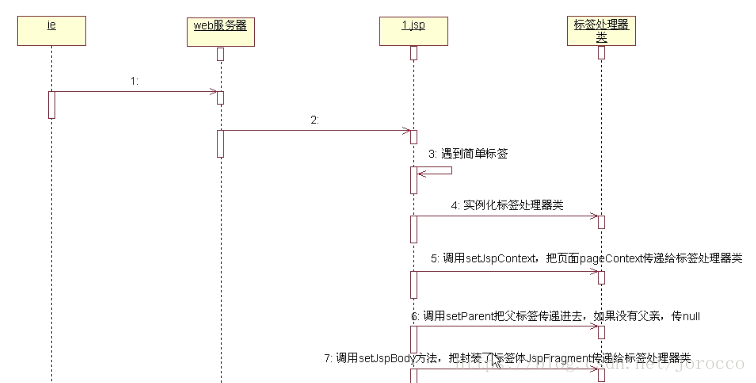
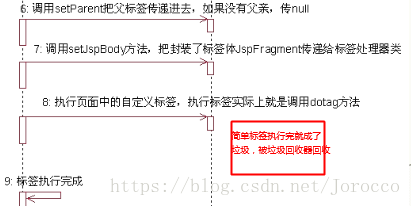
simpleitcast.tld
SimpleTagDemo1.java
1.jsp
SimpleTagDemo2.java
2.jsp
SimpleTagDemo3.java
3.jsp
SimpleTagDemo4.java
4.jsp
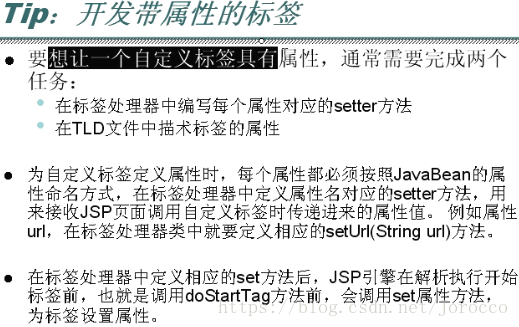
SimpleTagDemo5.java
5.jsp
5、自定义标签的应用
example.tld
RefererTag.java
example1.jsp
index.jsp
RefererTag2.java
RefererTag3.java
RefererTag3_1.java
RefererTag3_2.java
if-else.jsp
ForeachTag.java
add.jsp
ForeachTag2.java
add2.jsp
HtmlFilterTag.java
conver.jsp
6、JSTL标签库的应用
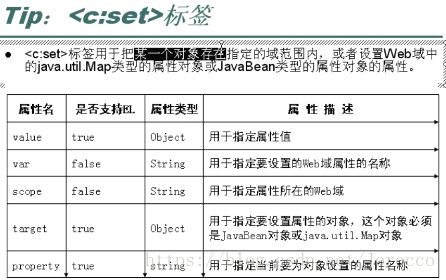
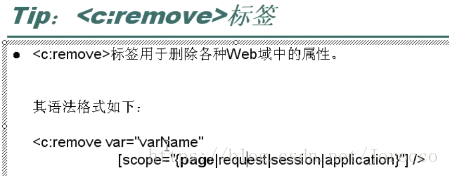
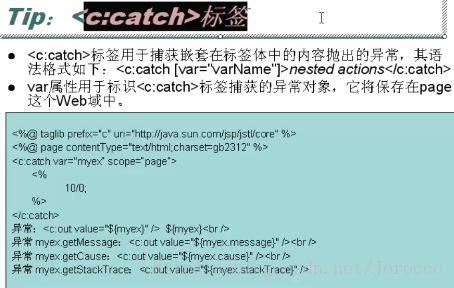
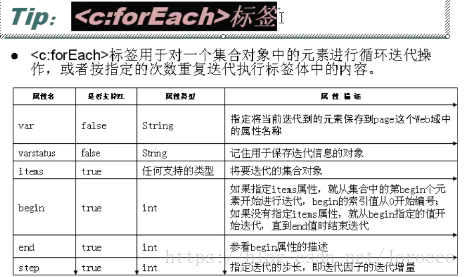
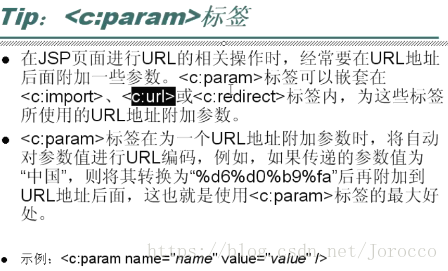
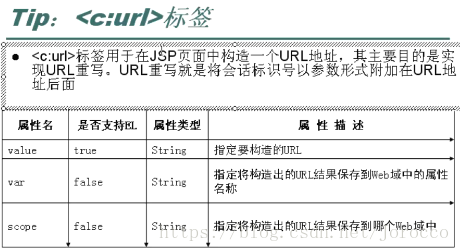
2、自定义标签原理
3、自定义标签开发过程
1、编写一个实现tag接口的java类
public class ViewIPTag extends TagSupport {
@Override public int doStartTag() throws JspException { HttpServletRequest request=(HttpServletRequest) this.pageContext.getRequest(); JspWriter out=this.pageContext.getOut(); String ip=request.getRemoteAddr(); try { out.print(ip); }catch(IOException e) { throw new RuntimeException(e); } return super.doStartTag(); }
}
2、在tld文件中对标签处理器类进行描述(tld文件的位置:WEB-INF下)
3、在jsp页面中导入和使用自定义标签
4、自定义标签案例
itcast.tld
<taglib xmlns="http://java.sun.com/xml/ns/j2ee" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://java.sun.com/xml/ns/j2ee http://java.sun.com/xml/ns/j2ee/web-jsptaglibrary_2_0.xsd" version="2.0"> <description>A tag library exercising SimpleTag handlers.</description> <tlib-version>1.0</tlib-version> <short-name>localhost</ 4000 short-name> <uri>http://localhost</uri><!-- 这里写我们访问的uri --> <tag> <name>ViewIP</name> <tag-class>cn.itcast.web.tag.ViewIPTag</tag-class> <body-content>empty</body-content> </tag> <tag> <name>tagdemo1</name><!-- 这里写标签名称 --> <tag-class>cn.itcast.web.tag.TagDemo1</tag-class><!-- 这里写java类路径 --> <body-content>JSP</body-content> <!--由于标签体就是一段JSP内容,所以这里写JSP --> </tag> <tag> <name>tagdemo3</name><!-- 这里写标签名称 --> <tag-class>cn.itcast.web.tag.TagDemo3</tag-class><!-- 这里写java类路径 --> <body-content>empty</body-content> <!--由于标签体就是一段JSP内容,所以这里写JSP --> </tag> <tag> <name>tagdemo4</name><!-- 这里写标签名称 --> <tag-class>cn.itcast.web.tag.TagDemo4</tag-class><!-- 这里写java类路径 --> <body-content>JSP</body-content> <!--由于标签体就是一段JSP内容,所以这里写JSP --> </tag> <tag> <name>tagdemo5</name><!-- 这里写标签名称 --> <tag-class>cn.itcast.web.tag.TagDemo5</tag-class><!-- 这里写java类路径 --> <body-content>JSP</body-content> <!--由于标签体就是一段JSP内容,所以这里写JSP --> </tag> </taglib>
ViewIPTag.java
package cn.itcast.web.tag;
import java.io.IOException;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.jsp.JspException;
import javax.servlet.jsp.JspWriter;
import javax.servlet.jsp.tagext.TagSupport;
public class ViewIPTag extends TagSupport {
@Override public int doStartTag() throws JspException { HttpServletRequest request=(HttpServletRequest) this.pageContext.getRequest(); JspWriter out=this.pageContext.getOut(); String ip=request.getRemoteAddr(); try { out.print(ip); }catch(IOException e) { throw new RuntimeException(e); } return super.doStartTag(); }
}
Tag1.jsp
<%@ page language="java" contentType="text/html; charset=utf-8" pageEncoding="utf-8"%> <%@taglib uri="http://localhost" prefix="localhost"%> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8"> <title>Insert title here</title> </head> <body> <%-- <!-- 没有自定义标签的方法 --> 您的ip是: <% String ip=request.getRemoteAddr(); out.print(ip); %> --%> 您的ip是:<localhost:ViewIP/> </body> </html>
TagDemo1.java
package cn.itcast.web.tag; import javax.servlet.jsp.JspException; import javax.servlet.jsp.tagext.Tag; import javax.servlet.jsp.tagext.TagSupport; public class TagDemo1 extends TagSupport { @Override public int doStartTag() throws JspException { // TODO Auto-generated method stub return Tag.EVAL_BODY_INCLUDE;//控制标签体的内容执行 // return Tag.SKIP_BODY;//控制标签体的内容不执行 } }
Tag2.jsp
<%@ page language="java" contentType="text/html; charset=utf-8" pageEncoding="utf-8"%> <%@taglib uri="http://localhost" prefix="localhost"%> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8"> <title>使用标签控制页面内容是否输出</title> </head> <body> <localhost:tagdemo1> aaaaaa </localhost:tagdemo1> </body> </html>
TagDemo3.java
package cn.itcast.web.tag; import javax.servlet.jsp.JspException; import javax.servlet.jsp.tagext.Tag; import javax.servlet.jsp.tagext.TagSupport; public class TagDemo3 extends TagSupport { @Override public int doEndTag() throws JspException { // TODO Auto-generated method stub // return Tag.SKIP_PAGE;//整个JSP页面不输出 return Tag.EVAL_PAGE;//页面输出 } }
Tag3.jsp
<%@ page language="java" contentType="text/html; charset=utf-8" pageEncoding="utf-8"%> <%@taglib uri="http://localhost" prefix="localhost" %> <localhost:tagdemo3/> <!-- 后面的整个页面都不会输出 --> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8"> <title>控制整个Jsp页面是否输出</title> </head> <body> this is my page </body> </html>
TagDemo4.java
package cn.itcast.web.tag; import javax.servlet.jsp.JspException; import javax.servlet.jsp.tagext.IterationTag; import javax.servlet.jsp.tagext.Tag; import javax.servlet.jsp.tagext.TagSupport; //控制标签体执行5次 public class TagDemo4 extends TagSupport { private int x=5; @Override public int doStartTag() throws JspException { // TODO Auto-generated method stub return Tag.EVAL_BODY_INCLUDE;//先让标签体执行 } @Override public int doAfterBody() throws JspException { // TODO Auto-generated method stub x--; if(x>0) { return IterationTag.EVAL_BODY_AGAIN;//标签体再执行一遍 }else { return IterationTag.SKIP_BODY;//标签体不执行 } } }
Tag4.jsp
<%@ page language="java" contentType="text/html; charset=utf 1c6f4 -8" pageEncoding="utf-8"%> <%@taglib uri="http://localhost" prefix="localhost" %> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8"> <title>用标签控制标签体重复执行</title> </head> <body> <localhost:tagdemo4> 重复输出 </localhost:tagdemo4> </body> </html>
TagDemo5.java
package cn.itcast.web.tag; import java.io.IOException; import javax.servlet.jsp.JspException; import javax.servlet.jsp.tagext.BodyContent; import javax.servlet.jsp.tagext.BodyTag; import javax.servlet.jsp.tagext.BodyTagSupport; import javax.servlet.jsp.tagext.Tag; //修改标签体,将标签体改为大写 public class TagDemo5 extends BodyTagSupport { @Override public int doStartTag() throws JspException { // TODO Auto-generated method stub return BodyTag.EVAL_BODY_BUFFERED;//将标签体内容封装到BodyContent对象中 } @Override public int doEndTag() throws JspException { // TODO Auto-generated method stub BodyContent bc=this.getBodyContent();//获取标签体内容 String content=bc.getString(); content=content.toUpperCase(); try { this.pageContext.getOut().write(content); }catch(IOException e) { throw new RuntimeException(e); } return Tag.EVAL_PAGE; } }
Tag5.jsp
<%@ page language="java" contentType="text/html; charset=utf-8" pageEncoding="utf-8"%> <%@taglib uri="http://localhost" prefix="localhost" %> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8"> <title>用标签修改jsp页面内容</title> </head> <body> <localhost:tagdemo5> dafadf </localhost:tagdemo5> </body> </html>
4、简单自定义标签
simpleitcast.tld
<taglib xmlns="http://java.sun.com/xml/ns/j2ee" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://java.sun.com/xml/ns/j2ee http://java.sun.com/xml/ns/j2ee/web-jsptaglibrary_2_0.xsd" version="2.0"> <description>A tag library exercising SimpleTag handlers.</description> <tlib-version>1.0</tlib-version> <short-name>simpleTag</short-name> <uri>http://localhost</uri><!-- 这里写我们访问的uri --> <tag> <name>demo1</name> <tag-class>cn.itcast.web.simpletag.SimpleTagDemo1</tag-class> <body-content>scriptless</body-content> </tag> <tag> <name>demo3</name> <tag-class>cn.itcast.web.simpletag.SimpleTagDemo3</tag-class> <body-content>scriptless</body-content> </tag> <tag> <name>demo4</name> <tag-class>cn.itcast.web.simpletag.SimpleTagDemo4</tag-class> <body-content>empty</body-content> </tag> <tag> <name>demo5</name> <tag-class>cn.itcast.web.simpletag.SimpleTagDemo5</tag-class> <body-content>scriptless</body-content> <attribute> <name>count</name> <required>true</required> <rtexprvalue>true</rtexprvalue><!--用true的话,那个属性既可以是el表达式,也可以是脚本片段,也可以是值 --> </attribute> <attribute> <name>date</name> <required>true</required> <rtexprvalue>true</rtexprvalue><!--用true的话,那个属性既可以是el表达式,也可以是脚本片段,也可以是值 --> </attribute> </tag> </taglib>
SimpleTagDemo1.java
package cn.itcast.web.simpletag; import java.io.IOException; import javax.servlet.jsp.JspException; import javax.servlet.jsp.tagext.JspFragment; import javax.servlet.jsp.tagext.SimpleTagSupport; //控制标签体是否执行 public class SimpleTagDemo1 extends SimpleTagSupport { @Override public void doTag() throws JspException, IOException { // TODO Auto-generated method stub JspFragment jf=this.getJspBody();//得到标签体 jf.invoke(this.getJspContext().getOut());//标签体输出 //jf.invoke(this.getJspContext().getOut());//标签体不输出 } }
1.jsp
<%@ page language="java" contentType="text/html; charset=utf-8" pageEncoding="utf-8"%> <%@taglib uri="http://localhost" prefix="simpleTag" %> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8"> <title>简单标签控制是否执行标签体</title> </head> <body> <simpleTag:demo1> aaaaa </simpleTag:demo1> </body> </html>
SimpleTagDemo2.java
package cn.itcast.web.simpletag; import java.io.IOException; import javax.servlet.jsp.JspException; import javax.servlet.jsp.tagext.JspFragment; import javax.servlet.jsp.tagext.SimpleTagSupport; //控制标签体重复执行 public class SimpleTagDemo2 extends SimpleTagSupport { @Override public void doTag() throws JspException, IOException { // TODO Auto-generated method stub JspFragment jf=this.getJspBody();//得到标签体 for(int i=0;i<5;i++) { jf.invoke(null);//这句话相当于下面的那句 // jf.invoke(this.getJspContext().getOut());//标签体输出 } } }
2.jsp
<%@ page language="java" contentType="text/html; charset=utf-8" pageEncoding="utf-8"%> <%@taglib uri="http://localhost" prefix="simpleTag" %> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8"> <title>控制标签体重复执行</title> </head> <body> <simpleTag:demo2> adadfa </simpleTag:demo2> </body> </html>
SimpleTagDemo3.java
package cn.itcast.web.simpletag; import java.io.IOException; import java.io.StringWriter; import javax.servlet.jsp.JspException; import javax.servlet.jsp.tagext.JspFragment; import javax.servlet.jsp.tagext.SimpleTagSupport; //修改标签体 public class SimpleTagDemo3 extends SimpleTagSupport { @Override public void doTag() throws JspException, IOException { // TODO Auto-generated method stub JspFragment jf=this.getJspBody();//得到标签体 StringWriter sw=new StringWriter(); jf.invoke(sw);//将标签体内容写入到缓冲流 String content=sw.toString();//将内容转成字符串 content=content.toUpperCase(); this.getJspContext().getOut().write(content);//输出 } }
3.jsp
<%@ page language="java" contentType="text/html; charset=utf-8" pageEncoding="utf-8"%> <%@taglib uri="http://localhost" prefix="simpleTag" %> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8"> <title>修改标签体</title> </head> <body> <simpleTag:demo3> adadfa </simpleTag:demo3> </body> </html>
SimpleTagDemo4.java
package cn.itcast.web.simpletag; import java.io.IOException; import java.io.StringWriter; import javax.servlet.jsp.JspException; import javax.servlet.jsp.SkipPageException; import javax.servlet.jsp.tagext.JspFragment; import javax.servlet.jsp.tagext.SimpleTagSupport; //控制标签余下的JSP是否执行 public class SimpleTagDemo4 extends SimpleTagSupport { @Override public void doTag() throws JspException, IOException { // TODO Auto-generated method stub throw new SkipPageException();//标签余下的jsp不执行 } }
4.jsp
<%@ page language="java" contentType="text/html; charset=utf-8" pageEncoding="utf-8"%> <%@taglib uri="http://localhost" prefix="simpleTag" %> <simpleTag:demo4/> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8"> <title>控制整个JSP是否执行</title> </head> <body> adadfa </body> </html>
SimpleTagDemo5.java
package cn.itcast.web.simpletag; import java.io.IOException; import java.io.StringWriter; import java.util.Date; import javax.servlet.jsp.JspException; import javax.servlet.jsp.SkipPageException; import javax.servlet.jsp.tagext.JspFragment; import javax.servlet.jsp.tagext.SimpleTagSupport; //带有属性的标签 public class SimpleTagDemo5 extends SimpleTagSupport { private int count;//基本属性 private Date date;//非基本属性 public void setCount(int count) { this.count=count; } public Date getDate() { return date; } public void setDate(Date date) { this.date = date; } @Override public void doTag() throws JspException, IOException { // TODO Auto-generated method stub JspFragment jf=this.getJspBody(); this.getJspContext().getOut().write(date.toLocaleString()+"<br/>");//因为服务器只能转换成8种基本数据类型,非基本数据类型就得转换 for(int i=0;i<count;i++) { jf.invoke(null); } } }
5.jsp
<%@ page language="java" contentType="text/html; charset=utf-8" import="java.util.Date" pageEncoding="utf-8"%> <%@taglib uri="http://localhost" prefix="simpleTag" %> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8"> <title>开发带属性的标签</title> </head> <body> <simpleTag:demo5 count="9" date="<%=new Date() %>"> ssss </simpleTag:demo5> </body> </html>
5、自定义标签的应用
example.tld
<taglib xmlns="http://java.sun.com/xml/ns/j2ee" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://java.sun.com/xml/ns/j2ee http://java.sun.com/xml/ns/j2ee/web-jsptaglibrary_2_0.xsd" version="2.0"> <description>A tag library exercising SimpleTag handlers.</description> <tlib-version>1.0</tlib-version> <short-name>localhost</short-name> <uri>/example</uri><!-- 这里写我们访问的uri --> <tag> <name>Referer</name> <tag-class>cn.itcast.web.tag.example.RefererTag</tag-class> <body-content>empty</body-content> <attribute> <name>site</name> <required>true</required> <rtexprvalue>true</rtexprvalue> </attribute> <attribute> <name>page</name> <required>true</required> <rtexprvalue>true</rtexprvalue> </attribute> </tag> <tag> <name>if</name> <tag-class>cn.itcast.web.tag.example.RefererTag2</tag-class> <body-content>scriptless</body-content> <attribute> <name>test</name> <required>true</required> <rtexprvalue>true</rtexprvalue> </attribute> </tag> <tag> <name>RefererTag3</name> <tag-class>cn.itcast.web.tag.example.RefererTag3</tag-class> <body-content>scriptless</body-content> </tag> <tag> <name>RefererTag3_1</name> <tag-class>cn.itcast.web.tag.example.RefererTag3_1</tag-class> <body-content>scriptless</body-content> <attribute> <name>test</name> <required>true</required> <rtexprvalue>true</rtexprvalue> </attribute> </tag> <tag> <name>RefererTag3_2</name> <tag-class>cn.itcast.web.tag.example.RefererTag3_2</tag-class> <body-content>scriptless</body-content> </tag> <tag> <name>foreach</name> <tag-class>cn.itcast.web.tag.example.ForeachTag</tag-class> <body-content>scriptless</body-content> <attribute> <name>items</name> <required>true</required> <rtexprvalue>true</rtexprvalue> </attribute> <attribute> <name>var</name> <required>true</required> <rtexprvalue>false</rtexprvalue> </attribute> </tag> <tag> <name>foreach2</name> <tag-class>cn.itcast.web.tag.example.ForeachTag2</tag-class> <body-content>scriptless</body-content> <attribute> <name>items</name> <required>true</required> <rtexprvalue>true</rtexprvalue> </attribute> <attribute> <name>var</name> <required>true</required> <rtexprvalue>false</rtexprvalue> </attribute> </tag> <tag> <name>htmlFilter</name> <tag-class>cn.itcast.web.tag.example.HtmlFilterTag</tag-class> <body-content>scriptless</body-content> </tag> </taglib>
RefererTag.java
package cn.itcast.web.tag.example; import java.io.IOException; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import javax.servlet.jsp.JspException; import javax.servlet.jsp.PageContext; import javax.servlet.jsp.SkipPageException; import javax.servlet.jsp.tagext.SimpleTagSupport; //防盗链 public class RefererTag extends SimpleTagSupport { private String site; private String page; public void setSite(String site) { this.site = site; } public void setPage(String page) { this.page = page; } @Override public void doTag() throws JspException, IOException { PageContext pageContext=(PageContext) this.getJspContext(); HttpServletRequest request=(HttpServletRequest) pageContext.getRequest(); HttpServletResponse response=(HttpServletResponse) pageContext.getResponse(); //1、得到来访者referer String referer=request.getHeader("referer"); if(referer==null||!referer.startsWith(site)) { response.sendRedirect(page); }else if(page.startsWith("/")) { response.sendRedirect(request.getContextPath()+page); }else { response.sendRedirect(request.getContextPath()+"/"+page); } throw new SkipPageException(); } }
example1.jsp
<%@ page language="java" contentType="text/html; charset=utf-8" pageEncoding="utf-8"%> <%@taglib uri="/example" prefix="e" %> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <e:Referer site="http://localhost" page="/index.jsp"/> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8"> <title>开发防盗链</title> </head> <body> fdsklaffdsa </body> </html>
index.jsp
<%@ page language="java" contentType="text/html; charset=utf-8" pageEncoding="utf-8"%> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8"> <title>Insert title here</title> </head> <body> 看广告 <a href="example1.jsp">查看</a> </body> </html>
RefererTag2.java
package cn.itcast.web.tag.example; import java.io.IOException; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import javax.servlet.jsp.JspException; import javax.servlet.jsp.PageContext; import javax.servlet.jsp.SkipPageException; import javax.servlet.jsp.tagext.SimpleTagSupport; //模仿if标签 public class RefererTag2 extends SimpleTagSupport { private boolean test; public void setTest(boolean test) { this.test = test; } @Override public void doTag() throws JspException, IOException { if(test) { this.getJspBody().invoke(null); } } }
<%@ page language="java" contentType="text/html; charset=utf-8" import="java.util.Date" pageEncoding="utf-8"%> <%@taglib uri="/example" prefix="c" %> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8"> <title>开发if标签</title> </head> <body> <% session.setAttribute("user", "aaaa"); %> <c:if test="${user==null }"> aaaa </c:if> <c:if test="${user!=null }"> bbbb </c:if> </body> </html>
RefererTag3.java
package cn.itcast.web.tag.example; import java.io.IOException; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import javax.servlet.jsp.JspException; import javax.servlet.jsp.PageContext; import javax.servlet.jsp.SkipPageException; import javax.servlet.jsp.tagext.SimpleTagSupport; //模仿if-else标签 public class RefererTag3 extends SimpleTagSupport { private boolean isDo; public boolean isDo() { return isDo; } public void setDo(boolean isDo) { this.isDo = isDo; } @Override public void doTag() throws JspException, IOException { // TODO Auto-generated method stub this.getJspBody().invoke(null); } }
RefererTag3_1.java
package cn.itcast.web.tag.example; import java.io.IOException; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import javax.servlet.jsp.JspException; import javax.servlet.jsp.PageContext; import javax.servlet.jsp.SkipPageException; import javax.servlet.jsp.tagext.SimpleTagSupport; //模仿if-else标签 public class RefererTag3_1 extends SimpleTagSupport { private boolean test; public void setTest(boolean test) { this.test = test; } @Override public void doTag() throws JspException, IOException { // TODO Auto-generated method stub RefererTag3 parent=(RefererTag3) this.getParent();//因为doTag就已经setParent,所以直接获取就得了 if(test && !parent.isDo()) { this.getJspBody().invoke(null); parent.setDo(true); } } }
RefererTag3_2.java
package cn.itcast.web.tag.example; import java.io.IOException; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import javax.servlet.jsp.JspException; import javax.servlet.jsp.PageContext; import javax.servlet.jsp.SkipPageException; import javax.servlet.jsp.tagext.SimpleTagSupport; //模仿if-else标签 public class RefererTag3_2 extends SimpleTagSupport { @Override public void doTag() throws JspException, IOException { // TODO Auto-generated method stub RefererTag3 parent=(RefererTag3) this.getParent();//因为doTag就已经setParent,所以直接获取就得了 if(!parent.isDo()) { this.getJspBody().invoke(null); parent.setDo(true); } } }
if-else.jsp
<%@ page language="java" contentType="text/html; charset=utf-8" import="java.util.Date" pageEncoding="utf-8"%> <%@taglib uri="/example" prefix="c" %> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8"> <title>开发if-else标签</title> </head> <body> <c:RefererTag3> <c:RefererTag3_1 test="${user==null }"> aaaa </c:RefererTag3_1> <c:RefererTag3_2> bbbb </c:RefererTag3_2> </c:RefererTag3> </body> </html>
ForeachTag.java
package cn.itcast.web.tag.example; import java.io.IOException; import java.util.Iterator; import java.util.List; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import javax.servlet.jsp.JspException; import javax.servlet.jsp.PageContext; import javax.servlet.jsp.SkipPageException; import javax.servlet.jsp.tagext.SimpleTagSupport; //模仿foreach标签 public class ForeachTag extends SimpleTagSupport { private Object items; private String var; public void setItems(Object items) { this.items = items; } public void setVar(String var) { this.var = var; } @Override public void doTag() throws JspException, IOException { List list=(List)items; Iterator it=list.iterator(); while(it.hasNext()) { Object value=it.next(); this.getJspContext().setAttribute(var, value); this.getJspBody().invoke(null); } } }
add.jsp
<%@ page language="java" contentType="text/html; charset=utf-8" import="java.util.*" pageEncoding="utf-8"%> <%@taglib uri="/example" prefix="c" %> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8"> <title>开发foreach迭代标签</title> </head> <body> <% List list=new ArrayList(); list.add("aaa"); list.add("bbb"); list.add("ccc"); request.setAttribute("list", list); %> <c:foreach var="str" items="${list }"> ${str } </c:foreach> </body> </html>
ForeachTag2.java
package cn.itcast.web.tag.example; import java.io.IOException; import java.lang.reflect.Array; import java.util.ArrayList; import java.util.Arrays; import java.util.Collection; import java.util.Iterator; import java.util.List; import java.util.Map; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import javax.servlet.jsp.JspException; import javax.servlet.jsp.PageContext; import javax.servlet.jsp.SkipPageException; import javax.servlet.jsp.tagext.SimpleTagSupport; //模仿foreach标签 public class ForeachTag2 extends SimpleTagSupport { private Object items; private String var; private Collection collection; /* * 先判断传递进来是不是单列集合:List、Set * 如果不是则判断它是不是map,是则将它转成entrySet(单列) * 或者判断它是不是数组,是也将它转成列表 * 总之,都是为了将之统一为单列集合,以便后面的迭代操作 * */ public void setItems(Object items) { this.items = items; if(items instanceof Collection) { collection=(Collection)items; } if(items instanceof Map) { Map map=(Map)items; collection=map.entrySet(); } if(items instanceof Object[]) { Object obj[]=(Object[])items; collection=Arrays.asList(obj); } /* * 能够对任意数组进行操作 * */ if(items.getClass().isArray()) { this.collection=new ArrayList(); int length=Array.getLength(items);//获取数组的长度(不管是什么类型数组) for(int i=0;i<length;i++) { Object value=Array.get(items, i);//获取数组中第i个位置的数据,不论是什么类型的数组都是对象,所以用Object this.collection.add(value); } } /* * 基本数据类型数组的判断(传统方法) if(items instanceof int[]) { int arr[]=(int[])items; this.collection=new ArrayList(); for(int i:arr) { this.collection.add(i); } }*/ } public void setVar(String var) { this.var = var; } @Override public void doTag() throws JspException, IOException { Iterator it=this.collection.iterator(); while(it.hasNext()) { Object value=it.next(); this.getJspContext().setAttribute(var, value); this.getJspBody().invoke(null); } } }
add2.jsp
<%@ page language="java" contentType="text/html; charset=utf-8" import="java.util.*,java.lang.Integer" pageEncoding="utf-8"%> <%@taglib uri="/example" prefix="c" %> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8"> <title>开发foreach迭代标签</title> </head> <body> <% List list=new ArrayList(); list.add("aaa"); list.add("bbb"); list.add("ccc"); request.setAttribute("list", list); %> <c:foreach2 var="str" items="${list }"> ${str } </c:foreach2> <hr/> <% Map map=new HashMap(); map.put("aaa","111"); map.put("bbb","222"); map.put("ccc","333"); request.setAttribute("map", map); %> <c:foreach2 var="entry" items="${map }"> ${entry.key }=${entry.value } </c:foreach2> <hr/> <% Integer num[] ={1,2,3,4}; request.setAttribute("num",num); %> <c:foreach2 var="i" items="${num }"> ${i } </c:foreach2> <br/>--------基本数据类型---------<br/> <% int arr[]={1,2,3,4}; request.setAttribute("arr", arr); %> <c:foreach2 items="${arr }" var="i"> ${i } </c:foreach2> <hr/> <% boolean b[]={true,false,true}; request.setAttribute("b", b); %> <c:foreach2 items="${b }" var="i"> ${i } </c:foreach2> </body> </html>
HtmlFilterTag.java
package cn.itcast.web.tag.example; import java.io.IOException; import java.io.StringWriter; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import javax.servlet.jsp.JspException; import javax.servlet.jsp.PageContext; import javax.servlet.jsp.SkipPageException; import javax.servlet.jsp.tagext.JspFragment; import javax.servlet.jsp.tagext.SimpleTagSupport; //转义标签 public class HtmlFilterTag extends SimpleTagSupport { @Override public void doTag() throws JspException, IOException { StringWriter sw=new StringWriter(); JspFragment jf=this.getJspBody(); jf.invoke(sw); String content=sw.getBuffer().toString(); content=filter(content); this.getJspContext().getOut().write(content); } public static String filter(String message) { if (message == null) return (null); char content[] = new char[message.length()]; message.getChars(0, message.length(), content, 0); StringBuilder result = new StringBuilder(content.length + 50); for (int i = 0; i < content.length; i++) { switch (content[i]) { case '<': result.append("<"); break; case '>': result.append(">"); break; case '&': result.append("&"); break; case '"': result.append("""); break; default: result.append(content[i]); } } return (result.toString()); } }
conver.jsp
<%@ page language="java" contentType="text/html; charset=utf-8" import="java.util.Date" pageEncoding="utf-8"%> <%@taglib uri="/example" prefix="c" %> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8"> <title>开发转义标签</title> </head> <body> <c:htmlFilter> <a href="">点点</a><!-- 就是能直接输出这个源码 --> </c:htmlFilter> </body> </html>
6、JSTL标签库的应用
<%@ page language="java" import="java.util.*,cn.itcast.domain.Person" contentType="text/html; charset=utf-8" pageEncoding="utf-8"%> <%@taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c"%> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8"> <title>测试jstl中的c:out</title> </head> <body> <br/>-------------------c:out-----------------------<br/> <c:out value="aaaa" default="bbbb" escapeXml="true"></c:out><!--default是默认值,如果没有传递数据,它就会输出默认值, escapeXml转义与否属性 --> <br/>-------------------c:set可以操作各个域-----------------------<br/> <c:set var="data" value="xxxx" scopr="page"></c:set><!--往page域中存关键字为data,值为xxx --> ${data } <% Map map=new HashMap(); request.setAttribute("map", map); %> <c:set property="dd" value="xxx" target="${map }"></c:set><!--往map域中属性为dd,值为xxx --> ${map.dd } <% Person p=new Person(); request.setAttribute("p", p); %> <c:set property="name" value="ssss" target="${p }"></c:set><!--往Javabean域中属性为dd,值为xxx --> ${p.name } <br/>-------------------c:catch-----------------------<br/> <c:catch var="myex"> <% int x=1/0; %> </c:catch> ${myex.message }<!--catch标签能够抓捕jsp页面异常,这里能打印出来 --> <br/>-------------------c:foreach-----------------------<br/> <c:foreach var="num" begin="1" end="9" step="1"> ${num } </c:foreach> <!--结果为:1,2,3,4,5,6,7,8,9 从1开始到9 步长为1--> <br/>-------------------c:url-----------------------<br/> <c:url var="url" value="/day11/xx"/> <a href="${url }">购买</a><!--url地址重写--> </body> </html>
相关文章推荐
- JavaWeb基础(servlet+jsp)
- JavaWeb学习笔记——jsp基础语法
- javaweb基础(15)_jsp基础语法
- javaweb(十五)——JSP基础语法
- JavaWeb学习笔记 ---- Jsp基础知识归纳篇(下)
- JavaWeb总结十五、JSP基础用法
- JavaEE-JSP基础-自定义标签
- javaWeb jsp基础
- JavaWeb系列-JSP基础语法
- J2EE基础:JSP中自定义标签的详细讲解
- JSP自定义标签学习(基础)
- JSP自定义标签学习(基础)
- JavaWeb基础—JSP自定义标签入门
- JSP自定义标签基础知识学习
- JavaWeb基础之一JSP语法(二) JSP基本语法(2)
- JSP自定义标签学习(基础)
- JavaWeb基础(5)—— 浅析 Servlet 与 JSP 两者之间的区别
- JavaWeb——JSP基础
- JavaWeb 之 JSP基础