如何自己实现spring aop相似的功能
2018-03-20 00:00
661 查看
AOP(Aspect Oriented Programming),即面向切面编程,它利用一种称为"横切"的技术,把软件系统分为两个部分:核心关注点和横切关注点,用来实现诸如权限认证、日志、事务等功能。简单讲就是在指定类的指定方法前后增加通用代码,减少冗余增强功能。
手动实现:
被代理的目标接口
被代理的目标实现类
需要增加的通用操作类
代理工厂类
spring配置文件
测试类
运行结果
手动实现:
被代理的目标接口
package com.xxx.service; public interface RunningService { public void running(); }
被代理的目标实现类
package com.xxx.service.impl; import org.springframework.stereotype.Component; import com.xxx.service.RunningService; @Component public class RunningServiceImpl implements RunningService { @Override public void running() { System.out.println("do something"); } }
需要增加的通用操作类
package com.xxx.proxy; import org.springframework.stereotype.Component; @Component public class Aop { public void before(){ System.out.println("before!"); } public void after(){ System.out.println("after!"); } }
代理工厂类
package com.xxx.proxy; import java.lang.reflect.InvocationHandler; import java.lang.reflect.Method; import java.lang.reflect.Proxy; public class ProxyFactory { public static Object newProxyInstance(final Object target,final Aop aop){ ClassLoader classLoader = target.getClass().getClassLoader(); Class<?>[] interfaces = target.getClass().getInterfaces(); InvocationHandler invocationHandler = new InvocationHandler() { @Override public Object invoke(Object proxy, Method method, Object[] args) throws Throwable { aop.before(); Object result = method.invoke(target, args); aop.after(); return result; } }; return Proxy.newProxyInstance(classLoader, interfaces, invocationHandler); } }
spring配置文件
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:p="http://www.springframework.org/schema/p" xmlns:context="http://www.springframework.org/schema/context" xsi:schemaLocation=" http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context.xsd" default-autowire="byType"> <!-- 开启注解扫描 --> <context:component-scan base-package="com.xxx.proxy"></context:component-scan> <!-- 调用工厂方法,返回runningService的代理对象 --> <bean id="runningService_proxy" class="com.xxx.proxy.ProxyFactory" factory-method="newProxyInstance"> <constructor-arg index="0" type="java.lang.Object" ref="runningServiceImpl"></constructor-arg> <constructor-arg index="1" type="com.xxx.proxy.Aop" ref="aop"></constructor-arg> </bean> </beans>
测试类
package com.xxx.proxy; import org.junit.Test; import org.springframework.context.support.ClassPathXmlApplicationContext; import com.xxx.proxy.service.RunningService; public class TestAop { @Test public void test(){ ClassPathXmlApplicationContext context = new ClassPathXmlApplicationContext("classpath:applicationContext.xml"); RunningService run = (RunningService)context.getBean("runningService_proxy"); run.running(); } }
运行结果
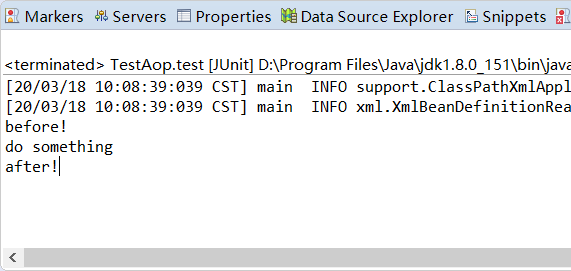
相关文章推荐
- 15_传智播客Spring2.5视频教程_使用JDK中的Proxy技术实现AOP功能 2
- 本人用SPRING 框架自己写DAO实现LIMIT功能
- spring aop 面向切面编程 如何来做一个强大的日志记录功能
- spring是如何实现AOP的
- 在Spring中,是如何实现 Aop 的,原理:动态代理+cglib 分步图解
- 15_传智播客Spring2.5视频教程_使用JDK中的Proxy技术实现AOP功能 3
- 如何在自己网页上实现加入google groups功能
- Spring如何实现IOC与AOP的
- springAOP理解——java中的proxy实现AOP功能
- 使用jdk和cglib实现spring的aop功能
- 编码实现用JDK中的Proxy实现springAOP功能
- 加强2注解。泛型。类加载器及其委托机制。代理的概念与作用原理,AOP概念。实现AOP功能的封装与配置。类似Spring。
- SSH集成框架下真正实现Spring AOP拦截功能
- Spring如何实现IOC与AOP的
- Spring AOP功能的实现
- ITCAST视频-Spring学习笔记(使用CGLIB实现AOP功能与AOP概念解释)
- 框架学习之Spring 第三节 采用Spring实现AOP功能
- 15_传智播客Spring2.5视频教程_使用JDK中的Proxy技术实现AOP功能
- 发现Windows程序 最主要的还是要理解消息和窗口的相互调用,相互影响是如何实现的,才能更准确的去理解Windows程序,去编好自己想要的程序功能