CXF+Spring+Hibernate实现RESTful webservice服务端实例
2018-03-17 14:08
525 查看
1.RESTful API接口定义
代码下载请访问 GitHub上的 witpool/wit-pluto
导入工程文件、编译、打包步骤如下:
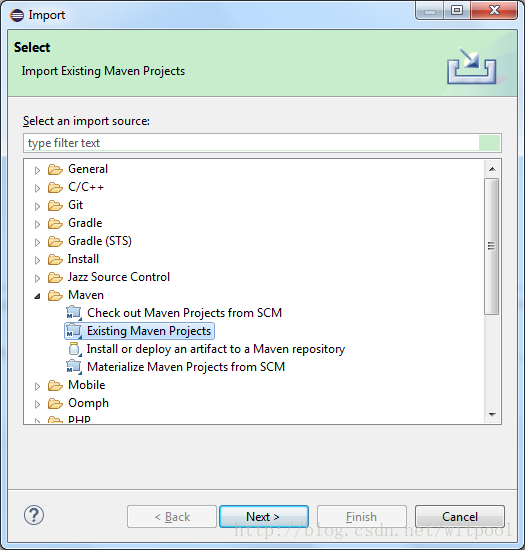
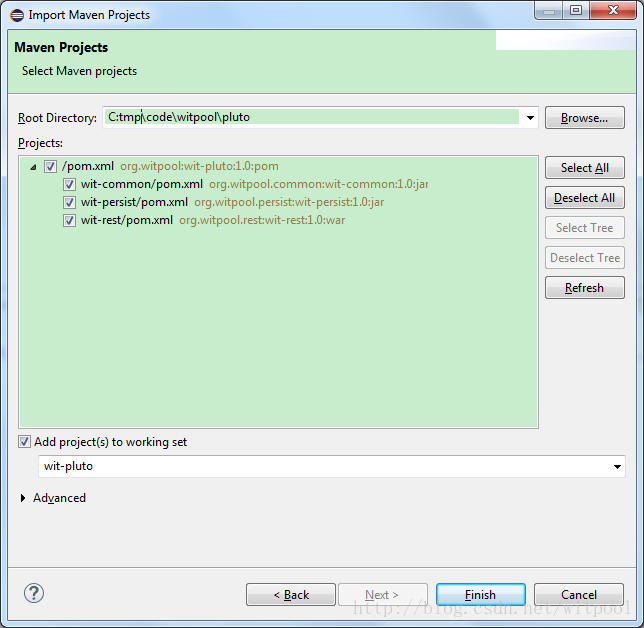
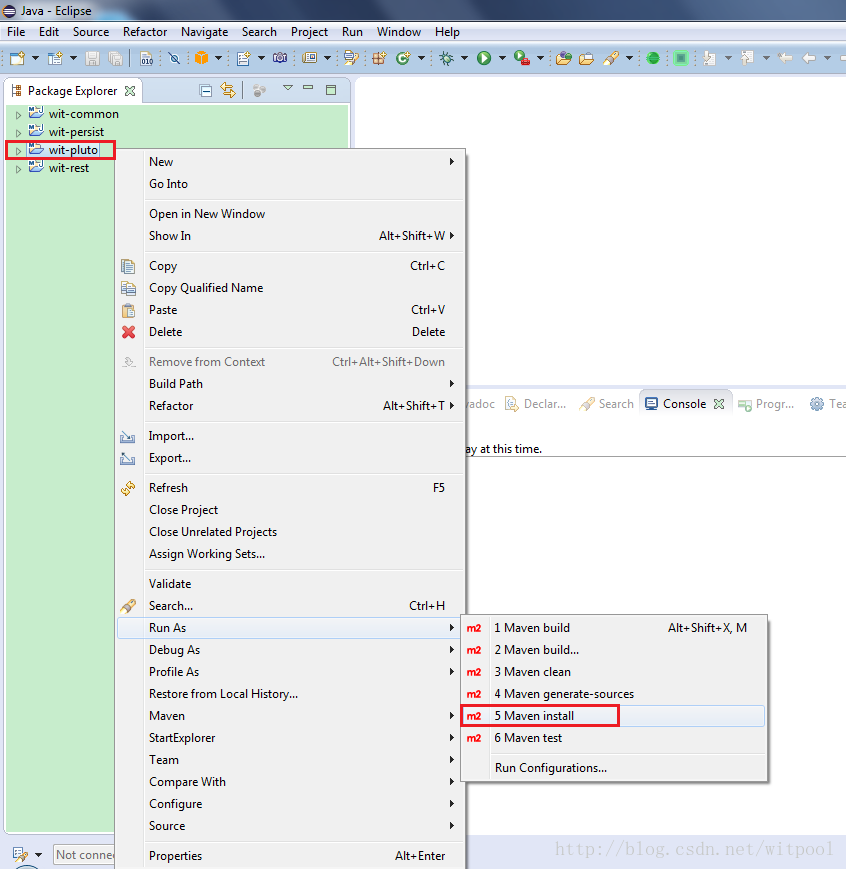
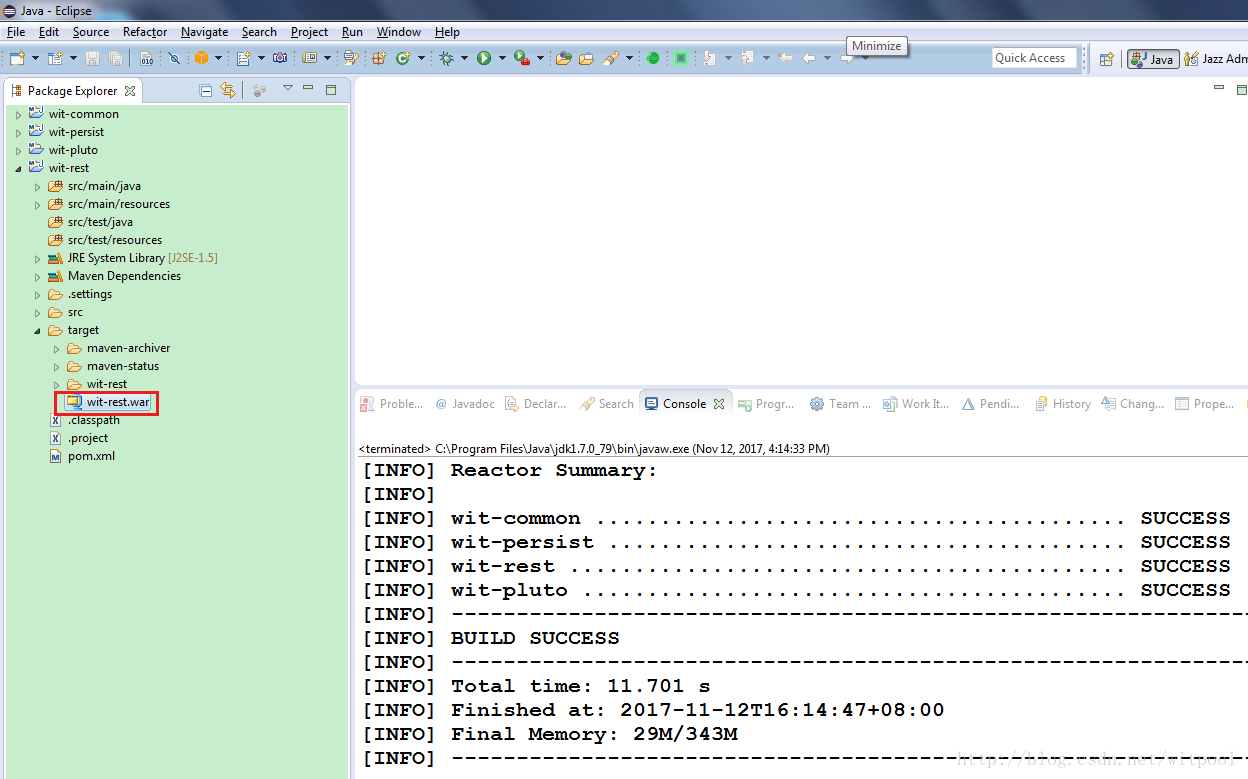
6.部署和UT步骤
将编译生成的包
UT步骤:
(1). 下载Wisdom RESTClient
(2). 双击 JAR包
导入测试用例文件:
/* * Copyright 2016-2017 WitPool.org All Rights Reserved. * * You may not use this file except in compliance with the License. * A copy of the License is located at * http://www.witpool.org/licenses * * or in the "license" file accompanying this file. This file is distributed * on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either * express or implied. See the License for the specific language governing * permissions and limitations under the License. */ package org.witpool.rest; import javax.ws.rs.DELETE; import javax.ws.rs.GET; import javax.ws.rs.POST; import javax.ws.rs.PUT; import javax.ws.rs.Path; import javax.ws.rs.PathParam; import org.witpool.common.model.bean.WitEntity; import org.witpool.common.model.po.WitAccount; /** * @ClassName: IWitAccount * @Description: Account service definition * @author Dom Wang * @date 2017-11-11 AM 11:21:55 * @version 1.0 */ @Path("/account") public interface IWitAccount { /** * * * @Title: addAccount * @Description: Add account * @param @param account * @param @return * @return WitEntity<WitAccount> * @throws */ @POST WitEntity<WitAccount> addAccount(WitAccount account); /** * * * @Title: updateAccount * @Description: Update account * @param @param account * @param @return * @return WitEntity<WitAccount> * @throws */ @PUT WitEntity<WitAccount> updateAccount(WitAccount account); /** * * * @Title: delAccount * @Description: Delete account by user ID * @param @param userId * @param @return * @return WitEntity<WitAccount> * @throws */ @DELETE @Path("/{userId}") WitEntity<WitAccount> delAccount(@PathParam("userId") Integer userId); /** * * * @Title: getAccount * @Description: Query account by user ID * @param @param userId * @param @return * @return WitEntity<WitAccount> * @throws */ @GET @Path("/{userId}") WitEntity<WitAccount> getAccount(@PathParam("userId") Integer userId); /** * * * @Title: getAccount * @Description: Query all the accounts * @param @param userId * @param @return * @return WitEntity<WitAccount> * @throws */ @GET @Path("/all") WitEntity<WitAccount> getAccounts(); }2.CXF与Spring的集成配置
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:jaxrs="http://cxf.apache.org/jaxrs" xmlns:cxf="http://cxf.apache.org/core" xmlns:util="http://www.springframework.org/schema/util" xmlns:p="http://www.springframework.org/schema/p" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/util http://www.springframework.org/schema/util/spring-util.xsd http://cxf.apache.org/jaxrs http://cxf.apache.org/schemas/jaxrs.xsd"> <import resource="classpath*:META-INF/cxf/cxf.xml"/> <import resource="classpath*:META-INF/cxf/cxf-servlet.xml"/> <import resource="classpath:persist-config.xml"/> <jaxrs:server id="witpool" address="/"> <jaxrs:inInterceptors> <bean class="org.apache.cxf.interceptor.LoggingInInterceptor"/> </jaxrs:inInterceptors> <jaxrs:outInterceptors> <bean class="org.apache.cxf.interceptor.LoggingOutInterceptor"/> </jaxrs:outInterceptors> <jaxrs:providers> <ref bean="jacksonProvider"/> </jaxrs:providers> <jaxrs:extensionMappings> <entry key="json" value="application/json"/> <entry key="xml" value="application/xml"/> </jaxrs:extensionMappings> <jaxrs:serviceBeans> <ref bean="witAccount"/> </jaxrs:serviceBeans> </jaxrs:server> <bean id="jaxbAnnotationIntrospector" class="com.fasterxml.jackson.module.jaxb.JaxbAnnotationIntrospector"/> <bean id="jsonmapper" class="org.springframework.http.converter.json.Jackson2ObjectMapperFactoryBean" p:annotationIntrospector-ref="jaxbAnnotationIntrospector"> <property name="featuresToEnable"> <array> <util:constant static-field="com.fasterxml.jackson.databind.SerializationFeature.INDENT_OUTPUT"/> <util:constant static-field="com.fasterxml.jackson.databind.SerializationFeature.WRITE_DATES_AS_TIMESTAMPS"/> </array> </property> <property name="featuresToDisable"> <array> <util:constant static-field="com.fasterxml.jackson.databind.SerializationFeature.WRITE_NULL_MAP_VALUES"/> <util:constant static-field="com.fasterxml.jackson.databind.SerializationFeature.WRITE_EMPTY_JSON_ARRAYS"/> </array> </property> <property name="objectMapper"> <bean class="com.fasterxml.jackson.databind.ObjectMapper"></bean> </property> </bean> <bean id="jacksonProvider" class="com.fasterxml.jackson.jaxrs.json.JacksonJaxbJsonProvider"> <property name="mapper" ref="jsonmapper"/> </bean> <bean id="witAccount" class="org.witpool.rest.impl.WitAccountImpl"/> </beans>3.Spring与Hibernate的集成配置
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:aop="http://www.springframework.org/schema/aop" xmlns:tx="http://www.springframework.org/schema/tx" xmlns:context="http://www.springframework.org/schema/context" xsi:schemaLocation=" http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.1.xsd http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-3.1.xsd http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx-3.1.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-3.1.xsd "> <!-- Scan Bean --> <context:component-scan base-package="org.witpool.common.model.po"> <context:exclude-filter type="annotation" expression="org.springframework.stereotype.Controller"/> </context:component-scan> <bean id="propertyConfigurer" class="org.springframework.beans.factory.config.PropertyPlaceholderConfigurer"> <property name="locations"> <list> <value>classpath:resources.properties</value> </list> </property> </bean> <bean id="dataSource" class="org.logicalcobwebs.proxool.ProxoolDataSource"> <property name="alias" value="proxoolDataSource"/> <property name="driver" value="${connection.driver_class}"/> <property name="driverUrl" value="${connection.url}"/> <property name="user" value="${connection.username}"/> <property name="password" value="${connection.password}"/> <property name="maximumConnectionCount" value="${proxool.maximum.connection.count}"/> <property name="minimumConnectionCount" value="${proxool.minimum.connection.count}"/> <property name="statistics" value="${proxool.statistics}"/> <property name="simultaneousBuildThrottle" value="${proxool.simultaneous.build.throttle}"/> </bean> <bean id="sessionFactory" class="org.springframework.orm.hibernate4.LocalSessionFactoryBean"> <property name="dataSource" ref="dataSource"/> <property name="packagesToScan"> <list> <value>org.witpool.common.model.po</value> </list> </property> <property name="hibernateProperties"> <props> <prop key="hibernate.dialect">${hibernate.dialect}</prop> <prop key="hibernate.show_sql">${hibernate.show_sql}</prop> <prop key="hibernate.format_sql">true</prop> <prop key="hibernate.query.substitutions">${hibernate.query.substitutions}</prop> <prop key="hibernate.default_batch_fetch_size">${hibernate.default_batch_fetch_size}</prop> <prop key="hibernate.max_fetch_depth">${hibernate.max_fetch_depth}</prop> <prop key="hibernate.generate_statistics">${hibernate.generate_statistics}</prop> <prop key="hibernate.bytecode.use_reflection_optimizer">${hibernate.bytecode.use_reflection_optimizer}</prop> <prop key="hibernate.hbm2ddl.auto">${hibernate.bytecode.use_reflection_optimizer}</prop> <prop key="hibernate.hbm2ddl.auto">${hibernate.hbm2ddl.auto}</prop> </props> </property> </bean> <tx:annotation-driven transaction-manager="transactionManager"/> <bean id="transactionManager" class="org.springframework.orm.hibernate4.HibernateTransactionManager"> <property name="sessionFactory" ref="sessionFactory"/> </bean> <aop:config> a681 <aop:pointcut id="rest-api" expression="execution(* org.witpool.rest.*.*(..))"/> <aop:advisor pointcut-ref="rest-api" advice-ref="txAdvice"/> </aop:config> <tx:advice id="txAdvice" transaction-manager="transactionManager"> <tx:attributes> <tx:method name="find*" read-only="false" propagation="NOT_SUPPORTED"/> <tx:method name="query*" read-only="false" propagation="NOT_SUPPORTED"/> <tx:method name="get*" read-only="false" propagation="NOT_SUPPORTED"/> <tx:method name="add" propagation="REQUIRED"/> <tx:method name="add*" propagation="REQUIRED"/> <tx:method name="update*" propagation="REQUIRED"/> <tx:method name="delete" propagation="REQUIRED"/> <tx:method name="delete*" propagation="REQUIRED"/> <tx:method name="save" propagation="REQUIRED"/> <tx:method name="save*" propagation="REQUIRED"/> <tx:method name="*" propagation="REQUIRED"/> </tx:attributes> </tx:advice> <bean id="baseDao" class="org.witpool.persist.dao.impl.BaseDaoImpl"> <property name="sessionFactory" ref="sessionFactory"/> <property name="baseDao" ref="baseDao"/> </bean> </beans>4.Hibernate的参数配置
hibernate.dialect=org.hibernate.dialect.MySQLDialect hibernate.hbm2ddl.auto=update hibernate.show_sql=true hibernate.query.substitutions=true 1, false 0 hibernate.default_batch_fetch_size=16 hibernate.max_fetch_depth=2 hibernate.bytecode.use_reflection_optimizer=true hibernate.cache.use_second_level_cache=true hibernate.cache.use_query_cache=true hibernate.cache.region.factory_class=org.hibernate.cache.EhCacheRegionFactory net.sf.ehcache.configurationResourceName=/ehcache_hibernate.xml hibernate.cache.use_structured_entries=true hibernate.generate_statistics=true connection.driver_class=com.mysql.jdbc.Driver connection.url=jdbc:mysql://localhost:3306/witpool?createDatabaseIfNotExist=true&useUnicode=true&characterEncoding=gbk&zeroDateTimeBehavior=convertToNull&transformedBitIsBoolean=true connection.username=root connection.password=123456 proxool.maximum.connection.count=40 proxool.minimum.connection.count=5 proxool.statistics=1m,15m,1h,1d proxool.simultaneous.build.throttle=305.代码下载、编译、打包
代码下载请访问 GitHub上的 witpool/wit-pluto
导入工程文件、编译、打包步骤如下:
6.部署和UT步骤
将编译生成的包
wit-rest.war复制到
Tomcat 8.5\webapps\,重启 tomcat
UT步骤:
(1). 下载Wisdom RESTClient
(2). 双击 JAR包
restclient-1.1.jar启动工具
导入测试用例文件:
相关文章推荐
- CXF+Spring+Hibernate实现RESTful webservice服务端实例
- CXF+Spring+Hibernate实现RESTful webservice服务端实例
- CXF+Spring+Hibernate实现RESTful webservice服务端示例
- Spring Boot 实现RESTful webservice服务端实例
- Spring Boot 实现RESTful webservice服务端实例
- CXF 开发RESTful WebService服务(Spring+JPA服务端,JAXRS2.0+WebClient客户端)
- CXF和Spring整合实现WebService服务端、客户端
- spring整合cxf实现restful webservice
- CXF和spring整合实现webservice实例
- Spring整合CXF webservice restful 实例
- Spring整合CXF webservice restful实例详解
- Spring Boot 实现RESTful webservice服务端示例
- Spring Boot实现RESTful webservice服务端示例
- Spring Boot 实现Restful webservice服务端示例代码
- CXF和spring整合实现webservice实例(二)
- CXF和spring整合实现webservice实例
- Spring Boot 实现RESTful webservice服务端示例
- Spring整合CXF webservice restful 实例
- 使用CXF与Spring集成实现RESTFul WebService
- spring + springmvc + cxf 实现webservice restful