用C#语言遍历读取和操纵XML文档
2018-03-14 15:10
302 查看
实验环境:visual studio2017
问题:
用C#语言编写控制台应用(.NET Framework)程序,为“Students.xml(该文件在上两篇博客中均写到,由于篇幅所限,不在书写)”为文档增加“总分”与“平均”元素,两元素的文本内容分别是“数学”与“英语”成绩的总分与平均分。
建立控制台应用程序,代码如下所示:
实验10_2.用C#语言编程实现以“Students.xml”为用户文件的Windows窗体登录与注册。
Step1. 创建Windows窗体应用(.NET Framework)程序
Step2. 在项目中添加Windows窗体:XMLBasedLoginRegister,并设置为启动窗体。
修改Program.cs里面 Main()函数的代码:
//Application.Run(newForm1()); //注释此行旧代码
Application.Run(new XMLBasedLoginRegister()); //新增代码,设置启动窗体。
Step3. 在XMLBasedLoginRegister窗体中添加控件并写入代码。
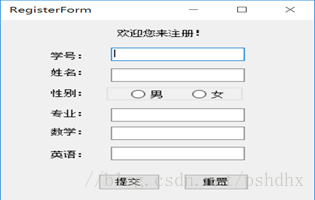
建立窗体应用程序,代码如下所示:注册按钮:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
using System.Xml;
namespace work2
{
public partial class RegisterForm : Form
{
public Regi
4000
sterForm()
{
InitializeComponent();
}
private void label7_Click(object sender, EventArgs e)
{
}
private void button1_Click(object sender, EventArgs e)
{
if ((textBox1.Text == "") || (textBox2.Text == ""))
{
label7.Text = "学号与姓名皆不能为空";
return;
}
string XMLFileName = "C:\\CSharpProg\\Students.xml";
XmlDocument doc = new XmlDocument();
doc.Load(XMLFileName);
XmlElement student = doc.CreateElement("学生");
doc.DocumentElement.AppendChild(student);
XmlElement number = doc.CreateElement("学号");
number.InnerText = textBox1.Text;
student.AppendChild(number);
XmlElement name = doc.CreateElement("姓名");
name.InnerText = textBox2.Text;
student.AppendChild(name);
XmlElement sex = doc.CreateElement("性别");
if (radioButton1.Checked)
{
sex.InnerText = radioButton1.Text;
}
if (radioButton2.Checked)
{
sex.InnerText = radioButton2.Text;
}
student.AppendChild(sex);
XmlElement english = doc.CreateElement("英语");
english.InnerText = textBox4.Text;
student.AppendChild(english);
doc.Save(XMLFileName);
label7.Text = "OK,您已经注册成功!";
MessageBox.Show("OK,您已经注册成功", "完成!");
this.Close();
}
}
}注册按钮.Designer.csnamespace work2
{
partial class RegisterForm
{
/// <summary>
/// Required designer variable.
/// </summary>
private System.ComponentModel.IContainer components = null;
/// <summary>
/// Clean up any resources being used.
/// </summary>
/// <param name="disposing">true if managed resources should be disposed; otherwise, false.</param>
protected override void Dispose(bool disposing)
{
if (disposing && (components != null))
{
components.Dispose();
}
base.Dispose(disposing);
}
#region Windows Form Designer generated code
/// <summary>
/// Required method for Designer support - do not modify
/// the contents of this method with the code editor.
/// </summary>
private void InitializeComponent()
{
this.label1 = new System.Windows.Forms.Label();
this.label2 = new System.Windows.Forms.Label();
this.label3 = new System.Windows.Forms.Label();
this.label4 = new System.Windows.Forms.Label();
this.label5 = new System.Windows.Forms.Label();
this.radioButton1 = new System.Windows.Forms.RadioButton();
this.radioButton2 = new System.Windows.Forms.RadioButton();
this.label6 = new System.Windows.Forms.Label();
this.label7 = new System.Windows.Forms.Label();
this.textBox1 = new System.Windows.Forms.TextBox();
this.textBox2 = new System.Windows.Forms.TextBox();
this.textBox3 = new System.Windows.Forms.TextBox();
this.textBox4 = new System.Windows.Forms.TextBox();
this.textBox5 = new System.Windows.Forms.TextBox();
this.button1 = new System.Windows.Forms.Button();
this.button2 = new System.Windows.Forms.Button();
this.SuspendLayout();
//
// label1
//
this.label1.AutoSize = true;
this.label1.Location = new System.Drawing.Point(168, 52);
this.label1.Name = "label1";
this.label1.Size = new System.Drawing.Size(97, 15);
this.label1.TabIndex = 0;
this.label1.Text = "欢迎您来注册";
//
// label2
//
this.label2.AutoSize = true;
this.label2.Location = new System.Drawing.Point(30, 98);
this.label2.Name = "label2";
this.label2.Size = new System.Drawing.Size(52, 15);
this.label2.TabIndex = 1;
this.label2.Text = "学号:";
//
// label3
//
this.label3.AutoSize = true;
this.label3.Location = new System.Drawing.Point(30, 128);
this.label3.Name = "label3";
this.label3.Size = new System.Drawing.Size(52, 15);
this.label3.TabIndex = 2;
this.label3.Text = "姓名:";
//
// label4
//
this.label4.AutoSize = true;
this.label4.Location = new System.Drawing.Point(30, 191);
this.label4.Name = "label4";
this.label4.Size = new System.Drawing.Size(52, 15);
this.label4.TabIndex = 3;
this.label4.Text = "专业:";
//
// label5
//
this.label5.AutoSize = true;
this.label5.Location = new System.Drawing.Point(30, 160);
this.label5.Name = "label5";
this.label5.Size = new System.Drawing.Size(52, 15);
this.label5.TabIndex = 4;
this.label5.Text = "性别:";
//
// radioButton1
//
this.radioButton1.AutoSize = true;
this.radioButton1.Location = new System.Drawing.Point(119, 160);
this.radioButton1.Name = "radioButton1";
this.radioButton1.Size = new System.Drawing.Size(43, 19);
this.radioButton1.TabIndex = 5;
this.radioButton1.TabStop = true;
this.radioButton1.Text = "男";
this.radioButton1.UseVisualStyleBackColor = true;
//
// radioButton2
//
this.radioButton2.AutoSize = true;
this.radioButton2.Location = new System.Drawing.Point(207, 160);
this.radioButton2.Name = "radioButton2";
this.radioButton2.Size = new System.Drawing.Size(43, 19);
this.radioButton2.TabIndex = 6;
this.radioButton2.TabStop = true;
this.radioButton2.Text = "女";
this.radioButton2.UseVisualStyleBackColor = true;
//
// label6
//
this.label6.AutoSize = true;
this.label6.Location = new System.Drawing.Point(30, 224);
this.label6.Name = "label6";
this.label6.Size = new System.Drawing.Size(52, 15);
this.label6.TabIndex = 7;
this.label6.Text = "数学:";
//
// label7
//
this.label7.AutoSize = true;
this.label7.Location = new System.Drawing.Point(30, 254);
this.label7.Name = "label7";
this.label7.Size = new System.Drawing.Size(52, 15);
this.label7.TabIndex = 8;
this.label7.Text = "英语:";
this.label7.Click += new System.EventHandler(this.label7_Click);
//
// textBox1
//
this.textBox1.Location = new System.Drawing.Point(119, 92);
this.textBox1.Name = "textBox1";
this.textBox1.Size = new System.Drawing.Size(157, 25);
this.textBox1.TabIndex = 9;
//
// textBox2
//
this.textBox2.Location = new System.Drawing.Point(119, 129);
this.textBox2.Name = "textBox2";
this.textBox2.Size = new System.Drawing.Size(157, 25);
this.textBox2.TabIndex = 10;
//
// textBox3
//
this.textBox3.Location = new System.Drawing.Point(120, 185);
this.textBox3.Name = "textBox3";
this.textBox3.Size = new System.Drawing.Size(157, 25);
this.textBox3.TabIndex = 11;
//
// textBox4
//
this.textBox4.Location = new System.Drawing.Point(119, 214);
this.textBox4.Name = "textBox4";
this.textBox4.Size = new System.Drawing.Size(157, 25);
this.textBox4.TabIndex = 12;
//
// textBox5
//
this.textBox5.Location = new System.Drawing.Point(120, 251);
this.textBox5.Name = "textBox5";
this.textBox5.Size = new System.Drawing.Size(157, 25);
this.textBox5.TabIndex = 13;
//
// button1
//
this.button1.Location = new System.Drawing.Point(52, 315);
this.button1.Name = "button1";
this.button1.Size = new System.Drawing.Size(75, 23);
this.button1.TabIndex = 14;
this.button1.Text = "提交";
this.button1.UseVisualStyleBackColor = true;
this.button1.Click += new System.EventHandler(this.button1_Click);
//
// button2
//
this.button2.Location = new System.Drawing.Point(189, 315);
this.button2.Name = "button2";
this.button2.Size = new System.Drawing.Size(75, 23);
this.button2.TabIndex = 15;
this.button2.Text = "重置";
this.button2.UseVisualStyleBackColor = true;
//
// RegisterForm
//
this.AutoScaleDimensions = new System.Drawing.SizeF(8F, 15F);
this.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font;
this.ClientSize = new System.Drawing.Size(482, 365);
this.Controls.Add(this.button2);
this.Controls.Add(this.button1);
this.Controls.Add(this.textBox5);
this.Controls.Add(this.textBox4);
this.Controls.Add(this.textBox3);
this.Controls.Add(this.textBox2);
this.Controls.Add(this.textBox1);
this.Controls.Add(this.label7);
this.Controls.Add(this.label6);
this.Controls.Add(this.radioButton2);
this.Controls.Add(this.radioButton1);
this.Controls.Add(this.label5);
this.Controls.Add(this.label4);
this.Controls.Add(this.label3);
this.Controls.Add(this.label2);
this.Controls.Add(this.label1);
this.Name = "RegisterForm";
this.Text = "RegisterForm";
this.ResumeLayout(false);
this.PerformLayout();
}
#endregion
private System.Windows.Forms.Label label1;
private System.Windows.Forms.Label label2;
private System.Windows.Forms.Label label3;
private System.Windows.Forms.Label label4;
private System.Windows.Forms.Label label5;
private System.Windows.Forms.RadioButton radioButton1;
private System.Windows.Forms.RadioButton radioButton2;
private System.Windows.Forms.Label label6;
private System.Windows.Forms.Label label7;
private System.Windows.Forms.TextBox textBox1;
private System.Windows.Forms.TextBox textBox2;
private System.Windows.Forms.TextBox textBox3;
private System.Windows.Forms.TextBox textBox4;
private System.Windows.Forms.TextBox textBox5;
private System.Windows.Forms.Button button1;
private System.Windows.Forms.Button button2;
}
}登陆按钮.csusing System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
using System.Xml;
namespace work2
{
public partial class XMLBasedLoginRegister : Form
{
public XMLBasedLoginRegister()
{
InitializeComponent();
}
private void label1_Click(object sender, EventArgs e)
{
}
private void button2_Click(object sender, EventArgs e)
{
RegisterForm rf = new RegisterForm();
rf.Show();
}
private void button1_Click(object sender, EventArgs e)
{
bool isstudent = false;
string file = "C:\\CSharpProg\\Students.xml";
XmlDocument doc = new XmlDocument();
doc.Load(file);
XmlElement roster = doc.DocumentElement;
XmlNode studentToSelect = roster.SelectSingleNode("学生/学号[text()='" + textBox1.Text + "']");
if(studentToSelect!=null){
XmlNode pn = studentToSelect.ParentNode;
if(textBox2.Text==pn.ChildNodes[1].InnerText){
isstudent = true;
}
}
if (isstudent)
{
label1.Text = "欢迎您," + textBox2.Text + "同学!";
MessageBox.Show("欢迎您," + textBox2.Text + "同学", "欢迎");
}
else {
label1.Text = "登陆失败,学号或姓名不正确!";
}
}
}
}登陆按钮Designer.csnamespace work2
{
partial class XMLBasedLoginRegister
{
/// <summary>
/// Required designer variable.
/// </summary>
private System.ComponentModel.IContainer components = null;
/// <summary>
/// Clean up any resources being used.
/// </summary>
/// <param name="disposing">true if managed resources should be disposed; otherwise, false.</param>
protected override void Dispose(bool disposing)
{
if (disposing && (components != null))
{
components.Dispose();
}
base.Dispose(disposing);
}
#region Windows Form Designer generated code
/// <summary>
/// Required method for Designer support - do not modify
/// the contents of this method with the code editor.
/// </summary>
private void InitializeComponent()
{
this.label1 = new System.Windows.Forms.Label();
this.label2 = new System.Windows.Forms.Label();
this.label3 = new System.Windows.Forms.Label();
this.textBox1 = new System.Windows.Forms.TextBox();
this.textBox2 = new System.Windows.Forms.TextBox();
this.button1 = new System.Windows.Forms.Button();
this.button2 = new System.Windows.Forms.Button();
this.SuspendLayout();
//
// label1
//
this.label1.AutoSize = true;
this.label1.Location = new System.Drawing.Point(145, 73);
this.label1.Name = "label1";
this.label1.Size = new System.Drawing.Size(181, 15);
this.label1.TabIndex = 0;
this.label1.Text = "基于XML文档的登陆与注册";
this.label1.Click += new System.EventHandler(this.label1_Click);
//
// label2
//
this.label2.AutoSize = true;
this.label2.Location = new System.Drawing.Point(37, 135);
this.label2.Name = "label2";
this.label2.Size = new System.Drawing.Size(52, 15);
this.label2.TabIndex = 1;
this.label2.Text = "学号:";
//
// label3
//
this.label3.AutoSize = true;
this.label3.Location = new System.Drawing.Point(40, 216);
this.label3.Name = "label3";
this.label3.Size = new System.Drawing.Size(52, 15);
this.label3.TabIndex = 2;
this.label3.Text = "姓名:";
//
// textBox1
//
this.textBox1.Location = new System.Drawing.Point(123, 130);
this.textBox1.Name = "textBox1";
this.textBox1.Size = new System.Drawing.Size(182, 25);
this.textBox1.TabIndex = 3;
//
// textBox2
//
this.textBox2.Location = new System.Drawing.Point(123, 211);
this.textBox2.Name = "textBox2";
this.textBox2.Size =
c3c3
new System.Drawing.Size(182, 25);
this.textBox2.TabIndex = 4;
//
// button1
//
this.button1.Location = new System.Drawing.Point(67, 301);
this.button1.Name = "button1";
this.button1.Size = new System.Drawing.Size(75, 23);
this.button1.TabIndex = 5;
this.button1.Text = "登陆";
this.button1.UseVisualStyleBackColor = true;
this.button1.Click += new System.EventHandler(this.button1_Click);
//
// button2
//
this.button2.Location = new System.Drawing.Point(251, 301);
this.button2.Name = "button2";
this.button2.Size = new System.Drawing.Size(75, 23);
this.button2.TabIndex = 6;
this.button2.Text = "注册";
this.button2.UseVisualStyleBackColor = true;
this.button2.Click += new System.EventHandler(this.button2_Click);
//
// XMLBasedLoginRegister
//
this.AutoScaleDimensions = new System.Drawing.SizeF(8F, 15F);
this.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font;
this.ClientSize = new System.Drawing.Size(481, 380);
this.Controls.Add(this.button2);
this.Controls.Add(this.button1);
this.Controls.Add(this.textBox2);
this.Controls.Add(this.textBox1);
this.Controls.Add(this.label3);
this.Controls.Add(this.label2);
this.Controls.Add(this.label1);
this.Name = "XMLBasedLoginRegister";
this.Text = "XMLBasedLoginRegister";
this.ResumeLayout(false);
this.PerformLayout();
}
#endregion
private System.Windows.Forms.Label label1;
private System.Windows.Forms.Label label2;
private System.Windows.Forms.Label label3;
private System.Windows.Forms.TextBox textBox1;
private System.Windows.Forms.TextBox textBox2;
private System.Windows.Forms.Button button1;
private System.Windows.Forms.Button button2;
}
}
实验10_3.用C#语言编写ASP.NET Web窗体页面,完成实验8中用JavaScript所实现的Web页面功能。如下图所示:
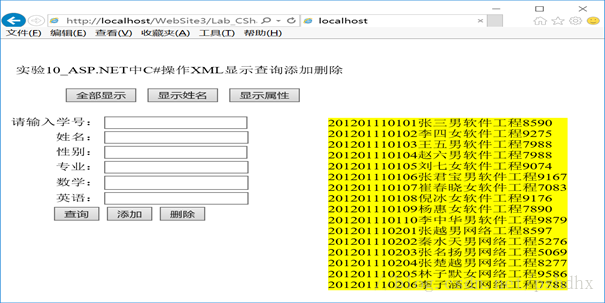
代码如下:
.aspx文件
<%@ Page Title="Home Page" Language="C#" MasterPageFile="~/Site.Master" AutoEventWireup="true" CodeFile="Default.aspx.cs" Inherits="_Default" %>
<asp:Content ID="BodyContent" ContentPlaceHolderID="MainContent" runat="server">
<!DOCTYPE html>
<html>
<head >
<meta http-equiv="Content-Type" content="text/html; charset=utf-8"/>
<title></title>
</head>
<body>
<div>
<asp:Label ID="Label1" runat="server" Text="实验10_ASP.NET中C#操作XML显示查询添加删除"></asp:Label>
</div>
<p>
</p>
<asp:Button ID="Button1" runat="server" Text="全部显示" OnClick="Button1_Click" />
<asp:Button ID="Button2" runat="server" Text="显示姓名" OnClick="Button2_Click" />
<asp:Button ID="Button3" runat="server" Text="显示属性" OnClick="Button3_Click" />
<p>
<asp:Label ID="Label2" runat="server" Text="请输入学号:"></asp:Label>
<asp:TextBox ID="TextBox1" runat="server"></asp:TextBox>
</p>
<asp:Label ID="Label3" runat="server" Text="姓名:"></asp:Label>
<asp:TextBox ID="TextBox2" runat="server"></asp:TextBox>
<p>
<asp:Label ID="Label4" runat="server" Text="性别:"></asp:Label>
<asp:TextBox ID="TextBox3" runat="server"></asp:TextBox>
</p>
<asp:Label ID="Label5" runat="server" Text="专业:"></asp:Label>
<asp:TextBox ID="TextBox4" runat="server"></asp:TextBox>
<p>
<asp:Label ID="Label6" runat="server" Text="数学:"></asp:Label>
<asp:TextBox ID="TextBox5" runat="server"></asp:TextBox>
</p>
<asp:Label ID="Label7" runat="server" Text="英语:"></asp:Label>
<asp:TextBox ID="TextBox6" runat="server"></asp:TextBox>
<p>
<asp:Button ID="Button4" runat="server" Text="查询" OnClick="Button4_Click" />
<asp:Button ID="Button5" runat="server" Text="添加" OnClick="Button5_Click" />
<asp:Button ID="Button6" runat="server" Text="删除" OnClick="Button6_Click" />
</p>
<div id="div1" runat="server" style="float:left;margin-left:400px;background-color:#ccc;margin-top:-200px;height:500px;">
</div>
</body>
</html>
</asp:Content>
aspx.cs文件using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Xml;
public partial class _Default : Page
{
protected void Page_Load(object sender, EventArgs e)
{
}
protected void Button1_Click(object sender, EventArgs e)
{
string content = "";
string file = Server.MapPath("App_Data\\Students.xml");
XmlDocument doc = new XmlDocument();
doc.Load(file);
XmlNodeList nodes = doc.DocumentElement.ChildNodes;
for (int i = 0; i < nodes.Count; i++)
{
content += nodes[i].InnerText + "<br/>\r\n";
}
div1.InnerHtml = content;
}
protected void Button2_Click(object sender, EventArgs e)
{
string content = "";
string file = Server.MapPath("App_Data\\Students.xml");
XmlDocument doc = new XmlDocument();
doc.Load(file);
XmlNodeList nodes = doc.DocumentElement.GetElementsByTagName("姓名");
for (int i = 0; i < nodes.Count;i++ )
{
content += nodes[i].InnerText + "<br/>\r\n";
}
div1.InnerHtml = content;
}
protected void Button3_Click(object sender, EventArgs e)
{
string content = "";
string file = Server.MapPath("App_Data\\Students.xml");
XmlDocument doc = new XmlDocument();
doc.Load(file);
var root = doc.DocumentElement;
var studentList = root.GetElementsByTagName("姓名");
for (int i = 0; i < studentList.Count; i++)
{
//content += nodes[i].InnerText + "<br/>\r\n";
if(studentList[i].Attributes.Count==1){
content += studentList[i].InnerText + "爱好是:" + studentList[i].Attributes[0].InnerText + "<br/>";
}
}
if (content != "")
{
div1.InnerHtml = content;
}
else {
div1.InnerHtml = "未找到爱好信息";
}
//div1.InnerHtml = content;
}
protected void Button4_Click(object sender, EventArgs e)
{
//以下是查询的代码
div1.InnerHtml = "";
string file = Server.MapPath("App_Data\\Students.xml");
XmlDocument doc = new XmlDocument();
doc.Load(file);
XmlElement root = doc.DocumentElement;
XmlNode studentToSelect = root.SelectSingleNode("学生/学号[text()='"+TextBox1.Text+"']");
if(studentToSelect!=null){
XmlNode pn = studentToSelect.ParentNode;
try {
TextBox1.Text = pn.ChildNodes[0].InnerText;
TextBox2.Text = pn.ChildNodes[1].InnerText;
TextBox3.Text = pn.ChildNodes[2].InnerText;
TextBox4.Text = pn.ChildNodes[3].InnerText;
TextBox5.Text = pn.ChildNodes[4].InnerText;
TextBox6.Text = pn.ChildNodes[5].InnerText;
foreach(XmlNode xn in pn.ChildNodes){
div1.InnerHtml += xn.InnerText + "";
Label1.Text = "<span style='font-weight:bold;color:blue;font-size:20px'>查询完毕,找到了!</span>";
}
}catch{
Label1.Text="<span style='font-weight:bold;color:blue;'>该同学数据不完整</span>";
}
}
else{
Label1.Text="<span style='font-weight:bold;color:blue;font-size:30px;'>未找到符合条件的记录</span>";
}
}
protected void Button5_Click(object sender, EventArgs e)
{
if((TextBox1.Text=="")||(TextBox2.Text=="")){
Label1.Text = "学号姓名不能未空!";
return;
}
string XMLFileName = Server.MapPath("App_Data\\Students.xml");
XmlDocument doc = new XmlDocument();
doc.Load(XMLFileName);
XmlElement root = doc.DocumentElement;
XmlNode studentToSelect = root.SelectSingleNode("学生/学号[text()='" + TextBox1.Text + "']");
if(studentToSelect!=null){
Label1.Text = "学号已存在,学生之间学号不能相同,未执行添加!";
return;
}
XmlElement student = doc.CreateElement("学生");
doc.DocumentElement.AppendChild(student);
XmlElement number = doc.CreateElement("学号");
number.InnerText = TextBox1.Text;
student.AppendChild(number);
XmlElement name = doc.CreateElement("姓名");
name.InnerText = TextBox2.Text;
student.AppendChild(name);
XmlElement sex = doc.CreateElement("性别");
sex.InnerText = TextBox3.Text;
student.AppendChild(sex);
XmlElement major = doc.CreateElement("专业");
major.InnerText = TextBox4.Text;
student.AppendChild(major);
XmlElement math = doc.CreateElement("数学");
math.InnerText = TextBox5.Text;
student.AppendChild(math);
XmlElement English = doc.CreateElement("英语");
English.InnerText = TextBox6.Text;
student.AppendChild(English);
doc.Save(XMLFileName);
Label1.Text = "<span style='font-weight:bold;color:blue;font-size:32px;'>成功添加!</span>";
}
protected void Button6_Click(object sender, EventArgs e)
{
string file = Server.MapPath("App_Data\\Students.xml");
XmlDocument doc = new XmlDocument();
doc.Load(file);
XmlElement roster = doc.DocumentElement;
XmlNode studentToSelect = roster.SelectSingleNode("学生/学号[text()='" + TextBox1.Text + "']");
if (studentToSelect != null)
{
XmlNode pn = studentToSelect.ParentNode;
roster.RemoveChild(pn);
doc.Save(file);
Label1.Text = "<span style='font-weight:bold;color:blue;font-size:30px;'>成功删除!</span>";
TextBox2.Text = "";
TextBox3.Text = "";
TextBox4.Text = "";
TextBox5.Text = "";
TextBox6.Text = "";
}
else {
Label1.Text = "<span style='font-weight:bold;color:blue;font-size:30px;'>不能删除,无此记录!</span>";
}
}
}程序结果如图:
显示姓名:
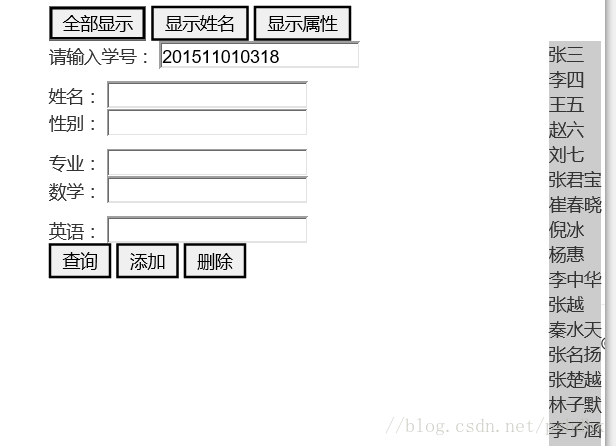
显示爱好属性
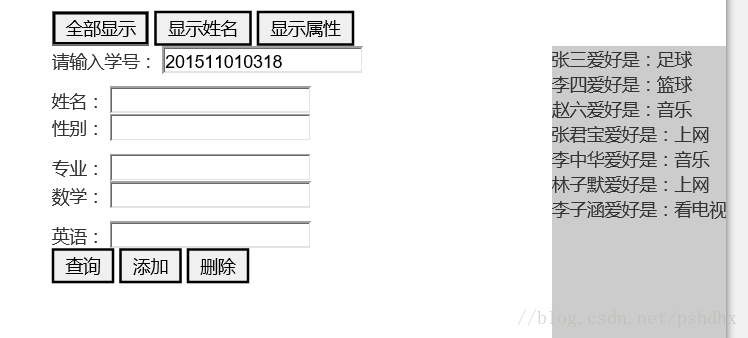
全部显示
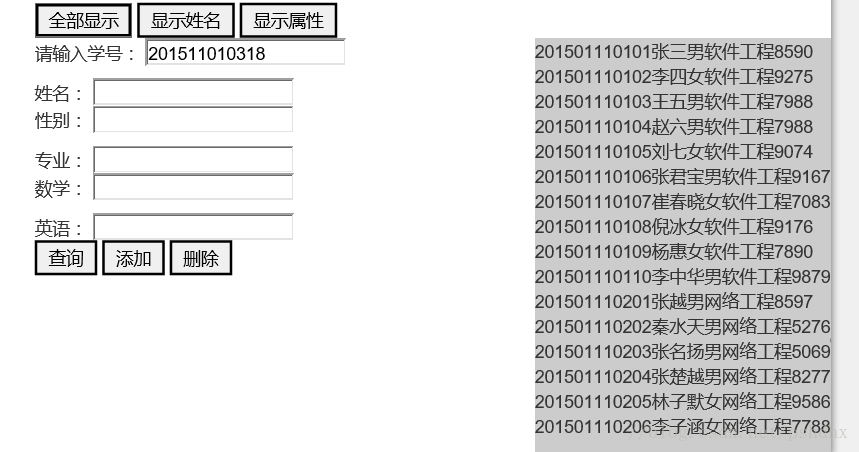
登陆成功
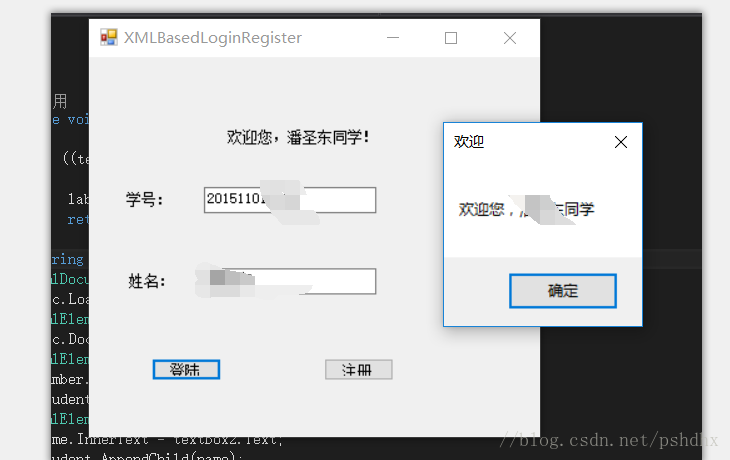
成功查询:
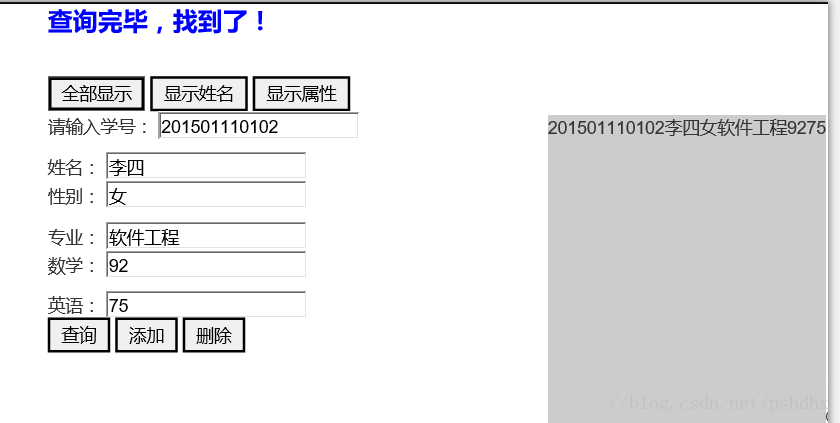
没有此记录,不能删除
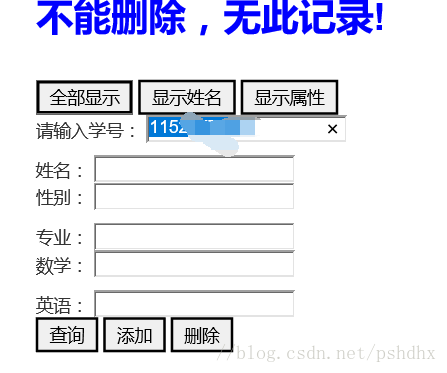
程序总结:
实验过程中,html标签中要加上server,否则在程序中取不到id。
问题:
用C#语言编写控制台应用(.NET Framework)程序,为“Students.xml(该文件在上两篇博客中均写到,由于篇幅所限,不在书写)”为文档增加“总分”与“平均”元素,两元素的文本内容分别是“数学”与“英语”成绩的总分与平均分。
建立控制台应用程序,代码如下所示:
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Xml; namespace work1 { class Program { static void Main(string[] args) { string XMLFileName = "c:\\CSharpProg\\Students.xml"; // XmlDouument doc = new XmlDouument(); XmlDocument doc = new XmlDocument(); doc.Load(XMLFileName); XmlNodeList nodes = doc.DocumentElement.GetElementsByTagName("总分"); if(nodes.Count!=0){ Console.WriteLine("文档中已有"+nodes.Count+"个“总分”节点,无需再添加了!"); Console.WriteLine(); return; } XmlNodeList students = doc.DocumentElement.ChildNodes; for (int i = 0; i < students.Count;i++ ) { double sum = Double.Parse(students[i].ChildNodes[4].InnerText) + Double.Parse(students[i].ChildNodes[5].InnerText) ; double average = sum / 2; XmlElement total = doc.CreateElement("总分"); total.InnerText = sum.ToString(); students[i].AppendChild(total); XmlElement mean = doc.CreateElement("平均"); mean.InnerText = average.ToString(); students[i].AppendChild(mean); } XmlComment c = doc.CreateComment("201511010xxx-xxx实现"); doc.InsertBefore(c,doc.DocumentElement); doc.Save(XMLFileName); Console.WriteLine("ok,成功添加!"); Console.ReadLine(); } } }
实验10_2.用C#语言编程实现以“Students.xml”为用户文件的Windows窗体登录与注册。
Step1. 创建Windows窗体应用(.NET Framework)程序
Step2. 在项目中添加Windows窗体:XMLBasedLoginRegister,并设置为启动窗体。
修改Program.cs里面 Main()函数的代码:
//Application.Run(newForm1()); //注释此行旧代码
Application.Run(new XMLBasedLoginRegister()); //新增代码,设置启动窗体。
Step3. 在XMLBasedLoginRegister窗体中添加控件并写入代码。
建立窗体应用程序,代码如下所示:注册按钮:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
using System.Xml;
namespace work2
{
public partial class RegisterForm : Form
{
public Regi
4000
sterForm()
{
InitializeComponent();
}
private void label7_Click(object sender, EventArgs e)
{
}
private void button1_Click(object sender, EventArgs e)
{
if ((textBox1.Text == "") || (textBox2.Text == ""))
{
label7.Text = "学号与姓名皆不能为空";
return;
}
string XMLFileName = "C:\\CSharpProg\\Students.xml";
XmlDocument doc = new XmlDocument();
doc.Load(XMLFileName);
XmlElement student = doc.CreateElement("学生");
doc.DocumentElement.AppendChild(student);
XmlElement number = doc.CreateElement("学号");
number.InnerText = textBox1.Text;
student.AppendChild(number);
XmlElement name = doc.CreateElement("姓名");
name.InnerText = textBox2.Text;
student.AppendChild(name);
XmlElement sex = doc.CreateElement("性别");
if (radioButton1.Checked)
{
sex.InnerText = radioButton1.Text;
}
if (radioButton2.Checked)
{
sex.InnerText = radioButton2.Text;
}
student.AppendChild(sex);
XmlElement english = doc.CreateElement("英语");
english.InnerText = textBox4.Text;
student.AppendChild(english);
doc.Save(XMLFileName);
label7.Text = "OK,您已经注册成功!";
MessageBox.Show("OK,您已经注册成功", "完成!");
this.Close();
}
}
}注册按钮.Designer.csnamespace work2
{
partial class RegisterForm
{
/// <summary>
/// Required designer variable.
/// </summary>
private System.ComponentModel.IContainer components = null;
/// <summary>
/// Clean up any resources being used.
/// </summary>
/// <param name="disposing">true if managed resources should be disposed; otherwise, false.</param>
protected override void Dispose(bool disposing)
{
if (disposing && (components != null))
{
components.Dispose();
}
base.Dispose(disposing);
}
#region Windows Form Designer generated code
/// <summary>
/// Required method for Designer support - do not modify
/// the contents of this method with the code editor.
/// </summary>
private void InitializeComponent()
{
this.label1 = new System.Windows.Forms.Label();
this.label2 = new System.Windows.Forms.Label();
this.label3 = new System.Windows.Forms.Label();
this.label4 = new System.Windows.Forms.Label();
this.label5 = new System.Windows.Forms.Label();
this.radioButton1 = new System.Windows.Forms.RadioButton();
this.radioButton2 = new System.Windows.Forms.RadioButton();
this.label6 = new System.Windows.Forms.Label();
this.label7 = new System.Windows.Forms.Label();
this.textBox1 = new System.Windows.Forms.TextBox();
this.textBox2 = new System.Windows.Forms.TextBox();
this.textBox3 = new System.Windows.Forms.TextBox();
this.textBox4 = new System.Windows.Forms.TextBox();
this.textBox5 = new System.Windows.Forms.TextBox();
this.button1 = new System.Windows.Forms.Button();
this.button2 = new System.Windows.Forms.Button();
this.SuspendLayout();
//
// label1
//
this.label1.AutoSize = true;
this.label1.Location = new System.Drawing.Point(168, 52);
this.label1.Name = "label1";
this.label1.Size = new System.Drawing.Size(97, 15);
this.label1.TabIndex = 0;
this.label1.Text = "欢迎您来注册";
//
// label2
//
this.label2.AutoSize = true;
this.label2.Location = new System.Drawing.Point(30, 98);
this.label2.Name = "label2";
this.label2.Size = new System.Drawing.Size(52, 15);
this.label2.TabIndex = 1;
this.label2.Text = "学号:";
//
// label3
//
this.label3.AutoSize = true;
this.label3.Location = new System.Drawing.Point(30, 128);
this.label3.Name = "label3";
this.label3.Size = new System.Drawing.Size(52, 15);
this.label3.TabIndex = 2;
this.label3.Text = "姓名:";
//
// label4
//
this.label4.AutoSize = true;
this.label4.Location = new System.Drawing.Point(30, 191);
this.label4.Name = "label4";
this.label4.Size = new System.Drawing.Size(52, 15);
this.label4.TabIndex = 3;
this.label4.Text = "专业:";
//
// label5
//
this.label5.AutoSize = true;
this.label5.Location = new System.Drawing.Point(30, 160);
this.label5.Name = "label5";
this.label5.Size = new System.Drawing.Size(52, 15);
this.label5.TabIndex = 4;
this.label5.Text = "性别:";
//
// radioButton1
//
this.radioButton1.AutoSize = true;
this.radioButton1.Location = new System.Drawing.Point(119, 160);
this.radioButton1.Name = "radioButton1";
this.radioButton1.Size = new System.Drawing.Size(43, 19);
this.radioButton1.TabIndex = 5;
this.radioButton1.TabStop = true;
this.radioButton1.Text = "男";
this.radioButton1.UseVisualStyleBackColor = true;
//
// radioButton2
//
this.radioButton2.AutoSize = true;
this.radioButton2.Location = new System.Drawing.Point(207, 160);
this.radioButton2.Name = "radioButton2";
this.radioButton2.Size = new System.Drawing.Size(43, 19);
this.radioButton2.TabIndex = 6;
this.radioButton2.TabStop = true;
this.radioButton2.Text = "女";
this.radioButton2.UseVisualStyleBackColor = true;
//
// label6
//
this.label6.AutoSize = true;
this.label6.Location = new System.Drawing.Point(30, 224);
this.label6.Name = "label6";
this.label6.Size = new System.Drawing.Size(52, 15);
this.label6.TabIndex = 7;
this.label6.Text = "数学:";
//
// label7
//
this.label7.AutoSize = true;
this.label7.Location = new System.Drawing.Point(30, 254);
this.label7.Name = "label7";
this.label7.Size = new System.Drawing.Size(52, 15);
this.label7.TabIndex = 8;
this.label7.Text = "英语:";
this.label7.Click += new System.EventHandler(this.label7_Click);
//
// textBox1
//
this.textBox1.Location = new System.Drawing.Point(119, 92);
this.textBox1.Name = "textBox1";
this.textBox1.Size = new System.Drawing.Size(157, 25);
this.textBox1.TabIndex = 9;
//
// textBox2
//
this.textBox2.Location = new System.Drawing.Point(119, 129);
this.textBox2.Name = "textBox2";
this.textBox2.Size = new System.Drawing.Size(157, 25);
this.textBox2.TabIndex = 10;
//
// textBox3
//
this.textBox3.Location = new System.Drawing.Point(120, 185);
this.textBox3.Name = "textBox3";
this.textBox3.Size = new System.Drawing.Size(157, 25);
this.textBox3.TabIndex = 11;
//
// textBox4
//
this.textBox4.Location = new System.Drawing.Point(119, 214);
this.textBox4.Name = "textBox4";
this.textBox4.Size = new System.Drawing.Size(157, 25);
this.textBox4.TabIndex = 12;
//
// textBox5
//
this.textBox5.Location = new System.Drawing.Point(120, 251);
this.textBox5.Name = "textBox5";
this.textBox5.Size = new System.Drawing.Size(157, 25);
this.textBox5.TabIndex = 13;
//
// button1
//
this.button1.Location = new System.Drawing.Point(52, 315);
this.button1.Name = "button1";
this.button1.Size = new System.Drawing.Size(75, 23);
this.button1.TabIndex = 14;
this.button1.Text = "提交";
this.button1.UseVisualStyleBackColor = true;
this.button1.Click += new System.EventHandler(this.button1_Click);
//
// button2
//
this.button2.Location = new System.Drawing.Point(189, 315);
this.button2.Name = "button2";
this.button2.Size = new System.Drawing.Size(75, 23);
this.button2.TabIndex = 15;
this.button2.Text = "重置";
this.button2.UseVisualStyleBackColor = true;
//
// RegisterForm
//
this.AutoScaleDimensions = new System.Drawing.SizeF(8F, 15F);
this.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font;
this.ClientSize = new System.Drawing.Size(482, 365);
this.Controls.Add(this.button2);
this.Controls.Add(this.button1);
this.Controls.Add(this.textBox5);
this.Controls.Add(this.textBox4);
this.Controls.Add(this.textBox3);
this.Controls.Add(this.textBox2);
this.Controls.Add(this.textBox1);
this.Controls.Add(this.label7);
this.Controls.Add(this.label6);
this.Controls.Add(this.radioButton2);
this.Controls.Add(this.radioButton1);
this.Controls.Add(this.label5);
this.Controls.Add(this.label4);
this.Controls.Add(this.label3);
this.Controls.Add(this.label2);
this.Controls.Add(this.label1);
this.Name = "RegisterForm";
this.Text = "RegisterForm";
this.ResumeLayout(false);
this.PerformLayout();
}
#endregion
private System.Windows.Forms.Label label1;
private System.Windows.Forms.Label label2;
private System.Windows.Forms.Label label3;
private System.Windows.Forms.Label label4;
private System.Windows.Forms.Label label5;
private System.Windows.Forms.RadioButton radioButton1;
private System.Windows.Forms.RadioButton radioButton2;
private System.Windows.Forms.Label label6;
private System.Windows.Forms.Label label7;
private System.Windows.Forms.TextBox textBox1;
private System.Windows.Forms.TextBox textBox2;
private System.Windows.Forms.TextBox textBox3;
private System.Windows.Forms.TextBox textBox4;
private System.Windows.Forms.TextBox textBox5;
private System.Windows.Forms.Button button1;
private System.Windows.Forms.Button button2;
}
}登陆按钮.csusing System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
using System.Xml;
namespace work2
{
public partial class XMLBasedLoginRegister : Form
{
public XMLBasedLoginRegister()
{
InitializeComponent();
}
private void label1_Click(object sender, EventArgs e)
{
}
private void button2_Click(object sender, EventArgs e)
{
RegisterForm rf = new RegisterForm();
rf.Show();
}
private void button1_Click(object sender, EventArgs e)
{
bool isstudent = false;
string file = "C:\\CSharpProg\\Students.xml";
XmlDocument doc = new XmlDocument();
doc.Load(file);
XmlElement roster = doc.DocumentElement;
XmlNode studentToSelect = roster.SelectSingleNode("学生/学号[text()='" + textBox1.Text + "']");
if(studentToSelect!=null){
XmlNode pn = studentToSelect.ParentNode;
if(textBox2.Text==pn.ChildNodes[1].InnerText){
isstudent = true;
}
}
if (isstudent)
{
label1.Text = "欢迎您," + textBox2.Text + "同学!";
MessageBox.Show("欢迎您," + textBox2.Text + "同学", "欢迎");
}
else {
label1.Text = "登陆失败,学号或姓名不正确!";
}
}
}
}登陆按钮Designer.csnamespace work2
{
partial class XMLBasedLoginRegister
{
/// <summary>
/// Required designer variable.
/// </summary>
private System.ComponentModel.IContainer components = null;
/// <summary>
/// Clean up any resources being used.
/// </summary>
/// <param name="disposing">true if managed resources should be disposed; otherwise, false.</param>
protected override void Dispose(bool disposing)
{
if (disposing && (components != null))
{
components.Dispose();
}
base.Dispose(disposing);
}
#region Windows Form Designer generated code
/// <summary>
/// Required method for Designer support - do not modify
/// the contents of this method with the code editor.
/// </summary>
private void InitializeComponent()
{
this.label1 = new System.Windows.Forms.Label();
this.label2 = new System.Windows.Forms.Label();
this.label3 = new System.Windows.Forms.Label();
this.textBox1 = new System.Windows.Forms.TextBox();
this.textBox2 = new System.Windows.Forms.TextBox();
this.button1 = new System.Windows.Forms.Button();
this.button2 = new System.Windows.Forms.Button();
this.SuspendLayout();
//
// label1
//
this.label1.AutoSize = true;
this.label1.Location = new System.Drawing.Point(145, 73);
this.label1.Name = "label1";
this.label1.Size = new System.Drawing.Size(181, 15);
this.label1.TabIndex = 0;
this.label1.Text = "基于XML文档的登陆与注册";
this.label1.Click += new System.EventHandler(this.label1_Click);
//
// label2
//
this.label2.AutoSize = true;
this.label2.Location = new System.Drawing.Point(37, 135);
this.label2.Name = "label2";
this.label2.Size = new System.Drawing.Size(52, 15);
this.label2.TabIndex = 1;
this.label2.Text = "学号:";
//
// label3
//
this.label3.AutoSize = true;
this.label3.Location = new System.Drawing.Point(40, 216);
this.label3.Name = "label3";
this.label3.Size = new System.Drawing.Size(52, 15);
this.label3.TabIndex = 2;
this.label3.Text = "姓名:";
//
// textBox1
//
this.textBox1.Location = new System.Drawing.Point(123, 130);
this.textBox1.Name = "textBox1";
this.textBox1.Size = new System.Drawing.Size(182, 25);
this.textBox1.TabIndex = 3;
//
// textBox2
//
this.textBox2.Location = new System.Drawing.Point(123, 211);
this.textBox2.Name = "textBox2";
this.textBox2.Size =
c3c3
new System.Drawing.Size(182, 25);
this.textBox2.TabIndex = 4;
//
// button1
//
this.button1.Location = new System.Drawing.Point(67, 301);
this.button1.Name = "button1";
this.button1.Size = new System.Drawing.Size(75, 23);
this.button1.TabIndex = 5;
this.button1.Text = "登陆";
this.button1.UseVisualStyleBackColor = true;
this.button1.Click += new System.EventHandler(this.button1_Click);
//
// button2
//
this.button2.Location = new System.Drawing.Point(251, 301);
this.button2.Name = "button2";
this.button2.Size = new System.Drawing.Size(75, 23);
this.button2.TabIndex = 6;
this.button2.Text = "注册";
this.button2.UseVisualStyleBackColor = true;
this.button2.Click += new System.EventHandler(this.button2_Click);
//
// XMLBasedLoginRegister
//
this.AutoScaleDimensions = new System.Drawing.SizeF(8F, 15F);
this.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font;
this.ClientSize = new System.Drawing.Size(481, 380);
this.Controls.Add(this.button2);
this.Controls.Add(this.button1);
this.Controls.Add(this.textBox2);
this.Controls.Add(this.textBox1);
this.Controls.Add(this.label3);
this.Controls.Add(this.label2);
this.Controls.Add(this.label1);
this.Name = "XMLBasedLoginRegister";
this.Text = "XMLBasedLoginRegister";
this.ResumeLayout(false);
this.PerformLayout();
}
#endregion
private System.Windows.Forms.Label label1;
private System.Windows.Forms.Label label2;
private System.Windows.Forms.Label label3;
private System.Windows.Forms.TextBox textBox1;
private System.Windows.Forms.TextBox textBox2;
private System.Windows.Forms.Button button1;
private System.Windows.Forms.Button button2;
}
}
实验10_3.用C#语言编写ASP.NET Web窗体页面,完成实验8中用JavaScript所实现的Web页面功能。如下图所示:
代码如下:
.aspx文件
<%@ Page Title="Home Page" Language="C#" MasterPageFile="~/Site.Master" AutoEventWireup="true" CodeFile="Default.aspx.cs" Inherits="_Default" %>
<asp:Content ID="BodyContent" ContentPlaceHolderID="MainContent" runat="server">
<!DOCTYPE html>
<html>
<head >
<meta http-equiv="Content-Type" content="text/html; charset=utf-8"/>
<title></title>
</head>
<body>
<div>
<asp:Label ID="Label1" runat="server" Text="实验10_ASP.NET中C#操作XML显示查询添加删除"></asp:Label>
</div>
<p>
</p>
<asp:Button ID="Button1" runat="server" Text="全部显示" OnClick="Button1_Click" />
<asp:Button ID="Button2" runat="server" Text="显示姓名" OnClick="Button2_Click" />
<asp:Button ID="Button3" runat="server" Text="显示属性" OnClick="Button3_Click" />
<p>
<asp:Label ID="Label2" runat="server" Text="请输入学号:"></asp:Label>
<asp:TextBox ID="TextBox1" runat="server"></asp:TextBox>
</p>
<asp:Label ID="Label3" runat="server" Text="姓名:"></asp:Label>
<asp:TextBox ID="TextBox2" runat="server"></asp:TextBox>
<p>
<asp:Label ID="Label4" runat="server" Text="性别:"></asp:Label>
<asp:TextBox ID="TextBox3" runat="server"></asp:TextBox>
</p>
<asp:Label ID="Label5" runat="server" Text="专业:"></asp:Label>
<asp:TextBox ID="TextBox4" runat="server"></asp:TextBox>
<p>
<asp:Label ID="Label6" runat="server" Text="数学:"></asp:Label>
<asp:TextBox ID="TextBox5" runat="server"></asp:TextBox>
</p>
<asp:Label ID="Label7" runat="server" Text="英语:"></asp:Label>
<asp:TextBox ID="TextBox6" runat="server"></asp:TextBox>
<p>
<asp:Button ID="Button4" runat="server" Text="查询" OnClick="Button4_Click" />
<asp:Button ID="Button5" runat="server" Text="添加" OnClick="Button5_Click" />
<asp:Button ID="Button6" runat="server" Text="删除" OnClick="Button6_Click" />
</p>
<div id="div1" runat="server" style="float:left;margin-left:400px;background-color:#ccc;margin-top:-200px;height:500px;">
</div>
</body>
</html>
</asp:Content>
aspx.cs文件using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Xml;
public partial class _Default : Page
{
protected void Page_Load(object sender, EventArgs e)
{
}
protected void Button1_Click(object sender, EventArgs e)
{
string content = "";
string file = Server.MapPath("App_Data\\Students.xml");
XmlDocument doc = new XmlDocument();
doc.Load(file);
XmlNodeList nodes = doc.DocumentElement.ChildNodes;
for (int i = 0; i < nodes.Count; i++)
{
content += nodes[i].InnerText + "<br/>\r\n";
}
div1.InnerHtml = content;
}
protected void Button2_Click(object sender, EventArgs e)
{
string content = "";
string file = Server.MapPath("App_Data\\Students.xml");
XmlDocument doc = new XmlDocument();
doc.Load(file);
XmlNodeList nodes = doc.DocumentElement.GetElementsByTagName("姓名");
for (int i = 0; i < nodes.Count;i++ )
{
content += nodes[i].InnerText + "<br/>\r\n";
}
div1.InnerHtml = content;
}
protected void Button3_Click(object sender, EventArgs e)
{
string content = "";
string file = Server.MapPath("App_Data\\Students.xml");
XmlDocument doc = new XmlDocument();
doc.Load(file);
var root = doc.DocumentElement;
var studentList = root.GetElementsByTagName("姓名");
for (int i = 0; i < studentList.Count; i++)
{
//content += nodes[i].InnerText + "<br/>\r\n";
if(studentList[i].Attributes.Count==1){
content += studentList[i].InnerText + "爱好是:" + studentList[i].Attributes[0].InnerText + "<br/>";
}
}
if (content != "")
{
div1.InnerHtml = content;
}
else {
div1.InnerHtml = "未找到爱好信息";
}
//div1.InnerHtml = content;
}
protected void Button4_Click(object sender, EventArgs e)
{
//以下是查询的代码
div1.InnerHtml = "";
string file = Server.MapPath("App_Data\\Students.xml");
XmlDocument doc = new XmlDocument();
doc.Load(file);
XmlElement root = doc.DocumentElement;
XmlNode studentToSelect = root.SelectSingleNode("学生/学号[text()='"+TextBox1.Text+"']");
if(studentToSelect!=null){
XmlNode pn = studentToSelect.ParentNode;
try {
TextBox1.Text = pn.ChildNodes[0].InnerText;
TextBox2.Text = pn.ChildNodes[1].InnerText;
TextBox3.Text = pn.ChildNodes[2].InnerText;
TextBox4.Text = pn.ChildNodes[3].InnerText;
TextBox5.Text = pn.ChildNodes[4].InnerText;
TextBox6.Text = pn.ChildNodes[5].InnerText;
foreach(XmlNode xn in pn.ChildNodes){
div1.InnerHtml += xn.InnerText + "";
Label1.Text = "<span style='font-weight:bold;color:blue;font-size:20px'>查询完毕,找到了!</span>";
}
}catch{
Label1.Text="<span style='font-weight:bold;color:blue;'>该同学数据不完整</span>";
}
}
else{
Label1.Text="<span style='font-weight:bold;color:blue;font-size:30px;'>未找到符合条件的记录</span>";
}
}
protected void Button5_Click(object sender, EventArgs e)
{
if((TextBox1.Text=="")||(TextBox2.Text=="")){
Label1.Text = "学号姓名不能未空!";
return;
}
string XMLFileName = Server.MapPath("App_Data\\Students.xml");
XmlDocument doc = new XmlDocument();
doc.Load(XMLFileName);
XmlElement root = doc.DocumentElement;
XmlNode studentToSelect = root.SelectSingleNode("学生/学号[text()='" + TextBox1.Text + "']");
if(studentToSelect!=null){
Label1.Text = "学号已存在,学生之间学号不能相同,未执行添加!";
return;
}
XmlElement student = doc.CreateElement("学生");
doc.DocumentElement.AppendChild(student);
XmlElement number = doc.CreateElement("学号");
number.InnerText = TextBox1.Text;
student.AppendChild(number);
XmlElement name = doc.CreateElement("姓名");
name.InnerText = TextBox2.Text;
student.AppendChild(name);
XmlElement sex = doc.CreateElement("性别");
sex.InnerText = TextBox3.Text;
student.AppendChild(sex);
XmlElement major = doc.CreateElement("专业");
major.InnerText = TextBox4.Text;
student.AppendChild(major);
XmlElement math = doc.CreateElement("数学");
math.InnerText = TextBox5.Text;
student.AppendChild(math);
XmlElement English = doc.CreateElement("英语");
English.InnerText = TextBox6.Text;
student.AppendChild(English);
doc.Save(XMLFileName);
Label1.Text = "<span style='font-weight:bold;color:blue;font-size:32px;'>成功添加!</span>";
}
protected void Button6_Click(object sender, EventArgs e)
{
string file = Server.MapPath("App_Data\\Students.xml");
XmlDocument doc = new XmlDocument();
doc.Load(file);
XmlElement roster = doc.DocumentElement;
XmlNode studentToSelect = roster.SelectSingleNode("学生/学号[text()='" + TextBox1.Text + "']");
if (studentToSelect != null)
{
XmlNode pn = studentToSelect.ParentNode;
roster.RemoveChild(pn);
doc.Save(file);
Label1.Text = "<span style='font-weight:bold;color:blue;font-size:30px;'>成功删除!</span>";
TextBox2.Text = "";
TextBox3.Text = "";
TextBox4.Text = "";
TextBox5.Text = "";
TextBox6.Text = "";
}
else {
Label1.Text = "<span style='font-weight:bold;color:blue;font-size:30px;'>不能删除,无此记录!</span>";
}
}
}程序结果如图:
显示姓名:
显示爱好属性
全部显示
登陆成功
成功查询:
没有此记录,不能删除
程序总结:
实验过程中,html标签中要加上server,否则在程序中取不到id。
相关文章推荐
- 用Java语言遍历读取和操纵XML文档
- C#来创建和读取XML文档(二)
- C#来创建和读取XML文档
- C#来创建和读取XML文档
- 艾伟_转载:C#来创建和读取XML文档
- C# 生成和读取Xml文档
- C#操纵XML文档(主要是应用程序的配置文件)
- C#读取XML文档
- c#读取xml文档
- 用C#读取XML文档
- C#来创建和读取XML文档
- C#读取XML文档实例
- 用C#创建XML文档和读取并修改XML文档
- 用C#读取XML文档
- C#来创建和读取XML文档
- 用C#读取XML文档
- 编程技巧——c#.net mvc:如何读取xml文档
- C#读取xml文档数据到DataSet
- C# 读取XML文档
- C#操作读取、写入XML文档的实用方法