Spring Boot + MyBatis + MySQL 整合(1)
2018-03-02 18:06
686 查看
最近在复习之前学的Spring Boot ,在这里结合我之前整合Spring Boot + MyBatis + MySQL 这三者的经验来总结一下,希望对小伙伴们有用。我使用的是采用全注解的方式来实现 MyBatis,这也正好符合 Spring Boot 的思想:使用注解,少用配置文件。好了,下面我们来进行整合吧。
整合步骤
1、在pom.xml文件里面引入 mybatis 和 mysql 的依赖
<!-- 添加 MyBatis -->
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter</artifactId>
<version>1.3.0</version>
</dependency>
<!-- 添加 MySQL -->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>5.1.41</version>
</dependency>2、在application.yml文件里面对数据库进行相应的配置
spring:
datasource:
driver-class-name: com.mysql.jdbc.Driver
username: root
password: xxx
url: jdbc:mysql://127.0.0.1/sell?characterEncoding=utf-8&useSSL=false
server:
context-path: /demo
port: 80884、为了mysql的测试,先在mysql中创建 product_category 表
create table `product_category` (
`category_id` int not null auto_increment,
`category_name` varchar(64) not null comment '类目名字',
`category_type` int not null comment '类目编号',
primary key (`category_id`)
);5、新建一个mapper 文件夹,里面放置mapper文件,新建ProductCategoryMapper,附注释说明
public interface ProductCategoryMapper {
/**
* 以map的方式插入
*
* @param map
* @return
*/
@Insert("insert into product_category(category_name, category_type) values (#{categoryName, jdbcType = VARCHAR}," +
"#{categoryType,jdbcType = INTEGER})")
int insertByMap(Map<String, Object> map);
/**
* 以对象的方式插入
*
* @param productCategory
<
4000
span style="color:#629755;">* @return
*/
@Insert("insert into product_category(category_name,category_type) values (#{categoryName , jdbcType = VARCHAR}," +
"#{productCategory , jdbcType = INTEGER})")
int insertByObject(ProductCategory productCategory);
@Select("select * from product_category where category_type = #{categoryType}")
//查询部分字段信息
@Results({
@Result(column = "category_id", property = "categoryId"),
@Result(column = "category_name", property = "categoryName"),
@Result(column = "category_type", property = "categoryType"),
})
List<ProductCategory> findByCategoryType(Integer type);
@Update("update product_category set category_name = #{categoryName} where category_type = #{categoryType}")
int updateByCategoryType(@Param("categoryName") String categoryName, @Param("categoryType") Integer categoryType);
@Update("update product_category set category_name = #{categoryName} where category_type = #{categoryType}")
int updateByObject(ProductCategory category);
@Delete("delete from product_category where category_type = #{categoryType}")
int deleteByCategoryType(Integer categoryType);
}6、然后编写测试类ProductCategoryMapperTest.java,看看是否测试通过
@RunWith(SpringRunner.class)
@SpringBootTest
public class ProductCategoryMapperTest {
@Autowired
private ProductCategoryMapper productCategoryMapper;
@Test
public void insertByMap() throws Exception {
Map<String, Object> map = new HashedMap();
map.put("categoryName", "我的最爱");
map.put("categoryType", 20);
int insertResult = productCategoryMapper.insertByMap(map);
Assert.assertEquals(1, insertResult);
}
@Test
public void insertByObject() throws Exception{
ProductCategory productCategory = new ProductCategory();
productCategory.setCategoryName("我讨厌的");
productCategory.setCategoryType(17);
int insertResult = productCategoryMapper.insertByObject(productCategory);
Assert.assertEquals(1, insertResult);
}
@Test
public void findByCategoryType() throws Exception {
List<ProductCategory> productCategory = productCategoryMapper.findByCategoryType(17);
Assert.assertNotNull(productCategory);
}
@Test
public void updateByCategoryType() throws Exception {
int updateResult = productCategoryMapper.updateByCategoryType("我讨厌的食物",17);
Assert.assertEquals(1,updateResult);
}
@Test
public void updateByObject() throws Exception {
ProductCategory productCategory = new ProductCategory();
productCategory.setCategoryName("我讨厌的");
productCategory.setCategoryType(17);
int updateResult = productCategoryMapper.updateByObject(productCategory);
Assert.assertEquals(1,updateResult);
}
@Test
public void deleteByCategoryType() throws Exception {
int deleteResult = productCategoryMapper.deleteByCategoryType(20);
Assert.assertEquals(1,deleteResult);
}
}7、其实到了这一步,mapper已经可以直接拿来用了,看到网上不少文章都是到了这一步就直接写controller了,但是,我们一般不这么写,虽然麻烦点,为了开发的规范,我们还是按照dao\service\controller这样的结构来继续完成吧,新建dao文件夹,用来存放dao,新建ProductCategoryDao.java(为了简洁点,这里只做select操作好了)
public class ProductCategoryDao {
@Autowired
private ProductCategoryMapper productCategoryMapper;
public List<ProductCategory> findByCategoryType(Integer type) {
return productCategoryMapper.findByCategoryType(type);
}
}8、然后在service目录下,新建ProductCategoryService 和 ProductCategoryServiceImpl
public interface ProductCategoryService {
List<ProductCategory> findByCategoryType(Integer type);
}
@RestController
public class ProductCategoryController {
@Autowired
private ProductCategoryService categoryService;
@GetMapping("db/findByCategoryType/{type}")
public ResultVO getCategory(@PathVariable("type") Integer type) {
return ResultVOUtil.success(categoryService.findByCategoryType(type));
}
}10、在浏览器上请求这个API: http://localhost:808 9b43
7/demo/db/findByCategoryType/17
得到结果
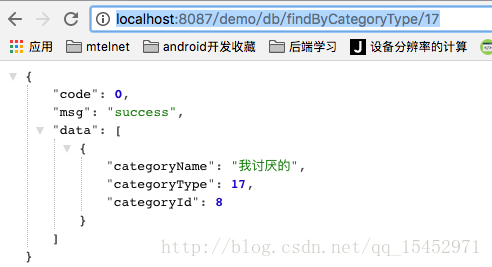
这样就OK了,是不是很简单。这里是用注解来配置mybatis,用XML来配置mybatis虽然现在官方不推荐,但现在还有不少人在用XML配置的方式,稍后再出一篇用XML来配置mybatis的文章。
整合步骤
1、在pom.xml文件里面引入 mybatis 和 mysql 的依赖
<!-- 添加 MyBatis -->
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter</artifactId>
<version>1.3.0</version>
</dependency>
<!-- 添加 MySQL -->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>5.1.41</version>
</dependency>2、在application.yml文件里面对数据库进行相应的配置
spring:
datasource:
driver-class-name: com.mysql.jdbc.Driver
username: root
password: xxx
url: jdbc:mysql://127.0.0.1/sell?characterEncoding=utf-8&useSSL=false
server:
context-path: /demo
port: 80884、为了mysql的测试,先在mysql中创建 product_category 表
create table `product_category` (
`category_id` int not null auto_increment,
`category_name` varchar(64) not null comment '类目名字',
`category_type` int not null comment '类目编号',
primary key (`category_id`)
);5、新建一个mapper 文件夹,里面放置mapper文件,新建ProductCategoryMapper,附注释说明
public interface ProductCategoryMapper {
/**
* 以map的方式插入
*
* @param map
* @return
*/
@Insert("insert into product_category(category_name, category_type) values (#{categoryName, jdbcType = VARCHAR}," +
"#{categoryType,jdbcType = INTEGER})")
int insertByMap(Map<String, Object> map);
/**
* 以对象的方式插入
*
* @param productCategory
<
4000
span style="color:#629755;">* @return
*/
@Insert("insert into product_category(category_name,category_type) values (#{categoryName , jdbcType = VARCHAR}," +
"#{productCategory , jdbcType = INTEGER})")
int insertByObject(ProductCategory productCategory);
@Select("select * from product_category where category_type = #{categoryType}")
//查询部分字段信息
@Results({
@Result(column = "category_id", property = "categoryId"),
@Result(column = "category_name", property = "categoryName"),
@Result(column = "category_type", property = "categoryType"),
})
List<ProductCategory> findByCategoryType(Integer type);
@Update("update product_category set category_name = #{categoryName} where category_type = #{categoryType}")
int updateByCategoryType(@Param("categoryName") String categoryName, @Param("categoryType") Integer categoryType);
@Update("update product_category set category_name = #{categoryName} where category_type = #{categoryType}")
int updateByObject(ProductCategory category);
@Delete("delete from product_category where category_type = #{categoryType}")
int deleteByCategoryType(Integer categoryType);
}6、然后编写测试类ProductCategoryMapperTest.java,看看是否测试通过
@RunWith(SpringRunner.class)
@SpringBootTest
public class ProductCategoryMapperTest {
@Autowired
private ProductCategoryMapper productCategoryMapper;
@Test
public void insertByMap() throws Exception {
Map<String, Object> map = new HashedMap();
map.put("categoryName", "我的最爱");
map.put("categoryType", 20);
int insertResult = productCategoryMapper.insertByMap(map);
Assert.assertEquals(1, insertResult);
}
@Test
public void insertByObject() throws Exception{
ProductCategory productCategory = new ProductCategory();
productCategory.setCategoryName("我讨厌的");
productCategory.setCategoryType(17);
int insertResult = productCategoryMapper.insertByObject(productCategory);
Assert.assertEquals(1, insertResult);
}
@Test
public void findByCategoryType() throws Exception {
List<ProductCategory> productCategory = productCategoryMapper.findByCategoryType(17);
Assert.assertNotNull(productCategory);
}
@Test
public void updateByCategoryType() throws Exception {
int updateResult = productCategoryMapper.updateByCategoryType("我讨厌的食物",17);
Assert.assertEquals(1,updateResult);
}
@Test
public void updateByObject() throws Exception {
ProductCategory productCategory = new ProductCategory();
productCategory.setCategoryName("我讨厌的");
productCategory.setCategoryType(17);
int updateResult = productCategoryMapper.updateByObject(productCategory);
Assert.assertEquals(1,updateResult);
}
@Test
public void deleteByCategoryType() throws Exception {
int deleteResult = productCategoryMapper.deleteByCategoryType(20);
Assert.assertEquals(1,deleteResult);
}
}7、其实到了这一步,mapper已经可以直接拿来用了,看到网上不少文章都是到了这一步就直接写controller了,但是,我们一般不这么写,虽然麻烦点,为了开发的规范,我们还是按照dao\service\controller这样的结构来继续完成吧,新建dao文件夹,用来存放dao,新建ProductCategoryDao.java(为了简洁点,这里只做select操作好了)
public class ProductCategoryDao {
@Autowired
private ProductCategoryMapper productCategoryMapper;
public List<ProductCategory> findByCategoryType(Integer type) {
return productCategoryMapper.findByCategoryType(type);
}
}8、然后在service目录下,新建ProductCategoryService 和 ProductCategoryServiceImpl
public interface ProductCategoryService {
List<ProductCategory> findByCategoryType(Integer type);
}
@Service public class ProductCategoryServiceImpl implements ProductCategoryService { @Autowired private ProductCategoryMapper productCategoryMapper; @Override public List<ProductCategory> findByCategoryType(Integer type) { return productCategoryMapper.findByCategoryType(type); } }9、终于到controller了
@RestController
public class ProductCategoryController {
@Autowired
private ProductCategoryService categoryService;
@GetMapping("db/findByCategoryType/{type}")
public ResultVO getCategory(@PathVariable("type") Integer type) {
return ResultVOUtil.success(categoryService.findByCategoryType(type));
}
}10、在浏览器上请求这个API: http://localhost:808 9b43
7/demo/db/findByCategoryType/17
得到结果
这样就OK了,是不是很简单。这里是用注解来配置mybatis,用XML来配置mybatis虽然现在官方不推荐,但现在还有不少人在用XML配置的方式,稍后再出一篇用XML来配置mybatis的文章。
相关文章推荐
- Spring boot+Shiro+ spring MVC+swagger UI +Mybatis+mysql+Vue +Element UI 之四 vue 整合Element UI
- Spring Boot+Mybatis+MySql 完整整合教程
- gradle+spring-boot+mybatis(基于xml)+mysql 整合
- spring boot整合mybatis使用c3p0数据源连接mysql
- spring boot整合mybatis使用c3p0数据源连接mysql
- spring boot+mybatis+mysql+FreeMarker整合(1)
- spring-boot结合mysql整合mybatis
- Spring Boot + MyBatis + MySQL 整合
- Spring boot----整合mybatis(mysql)
- Spring Boot + MyBatis + MySQL 整合(2)
- Spring Boot 整合 Mybatis 和 MySQL
- SpringBoot整合MyBatis,MySql之从前台页面到数据库的小Demo
- spring boot整合mybatis利用Mysql实现主键UUID的方法
- Spring boot+Shiro+ spring MVC+swagger UI +Mybatis+mysql+Vue +Element UI 之一vue和spring boot整合
- Spring boot + mysql +mybatis 配置整合实例
- springboot和mybatis整合
- SpringCloud SpringBoot mybatis 分布式微服务(二十四)整合Redis
- 一个简单的SpringBoot整合Mybatis项目
- SpringBoot构建微服务实战 之 整合Mybatis(一)
- Spring boot+mybatis整合