Java语言程序设计 第十四章 (14.26、14.27、14.28、14.29)
2018-01-21 13:41
405 查看
程序小白,希望和大家多交流,共同学习
因为没有第十版的汉语电子书,(有的汉语版是第八版,使用的还是Swing)这部分内容只能使用英语版截屏。
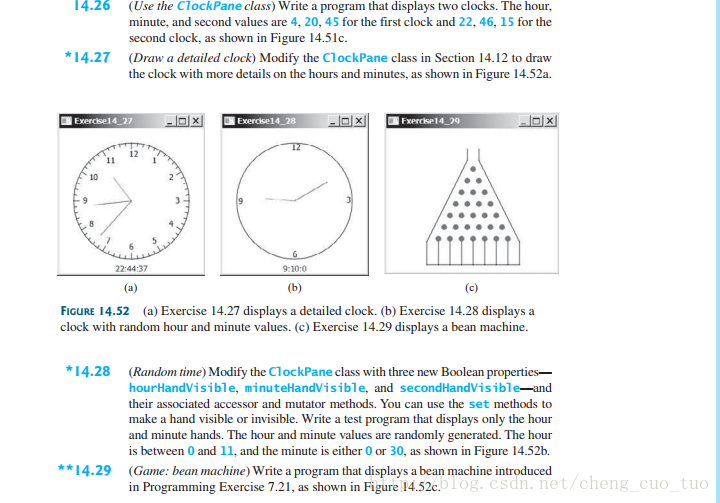
14.26
14.27
14.28
14.29
因为没有第十版的汉语电子书,(有的汉语版是第八版,使用的还是Swing)这部分内容只能使用英语版截屏。
14.26
//继承Pane的时钟面板 import java.util.Calendar; import java.util.GregorianCalendar; import javafx.scene.layout.Pane; import javafx.scene.paint.Color; import javafx.scene.shape.Circle; import javafx.scene.shape.Line; import javafx.scene.text.Text; public class ClockPane extends Pane { private int hour; private int minute; private int second; private double w = 250, h = 250; public ClockPane() { setCurrentTime(); } public ClockPane(int hour, int minute, int second) { this.hour = hour; this.minute = minute; this.second = second; paintClock(); } public int getHour() { return hour; } public void setHour(int hour) { this.hour = hour; paintClock(); } public int getMinute() { return minute; } public void setMinute(int minute) { this.minute = minute; paintClock(); } public int getSecond() { return second; } public void setSecond(int second) { this.second = second; paintClock(); } public double getW() { return w; } public void setW(double w) { this.w = w; paintClock(); } public double getH() { return h; } public void setH(double h) { this.h = h; paintClock(); } public void setCurrentTime() { Calendar calendar = new GregorianCalendar(); this.hour = calendar.get(Calendar.HOUR_OF_DAY); this.minute = calendar.get(Calendar.MINUTE); this.second = calendar.get(Calendar.SECOND); paintClock(); } //画指针 public void paintClock() { double clockRadius = Math.min(w, h) * 0.8 * 0.5; double centerX = w / 2; double centerY = h / 2; Circle circle = new Circle(centerX, centerY, clockRadius); circle.setFill(Color.WHITE); circle.setStroke(Color.BLACK); Text t1 = new Text(centerX - 5, centerY - clockRadius + 12, "12"); Text t2 = new Text(centerX - clockRadius + 3, centerY + 5, "9"); Text t3 = new Text(centerX + clockRadius - 10, centerY + 3, "3"); Text t4 = new Text(centerX - 3, centerY + clockRadius - 3, "6"); double sLength = clockRadius * 0.8; double secondX = centerX + sLength * Math.sin(second * (2 * Math.PI / 60)); double secondY = centerY - sLength * Math.cos(second * (2 * Math.PI / 60)); Line sLine = new Line(centerX, centerY, secondX, secondY); sLine.setStroke(Color.RED); double mLength = clockRadius * 0.65; double minuteX = centerX + mLength * Math.sin(minute * (2 * Math.PI / 60)); double minuteY = centerY - mLength * Math.cos(minute * (2 * Math.PI / 60)); Line mLine = new Line(centerX, centerY, minuteX, minuteY); mLine.setStroke(Color.BLUE); double hLength = clockRadius * 0.5; double hourX = centerX + hLength * Math.sin((hour % 12 + minute / 60.0) * (2 * Math.PI / 12)); double hourY = centerY - hLength * Math.cos((hour % 12 + minute / 60.0) * (2 * Math.PI / 12)); Line hLine = new Line(centerX, centerY, hourX, hourY); hLine.setStroke(Color.GREEN); getChildren().clear(); getChildren().addAll(circle, t1, t2, t3, t4, sLine, mLine, hLine); } }
//使用ClockPane显示给定的两个表盘 import javafx.application.Application; import javafx.stage.Stage; import javafx.scene.Scene; import javafx.scene.layout.Pane; public class ShowTwoClock extends Application { @Override public void start(Stage primaryStage) { Pane pane = new Pane(); ClockPane clock1 = new ClockPane(4, 20, 45); pane.getChildren().add(clock1); Scene scene1 = new Scene(pane, 250, 250); primaryStage.setTitle("Clock1"); primaryStage.setScene(scene1); primaryStage.show(); Stage stage = new Stage(); Pane pane2 = new Pane(); ClockPane clock2 = new ClockPane(22, 46, 15); pane2.getChildren().add(clock2); Scene scene2 = new Scene(pane2, 250, 250); stage.setTitle("Clock2"); stage.setScene(scene2); stage.show(); } }
14.27
//画一个详细的钟 import javafx.scene.layout.Pane; import javafx.scene.paint.Color; import javafx.scene.shape.Line; import javafx.scene.text.Text; import javafx.scene.shape.Circle; import java.util.Calendar; import java.util.GregorianCalendar; public class MoreClockPane extends Pane { private int hour; private int minute; private int second; private double width = 250, height = 250; //默认为当前时间 public MoreClockPane() { Calendar time = new GregorianCalendar(); this.hour = time.get(Calendar.HOUR_OF_DAY); this.minute = time.get(Calendar.MINUTE); this.second = time.get(Calendar.SECOND); paintClock(); } public MoreClockPane(int hour, int minute, int second) { this.hour = hour; this.minute = minute; this.second = second; paintClock(); } public void setHour(int hour) { this.hour = hour; paintClock(); } public int getHour() { return hour; } public void setMinute(int minute) { this.minute = minute; paintClock(); } public int getMinute() { return minute; } public void setSecond(int second) { this.second = second; paintClock(); } public int getSecond() { return second; } public void paintClock() { getChildren().clear(); double centerX = width / 2.0; double centerY = height / 2.0; double radius = Math.min(width, height) * 0.4; Circle clock = new Circle(centerX, centerY, radius); clock.setFill(Color.WHITE); clock.setStroke(Color.BLACK); super.getChildren().add(clock); //画秒针刻度线 double sDegree = 2 * Math.PI / 60; for (int i = 0; i < 60; i++) { if (i % 5 != 0) { double sxs = centerX + radius * Math.sin(sDegree * i) * 0.95; double sys = centerY - radius * Math.cos(sDegree * i) * 0.95; double sxe = centerX + radius * Math.sin(sDegree * i); double sye = centerY - radius * Math.cos(sDegree * i); Line line = new Line(sxs, sys, sxe, sye); super.getChildren().add(line); } } //画分针刻度线,标小时 double mDegree = 2 * Math.PI / 12; for (int i = 1; i <= 12; i++) { double mxs = centerX + radius * Math.sin(mDegree * i) * 0.90; double mys = centerY - radius * Math.cos(mDegree * i) * 0.90; double mxe = centerX + radius * Math.sin(mDegree * i); double mye = centerY - radius * Math.cos(mDegree * i); double txs = centerX + radius * Math.sin(mDegree * i) * 0.80; double tys = centerY - radius * Math.cos(mDegree * i) * 0.80 ; Line line = new Line(mxs, mys, mxe, mye); Text hText = new Text(txs,tys, i + ""); super.getChildren().addAll(line, hText); } //画指针 double sL = radius * 0.8; double secondX = centerX + sL * Math.sin(second * 2 * Math.PI / 60); double secondY = centerY - sL * Math.cos(second * 2 * Math.PI / 60); Line secondLine = new Line(centerX, centerY, secondX, secondY); secondLine.setStroke(Color.RED); double mL = radius * 0.65; double minuteX = centerX + mL * Math.sin(minute * 2 * Math.PI / 60); double minuteY = centerY - mL * Math.cos(minute * 2 * Math.PI / 60); Line minuteLine = new Line(centerX, centerY, minuteX, minuteY); minuteLine.setStroke(Color.BLUE); double hL = radius * 0.6; //注意此处 minute 要除以60.0 不是60,还有时钟除以 12 double hourX = centerX + hL * Math.sin((hour + minute / 60.0) * (2 * Math.PI / 12)); double hourY = centerY - hL * Math.cos((hour + minute / 60.0) * (2 * Math.PI / 12)); Line hourLine = new Line(centerX, centerY, hourX, hourY); hourLine.setStroke(Color.GREEN); super.getChildren().addAll(secondLine, minuteLine, hourLine); } //返回数字时间 public static String getTime() { Calendar time = new GregorianCalendar(); return time.get(Calendar.HOUR_OF_DAY) + ":" + time.get(Calendar.MINUTE) + ":" + time.get(Calendar.SECOND); } }
//测试类展示MoreClockPane import javafx.application.Application; import javafx.stage.Stage; import javafx.scene.Scene; import javafx.scene.control.Label; import javafx.scene.layout.BorderPane; import javafx.geometry.Insets; import javafx.geometry.Pos; public class ShowClockPane extends Application { @Override public void start(Stage primaryStage) { MoreClockPane clock = new MoreClockPane(); Label time = new Label(MoreClockPane.getTime()); BorderPane pane = new BorderPane(); pane.setCenter(clock); pane.setBottom(time); BorderPane.setAlignment(time, Pos.TOP_CENTER); //设置某一的节点的在其所在面板的相对位置,setAlignment是BorderPane的静态方法 Scene scene = new Scene(pane, 250, 250); primaryStage.setTitle("Clock"); primaryStage.setScene(scene); primaryStage.show(); } }
14.28
//新的显示指针表盘的面板 //添加时针分针秒针是否可见 import java.util.Calendar; import java.util.GregorianCalendar; import javafx.scene.layout.Pane; import javafx.scene.paint.Color; import javafx.scene.shape.Circle; import javafx.scene.shape.Line; import javafx.scene.text.Text; public class NewClockPane extends Pane { private int hour; private int minute; private int second; private boolean hourHandVisible; private boolean minuteHandVisible; private boolean secondHandVisible; private double w = 250, h = 250; public NewClockPane() { hourHandVisible = true; minuteHandVisible = true; secondHandVisible = true; setCurrentTime(); } public NewClockPane(int hour, int minute, int second) { hourHandVisible = true; minuteHandVisible = true; secondHandVisible = true; this.hour = hour; this.minute = minute; this.second = second; paintClock(); } public void setHourHandVisible(boolean visible) { this.hourHandVisible = visible; paintClock(); } public void setMinuteHandVisible(boolean visible) { this.minuteHandVisible = visible; paintClock(); } public void setSecondHandVisible(boolean visible) { this.secondHandVisible = visible; paintClock(); } public int getHour() { return hour; } public void setHour(int hour) { this.hour = hour; paintClock(); } public int getMinute() { return minute; } public void setMinute(int minute) { this.minute = minute; paintClock(); } public int getSecond() { return second; } public void setSecond(int second) { this.second = second; paintClock(); } public double getW() { return w; } public void setW(double w) { this.w = w; paintClock(); } public double getH() { return h; } public void setH(double h) { this.h = h; paintClock(); } public void setCurrentTime() { Calendar calendar = new GregorianCalendar(); this.hour = calendar.get(Calendar.HOUR_OF_DAY); this.minute = calendar.get(Calendar.MINUTE); this.second = calendar.get(Calendar.SECOND); paintClock(); } public void paintClock() { double clockRadius = Math.min(w, h) * 0.8 * 0.5; double centerX = w / 2; double centerY = h / 2; Circle circle = new Circle(centerX, centerY, clockRadius); circle.setFill(Color.WHITE); circle.setStroke(Color.BLACK); Text t1 = new Text(centerX - 5, centerY - clockRadius + 12, "12"); Text t2 = new Text(centerX - clockRadius + 3, centerY + 5, "9"); Text t3 = new Text(centerX + clockRadius - 10, centerY + 3, "3"); Text t4 = new Text(centerX - 3, centerY + clockRadius - 3, "6"); double sLength = clockRadius * 0.8; double secondX = centerX + sLength * Math.sin(second * (2 * Math.PI / 60)); double secondY = centerY - sLength * Math.cos(second * (2 * Math.PI / 60)); Line sLine = new Line(centerX, centerY, secondX, secondY); sLine.setStroke(Color.RED); double mLength = clockRadius * 0.65; double minuteX = centerX + mLength * Math.sin(minute * (2 * Math.PI / 60)); double minuteY = centerY - mLength * Math.cos(minute * (2 * Math.PI / 60)); Line mLine = new Line(centerX, centerY, minuteX, minuteY); mLine.setStroke(Color.BLUE); double hLength = clockRadius * 0.5; double hourX = centerX + hLength * Math.sin((hour % 12 + minute / 60.0) * (2 * Math.PI / 12)); double hourY = centerY - hLength * Math.cos((hour % 12 + minute / 60.0) * (2 * Math.PI / 12)); Line hLine = new Line(centerX, centerY, hourX, hourY); hLine.setStroke(Color.GREEN); getChildren().clear(); getChildren().addAll(circle, t1, t2, t3, t4); if (hourHandVisible) { getChildren().add(hLine); } if (minuteHandVisible) { getChildren().add(mLine); } if (secondHandVisible) { getChildren().add(sLine); } } }
//测试新的时钟面板 import javafx.application.Application; import javafx.stage.Stage; import javafx.scene.Scene; import javafx.scene.control.Label; import javafx.scene.layout.BorderPane; import javafx.geometry.Pos; import java.util.Random; public class TestNewClockPane extends Application { @Override public void start(Stage primaryStage) { BorderPane pane = new BorderPane(); NewClockPane clock = new NewClockPane(); Random random = new Random(); int hour = random.nextInt(12); int minuteChoose = random.nextInt(2); int minute; if (minuteChoose == 0) { minute = 0; } else minute = 30; clock.setHour(hour); clock.setMinute(minute); clock.setSecondHandVisible(false); String timeString = clock.getHour() + " : " + clock.getMinute(); Label lblCurrentTime = new Label(timeString); pane.setCenter(clock); pane.setBottom(lblCurrentTime); BorderPane.setAlignment(lblCurrentTime, Pos.TOP_CENTER); Scene scene = new Scene(pane, 250, 250); primaryStage.setTitle("Clock"); primaryStage.setScene(scene); primaryStage.show(); } }
14.29
//画分豆机 //采用的是等边三角形划分各个点 import javafx.application.Application; import javafx.stage.Stage; import javafx.scene.Scene; import javafx.scene.shape.Circle; import javafx.scene.shape.Polyline; import javafx.scene.layout.Pane; import javafx.scene.layout.BorderPane; import javafx.geometry.Insets; import javafx.collections.ObservableList; import javafx.scene.shape.Line; public class DrawBeanDividing extends Application { @Override public void start(Stage primaryStage) { double width = 100; Pane pane = new Pane(); drawInside(pane, width); drawOutside(pane, width); BorderPane borderPane = new BorderPane(); borderPane.setPadding(new Insets(20, 20, 20, 20)); borderPane.setCenter(pane); Scene scene = new Scene(borderPane); primaryStage.setTitle("DrawBeanDividing"); primaryStage.setScene(scene); primaryStage.show(); } //画分豆机的内部 public void drawInside(Pane pane, double width) { for (int i = 1; i <= 7; i++) { double firstCenterX = width / 3 + width - (i - 1) * width / 6; double firstCenterY = 100 + (i - 1) * Math.sqrt(3) / 6 * width; for (int j = 0; j < i; j++) { double centerX = firstCenterX + 2 * j * width / 6; double centerY = firstCenterY; Circle circle = new Circle(centerX, centerY, 5); pane.getChildren().add(circle); if (i == 7) { Line line = new Line(centerX, centerY, centerX, centerY + 100); pane.getChildren().add(line); } } } } //画分豆机的外部 public void drawOutside(Pane pane, double width) { Polyline line = new Polyline(); pane.getChildren().add(line); ObservableList<Double> list = line.getPoints(); //1 list.add(width / 3 + width - width / 6); list.add(0.0); //2 list.add(width / 3 + width - width / 6); list.add(100.0); //3 list.add(0.0); list.add(100 + Math.sqrt(3)* width); //4 list.add(0.0); list.add(100 + Math.sqrt(3)* width + 100); //5 list.add(width + width + width / 3 * 2); list.add(100 + Math.sqrt(3)* width + 100); //6 list.add(width + width + width / 3 * 2); list.add(100 + Math.sqrt(3)* width); //7 list.add(width / 3 + width - width / 6 + width / 3); list.add(100.0); //8 list.add(width / 3 + width - width / 6 + width / 3); list.add(0.0); } }
相关文章推荐
- java语言程序设计 第十四章(14.7、14.8、14.9、14.10、14.11、14.12、14.13、14.14、14.15)
- java语言程序设计 第十四章 (样例代码)
- Java语言程序设计 第十四章 (14.1、14.2、14.3、14.4、12.5、14.6)
- Java语言程序设计 第十四章 (14.16、14.17、14.18、14.19、14.21、14.22、14.23、14.24、14.25)
- Java 语言程序设计 -图形与多媒体处理
- Java 语言程序设计课件【1~7章】(Word版)
- java语言程序设计第十版(Introduce to java 10th) 课后习题 chapter7-23
- Java语言程序设计-基础篇-第八版-编程练习题-第九章
- 3.2 程序设计语言基础-Java
- 【JAVA语言程序设计基础篇】--图形-- 三种时钟--增强对类的理解和应用
- 专业语言:Java程序设计的基本结构
- Java语言程序设计 第二章 (2.2)
- Java语言程序设计 第二章(2.8)
- JAVA语言程序设计基础课后习题第五章
- Java语言程序设计【基础篇】【chapter08_8.7】
- Java语言程序设计 第十五章(15.29、15.30、15.31、15.32)
- Java语言程序设计-进阶篇(四)集合和映射表
- Java 语言程序设计基础篇原书第八版_第十二章_第八题_程序分享
- java语言程序设计 第十五章 (15.1、15.2、15.3、15.4、15.5、15.6、15.7)
- 【JAVA语言程序设计基础篇】--事件驱动程序设计--键盘事件