SpringMVC实现页面和java模型的数据交互以及文件上传下载和数据校验
2018-01-19 19:52
926 查看
1. 项目结构
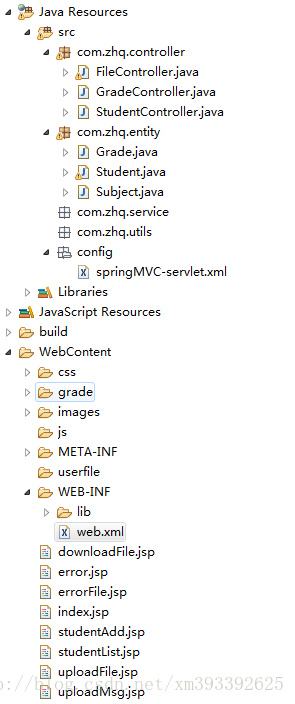
2. springMVC-servlet.xml 配置文件
3. web.xml 主配置文件
4. com.zhq.entity
5. com.zhq.controller
6. 页面
7. 最终效果
2. springMVC-servlet.xml 配置文件
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:tx="http://www.springframework.org/schema/tx" xmlns:context="http://www.springframework.org/schema/context" xmlns:mvc="http://www.springframework.org/schema/mvc" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-4.0.xsd http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx-4.0.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-4.0.xsd http://www.springframework.org/schema/mvc http://www.springframework.org/schema/mvc/spring-mvc-4.0.xsd"> <!-- 启用注解 --> <mvc:annotation-driven/> <context:component-scan base-package="com.zhq.controller"/> <!--配置静态资源访问 --> <mvc:resources location="/images/" mapping="/images/**"/> <mvc:resources location="/css/" mapping="/css/**"/> <mvc:resources location="/js/" mapping="/js/**"/> <!--视图分解器,把controller控制器返回的视图名称加上前缀及后缀,视图的路径最终得到/视图名.jsp --> <bean id="viewResolver" class="org.springframework.web.servlet.view.InternalResourceViewResolver"> <property name="prefix" value="/"></property> <property name="suffix" value=".jsp"></property> </bean> <!--校验器,配置validator --> <bean id="validator" class="org.springframework.validation.beanvalidation.LocalValidatorFactoryBean"> <property name="providerClass" value="org.hibernate.validator.HibernateValidator"/> <property name="validationMessageSource" ref="reloadableResourceBundleMessageSource"/> </bean> <!-- 配置验证消息 --> <bean id="reloadableResourceBundleMessageSource" class="org.springframework.context.support.ReloadableResourceBundleMessageSource"></bean> <!--文件上传的参数配置 --> <bean id="multipartResolver" class="org.springframework.web.multipart.commons.CommonsMultipartResolver"> <!--10485760字节,表示所有文件大小总和不超过10M --> <property name="maxUploadSize" value="10485760"/> <!--设置上传文件时缓存区的大小 --> <property name="maxInMemorySize" value="4096"/> <!--设置字符编码 --> <property name="defaultEncoding" value="UTF-8"/> </bean> <!--文件上传超过大小时的异常处理,页面跳转 --> <bean id="exceptionResolver" class="org.springframework.web.servlet.handler.SimpleMappingExceptionResolver"> <property name="exceptionMappings"> <props> <!--配置捕获异常类型 --> <prop key="org.springframework.web.multipart.MaxUploadSizeExceededException">/errorFile</prop> </props> </property> </bean> </beans>
3. web.xml 主配置文件
<?xml version="1.0" encoding="UTF-8"?> <web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://xmlns.jcp.org/xml/ns/javaee" xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee http://xmlns.jcp.org/xml/ns/javaee/web-app_3_1.xsd" id="WebApp_ID" version="3.1"> <display-name>SpringMVC_01</display-name> <welcome-file-list> <welcome-file>index.html</welcome-file> <welcome-file>index.htm</welcome-file> <welcome-file>index.jsp</welcome-file> <welcome-file>default.html</welcome-file> <welcome-file>default.htm</welcome-file> <welcome-file>default.jsp</welcome-file> </welcome-file-list> <!--配置springMVC的启动 --> <servlet> <!--可以约束springMVC的主配置文件如果在默认路径下时的文件名 --> <servlet-name>springMVC</servlet-name> <!--配置前端控制器 --> <servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class> <!--当主配置文件不在默认路径下,则需要配置文件的路径 --> <init-param> <param-name>contextConfigLocation</param-name> <param-value>classpath:config/springMVC-servlet.xml</param-value> </init-param> <!--配置springMVC 随程序启动而启动 --> <load-on-startup>1</load-on-startup> </servlet> <servlet-mapping> <servlet-name>springMVC</servlet-name> <!--/:表示拦截所有请求 --> <url-pattern>/</url-pattern> </servlet-mapping> <!-- springMVC中文乱码过滤器 --> <filter> <filter-name>characterEncodingFilter</filter-name> <filter-class>org.springframework.web.filter.CharacterEncodingFilter</filter-class> <!--初始化 encoding forceEncoding参数--> <init-param> <param-name>encoding</param-name> <param-value>UTF-8</param-value> </init-param> <init-param> <param-name>forceEncoding</param-name> <param-value>true</param-value> </init-param> </filter> <filter-mapping> <filter-name>characterEncodingFilter</filter-name> <url-pattern>/*</url-pattern> </filter-mapping> <error-page> <error-code>404</error-code> <location>/error.jsp</location> </error-page> </web-app>
4. com.zhq.entity
package com.zhq.entity; import java.util.List; public class Grade { private int gradeId; private String gradeName; private List<Student> students=null; public Grade() { super(); } public Grade(int gradeId, String gradeName) { super(); this.gradeId = gradeId; this.gradeName = gradeName; } public int getGradeId() { return gradeId; } public void setGradeId(int gradeId) { this.gradeId = gradeId; } public String getGradeName() { return gradeName; } public void setGradeName(String gradeName) { this.gradeName = gradeName; } public List<Student> getStudents() { return students; } public void setStudents(List<Student> students) { this.students = students; } @Override public String toString() { return "Grade [gradeId=" + gradeId + ", gradeName=" + gradeName + "]"; } }
package com.zhq.entity; import java.text.SimpleDateFormat; import java.util.Date; import javax.validation.constraints.Size; import org.hibernate.validator.constraints.Email; import org.hibernate.validator.constraints.NotEmpty; import org.hibernate.validator.constraints.Range; import org.springframework.format.annotation.DateTimeFormat; import jdk.nashorn.internal.objects.annotations.SpecializedFunction; public class Student { private int studentNo; @Size(min=2,max=10,message="学生姓名为2-10") private String name; private String sex; @Range(min=18,max=45,message="年龄18-45") private int age; @NotEmpty(message="手机不能为空") private String phone; @NotEmpty(message="地址不能为空") private String address; @DateTimeFormat(pattern="yyyy-MM-dd") private Date birthday; @Email(message="邮箱格式不正确") private String email; private Grade grade; public Student() { super(); } public Student(int studentNo, String name, String sex, int age, String phone, String address ) { super(); this.studentNo = studentNo; this.name = name; this.sex = sex; this.age = age; this.phone = phone; this.address = address; } public int getStudentNo() { return studentNo; } public void setStudentNo(int studentNo) { this.studentNo = studentNo; } public String getName() { return name; } public void setName(String name) { this.name = name; } public String getSex() { return sex; } public void setSex(String sex) { this.sex = sex; } public Date getBirthday() { return birthday; } public void setBirthday(Date birthday) { this.birthday = birthday; } public String getPhone() { return phone; } public void setPhone(String phone) { this.phone = phone; } public String getAddress() { return address; } public void setAddress(String address) { this.address = address; } public String getEmail() { return email; } public void setEmail(String email) { this.email = email; } public Grade getGrade() { return grade; } public void setGrade(Grade grade) { this.grade = grade; } public int getAge() { return age; } public void setAge(int age) { this.age = age; } SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd"); @Override public String toString() { return "Student [studentNo=" + studentNo + ", name=" + name + ", sex=" + sex + ", age=" + age + ", phone=" + phone + ", address=" + address + ", birthday=" + sdf.format(birthday) + ", email=" + email + "]"; } }
package com.zhq.entity; public class Subject { private int subjectNo; private String subjectName; private int classHour; private Grade grade; public int getSubjectNo() { return subjectNo; } public void setSubjectNo(int subjectNo) { this.subjectNo = subjectNo; } public String getSubjectName() { return subjectName; } public void setSubjectName(String subjectName) { this.subjectName = subjectName; } public int getClassHour() { return classHour; } public void setClassHour(int classHour) { this.classHour = classHour; } public Grade getGrade() { return grade; } public void setGrade(Grade grade) { this.grade = grade; } @Override public String toString() { return "Subject [subjectNo=" + subjectNo + ", subjectName=" + subjectName + ", classHour=" + classHour + "]"; } }
5. com.zhq.controller
package com.zhq.controller; import java.io.File; import java.io.FileInputStream; import java.io.FileNotFoundException; import java.io.IOException; import java.io.InputStream; import java.io.OutputStream; import java.io.UnsupportedEncodingException; import java.net.URLEncoder; import java.util.HashMap; import java.util.Iterator; import java.util.Map; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import org.springframework.stereotype.Controller; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.multipart.MultipartFile; import org.springframework.web.multipart.MultipartHttpServletRequest; import org.springframework.web.multipart.commons.CommonsMultipartResolver; @Controller @RequestMapping("/file") public class FileController { //文件上传 @RequestMapping("springUpload") public String springUpload(HttpServletRequest request) { //将当前上下文初始化给CommonsMultipartResolver(多部分解析器) CommonsMultipartResolver multipartResolver=new CommonsMultipartResolver(request.getSession().getServletContext()); //检查form中是否有multipart/form-data if(multipartResolver.isMultipart(request)) { //将request变成多部分request MultipartHttpServletRequest multiRequest=(MultipartHttpServletRequest) request; //获取multiRequest中所有文件名 Iterator iter=multiRequest.getFileNames(); //遍历所有文件 while(iter.hasNext()) { MultipartFile file=multiRequest.getFile(iter.next().toString()); if(file!=null&&file.getOriginalFilename()!="") { String path=request.getSession(). getServletContext().getRealPath("/userfile")+"/"+ file.getOriginalFilename(); //上传 try { file.transferTo(new File(path)); System.out.println(path); request.setAttribute("msg", "上传成功!"); } catch (IllegalStateException e) { e.printStackTrace(); } catch (IOException e) { e.printStackTrace(); } } } }else { request.setAttribute("msg", "上传失败!"); } return "uploadMsg e498 "; } //获取下载文件名列表 @RequestMapping("springFilelist") public String springFilelist(HttpServletRequest request) { //实现思路:先获取userfile目录下所有文件的文件名,保存,然后跳转到downloadFile.jsp展示 //创建集合Map<包含唯一标记的文件名,简短文件名> Map<String,String> fileNames=new HashMap<String,String>(); //获取上传目录,以及其下所有的文件名 String basePath=request.getSession().getServletContext().getRealPath("/userfile")+"/"; //目录 File file=new File(basePath); //目录下,所有文件名 String list[]=file.list(); //遍历,封装 if(list!=null&&list.length>0) { for(int i=0;i<list.length;i++) { //全名 String fileName=list[i]; //短名 String shortName=fileName.substring(fileName.lastIndexOf("\\")+1); //封装 fileNames.put(fileName, shortName); } } //保存到request域 try { request.setCharacterEncoding("UTF-8"); } catch (UnsupportedEncodingException e) { e.printStackTrace(); } request.setAttribute("fileNames", fileNames); return "downloadFile"; } //根据文件名下载文件 @RequestMapping("springDownload") public void springDownload(HttpServletRequest request,HttpServletResponse response) { //获取下载文件名称 String fileName=request.getParameter("fileName"); //GET方式获得的汉字需要转码,框架已经设置所以此处不用 /*fileName = new String(fileName.getBytes("ISO8859-1"),"UTF-8");*/ //先获取上传目录路径 String basePath=request.getSession().getServletContext().getRealPath("/userfile")+"/"; try { //获取一个文件流 InputStream in = new FileInputStream(new File(basePath,fileName)); // 如果文件名是中文,需要进行url编码 fileName = URLEncoder.encode(fileName, "UTF-8"); // 设置下载的响应头 response.setHeader("content-disposition", "attachment;fileName=" + fileName); //获取response字节流 OutputStream out = response.getOutputStream(); byte[] b = new byte[1024]; int len = -1; while ((len = in.read(b)) != -1){ out.write(b, 0, len); } // 关闭 out.close(); in.close(); } catch (FileNotFoundException e) { e.printStackTrace(); } catch (IOException e) { e.printStackTrace(); } } }
package com.zhq.controller; import java.util.ArrayList; import java.util.HashMap; import java.util.List; import java.util.Map; import org.springframework.stereotype.Controller; import org.springframework.ui.Model; import org.springframework.web.bind.annotation.RequestMapping; import com.zhq.entity.Grade; @Controller @RequestMapping("/grade") public class GradeController { //方式一传递数据到前端 @RequestMapping("/list/map")//默认返回grade/list/map.jsp public Map<String,Grade> gradeList(){ Map<String,Grade> map=new HashMap<String, Grade>(); Grade grade=new Grade(); grade.setGradeName("一年级"); map.put("a", grade); return map; } //方式二传递数据到前端 @RequestMapping("/list") public String gradeList(Model model){ List<Grade> gradelist=new ArrayList<Grade>(); Grade grade=new Grade(); grade.setGradeName("二年级"); gradelist.add(grade); model.addAttribute("gradelist", gradelist); return "grade/gradelist";//返回视图grade/gradelist.jsp } }
package com.zhq.controller; import java.util.ArrayList; import java.util.HashMap; import java.util.List; import java.util.Map; /*import javax.servlet.http.HttpServletRequest;*/ import org.springframework.stereotype.Controller; import org.springframework.ui.Model; import org.springframework.validation.BindingResult; import org.springframework.validation.FieldError; import org.springframework.validation.annotation.Validated; import org.springframework.web.bind.annotation.PathVariable; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RequestMethod; import org.springframework.web.servlet.ModelAndView; import com.zhq.entity.Grade; import com.zhq.entity.Student; @Controller @RequestMapping(value= {"/student","/stu"}) public class StudentController { @RequestMapping("/list") public ModelAndView list() { List<Student> list=new ArrayList<Student>(); list.add(new Student(1000, "张三", "男", 20, "13125283736", "广东广州")); list.add(new Student(1001, "李四", "男", 21, "13838384388", "广东珠海")); list.add(new Student(1002, "王婷", "女", 22, "15238384499", "广东中山")); return new ModelAndView("studentList","data",list); } //method=RequestMethod.GET表示只接收GET请求,一般要初始化数据时调用 @RequestMapping(value={"/add","/add1"},method=RequestMethod.GET) public ModelAndView add() { List<Grade> list=new ArrayList<Grade>();//初始化年级下拉框数据 list.add(new Grade(1, "一年级")); list.add(new Grade(2, "二年级")); list.add(new Grade(3, "三年级")); return new ModelAndView("studentAdd","glist",list); } //method=RequestMethod.POST表示只接收POST请求 //@Validated作用就是将pojo内的注解数据校验规则生效,BindingResult用于获取校验失败信息 @RequestMapping(value={"/add","/add2"},method=RequestMethod.POST) public ModelAndView add(@Validated Student student,BindingResult result,Model model) { //传参取值有三种方式(前两种标签名和参数名要相同): //1.ModelAndView add(Student student)自动封装成对象 //2.ModelAndView add(String name, int gradeId)自动匹配参数 //3.ModelAndView add(HttpServletRequest request)获取请求 if(result.hasErrors()) { model.addAttribute("msg", "验证信息错误!"); Map<String,String> errors=new HashMap<String,String>(); for(FieldError err:result.getFieldErrors()) {//遍历错误提示将错误信息存入Map errors.put(err.getField(), err.getDefaultMessage()); } model.addAttribute("errors", errors); List<Grade> list=new ArrayList<Grade>();//年级下拉框信息 list.add(new Grade(1, "一年级")); list.add(new Grade(2, "二年级")); list.add(new Grade(3, "三年级")); model.addAttribute("glist", list); return new ModelAndView("studentAdd"); /*return new ModelAndView("forward:/student/add1");*/ }else { //转发到/student/list方法或者使用redirect:/student/list return new ModelAndView("redirect:/student/list"); } } //占位符方式传递参数/del/100 @RequestMapping("/del/{id:\\d+}")//\\d+表示只匹配数字 public ModelAndView delete(@PathVariable(value="id") Integer id) { System.out.println(id); return new ModelAndView("redirect:/student/list","id",id); } //默认传递参数方式/find?id=100 @RequestMapping("/find") public ModelAndView findStudentByid(Integer id) { System.out.println(id); return new ModelAndView("studentAdd","id",id); } }
6. 页面
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Insert title here</title> <link rel="stylesheet" href="css/index.css" /> </head> <body> <img src="images/ws.gif"><br> <a href="${pageContext.request.contextPath}/student/list">学生信息列表</a><br> <a href="${pageContext.request.contextPath}/student/add">添加学生</a><br> <a href="${pageContext.request.contextPath}/grade/list/map">年级列表1</a><br> <a href="${pageContext.request.contextPath}/grade/list">年级列表2</a><br> <a href="${pageContext.request.contextPath}/uploadFile.jsp">多文件上传</a><br> <a href="${pageContext.request.contextPath}/file/springFilelist">文件下载</a><br> </body> </html>
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Insert title here</title> <style type="text/css"> table {border-collapse:collapse;} table,th,td {border: 1px solid black;} </style> </head> <body> 欢迎! <table> <tr> <th>姓名</th> <th>性别</th> <th>年龄</th> <th>电话</th> <th>地址</th> <th>操作</th> </tr> <c:forEach items="${data}" var="student" > <tr> <td>${student.name}</td> <td>${student.sex}</td> <td>${student.age}</td> <td>${student.phone}</td> <td>${student.address}</td> <td><a href="${pageContext.request.contextPath}/student/del/${student.studentNo}" onclick="return confirm('你确定要删除吗?')">删除</a></td> </tr> </c:forEach> </table> </body> </html>
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Insert title here</title> </head> <body> 添加页面! <form action="${pageContext.request.contextPath}/student/add" method="post"> <p>姓名:<input name="name">${errors.name}<br></p> <p>年龄:<input name="age">${errors.age}<br></p> <p>性别: <select name="sex"> <option value="男">男</option> <option value="女">女</option> </select> </p> <p>地址:<input name="address">${errors.address}<br></p> <p>班级:
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Insert title here</title> </head> <body> 添加页面! <form action="${pageContext.request.contextPath}/student/add" method="post"> <p>姓名:<input name="name">${errors.name}<br></p> <p>年龄:<input name="age">${errors.age}<br></p> <p>性别: <select name="sex"> <option value="男">男</option> <option value="女">女</option> </select> </p> <p>地址:<input name="address">${errors.address}<br></p> <p>班级:
<select name="grade.gradeId">
<c:forEach items="${glist}" var="grade">
<option value="${grade.gradeId}">${grade.gradeName}</option>
</c:forEach>
</select><br>
</p>
<p><input type="submit" value="保存">${msg}<br></p>
</form>
</body>
</html>
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Insert title here</title> </head> <body> <c:forEach items="${map}" var="entry"> ${entry.key}:${entry.value.gradeName}<br> </c:forEach> ${a.gradeName}<br> </body> </html>
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Insert title here</title> </head> <body> <c:forEach items="${gradelist}" var="grade"> ${grade.gradeName}<br> </c:forEach> </body> </html>
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Insert title here</title> </head> <body> <h2>多文件上传</h2> <form action="${pageContext.request.contextPath}/file/springUpload" name="uploadFile" enctype="multipart/form-data" method="post"> <p><input type="file" name="file1"></p> <p><input type="file" name="file2"></p> <p><input type="file" name="file3"></p> <input type="submit" value="上传"> </form> </body> </html>
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Insert title here</title> </head> <body> ${msg} </body> </html>
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Insert title here</title> </head> <body> 文件超出大小10M!上传失败! <a href="${pageContext.request.contextPath}/uploadFile.jsp">返回</a> </body> </html>
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>下载页面</title> <style type="text/css"> table {border-collapse:collapse;} table,th,td {border: 1px solid black;} </style> </head> <body> <h3 align="center">下载列表</h3> <table align="center"> <tr> <th>序号</th> <th>文件名</th> <th>操作</th> </tr> <c:forEach var="en" items="${requestScope.fileNames}" varStatus="vs"> <tr> <td>${vs.count }</td> <td>${en.value }</td> <td> <%--<a href="${pageContext.request.contextPath }/springDownload?method=down&..">下载</a>--%> <!-- 构建一个地址 --> <c:url var="url" value="springDownload"> <c:param name="method" value="down"></c:param> <c:param name="fileName" value="${en.key}"></c:param> </c:url> <!-- 使用上面地址 --> <a href="${url}">下载</a> </td> </tr> </c:forEach> </table> </body> </html>
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Insert title here</title> </head> <body> 错误页面!404 </body> </html>
7. 最终效果
相关文章推荐
- java利用jcraft实现和远程服务器交互,实现上传下载文件
- Java 文件上传 以及 springmvc实现文件上传
- SpringMVC整合fastdfs-client-java实现web文件上传下载
- SpringMVC整合fastdfs-client-java实现web文件上传下载
- java操作hdfs,实现文件上传、下载以及查看当前文件夹下所有文件
- ftp 实现文件的上传下载以及列出文件列表Java代码(未测试)
- SpringMVC 实现POI读取Excle文件中数据导入数据库(上传)、导出数据库中数据到Excle文件中(下载)
- java文件上传(文件大小校验)、下载、删除的实现代码
- springMVC高级部分(数据校验,数据错误回显(自定义格式错误显示),拦截器,异常处理,文件上传,文件下载,springmvc运行流程以及springmvc和struts2对比)
- SpringMVC 实现POI读取Excle文件中数据导入数据库(上传)、导出数据库中数据到Excle文件中(下载)
- ftp 实现文件的上传下载以及列出文件列表Java代码
- 使用ajaxFileUpload与SpringMVC实现异步上传下载文件并返回json数据
- Java中实现FTP文件上传下载 涉及中文路径以及中文文件
- SpringMVC整合fastdfs-client-java实现web文件上传下载
- SpringMVC整合fastdfs-client-java实现web文件上传下载
- Java导出页面数据或数据库数据至Excel文件并下载,采用JXL技术,小demo(servlet实现)
- java实现简单文件复制(文件上传)以及springMVC中上传文件方式
- Java中如何使用组件实现文件上传下载
- java中如何实现文件打包上传以及自动解压
- JAVA 文件上传下载笔记之使用基本IO流实现上传