SpringBoot学习-(4)集成SpringDataJpa
2018-01-13 22:01
525 查看
这里面需要知道什么是JPA,什么是SpringData,什么是Hibernate,什么是SpringDataJpa,如果不知道的,还是要补充一下这块内容。
前面几篇没有介绍有关配置文件的事儿,比如我想修改端口怎么改,修改访问路径怎么改,连接数据库的参数在哪里配置,本篇文章都会有介绍。
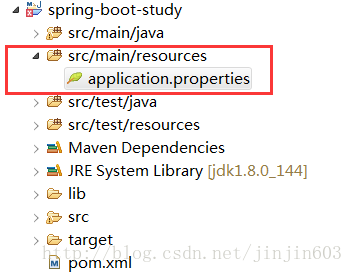
之前的配置不再展示,详情看springboot系列前面的几篇内容
UserInfo
这里配置@Entity标记实体@Table设置对应的数据库表@Id指定id@GeneratedValue指定id自增。
运行App启动springboot,自动创建数据表springboot_userinfo
2.添加数据
在表中添加数据:

3.创建repository,service,controller三层
UserInfoRepository
UserInfoService
UserInfoServiceImpl
UserInfoController
4.启动项目访问测试

这里具体的每个增删改查的测试就不一一列举了。
前面几篇没有介绍有关配置文件的事儿,比如我想修改端口怎么改,修改访问路径怎么改,连接数据库的参数在哪里配置,本篇文章都会有介绍。
1.创建配置文件application.properties
springboot的配置文件默认application.properties(其他名字不行)。存放在src/main/resources目录下。2.添加依赖
pom.xml之前的配置不再展示,详情看springboot系列前面的几篇内容
<!-- mysql --> <dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> </dependency> <!-- spring-data-jpa --> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-data-jpa</artifactId> </dependency>
3.配置数据源
打开application.properties配置文件#配置访问端口 server.port=8080 #访问路径配置 server.context-path=/springboot #配置数据源 spring.datasource.driver-class-name=com.mysql.jdbc.Driver spring.datasource.url=jdbc:mysql://localhost:3306/td_xkd spring.datasource.username=root spring.datasource.password=1234 spring.datasource.dbcp.max-active=20 spring.datasource.dbcp.max-idle=8 spring.datasource.dbcp.min-idle=8 #jpa spring.jpa.hibernate.ddl-auto=update spring.jpa.show-sql=true
4.CRUD小例子
1.修改实体类UserInfo
这里配置@Entity标记实体@Table设置对应的数据库表@Id指定id@GeneratedValue指定id自增。
package com.tang.bean; import java.util.Date; import javax.persistence.Entity; import javax.persistence.GeneratedValue; import javax.persistence.Id; import javax.persistence.Table; import com.alibaba.fastjson.annotation.JSONField; @Entity @Table(name="springboot_userinfo") public class UserInfo { @Id @GeneratedValue private Integer id; private String userName; private String loginName; //不返回json @JSONField(serialize=false) private String password; private Integer age; @JSONField(format="yyyy-MM-dd HH:mm") private Date birthDate; public Integer getId() { return id; } public void setId(Integer id) { this.id = id; } public String getUserName() { return userName; } public void setUserName(String userName) { this.userName = userName; } public String getLoginName() { return loginName; } public void setLoginName(String loginName) { this.loginName = loginName; } public String getPassword() { return password; } public void setPassword(String password) { this.password = password; } public Integer getAge() { return age; } public void setAge(Integer age) { this.age = age; } public Date getBirthDate() { return birthDate; } public void setBirthDate(Date birthDate) { this.birthDate = birthDate; } @O 4000 verride public String toString() { return "UserInfo [id=" + id + ", userName=" + userName + ", loginName=" + loginName + ", password=" + password + ", age=" + age + ", birthDate=" + birthDate + "]"; } }
运行App启动springboot,自动创建数据表springboot_userinfo
2.添加数据
在表中添加数据:
3.创建repository,service,controller三层
UserInfoRepository
package com.tang.repository; import org.springframework.data.jpa.repository.JpaRepository; import com.tang.bean.UserInfo; public interface UserInfoRepository extends JpaRepository<UserInfo, Integer>{ }
UserInfoService
package com.tang.service; import java.util.List; import com.tang.bean.UserInfo; public interface UserInfoService { public UserInfo addUser(UserInfo user); public List<UserInfo> getAllUser(); public UserInfo getOneUser(Integer id); public void deleteUser(Integer id); public UserInfo updateUser(UserInfo user); }
UserInfoServiceImpl
package com.tang.service.impl; import java.util.List; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Service; import com.tang.bean.UserInfo; import com.tang.repository.UserInfoRepository; import com.tang.service.UserInfoService; @Service public class UserInfoServiceImpl implements UserInfoService{ @Autowired private UserInfoRepository userInfoRepository; public UserInfo addUser(UserInfo user) { return userInfoRepository.save(user); } public List<UserInfo> getAllUser() { return userInfoRepository.findAll(); } public UserInfo getOneUser(Integer id) { return userInfoRepository.findOne(id); } public void deleteUser(Integer id) { userInfoRepository.delete(id); } public UserInfo updateUser(UserInfo user) { return userInfoRepository.save(user); } }
UserInfoController
package com.tang.controller; import java.util.Date; import java.util.List; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.PathVariable; import org.springframework.web.bind.annotation.PostMapping; import org.springframework.web.bind.annotation.RestController; import com.tang.bean.UserInfo; import com.tang.service.UserInfoService; @RestController public class UserInfoController { @Autowired private UserInfoService userInfoService; @GetMapping("/getAllUser") public List<UserInfo> getAllUserInfo(){ return userInfoService.getAllUser(); } @GetMapping("/getOneUser/{uid}") public UserInfo getUserInfo(@PathVariable("uid") Integer id){ return userInfoService.getOneUser(id); } @PostMapping("/addUser") public UserInfo addUser(UserInfo user){ return userInfoService.addUser(user); } }
4.启动项目访问测试
这里具体的每个增删改查的测试就不一一列举了。
相关文章推荐
- 【系统学习SpringBoot】再遇Spring Data JPA之JPA应用详解(自定义查询及复杂查询)
- 深入学习spring-boot系列(二)--使用spring-data-jpa
- springboot学习三(springboot结合spring data jpa和freemarker显示)
- 深入学习spring-boot系列(二)--使用spring-data-jpa
- 深入学习spring-boot系列(二)--使用spring-data-jpa
- 【SpringBoot学习笔记】SpringBoot_03_SpringData—JpaRepository部分字段查询功能
- 深入学习spring-boot系列(二)--使用spring-data-jpa
- springboot集成spring-data-jpa
- 深入学习spring-boot系列(二)--使用spring-data-jpa
- 深入学习spring-boot系列(二)--使用spring-data-jpa
- springboot 学习笔记【3】Spring Boot中使用Spring-data-jpa
- Spring Boot 系列(九)数据层-集成Spring-data-jpa
- 【系统学习SpringBoot】SpringBoot初遇Spring-Data-JPA
- 深入学习spring-boot系列(二)--使用spring-data-jpa
- springboot学习-springboot使用spring-data-jpa操作MySQL数据库
- 深入学习spring-boot系列(二)--使用spring-data-jpa
- 深入学习spring-boot系列(二)--使用spring-data-jpa
- 深入学习spring-boot系列(二)--使用spring-data-jpa
- 深入学习spring-boot系列(二)--使用spring-data-jpa
- SpringBoot学习笔记 - 数据访问(Spring Data JPA)