Spring(10):使用注解实现IoC配置与示例
2017-12-30 17:02
465 查看
2017/12/30
Spring框架可以利用XML文件进行IoC配置,但从2.0版本开始引入了注解的配置方式--将Bean的配置信息和Bean实现类结合在一起,可以进一步减少配置文件的代码量。
【1】导入必要的jar包
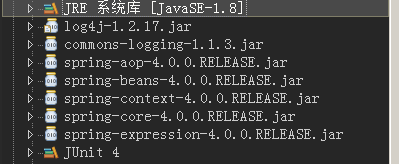
文件框架:
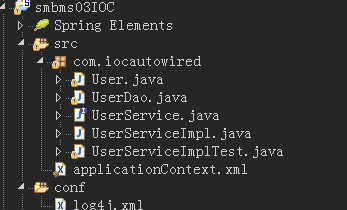
【2】新建User.java / UserDao.java / UserService.java接口 / 实现类UserServiceImpl.java :
User.java :
package com.iocautowired;
import java.util.Date;
import java.util.List;
import org.springframework.stereotype.Component;
@Component("user")
public class User {
private Integer id;
private String userCode;
private String userName;
private String userPassword;
private Integer gender;
private Date birthday;
private String phone;
private String address ;
private Integer userRole;
private Integer createdBy;
private Date creationDate;
private Integer modifyBy;
private Date modifyDate;
private String userRoleName;
public String getUserRoleName() {
return userRoleName;
}
public void setUserRoleName(String userRoleName) {
this.userRoleName = userRoleName;
}
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public String getUserCode() {
return userCode;
}
public void setUserCode(String userCode) {
this.userCode = userCode;
}
public String getUserName() {
return userName;
}
public void setUserName(String userName) {
this.userName = userName;
}
public String getUserPassword() {
return userPassword;
}
public void setUserPassword(String userPassword) {
this.userPassword = userPassword;
}
public Integer getGender() {
return gender;
}
public void setGender(Integer gender) {
this.gender = gender;
}
public Date getBirthday() {
return birthday;
}
public void setBirthday(Date birthday) {
this.birthday = birthday;
}
public String getPhone() {
return phone;
}
public void setPhone(String phone) {
this.phone = phone;
}
public String getAddress() {
return address;
}
public void setAddress(String address) {
this.address = address;
}
public Integer getUserRole() {
return userRole;
}
public void setUserRole(Integer userRole) {
this.userRole = userRole;
}
public Integer getCreatedBy() {
return createdBy;
}
public void setCreatedBy(Integer createdBy) {
this.createdBy = createdBy;
}
public Date getCreationDate() {
return creationDate;
}
public void setCreationDate(Date creationDate) {
this.creationDate = creationDate;
}
public Integer getModifyBy() {
return modifyBy;
}
public void setModifyBy(Integer modifyBy) {
this.modifyBy = modifyBy;
}
public Date getModifyDate() {
return modifyDate;
}
public void setModifyDate(Date modifyDate) {
this.modifyDate = modifyDate;
}
public User(Integer id, String userCode, String userName, String userPassword, Integer gender, Date birthday,
String phone, String address, Integer userRole, Integer createdBy, Date creationDate, Integer modifyBy,
Date modifyDate) {
super();
this.id = id;
this.userCode = userCode;
this.userName = userName;
this.userPassword = userPassword;
this.gender = gender;
this.birthday = birthday;
this.phone = phone;
this.address = address;
this.userRole = userRole;
this.createdBy = createdBy;
this.creationDate = creationDate;
this.modifyBy = modifyBy;
this.modifyDate = modifyDate;
}
public User() {
super();
// TODO 自动生成的构造函数存根
}
@Override
public String toString() {
return "User [id=" + id + ", userCode=" + userCode + ", userName=" + userName + ", userPassword=" + userPassword
+ ", gender=" + gender + ", birthday=" + birthday + ", phone=" + phone + ", address=" + address
+ ", userRole=" + userRole + ", createdBy=" + createdBy + ", creationDate=" + creationDate
+ ", modifyBy=" + modifyBy + ", modifyDate=" + modifyDate + "]";
}
}
UserDao.java:
package com.iocautowired;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
/**
* 用户DAO类,实现UserDao接口,负责User类的持久化操作
* */
//通过注解定义了UserDao
@Component("userdao")
public class UserDao {
@Autowired
private User user;
}
UserService.java接口:
package com.iocautowired;
public interface UserService {
public void addNewUser(User user);
}
UserServiceImpl.java实现类:
package com.iocautowired;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Qualifier;
import org.springframework.stereotype.Service;
@Service("UserServiceImpl")
public class UserServiceImpl implements UserService{
@Autowired
@Qualifier("userdao")
private UserDao dao;
public UserServiceImpl() {}
public UserServiceImpl(UserDao dao) {
super();
this.dao = dao;
}
@Override
public void addNewUser(User user) {
//dao.save(user);
System.out.println("dao.save(user)");
}
}
【3】写 UserServiceImplTest.java 单元测试:
package com.iocautowired;
import org.junit.Test;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class UserServiceImplTest {
@Test
public void test() {
User user = new User();
user.setUserRole(101);
ClassPathXmlApplicationContext classPathXmlApplicationContext = new ClassPathXmlApplicationContext("applicationContext.xml");
UserService bean1 = (UserService)classPathXmlApplicationContext.getBean("UserServiceImpl");
bean1.addNewUser(user);
}
}
【4】写 applicationContext.xml 配置文件:
记住要把依赖文件关系写进去(标注红色部分)
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:aop="http://www.springframework.org/schema/aop"
xmlns:tx="http://www.springframework.org/schema/tx"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-4.2.xsd http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-4.2.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-2.5.xsd">
<!-- 下面是 com\spring\aop进行注解实现IoC配置-->
<context:component-scan base-package="com.iocautowired"></context:component-scan>
</beans>
【5】输出结果:
dao.save(user)
实例分析:
1、@Component("XXX") 的作用等效于 :<bean id="XXX" class="XXX.XX.X"></bean> ;
2、除了@Component("XXX") ,Spring还提供了:@Repository【标注DAO类】、@Service【标注业务类】、@Controller【标注控制器类】;
3、Bean的装配则提供了@Autowired注解实现,采用的是类型匹配,即容器自动查找和属性类型匹配的Bean组件,并自动注入,
4、若是容器发现有一个以上的类型匹配的Bean,则可以使用@Qualifier 指定Bean的名称。
综上,以上是一个Spring的注解IoC小Demo。
Spring框架可以利用XML文件进行IoC配置,但从2.0版本开始引入了注解的配置方式--将Bean的配置信息和Bean实现类结合在一起,可以进一步减少配置文件的代码量。
【1】导入必要的jar包
文件框架:
【2】新建User.java / UserDao.java / UserService.java接口 / 实现类UserServiceImpl.java :
User.java :
package com.iocautowired;
import java.util.Date;
import java.util.List;
import org.springframework.stereotype.Component;
@Component("user")
public class User {
private Integer id;
private String userCode;
private String userName;
private String userPassword;
private Integer gender;
private Date birthday;
private String phone;
private String address ;
private Integer userRole;
private Integer createdBy;
private Date creationDate;
private Integer modifyBy;
private Date modifyDate;
private String userRoleName;
public String getUserRoleName() {
return userRoleName;
}
public void setUserRoleName(String userRoleName) {
this.userRoleName = userRoleName;
}
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public String getUserCode() {
return userCode;
}
public void setUserCode(String userCode) {
this.userCode = userCode;
}
public String getUserName() {
return userName;
}
public void setUserName(String userName) {
this.userName = userName;
}
public String getUserPassword() {
return userPassword;
}
public void setUserPassword(String userPassword) {
this.userPassword = userPassword;
}
public Integer getGender() {
return gender;
}
public void setGender(Integer gender) {
this.gender = gender;
}
public Date getBirthday() {
return birthday;
}
public void setBirthday(Date birthday) {
this.birthday = birthday;
}
public String getPhone() {
return phone;
}
public void setPhone(String phone) {
this.phone = phone;
}
public String getAddress() {
return address;
}
public void setAddress(String address) {
this.address = address;
}
public Integer getUserRole() {
return userRole;
}
public void setUserRole(Integer userRole) {
this.userRole = userRole;
}
public Integer getCreatedBy() {
return createdBy;
}
public void setCreatedBy(Integer createdBy) {
this.createdBy = createdBy;
}
public Date getCreationDate() {
return creationDate;
}
public void setCreationDate(Date creationDate) {
this.creationDate = creationDate;
}
public Integer getModifyBy() {
return modifyBy;
}
public void setModifyBy(Integer modifyBy) {
this.modifyBy = modifyBy;
}
public Date getModifyDate() {
return modifyDate;
}
public void setModifyDate(Date modifyDate) {
this.modifyDate = modifyDate;
}
public User(Integer id, String userCode, String userName, String userPassword, Integer gender, Date birthday,
String phone, String address, Integer userRole, Integer createdBy, Date creationDate, Integer modifyBy,
Date modifyDate) {
super();
this.id = id;
this.userCode = userCode;
this.userName = userName;
this.userPassword = userPassword;
this.gender = gender;
this.birthday = birthday;
this.phone = phone;
this.address = address;
this.userRole = userRole;
this.createdBy = createdBy;
this.creationDate = creationDate;
this.modifyBy = modifyBy;
this.modifyDate = modifyDate;
}
public User() {
super();
// TODO 自动生成的构造函数存根
}
@Override
public String toString() {
return "User [id=" + id + ", userCode=" + userCode + ", userName=" + userName + ", userPassword=" + userPassword
+ ", gender=" + gender + ", birthday=" + birthday + ", phone=" + phone + ", address=" + address
+ ", userRole=" + userRole + ", createdBy=" + createdBy + ", creationDate=" + creationDate
+ ", modifyBy=" + modifyBy + ", modifyDate=" + modifyDate + "]";
}
}
UserDao.java:
package com.iocautowired;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
/**
* 用户DAO类,实现UserDao接口,负责User类的持久化操作
* */
//通过注解定义了UserDao
@Component("userdao")
public class UserDao {
@Autowired
private User user;
}
UserService.java接口:
package com.iocautowired;
public interface UserService {
public void addNewUser(User user);
}
UserServiceImpl.java实现类:
package com.iocautowired;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Qualifier;
import org.springframework.stereotype.Service;
@Service("UserServiceImpl")
public class UserServiceImpl implements UserService{
@Autowired
@Qualifier("userdao")
private UserDao dao;
public UserServiceImpl() {}
public UserServiceImpl(UserDao dao) {
super();
this.dao = dao;
}
@Override
public void addNewUser(User user) {
//dao.save(user);
System.out.println("dao.save(user)");
}
}
【3】写 UserServiceImplTest.java 单元测试:
package com.iocautowired;
import org.junit.Test;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class UserServiceImplTest {
@Test
public void test() {
User user = new User();
user.setUserRole(101);
ClassPathXmlApplicationContext classPathXmlApplicationContext = new ClassPathXmlApplicationContext("applicationContext.xml");
UserService bean1 = (UserService)classPathXmlApplicationContext.getBean("UserServiceImpl");
bean1.addNewUser(user);
}
}
【4】写 applicationContext.xml 配置文件:
记住要把依赖文件关系写进去(标注红色部分)
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:aop="http://www.springframework.org/schema/aop"
xmlns:tx="http://www.springframework.org/schema/tx"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-4.2.xsd http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-4.2.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-2.5.xsd">
<!-- 下面是 com\spring\aop进行注解实现IoC配置-->
<context:component-scan base-package="com.iocautowired"></context:component-scan>
</beans>
【5】输出结果:
dao.save(user)
实例分析:
1、@Component("XXX") 的作用等效于 :<bean id="XXX" class="XXX.XX.X"></bean> ;
2、除了@Component("XXX") ,Spring还提供了:@Repository【标注DAO类】、@Service【标注业务类】、@Controller【标注控制器类】;
3、Bean的装配则提供了@Autowired注解实现,采用的是类型匹配,即容器自动查找和属性类型匹配的Bean组件,并自动注入,
4、若是容器发现有一个以上的类型匹配的Bean,则可以使用@Qualifier 指定Bean的名称。
综上,以上是一个Spring的注解IoC小Demo。
相关文章推荐
- spring 框架中的依赖注入(IOC--设值注入)--使用注解--的具体实例的简单实现
- Spring(四)基于注解配置IOC容器&基于注解实现声明式事务
- Spring实现AOP方式之二:使用注解配置 Spring AOP
- 使用spring注解 自动装配以及自动扫描机制 实现零xml配置的前提
- SpringBoot使用自定义注解实现权限拦截的示例
- Spring中IOC配置xml实现和IOC注解实现
- 使用spring注解 自动装配以及自动扫描机制 实现零xml配置的前提
- 使用注解,实现ssh项目中spring配置文件的零配置,约定优于配置
- Spring 使用注解的方式实现IOC和DI(控制反转和依赖注入)
- Spring学习(九)使用ioc注解方式配置bean
- Spring解决在Dao层注解配置无法注入SessionFactory的办法 实现在Dao层使用注解配置
- 依赖注入(DI)和控制反转(IOC)的详细讲解 spring容器(spring注解实现,而不是xml配置文件)
- Spring使用AspectJ注解和XML配置实现AOP
- 通过注解方式配置Spring实现Ioc
- 注解方式配置Spring实现Ioc
- spring 框架中的依赖注入(IOC--设值注入)---使用xml简单配置文件---的具体实例的简单实现
- Spring(四)基于注解配置IOC容器&基于注解实现声明式事务
- (10) 使用Spring的注解方式实现AOP入门 以及 细节
- Spring学习(九)使用ioc注解方式配置bean
- Spring核心框架IOC---- 使用注解完成IOC配置---- 使用注解配置spring组件