c++中boost协程5种使用实例
2017-12-19 23:00
295 查看
[java]
view plain
copy
#include <iostream>
#include <boost/coroutine/all.hpp>
using namespace boost::coroutines;
//coroutine函数
void cooperative(coroutine<void>::push_type &sink)
{
std::cout << "Hello";
//之所以能够执行是因为重载了操作符()
//返回main()函数继续运行
sink();
std::cout << "world";
//执行完毕,返回main继续执行
}
int main()
{
//c++11新特性:统一初始化
//source对象由于是pull_type类型,所以会马上调用cooperative, push_type类型不会立即执行
coroutine<void>::pull_type source{ cooperative };
std::cout << ", ";
//返回cooperative函数继续执行
source();
std::cout << "!";
std::cout << "\n";
}
输出结果
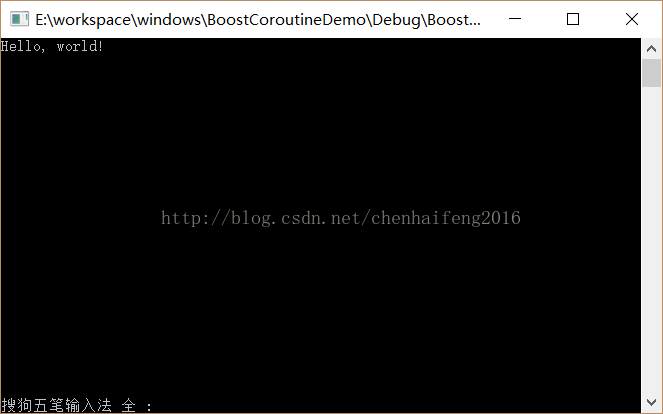
[java]
view plain
copy
#include <functional>
#include <iostream>
#include <boost/coroutine/all.hpp>
using boost::coroutines::coroutine;
void cooperative(coroutine<int>::push_type &sink, int i)
{
int j = i;
//调用main
sink(++j);
//调用main
sink(++j);
std::cout << "end\n";
}
int main()
{
using std::placeholders::_1;
//传入一个参数,初始值为0
coroutine<int>::pull_type source{ std::bind(cooperative, _1, 0) };
std::cout << source.get() << '\n';
//调用cooperative
source();
std::cout << source.get() << '\n';
//调用cooperative
source();
}
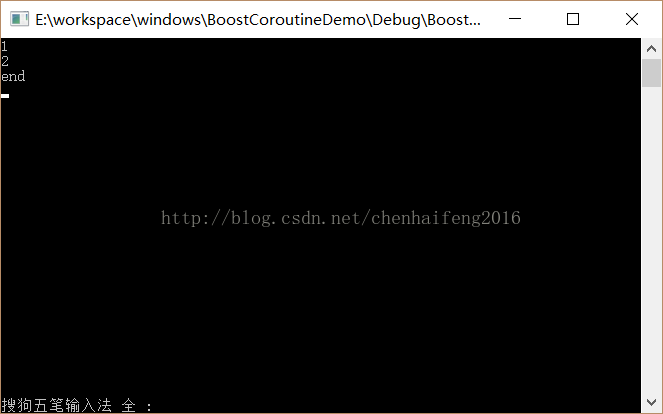
[java]
view plain
copy
#include <tuple>
#include <string>
#include <iostream>
#include <boost/coroutine/all.hpp>
using boost::coroutines::coroutine;
void cooperative(coroutine<std::tuple<int, std::string>>::pull_type &source)
{
auto args = source.get();
std::cout << std::get<0>(args) << " " << std::get<1>(args) << '\n';
source();
args = source.get();
std::cout << std::get<0>(args) << " " << std::get<1>(args) << '\n';
}
int main()
{
coroutine<std::tuple<int, std::string>>::push_type sink{ cooperative };
//通过tuple传递多个参数
sink(std::make_tuple(0, "aaa"));
//通过tuple传递多个参数
sink(std::make_tuple(1, "bbb"));
std::cout << "end\n";
}
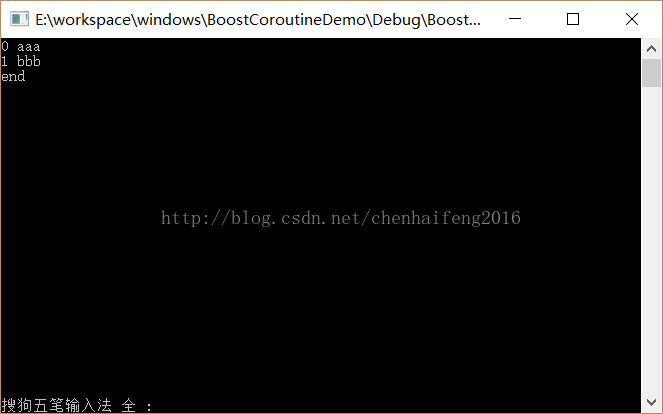
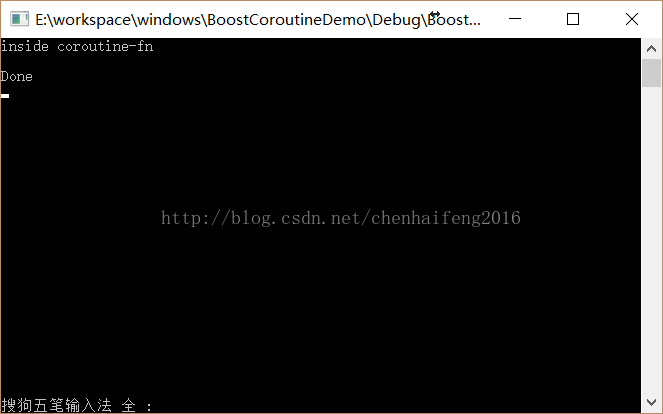
view plain
copy
#include <iostream>
#include <boost/coroutine/all.hpp>
using namespace boost::coroutines;
//coroutine函数
void cooperative(coroutine<void>::push_type &sink)
{
std::cout << "Hello";
//之所以能够执行是因为重载了操作符()
//返回main()函数继续运行
sink();
std::cout << "world";
//执行完毕,返回main继续执行
}
int main()
{
//c++11新特性:统一初始化
//source对象由于是pull_type类型,所以会马上调用cooperative, push_type类型不会立即执行
coroutine<void>::pull_type source{ cooperative };
std::cout << ", ";
//返回cooperative函数继续执行
source();
std::cout << "!";
std::cout << "\n";
}
输出结果
[java]
view plain
copy
#include <functional>
#include <iostream>
#include <boost/coroutine/all.hpp>
using boost::coroutines::coroutine;
void cooperative(coroutine<int>::push_type &sink, int i)
{
int j = i;
//调用main
sink(++j);
//调用main
sink(++j);
std::cout << "end\n";
}
int main()
{
using std::placeholders::_1;
//传入一个参数,初始值为0
coroutine<int>::pull_type source{ std::bind(cooperative, _1, 0) };
std::cout << source.get() << '\n';
//调用cooperative
source();
std::cout << source.get() << '\n';
//调用cooperative
source();
}
[java]
view plain
copy
#include <tuple>
#include <string>
#include <iostream>
#include <boost/coroutine/all.hpp>
using boost::coroutines::coroutine;
void cooperative(coroutine<std::tuple<int, std::string>>::pull_type &source)
{
auto args = source.get();
std::cout << std::get<0>(args) << " " << std::get<1>(args) << '\n';
source();
args = source.get();
std::cout << std::get<0>(args) << " " << std::get<1>(args) << '\n';
}
int main()
{
coroutine<std::tuple<int, std::string>>::push_type sink{ cooperative };
//通过tuple传递多个参数
sink(std::make_tuple(0, "aaa"));
//通过tuple传递多个参数
sink(std::make_tuple(1, "bbb"));
std::cout << "end\n";
}
#include <iostream> #include <cstdlib> #include <boost/coroutine2/all.hpp> int main() { int i = 0; boost::coroutines2::coroutine< void >::push_type sink( [&](boost::coroutines2::coroutine< void >::pull_type & source) { std::cout << "inside coroutine-fn" << std::endl; }); sink(); std::cout << "\nDone" << std::endl; return EXIT_SUCCESS; }
#include <stdexcept> #include <iostream> #include <boost/coroutine/all.hpp> using boost::coroutines::coroutine; void cooperative(coroutine<void>::push_type &sink) { //返回main sink(); throw std::runtime_error("error"); } int main() { coroutine<void>::pull_type source{ cooperative }; try { //调用cooperative source(); //捕获抛出的异常std::runtime_error } catch (const std::runtime_error &e) { std::cerr << e.what() << '\n'; } }
相关文章推荐
- C++ 调用Boost 使用正则表达式实例
- LinuxC/C++编程基础(20) 使用boost::asio搭建服务器简单实例
- LinuxC/C++编程基础(21) 使用boost::asio搭建服务器简单实例(续)
- c++中的引用的使用原理和使用实例 (3)
- boost::function,让C++的函数也能当第一类值使用
- c++ boost正则使用
- 使用 Boost.Python 嵌入 Python 模块到 C++
- (引用)使用 C# 和 C++.NET 开发的 .NET 应用程序实例列表
- C++中break语句和continue语句讲解及使用实例
- c++ python交互之boost.python 简集之Vector(map)混合使用
- Ubuntu编译安装boost并在eclipse C/C++中使用
- boost.array 使用实例
- C++ 中vector的使用实例
- C++ map使用实例
- c++ boost正则使用
- c++中的引用的使用原理以及使用实例 (1)
- 如何在Open C/C++应用中使用Boost.Regex
- c++中的引用的使用原理以及使用实例 (2)
- Silverlight实用窍门系列:22.Silverlight使用WebService调用C++,Delphi编写的DLL文件【实例源码下载】
- C++中使用boost::serialization库――应用篇