第十五周Java作业
2017-12-14 14:07
344 查看
编写多线程程序,模拟多个人通过一个山洞。这个山洞每次只能通过一个人,每个人通过山洞的时间为2秒(sleep)。随机生成10个人,都要通过此山洞,用随机值对应的字符串表示人名,打印输出每次通过山洞的人名。提示:利用线程同步机制,过山洞用一条输出语句表示,该输出语句打印输出当前过山洞的人名,每个人过山洞对应一个线程,哪个线程执行这条输出语句,就表示哪个人过山洞
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
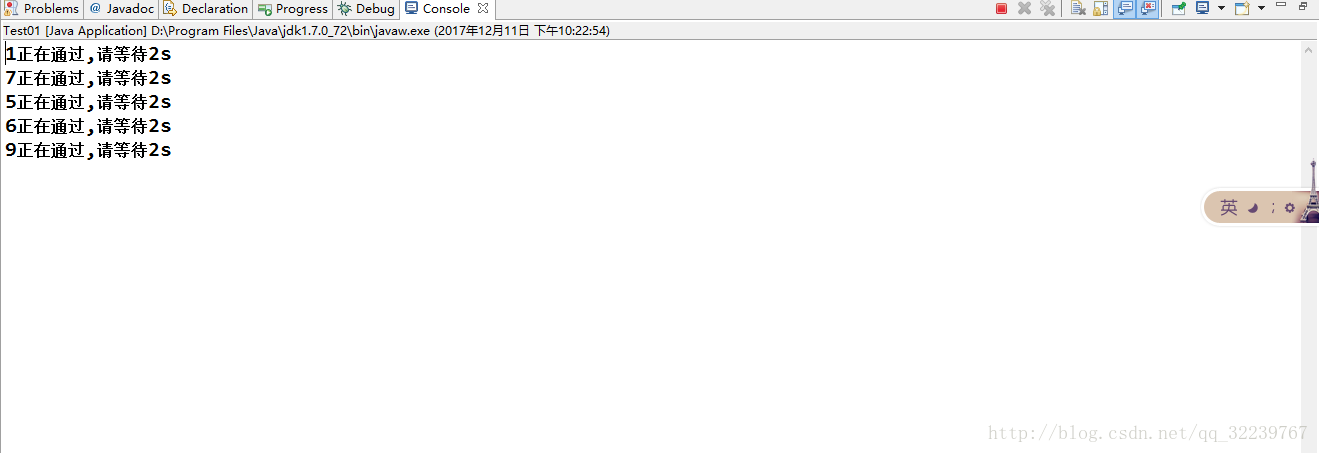
用两个线程玩猜数字游戏,第一个线程负责随机给出1~100之间的一个整数,第二个线程负责猜出这个数。要求每当第二个线程给出自己的猜测后,第一个线程都会提示“猜小了”、“猜大了”或“猜对了”。猜数之前,要求第二个线程要等待第一个线程设置好要猜测的数。第一个线程设置好猜测数之后,两个线程还要相互等待,其原则是:第二个线程给出自己的猜测后,等待第一个线程给出的提示;第一个线程给出提示后,等待给第二个线程给出猜测,如此进行,直到第二个线程给出正确的猜测后,两个线程进入死亡状态。
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
编写多线程程序,模拟多个人通过一个山洞。这个山洞每次只能通过一个人,每个人通过山洞的时间为2秒(sleep)。随机生成10个人,都要通过此山洞,用随机值对应的字符串表示人名,打印输出每次通过山洞的人名。提示:利用线程同步机制,过山洞用一条输出语句表示,该输出语句打印输出当前过山洞的人名,每个人过山洞对应一个线程,哪个线程执行这条输出语句,就表示哪个人过山洞
package week15; public class Test01 { public static void main(String[] args) { //实例化山洞 Shandong shandong = new Shandong(); //创建10个线程 Thread thread1 = new Thread(shandong, "1"); Thread thread2 = new Thread(shandong, "2"); Thread thread3 = new Thread(shandong, "3"); Thread thread4 = new Thread(shandong, "4"); Thread thread5 = new Thread(shandong, "5"); Thread thread6 = new Thread(shandong, "6"); Thread thread7 = new Thread(shandong, "7"); Thread thread8 = new Thread(shandong, "8"); Thread thread9 = new Thread(shandong, "9"); Thread thread10 = new Thread(shandong, "10"); thread1.start(); thread2.start(); thread3.start(); thread4.start(); thread5.start(); thread6.start(); thread7.start(); thread8.start(); thread9.start(); thread10.start(); } } //山洞 class Shandong extends Thread{ @Override public void run() { //上锁 synchronized(this){ System.out.println(Thread.currentThread().getName()+"正在通过,请等待5s"); try { sleep(5000); } catch (InterruptedException e) { // TODO Auto-generated catch block e.printStackTrace(); } } } }1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
用两个线程玩猜数字游戏,第一个线程负责随机给出1~100之间的一个整数,第二个线程负责猜出这个数。要求每当第二个线程给出自己的猜测后,第一个线程都会提示“猜小了”、“猜大了”或“猜对了”。猜数之前,要求第二个线程要等待第一个线程设置好要猜测的数。第一个线程设置好猜测数之后,两个线程还要相互等待,其原则是:第二个线程给出自己的猜测后,等待第一个线程给出的提示;第一个线程给出提示后,等待给第二个线程给出猜测,如此进行,直到第二个线程给出正确的猜测后,两个线程进入死亡状态。
package week15; import java.util.Random; public class Test02 { static Object setnumber=new Object(); static Object guess=new Object(); public static void main(String[] args) { Question question=new Question(setnumber,guess); Answer answer=new Answer(setnumber,guess); new Thread(question).start(); new Thread(answer).start(); } } class Question implements Runnable{ Object setnumber; Object guess; int number; public Question(Object setnumber, Object guess) { // TODO Auto-generated constructor stub this.setnumber=setnumber; this.guess=guess; } @Override public void run() { // TODO Auto-generated method stub synchronized (setnumber) { Random rand = new Random(); number=rand.nextInt(100); State.initialize=1; System.out.println("我生成了数字:"+number); } // synchronized() while(true){ System.out.println("question:"+State.answed); if(State.answed!=0){ try { Thread.sleep(100); } catch (InterruptedException e) { // TODO Auto-generated catch block e.printStackTrace(); } }else { synchronized (guess) { System.out.println("你猜的是:"+State.answednumber); if(State.answednumber<number){ State.answed=-1; System.out.println("猜小了"+State.answed); }if(State.answednumber>number){ State.answed=1; System.out.println("猜大了"+State.answed); }if(State.answednumber==number){ State.answed=2; System.out.println("猜对了,就是"+State.answed); break; } Thread.yield(); } } } } } class Answer implements Runnable{ Object setnumber; Object guess; int min=0; int max=100; Random rand = new Random(); public Answer(Object setnumber, Object guess) { // TODO Auto-generated constructor stub this.setnumber=setnumber; this.guess=guess; } @Override public void run() { // TODO Auto-generated method stub while(State.initialize==0){//如果没有运行question方法就等待。 try { Thread.sleep(100); } catch (InterruptedException e) { // TODO Auto-generated catch block e.printStackTrace(); } } synchronized (setnumber) { while(true){ if(State.answed==0){ try { Thread.sleep(100); } catch (InterruptedException e) { // TODO Auto-generated catch block e.printStackTrace(); } } else { synchronized (guess) { if(State.answed==1){ max=State.answednumber; State.answednumber=rand.nextInt(max-min)+min; System.out.println("我猜:"+State.answednumber); } if(State.answed==-1){ min=State.answednumber; State.answednumber=rand.nextInt(max-min)+min; System.out.println("我猜:"+State.answednumber); } if(State.answed==2) { System.out.println("我特么终于猜对了~"); break; } State.answed=0; Thread.yield(); } } } } } } class State{//内部static变量充当全局变量的作用来为线程间进行通讯 static int initialize=0;//是否被初始化。 static int answed=0;//用来表示是菜大了还是猜小了 static int answednumber=100;//猜的数字 }1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
相关文章推荐
- 12.6第十五周JAVA作业
- 第十五周Java作业-1线程同步机制
- 第十五周java作业 -猜数字
- 第十五周Java作业--线程
- 《Java程序设计》第二次作业:MySQL数据库及Java操作MySQL数据库 之二
- java课后作业
- java新手 if else作业题
- IT十八掌作业_java基础第21天_mysql
- 【求助】一个菜鸟java作业,帮忙看一下错在哪儿,题目是判断回文数
- java作业1
- Java作业:大数累加(我是真不知道有什么用)
- java第六次作业 抽奖机
- IT十八掌作业_java基础第五天_静态代码块、类的继承和接口
- IT十八掌作业_java基础第六天_接口与适配器模式、多态、内部类
- JAVA--第十周作业编写之一个Teacher类负责给出算术题目,随机给出两个整数并进行运算,并判断回答者的答案是否正确;编写一个GUI类ComputerFrame,回答者可以通过GUI看到题目并给出
- Java第三次作业
- 吉软_java57_第二次作业
- java第三次作业
- Java 作业 金额的中文大写方式
- 大三学长带我学习JAVA.作业4.流程控制语句.续.Flow.Control.Statement.Cont 理解面向对象程序设计 学长带我学java的作业4