java图片压缩--Thumbnailator
2017-11-20 16:15
211 查看
最近在java开发中遇到了图片处理的问题,我使用的是Thumbnailator。Thumbnailator是一个优秀的图片处理的开源java类库,使用起来很方便。
图片原图:
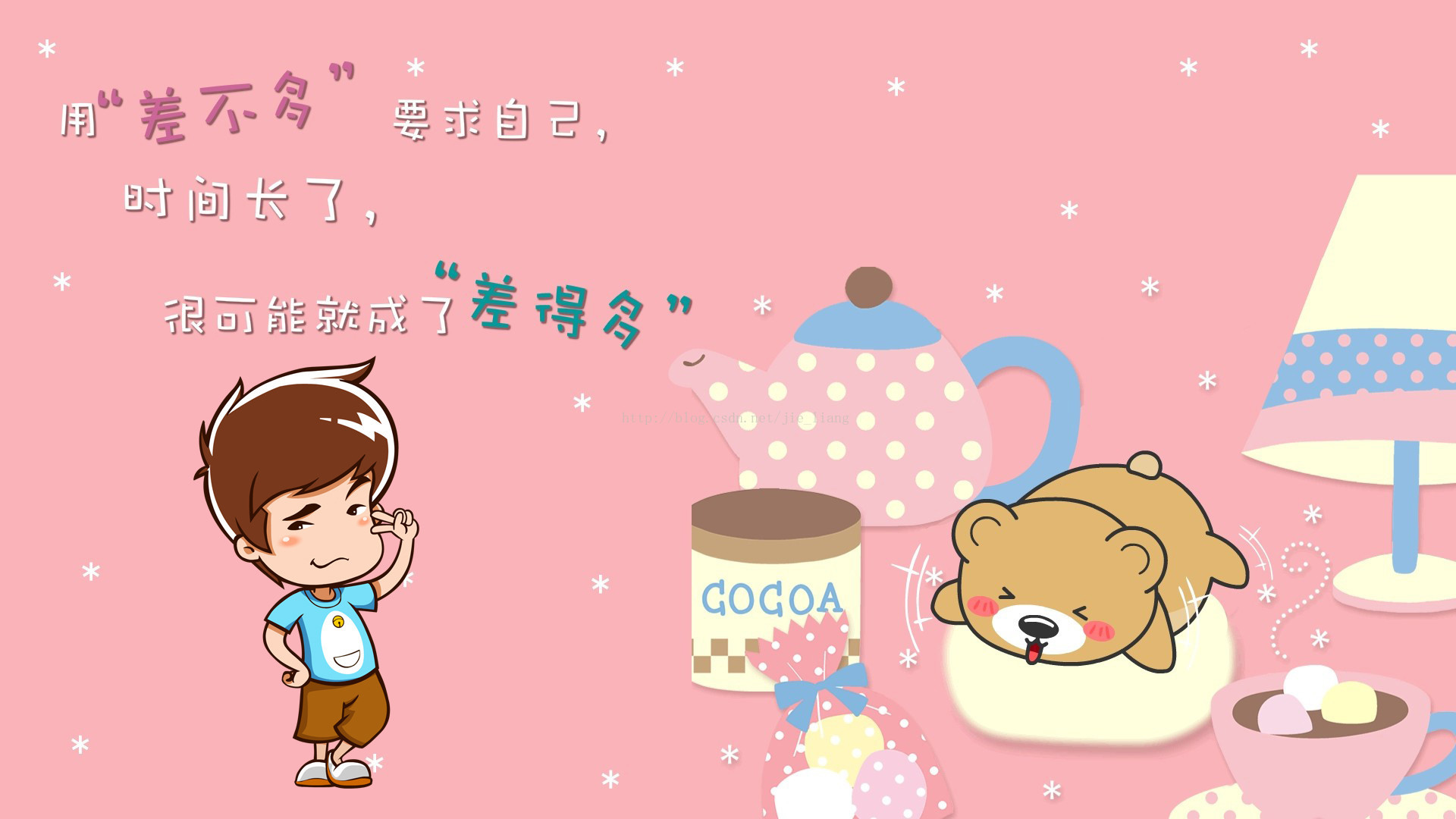
1、指定大小进行缩放
public class PicUtil {
public static void main(String[] args) {
PicUtil.commpressPicForSize("G:\\images\\ceshi.jpg",
"G:\\images\\datas\\ceshi.jpg", 100, 0.3); // 图片小于100kb
}
/**
* 根据指定大小和指定精度压缩图片
*
* @param srcPath
* 源图片地址
* @param desPath
* 目标图片地址
* @param desFilesize
* 指定图片大小,单位kb
* @param accuracy
* 精度,递归压缩的比率,建议小于0.9
* @return
*/
public static String commpressPicForSize(String srcPath, String desPath,
long desFileSize, double accuracy) {
if (StringUtils.isEmpty(srcPath) || StringUtils.isEmpty(srcPath)) {
return null;
}
if (!new File(srcPath).exists()) {
return null;
}
try {
File srcFile = new File(srcPath);
long srcFileSize = srcFile.length();
System.out.println("源图片:" + srcPath + ",大小:" + srcFileSize / 1024
+ "kb");
// 1、先转换成jpg
Thumbnails.of(srcPath).scale(1f).toFile(desPath);
// 递归压缩,直到目标文件大小小于desFileSize
commpressPicCycle(desPath, desFileSize, accuracy);
File desFile = new File(desPath);
System.out.println("目标图片:" + desPath + ",大小" + desFile.length()
/ 1024 + "kb");
System.out.println("图片压缩完成!");
} catch (Exception e) {
e.printStackTrace();
return null;
}
return desPath;
}
/**
* 图片压缩:按指定大小把图片进行缩放(会遵循原图高宽比例)
* 并设置图片文件大小
*/
private static void commpressPicCycle(String desPath, long desFileSize,
double accuracy) throws IOException {
File srcFileJPG = new File(desPath);
long srcFileSizeJPG = srcFileJPG.length();
// 2、判断大小,如果小于指定大小,不压缩;如果大于等于指定大小,压缩
if (srcFileSizeJPG <= desFileSize * 1024) {
return;
}
// 计算宽高
BufferedImage bim = ImageIO.read(srcFileJPG);
int srcWdith = bim.getWidth();
int srcHeigth = bim.getHeight();
int desWidth = new BigDecimal(srcWdith).multiply(
new BigDecimal(accuracy)).intValue();
int desHeight = new BigDecimal(srcHeigth).multiply(
new BigDecimal(accuracy)).intValue();
Thumbnails.of(desPath).size(desWidth, desHeight)
.outputQuality(accuracy).toFile(desPath);
commpressPicCycle(desPath, desFileSize, accuracy);
}
}压缩后图片展示:
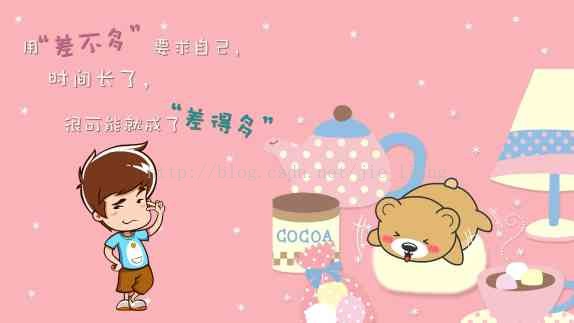
运行结果打印:

2、按照比例进行缩放
public class PicUtil {
public static void main(String[] args) {
PicUtil.commpressPicForScale("G:\\images\\ceshi.jpg",
"G:\\images\\scales\\ceshi.jpg", 100,0.3);
}
public static String commpressPicForScale(String srcPath, String desPath,
long desFileSize, double accuracy) {
if (StringUtils.isEmpty(srcPath) || StringUtils.isEmpty(srcPath)) {
return null;
}
if (!new File(srcPath).exists()) {
return null;
}
try {
File srcFile = new File(srcPath);
long srcFileSize = srcFile.length();
System.out.println("源图片:" + srcPath + ",大小:" + srcFileSize / 1024
+ "kb");
// 1、先转换成jpg
Thumbnails.of(srcPath).scale(1f).toFile(desPath);
//按照比例进行缩放
imgScale(desPath, desFileSize, accuracy);
File desFile = new File(desPath);
System.out.println("目标图片:" + desPath + ",大小" + desFile.length()
/ 1024 + "kb");
System.out.println("图片压缩完成!");
} catch (Exception e) {
e.printStackTrace();
return null;
}
return desPath;
}
/**
* 按照比例进行缩放
*
*/
private static void imgScale(String desPath, long desFileSize,
double accuracy) throws IOException{
File file=new File(desPath);
long fileSize=file.length();
//判断大小,如果小于指定大小,不压缩;如果大于等于指定大小,压缩
if(fileSize<=desFileSize*1024){
return;
}
//按照比例进行缩小
Thumbnails.of(desPath).scale(accuracy).toFile(desPath);//按比例缩小
System.out.println("按照比例进行缩放");
imgScale(desPath, desFileSize, accuracy);
}
}
图片压缩效果:
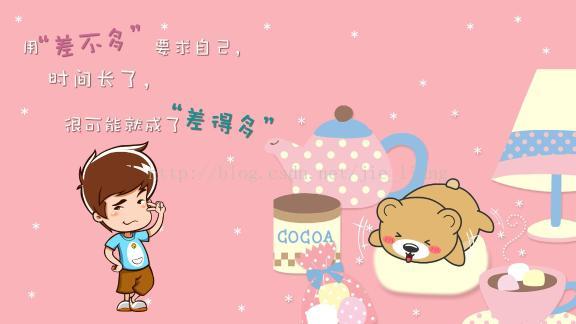
运行结果打印:
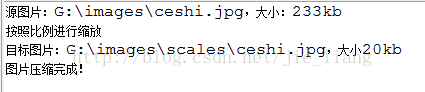
3、图片尺寸不变,大小改变
public class PicUtil {
public static void main(String[] args) {
PicUtil.commpressPicForScaleSize("G:\\images\\ceshi.jpg",
"G:\\images\\scaleSize\\ceshi.jpg", 100,0.25);
}
public static String commpressPicForScaleSize(String srcPath, String desPath,
long desFileSize, double accuracy) {
if (StringUtils.isEmpty(srcPath) || StringUtils.isEmpty(srcPath)) {
return null;
}
if (!new File(srcPath).exists()) {
return null;
}
try {
File srcFile = new File(srcPath);
long srcFileSize = srcFile.length();
System.out.println("源图片:" + srcPath + ",大小:" + srcFileSize / 1024
+ "kb");
// 1、先转换成jpg
Thumbnails.of(srcPath).scale(1f).toFile(desPath);
//按照比例进行缩放
imgScaleSize(desPath, desFileSize, accuracy);
File desFile = new File(desPath);
System.out.println("目标图片:" + desPath + ",大小" + desFile.length()
/ 1024 + "kb");
System.out.println("图片压缩完成!");
} catch (Exception e) {
e.printStackTrace();
return null;
}
return desPath;
}
/**
* 图片尺寸不变,压缩文件大小
*/
private static void imgScaleSize(String desPath, long desFileSize,
double accuracy) throws IOException{
File fileName=new File(desPath);
long fileNameSize=fileName.length();
//判断大小,如果小于指定大小,不压缩;如果大于等于指定大小,压缩
if(fileNameSize<=desFileSize*1024){
return;
}
//图片尺寸不变,压缩图片文件大小
//图片尺寸不变,压缩图片文件大小outputQuality实现,参数1为最高质量
Thumbnails.of(desPath).scale(1f).outputQuality(accuracy).toFile(desPath);
System.out.println("图片尺寸不变,压缩文件大小");
imgScaleSize(desPath, desFileSize, accuracy);
}
}图片压缩效果展示:
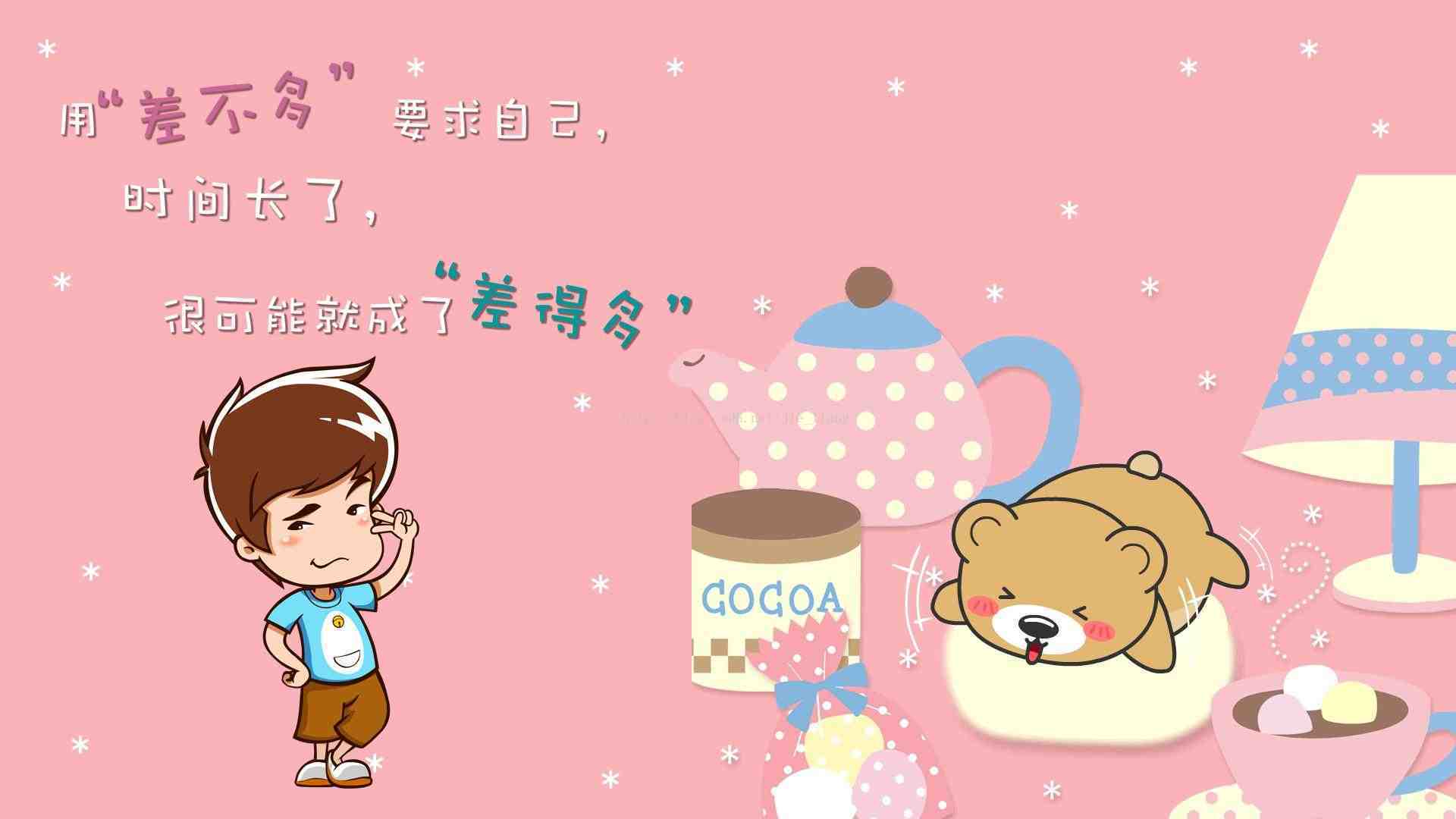
运行结果打印:

参考文章链接:http://blog.csdn.net/u010355502/article/details/77197616
图片原图:
1、指定大小进行缩放
public class PicUtil {
public static void main(String[] args) {
PicUtil.commpressPicForSize("G:\\images\\ceshi.jpg",
"G:\\images\\datas\\ceshi.jpg", 100, 0.3); // 图片小于100kb
}
/**
* 根据指定大小和指定精度压缩图片
*
* @param srcPath
* 源图片地址
* @param desPath
* 目标图片地址
* @param desFilesize
* 指定图片大小,单位kb
* @param accuracy
* 精度,递归压缩的比率,建议小于0.9
* @return
*/
public static String commpressPicForSize(String srcPath, String desPath,
long desFileSize, double accuracy) {
if (StringUtils.isEmpty(srcPath) || StringUtils.isEmpty(srcPath)) {
return null;
}
if (!new File(srcPath).exists()) {
return null;
}
try {
File srcFile = new File(srcPath);
long srcFileSize = srcFile.length();
System.out.println("源图片:" + srcPath + ",大小:" + srcFileSize / 1024
+ "kb");
// 1、先转换成jpg
Thumbnails.of(srcPath).scale(1f).toFile(desPath);
// 递归压缩,直到目标文件大小小于desFileSize
commpressPicCycle(desPath, desFileSize, accuracy);
File desFile = new File(desPath);
System.out.println("目标图片:" + desPath + ",大小" + desFile.length()
/ 1024 + "kb");
System.out.println("图片压缩完成!");
} catch (Exception e) {
e.printStackTrace();
return null;
}
return desPath;
}
/**
* 图片压缩:按指定大小把图片进行缩放(会遵循原图高宽比例)
* 并设置图片文件大小
*/
private static void commpressPicCycle(String desPath, long desFileSize,
double accuracy) throws IOException {
File srcFileJPG = new File(desPath);
long srcFileSizeJPG = srcFileJPG.length();
// 2、判断大小,如果小于指定大小,不压缩;如果大于等于指定大小,压缩
if (srcFileSizeJPG <= desFileSize * 1024) {
return;
}
// 计算宽高
BufferedImage bim = ImageIO.read(srcFileJPG);
int srcWdith = bim.getWidth();
int srcHeigth = bim.getHeight();
int desWidth = new BigDecimal(srcWdith).multiply(
new BigDecimal(accuracy)).intValue();
int desHeight = new BigDecimal(srcHeigth).multiply(
new BigDecimal(accuracy)).intValue();
Thumbnails.of(desPath).size(desWidth, desHeight)
.outputQuality(accuracy).toFile(desPath);
commpressPicCycle(desPath, desFileSize, accuracy);
}
}压缩后图片展示:
运行结果打印:
2、按照比例进行缩放
public class PicUtil {
public static void main(String[] args) {
PicUtil.commpressPicForScale("G:\\images\\ceshi.jpg",
"G:\\images\\scales\\ceshi.jpg", 100,0.3);
}
public static String commpressPicForScale(String srcPath, String desPath,
long desFileSize, double accuracy) {
if (StringUtils.isEmpty(srcPath) || StringUtils.isEmpty(srcPath)) {
return null;
}
if (!new File(srcPath).exists()) {
return null;
}
try {
File srcFile = new File(srcPath);
long srcFileSize = srcFile.length();
System.out.println("源图片:" + srcPath + ",大小:" + srcFileSize / 1024
+ "kb");
// 1、先转换成jpg
Thumbnails.of(srcPath).scale(1f).toFile(desPath);
//按照比例进行缩放
imgScale(desPath, desFileSize, accuracy);
File desFile = new File(desPath);
System.out.println("目标图片:" + desPath + ",大小" + desFile.length()
/ 1024 + "kb");
System.out.println("图片压缩完成!");
} catch (Exception e) {
e.printStackTrace();
return null;
}
return desPath;
}
/**
* 按照比例进行缩放
*
*/
private static void imgScale(String desPath, long desFileSize,
double accuracy) throws IOException{
File file=new File(desPath);
long fileSize=file.length();
//判断大小,如果小于指定大小,不压缩;如果大于等于指定大小,压缩
if(fileSize<=desFileSize*1024){
return;
}
//按照比例进行缩小
Thumbnails.of(desPath).scale(accuracy).toFile(desPath);//按比例缩小
System.out.println("按照比例进行缩放");
imgScale(desPath, desFileSize, accuracy);
}
}
图片压缩效果:
运行结果打印:
3、图片尺寸不变,大小改变
public class PicUtil {
public static void main(String[] args) {
PicUtil.commpressPicForScaleSize("G:\\images\\ceshi.jpg",
"G:\\images\\scaleSize\\ceshi.jpg", 100,0.25);
}
public static String commpressPicForScaleSize(String srcPath, String desPath,
long desFileSize, double accuracy) {
if (StringUtils.isEmpty(srcPath) || StringUtils.isEmpty(srcPath)) {
return null;
}
if (!new File(srcPath).exists()) {
return null;
}
try {
File srcFile = new File(srcPath);
long srcFileSize = srcFile.length();
System.out.println("源图片:" + srcPath + ",大小:" + srcFileSize / 1024
+ "kb");
// 1、先转换成jpg
Thumbnails.of(srcPath).scale(1f).toFile(desPath);
//按照比例进行缩放
imgScaleSize(desPath, desFileSize, accuracy);
File desFile = new File(desPath);
System.out.println("目标图片:" + desPath + ",大小" + desFile.length()
/ 1024 + "kb");
System.out.println("图片压缩完成!");
} catch (Exception e) {
e.printStackTrace();
return null;
}
return desPath;
}
/**
* 图片尺寸不变,压缩文件大小
*/
private static void imgScaleSize(String desPath, long desFileSize,
double accuracy) throws IOException{
File fileName=new File(desPath);
long fileNameSize=fileName.length();
//判断大小,如果小于指定大小,不压缩;如果大于等于指定大小,压缩
if(fileNameSize<=desFileSize*1024){
return;
}
//图片尺寸不变,压缩图片文件大小
//图片尺寸不变,压缩图片文件大小outputQuality实现,参数1为最高质量
Thumbnails.of(desPath).scale(1f).outputQuality(accuracy).toFile(desPath);
System.out.println("图片尺寸不变,压缩文件大小");
imgScaleSize(desPath, desFileSize, accuracy);
}
}图片压缩效果展示:
运行结果打印:
参考文章链接:http://blog.csdn.net/u010355502/article/details/77197616
相关文章推荐
- java中用Thumbnailator对图片各种处理的方法(可做到对原图片压缩仅改变大小)
- Thumbnailator java图片压缩,加水印,批量生成缩略图
- Java图片缩略图裁剪水印缩放旋转压缩转格式-Thumbnailator图像处理
- Java图片缩略图裁剪水印缩放旋转压缩转格式-Thumbnailator图像处理
- Thumbnailator java图片压缩,加水印,批量生成缩略图
- Java图片缩略图裁剪水印缩放旋转压缩转格式-Thumbnailator图像处理
- Java图片缩略图裁剪水印缩放旋转压缩转格式-Thumbnailator图像处理
- Java图片缩略图裁剪水印缩放旋转压缩转格式-Thumbnailator图像处理
- Java使用google的thumbnailator工具对图片压缩水印等做处理
- JAVA使用thumbnailator对base64图片进行压缩
- Thumbnailator java图片压缩,加水印,批量生成缩略图
- Java图片缩略图裁剪水印缩放旋转压缩转格式-Thumbnailator图像处理
- java 使用Thumbnailator 上传图片 并压缩图片大小
- Thumbnailator java图片压缩,加水印,批量生成缩略图
- java使用Thumbnailator操作图片
- java简单的图片压缩
- Java中图片按质量压缩
- java对图片进行“高保真”压缩
- 纯Java代码 图片压缩
- JAVA开发之压缩图片并打成ZIP文件