【JavaEE学习笔记】JSP,EL,JSTL
2017-10-30 10:37
791 查看
A.JSP
1.概述
JSP:Java Server Page Java服务器页面语言
本质:是一个Servlet,即可以写HTML语言,也可以写Java语言
语法:早期技术,JSP脚本
<%! int num = 1000;%>生成的Java代码在成员位置
<%System.out.println("hello world");%>生成的代码在Service()方法中
<%="hello world"%>生成的东西在页面上,即:out.print("hello world");
JSP专用注释:<%-- --%>:不会发送到客户端,可以注释代码
原理:
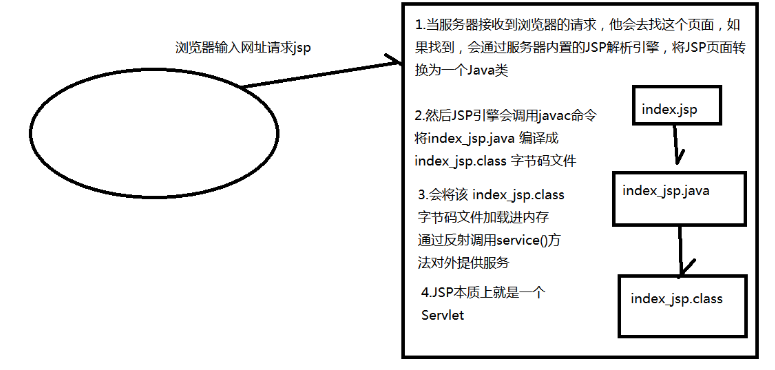
2.3大指令
a.概述
指令:配置JSP页面的属性或者导入一些额外的内容
格式:<%@ 指令名称 属性名="属性值"%>
分类:
page:配置JSP页面的属性
include:静态包含(不常用,基本用动态包含)
taglib:导入额外的内容
d.page指令:
language:指定jsp页面使用的语言,只有一个值
import:导入软件包
pageEncoding:设置页面编码
指定jsp文件的编码
高速浏览器页面使用的编码格式
autoFlush:自动刷新
errorPage:指定错误页面,当页面发生异常时,指定跳转的页面
一般在开发完成后,会在web.xml中通过<error-page>标签来配置整个项目的错误页面
当服务器出现问题时,用户不会看到404或者500页面,不让用户知道服务器出现问题
isErrorPage:该页面是否是错误页面
true:是,可以使用内置对象exception,上面提到的404.jsp就要设置为错误页面
false:不是
e.taglib指令
导入额外的内容,如jstl标签
f.include指令
静态包含,包含后,只会存在一个.class文件
包含进去的内容只会显示在页面最上方,所以不常用
3.6个常用动作标签
a.替换Java代码,简化书写
1)创建一个Student类
成员变量:username,age
有参无参构造
set/get方法
2)使用Jsp脚本创建对象,并设置获取参数
b.<jsp:useBean id="stu" class="org.xxxx.demo.Student"> 创建对象
id:对象名
class:类的全路径名称
Student stu = new Student()
c.<jsp:setProperty property="username" name="stu" value="张三">设置属性值
property:字段名--setUsername()中,set后面的就叫字段
name:对象名
value:属性值
stu.setUsername("张三");
d.<jsp:getProperty property="username" name="stu">获取属性值
property:字段名
name:对象名
stu.getUsername
e.<jsp:include>动态包含文件
f.<jsp:forward>请求转发
一般服务器WebRoot目录下只放一个首页,用户可以通过路径访问
其他内部页面放在Web-INF目录下,该目录下文件无法通过链接直接访问
必须通过内部跳转才能访问
防止用户通过输入链接而直接越过首页
1)WebRoot目录下的index.jsp(全删除,就写这三行)
2)提交到后台Servlet,做一些逻辑判断,实现跳转到内部链接
3)内部链接MyJSP.jsp
4)运行服务器,观察浏览器地址栏不会发生变化,用户并不知道我们的网页发生跳转
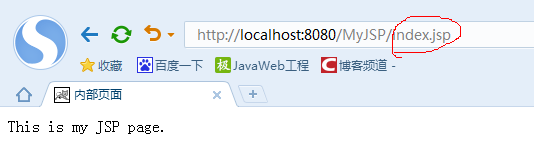
5)手动输入MyJSP.jsp地址,看能否进入该页面
g.<jsp:param>传递请求参数
4.9个常用内置对象
a.当jsp转换成service()方法时,会在该方法里声明好9个内置对象
b.pageContext
页面域,可以通过它获取其他八个对象
c.application:ServletContext 全局域
d.session:HttpSession 会话域
e.request:HttpServletRequest 请求域
f.out:JspWriter 输出内容在body内
常用
g.response:HttpServletResponse 输出内容在html标签外
不常用
h.config:ServletConfig 配置对象
i.page:this 当前类
j.expection:Throwable:错误信息,(必须设定为错误页面方可调用)
B.EL
1.概述
JSP的内置表达式语言,用来代替JSP脚本
作用:
a.获取域中数据
b.执行运算
c.获取常见的web对象
d.调用java的方法
格式:${el表达式}
2.获取域中的数据
a.简单的数据
${属性名}:依次从pageContext,request,session,applocation查找
如果查找不到,返回""
1)例子
运行,验证输出
2)当四个域的key都一样,会获取最小域(pageContext)的值
如果要都获取怎么办?Scope(pageContext不需要)
b.获取复杂数据
1)获取数组中的数据
${域中的名称[index]}
2)获取list中的数据
${域中的名称[index]}
3)获取map中的数据
${域中的名称.键名}
c.获取对象中的字段值
d.执行运算
算术运算,逻辑运算,三元运算
empty:判断一个容器的长度是否为0(数组,集合)还可以判断一个对象是否为空
${empty 域中的对象名称}
判断非空:${not emoty 域中的对象名称}
注意:+只能进行加法运算,字符串形式数字可以进行加法运算
代码演示:
3.11个内置对象
pageScope
requestScope
sessionScope
applicationScope
parm
parmValues
header
headerValues
initParam
cookie
pageContext
4.cookie内置对象
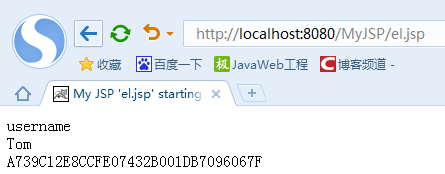
5.pageContext
在jsp页面中获取项目名
在使用跳转可以使用动态路径
做一个跳转页面
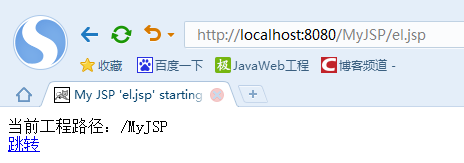
点击跳转,观察控制台
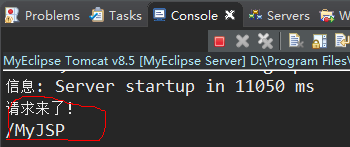
C.JSTL
1.概述
JSP标准的标签库语言,apache开发,用来替代jsp脚本,结合EL使用
2.使用步骤
a.导入jar包(jstl.jar和standard.jar)
b.在页面上导入标签库
<%@taglib prefix="" uri=""%>
MyEclipse中已经集成了jar包,我们只需要导入就行
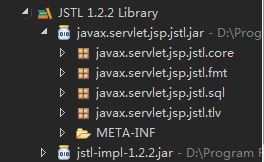
3.jstl的分类
core:核心类库
c:if
c:foreach
fmt:格式化|国际化
xml:过时
sql:过时
函数库:很少用
4.c:if判断
<c:if test="${el表达式}">满足的时候输出内容</c:if>;没有else
5.c:forEach循环
a.格式1:
<c:forEach begin="起始" end="结束" step="步长" var="变量名" varStatus="循环状态变量">
varStatus:用来记录循环的状态
count:记录次数
current:当前便利的内容
index:索引从0开始
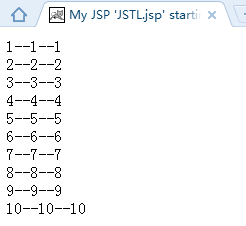
b.格式2:
<c:forEach items="${要遍历的集合,数组}" var="元素">
1)遍历list集合
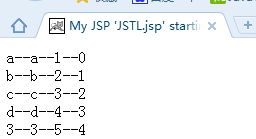
2)遍历map集合
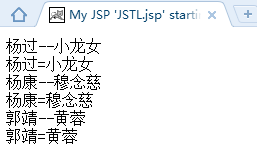
6.c:url
作用:补全项目路径;自动进行url重写
1.概述
JSP:Java Server Page Java服务器页面语言
本质:是一个Servlet,即可以写HTML语言,也可以写Java语言
语法:早期技术,JSP脚本
<%! int num = 1000;%>生成的Java代码在成员位置
<%System.out.println("hello world");%>生成的代码在Service()方法中
<%="hello world"%>生成的东西在页面上,即:out.print("hello world");
JSP专用注释:<%-- --%>:不会发送到客户端,可以注释代码
原理:
2.3大指令
a.概述
指令:配置JSP页面的属性或者导入一些额外的内容
格式:<%@ 指令名称 属性名="属性值"%>
分类:
page:配置JSP页面的属性
include:静态包含(不常用,基本用动态包含)
taglib:导入额外的内容
d.page指令:
language:指定jsp页面使用的语言,只有一个值
import:导入软件包
pageEncoding:设置页面编码
指定jsp文件的编码
高速浏览器页面使用的编码格式
autoFlush:自动刷新
errorPage:指定错误页面,当页面发生异常时,指定跳转的页面
一般在开发完成后,会在web.xml中通过<error-page>标签来配置整个项目的错误页面
当服务器出现问题时,用户不会看到404或者500页面,不让用户知道服务器出现问题
<error-page> <error-code>500</error-code> <location>/500.jsp</location> </error-page> <error-page> <error-code>404</error-code> <location>/404.jsp</location> </error-page>
isErrorPage:该页面是否是错误页面
true:是,可以使用内置对象exception,上面提到的404.jsp就要设置为错误页面
false:不是
<%@ page language="java" import="java.util.*" pageEncoding="utf-8"%> <!-- errorPage在这里不设置,一般都在web.xml里配置 --> <%@ page autoFlush="true" buffer="8kb" errorPage="/500.jsp" isErrorPage="true" %>
e.taglib指令
导入额外的内容,如jstl标签
<!-- 一般都定义为c标签 --> <%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %>
f.include指令
静态包含,包含后,只会存在一个.class文件
包含进去的内容只会显示在页面最上方,所以不常用
<%@ include file="/demo.jsp" %>
3.6个常用动作标签
a.替换Java代码,简化书写
1)创建一个Student类
成员变量:username,age
有参无参构造
set/get方法
2)使用Jsp脚本创建对象,并设置获取参数
<body> <% Student stu = new Student(); stu.setUsername("张三"); stu.setAge("20"); String username = stu.getUsername(); String age = stu.getAge(); // 请求转发 request.getRequestDispatcher("").forward(request, response); // 包含页面 request.getRequestDispatcher("").include(request, response); %> </body>
b.<jsp:useBean id="stu" class="org.xxxx.demo.Student"> 创建对象
id:对象名
class:类的全路径名称
Student stu = new Student()
c.<jsp:setProperty property="username" name="stu" value="张三">设置属性值
property:字段名--setUsername()中,set后面的就叫字段
name:对象名
value:属性值
stu.setUsername("张三");
d.<jsp:getProperty property="username" name="stu">获取属性值
property:字段名
name:对象名
stu.getUsername
e.<jsp:include>动态包含文件
f.<jsp:forward>请求转发
一般服务器WebRoot目录下只放一个首页,用户可以通过路径访问
其他内部页面放在Web-INF目录下,该目录下文件无法通过链接直接访问
必须通过内部跳转才能访问
防止用户通过输入链接而直接越过首页
1)WebRoot目录下的index.jsp(全删除,就写这三行)
<jsp:forward page="/demo01"> <jsp:param value="100" name="num"/> </jsp:forward>
2)提交到后台Servlet,做一些逻辑判断,实现跳转到内部链接
package org.xxxx.demo; import java.io.IOException; import javax.servlet.ServletException; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; public class MyServlet extends HttpServlet { public void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { // 做逻辑判断 String parameter = request.getParameter("num"); System.out.println(parameter); // 请求转发 request.getRequestDispatcher("/WEB-INF/MyJSP.jsp").forward(request, response); } public void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { this.doGet(request, response); } }
3)内部链接MyJSP.jsp
<%@ page language="java" import="java.util.*" pageEncoding="UTF-8"%> <% String path = request.getContextPath(); String basePath = request.getScheme()+"://"+request.getServerName()+":"+request.getServerPort()+path+"/"; %> <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN"> <html> <head> <base href="<%=basePath%>"> <title>内部页面</title> <meta http-equiv="pragma" content="no-cache"> <meta http-equiv="cache-control" content="no-cache"> <meta http-equiv="expires" content="0"> <meta http-equiv="keywords" content="keyword1,keyword2,keyword3"> <meta http-equiv="description" content="This is my page"> </head> <body> This is my JSP page. <br> </body> </html>
4)运行服务器,观察浏览器地址栏不会发生变化,用户并不知道我们的网页发生跳转
5)手动输入MyJSP.jsp地址,看能否进入该页面
g.<jsp:param>传递请求参数
<body> <%-- 创建对象 id:创建的对象名 class:类的全路径名称 Student stu = new Student --%> <jsp:useBean id="stu" class="org.xxxx.demo.Student"></jsp:useBean> <%-- 设置属性名 property:字段名(setUsername/getUsername后面的部分username被称为字段) --%> <%-- name:对象名 value:属性值 --%> <jsp:setProperty property="username" name="stu" value="张三"/> <jsp:setProperty property="age" name="stu" value="20"/> <%-- 获取属性值 --%> <jsp:getProperty property="username" name="stu"/> <jsp:getProperty property="age" name="stu"/> <%-- 动态包含 将demo.jsp内容包含进来 --%> <jsp:include page="/demo.jsp"></jsp:include> <%-- 请求转发 跳转到demo.jsp中--%> <jsp:forward page="/demo.jsp"> <%-- 携带请求参数 将num转发到demo.jsp中 --%> <jsp:param value="100" name="num"/> </jsp:forward> </body>
4.9个常用内置对象
a.当jsp转换成service()方法时,会在该方法里声明好9个内置对象
b.pageContext
页面域,可以通过它获取其他八个对象
<body> <% Exception exception2 = pageContext.getException(); JspWriter out2 = pageContext.getOut(); Object page2 = pageContext.getPage(); ServletRequest request2 = pageContext.getRequest(); ServletResponse response2 = pageContext.getResponse(); HttpSession session2 = pageContext.getSession(); ServletContext application2 = pageContext.getServletContext(); ServletConfig config2 = pageContext.getServletConfig(); %> </body>
c.application:ServletContext 全局域
d.session:HttpSession 会话域
e.request:HttpServletRequest 请求域
f.out:JspWriter 输出内容在body内
常用
out.print("hello world");
g.response:HttpServletResponse 输出内容在html标签外
不常用
response.getWriter().print("hello world");
h.config:ServletConfig 配置对象
i.page:this 当前类
j.expection:Throwable:错误信息,(必须设定为错误页面方可调用)
B.EL
1.概述
JSP的内置表达式语言,用来代替JSP脚本
作用:
a.获取域中数据
b.执行运算
c.获取常见的web对象
d.调用java的方法
格式:${el表达式}
2.获取域中的数据
a.简单的数据
${属性名}:依次从pageContext,request,session,applocation查找
如果查找不到,返回""
1)例子
<body> <% request.setAttribute("rkey", "rvalue"); session.setAttribute("skey", "svalue"); pageContext.setAttribute("pkey", "pvalue"); application.setAttribute("akey", "avalue"); %> <%-- 老方式 --%> <%=request.getAttribute("rkey") %><br /> <%-- el表达式 --%> ${ rkey }<br /> ${ skey }<br /> ${ pkey }<br /> ${ akey }<br /> </body>
运行,验证输出
2)当四个域的key都一样,会获取最小域(pageContext)的值
如果要都获取怎么办?Scope(pageContext不需要)
<body> <% request.setAttribute("key", "rvalue"); session.setAttribute("key", "svalue"); pageContext.setAttribute("key", "pvalue"); application.setAttribute("key", "avalue"); %> <%-- el表达式 --%> ${ requestScope.key }<br /> ${ sessionScope.key }<br /> ${ pageScope.key }<br /> ${ applicationScope.key }<br /> </body>
b.获取复杂数据
1)获取数组中的数据
${域中的名称[index]}
<body> <% // 往域中存放数组 request.setAttribute("arr", new String[]{"a", "b", "c", "d"}); %> <%-- 获取域中的数组 --%> el方式:${ arr[1] } </body>
2)获取list中的数据
${域中的名称[index]}
<body> <% // 往域中存放list集合 ArrayList<String> list = new ArrayList(); list.add("hello"); list.add("world"); list.add("java"); request.setAttribute("list", list); %> <%-- 获取域中的集合 --%> el方式:${ list[1] }<br> list的长度:${ list.size() } </body>
3)获取map中的数据
${域中的名称.键名}
<body> <% // 往域中存放map集合 HashMap<String, String> map = new HashMap(); map.put("username", "Tom"); map.put("age", "20"); request.setAttribute("map", map); %> <%-- 获取域中的集合 --%> ${ map.username }<br> ${ map.age }<br> </body>
c.获取对象中的字段值
<body> <%-- 创建对象 --%> <jsp:useBean id="stu" class="org.xxxx.demo.Student"></jsp:useBean> <jsp:setProperty property="username" name="stu" value="张三"/> <jsp:setProperty property="age" name="stu" value="20"/> <%-- 将对象放入域中 --%> <% request.setAttribute("student", stu); %> <%-- 获取字段值 --%> ${ student.username }<br> ${ student.age }<br> </body>
d.执行运算
算术运算,逻辑运算,三元运算
empty:判断一个容器的长度是否为0(数组,集合)还可以判断一个对象是否为空
${empty 域中的对象名称}
判断非空:${not emoty 域中的对象名称}
注意:+只能进行加法运算,字符串形式数字可以进行加法运算
代码演示:
<body> <%-- 将对象放入域中 --%> <% request.setAttribute("i", 3); request.setAttribute("j", 4); request.setAttribute("q", 12); // 空集合 ArrayList<String> list = new ArrayList(); request.setAttribute("list", list); // 空对象 Student stu1 = null; request.setAttribute("stu1", stu1); // 非空对象 Student stu2 = new Student(); request.setAttribute("stu2", stu2); %> <%-- 获取字段值 --%> i + j = ${ i + j }<br> i + k = ${ i + k }<br> q / i = ${ q / i }<br> list长度是否为0:${ empty list }<br> list长度是否不为0:${ not empty list }<br> stu1是否为空:${ empty stu1 }<br> stu1是否不为空:${ not empty stu1 }<br> stu2是否为空:${ empty stu2 }<br> stu2是否不为空:${ not empty stu2 }<br> </body>
3.11个内置对象
pageScope
requestScope
sessionScope
applicationScope
parm
parmValues
header
headerValues
initParam
cookie
pageContext
4.cookie内置对象
<body> <%-- 将对象放入域中 --%> <% Cookie c = new Cookie("username", "Tom"); c.setMaxAge(60 * 60 * 24 * 7); response.addCookie(c); %> <%-- 获取Cookie值 --%> ${ cookie.username.name }<br> ${ cookie.username.value }<br> ${ cookie.JSESSIONID.value }<br> </body>
5.pageContext
在jsp页面中获取项目名
在使用跳转可以使用动态路径
做一个跳转页面
<%@page import="org.xxxx.demo.Student"%> <%@ page language="java" import="java.util.*" pageEncoding="UTF-8"%> <% String path = request.getContextPath(); String basePath = request.getScheme()+"://"+request.getServerName()+":"+request.getServerPort()+path+"/"; %> <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN"> <html> <head> <base href="<%=basePath%>"> <title>My JSP 'el.jsp' starting page</title> <meta http-equiv="pragma" content="no-cache"> <meta http-equiv="cache-control" content="no-cache"> <meta http-equiv="expires" content="0"> <meta http-equiv="keywords" content="keyword1,keyword2,keyword3"> <meta http-equiv="description" content="This is my page"> </head> <body> 当前工程路径:${ pageContext.request.contextPath }<br> <a href="${ pageContext.request.contextPath }/demo01">跳转</a> </body> </html>跳转到服务器页面
package org.xxxx.demo; import java.io.IOException; import javax.servlet.ServletException; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; public class MyServlet extends HttpServlet { public void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { System.out.println("请求来了!"); String contextPath = request.getContextPath(); System.out.println(contextPath); } public void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { this.doGet(request, response); } }运行浏览器
点击跳转,观察控制台
C.JSTL
1.概述
JSP标准的标签库语言,apache开发,用来替代jsp脚本,结合EL使用
2.使用步骤
a.导入jar包(jstl.jar和standard.jar)
b.在页面上导入标签库
<%@taglib prefix="" uri=""%>
MyEclipse中已经集成了jar包,我们只需要导入就行
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %>prefix默认写c,行内通用
3.jstl的分类
core:核心类库
c:if
c:foreach
fmt:格式化|国际化
xml:过时
sql:过时
函数库:很少用
4.c:if判断
<c:if test="${el表达式}">满足的时候输出内容</c:if>;没有else
<%@ page language="java" import="java.util.*" pageEncoding="UTF-8"%> <%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %> <% String path = request.getContextPath(); String basePath = request.getScheme()+"://"+request.getServerName()+":"+request.getServerPort()+path+"/"; %> <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN"> <html> <head> <base href="<%=basePath%>"> <title>My JSP 'JSTL.jsp' starting page</title> </head> <body> <c:if test="${ 3 > 4 }"> 3大于4 </c:if> <c:if test="${ 3 <= 4 }"> 3不大于4 </c:if> </body> </html>
5.c:forEach循环
a.格式1:
<c:forEach begin="起始" end="结束" step="步长" var="变量名" varStatus="循环状态变量">
varStatus:用来记录循环的状态
count:记录次数
current:当前便利的内容
index:索引从0开始
<body> <c:forEach begin="1" end="10" step="1" var="i" varStatus="vs"> ${ i }--${ vs.count }--${ vs.current }<br> </c:forEach> </body>
b.格式2:
<c:forEach items="${要遍历的集合,数组}" var="元素">
1)遍历list集合
<body> <% ArrayList<String> list = new ArrayList(); list.add("a"); list.add("b"); list.add("c"); list.add("d"); list.add("3"); session.setAttribute("list", list); %> <c:forEach items="${ list }" var="str" varStatus="vs"> ${ str }--${ vs.current }--${ vs.count }--${ vs.index }<br> </c:forEach> </body>
2)遍历map集合
<body> <% HashMap<String, String> hm = new HashMap(); hm.put("郭靖", "黄蓉"); hm.put("杨康", "穆念慈"); hm.put("杨过", "小龙女"); session.setAttribute("map", hm); %> <%-- 获取键值是getKey()/getValue(),字段名就是key/value --%> <c:forEach items="${ map }" var="m" varStatus="vs"> ${ m.key }--${ m.value }<br> ${ vs.current }<br> </c:forEach> </body>
6.c:url
作用:补全项目路径;自动进行url重写
<body> <%-- 补全路径 --%> <c:url value="/index.jsp"></c:url> </body>
相关文章推荐
- 【JavaEE】jsp学习笔记之el技术和jstl
- jsp学习笔记,EL,JSTL
- 【JAVAWEB学习笔记】18_el&jstl&javaee的开发模式
- JSP学习笔记--EL和JSTL
- JavaEE学习笔记之Servlet/JSP(6)
- SpringMVC学习笔记(4) ModelView传值 EL+JSTL
- EL表达式 学习笔记(JSTL)
- JSP之JSTL学习笔记
- 【知了堂学习笔记】_Java中EL和JSTL的学习
- JSP标签学习笔记(内置标签+JSTL标签)
- 【JSTL EL】 jsp 页面学习
- 重温JSP学习笔记--JSTL标签库
- Servlet与Jsp学习笔记--8、JSTL
- JSP学习笔记1_JSP脚本语言(来自李刚的JAVAEE)
- 重温JSP学习笔记--与日期数字格式化有关的jstl标签库
- JSP EL学习笔记
- 关于ognl+struts-tag与el+jstl互相代替,以及el和jstl的学习笔记
- jstl与el学习笔记
- EL&JSTL表达式学习笔记 -- day01
- [读书笔记]JSP_Servlet学习笔记-JSTL