WebService之CXF整合Spring框架发布REST服务
2017-10-29 19:36
561 查看
前一段时间曾用过CXF发布SOAP服务生成静态页面,因为对安全性上有要求,并且也不需要返回特别复杂的数据,只返回一个标志,所以没有选择发布REST,不过REST还是需要知道的,因为REST优点还是很多的。
创建Web项目,并将CXF中的jar包copy到项目
创建bean实体类
3.创建接口
创建接口的实现类
在项目中创建config文件夹(Source Folder),并创建applicationContext.xml文件,内容如下:
配置web.xml文件
启动Tomcat发布服务
查看服务说明,地址:http://localhost:8080/cxfRestSpringService/rest/item/shop?_wadl(说明:cxfRestSpringService是项目名称,rest是在web.xml配置的拦截转发路径,item是applicationContext.xml配置的address,shop是接口上注解的@Path(“/shop”)值 ,而REST服务不是W3C认证的,所以他有自己的服务说明访问地址,在后面加上”?_wadl”,这是REST服务独有的。
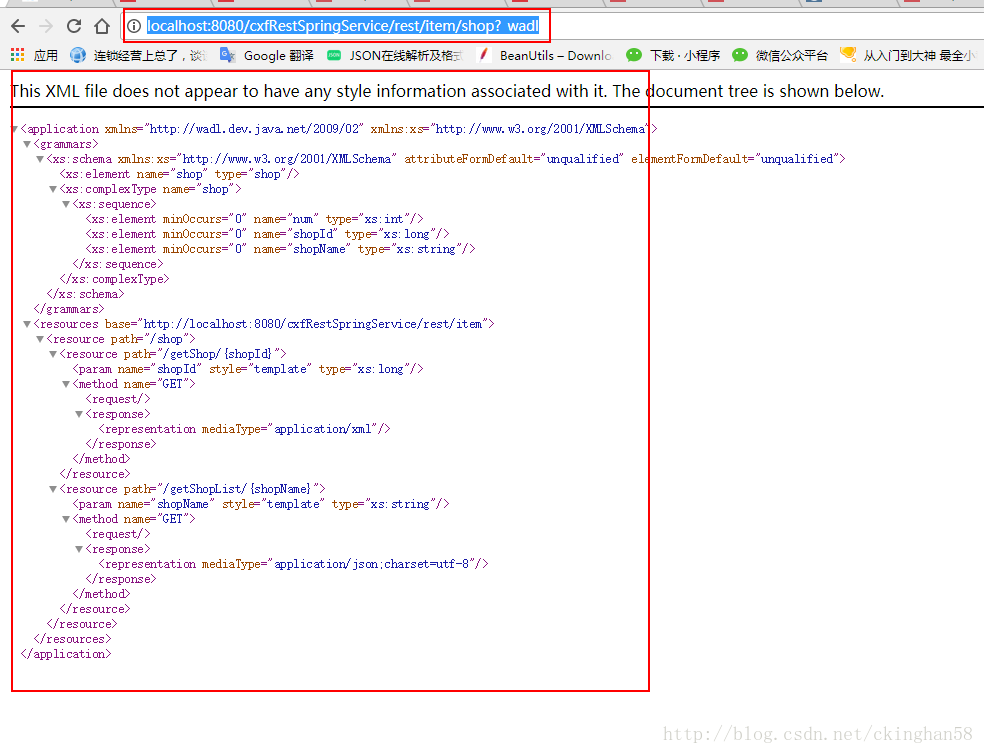
访问接口方法一:http://localhost:8080/cxfRestSpringService/rest/item/shop/getShop/1001
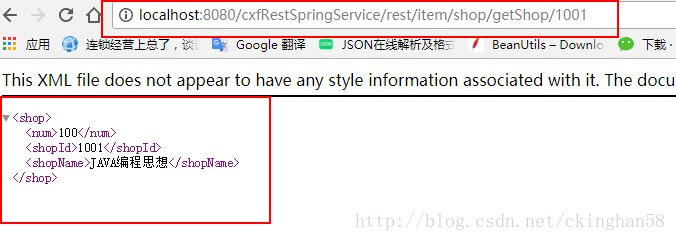
访问接口方法二:http://localhost:8080/cxfRestSpringService/rest/item/shop/getShopList/JAVA%E7%BC%96%E7%A8%8B%E6%80%9D%E6%83%B3

如果能出现上述的页面,说明 已经 发布功能了,至于客户端……这不须要客户端了,因为它已经返回了数据,你只需要将数据进行解析就行了。你可以在网页上通过AJAX、http直接访问获取数据。。。
创建Web项目,并将CXF中的jar包copy到项目
创建bean实体类
package com.ckinghan.bean; import javax.xml.bind.annotation.XmlRootElement; //XML与实体类进行转换,并指定名称 @XmlRootElement(name="shop") public class Shop { private Long shopId; private String shopName; private Integer num; public Long getShopId() { return shopId; } public void setShopId(Long shopId) { this.shopId = shopId; } public String getShopName() { return shopName; } public void setShopName(String shopName) { this.shopName = shopName; } public Integer getNum() { return num; } public void setNum(Integer num) { this.num = num; } }
3.创建接口
package com.ckinghan.cxf.rest.service; import java.util.List; import javax.jws.WebService; import javax.ws.rs.GET; import javax.ws.rs.Path; import javax.ws.rs.PathParam; import javax.ws.rs.Produces; import javax.ws.rs.core.MediaType; import com.ckinghan.bean.Shop; //加入webService的注解 @WebService //访问路径 @Path("/shop") public interface ShopService { //只能使用get方式访问 @GET //指定返回值类型为XML @Produces(MediaType.APPLICATION_XML) //访问路径及参数 @Path("/getShop/{shopId}") public Shop getShopById(@PathParam("shopId")Long shopId); //指定路径参数,要与上面的"@Path("/getShop/{shopId}")"中的shopId相同 @GET //指定返回值类型为JSON,并指定了编码,以免出现中文乱码的问题 @Produces("application/json;charset=utf-8") @Path("/getShopList/{shopName}") public List<Shop> getShopListByName(@PathParam("shopName")String shopName); }
创建接口的实现类
package com.ckinghan.cxf.rest.service; import java.util.ArrayList; import java.util.List; import com.ckinghan.bean.Shop; public class ShopServiceImpl implements ShopService { @Override public Shop getShopById(Long shopId) { if(shopId == 1001L){ Shop shop = new Shop(); shop.setNum(100); shop.setShopId(1001L); shop.setShopName("JAVA编程思想"); return shop; } return null; } @Override public List<Shop> getShopListByName(String shopName) { if(shopName == null || !"JAVA编程思想".equals(shopName)){ return null; } List<Shop> shopList = new ArrayList<Shop>(); Shop shop = new Shop(); shop.setNum(100); shop.setShopId(1001L); shop.setShopName("JAVA编程思想"); Shop shop1 = new Shop(); shop1.setNum(20); shop1.setShopId(1002L); shop1.setShopName("JAVA编程思想"); Shop shop2 = new Shop(); shop2.setNum(92); shop2.setShopId(1003L); shop2.setShopName("JAVA编程思想"); shopList.add(shop); shopList.add(shop1); shopList.add(shop2); return shopList; } }
在项目中创建config文件夹(Source Folder),并创建applicationContext.xml文件,内容如下:
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:jaxws="http://cxf.apache.org/jaxws" xmlns:jaxrs="http://cxf.apache.org/jaxrs" xmlns:cxf="http://cxf.apache.org/core" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://cxf.apache.org/jaxrs http://cxf.apache.org/schemas/jaxrs.xsd http://cxf.apache.org/jaxws http://cxf.apache.org/schemas/jaxws.xsd http://cxf.apache.org/core http://cxf.apache.org/schemas/core.xsd"> <!-- 配置WebService的rest服务发布,它是对jaxrsServerFactoryBean的封装。--> <jaxrs:server address="/item" > <jaxrs:serviceBeans> <ref bean="shopService"/> </jaxrs:serviceBeans> </jaxrs:server> <!-- 配置shopService实现类 --> <bean name="shopService" class="com.ckinghan.cxf.rest.service.ShopServiceImpl"/> </beans>
配置web.xml文件
<?xml version="1.0" encoding="UTF-8"?> <web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://java.sun.com/xml/ns/javaee" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd" id="WebApp_ID" version="3.0"> <display-name>cxfRestSpringService</display-name> <context-param> <param-name>contextConfigLocation</param-name> <param-value>classpath:applicationContext.xml</param-value> </context-param> <listener> <listener-class>org.springframework.web.context.ContextLoaderListener</listener-class> </listener> <servlet> <servlet-name>CXF_REST</servlet-name> <servlet-class>org.apache.cxf.transport.servlet.CXFServlet</servlet-class> </servlet> <servlet-mapping> <servlet-name>CXF_REST</servlet-name> <url-pattern>/rest/*</url-pattern> </servlet-mapping> <welcome-file-list> <welcome-file>index.html</welcome-file> <welcome-file>index.htm</welcome-file> <welcome-file>index.jsp</welcome-file> <welcome-file>default.html</welcome-file> <welcome-file>default.htm</welcome-file> <welcome-file>default.jsp</welcome-file> </welcome-file-list> </web-app>
启动Tomcat发布服务
查看服务说明,地址:http://localhost:8080/cxfRestSpringService/rest/item/shop?_wadl(说明:cxfRestSpringService是项目名称,rest是在web.xml配置的拦截转发路径,item是applicationContext.xml配置的address,shop是接口上注解的@Path(“/shop”)值 ,而REST服务不是W3C认证的,所以他有自己的服务说明访问地址,在后面加上”?_wadl”,这是REST服务独有的。
访问接口方法一:http://localhost:8080/cxfRestSpringService/rest/item/shop/getShop/1001
访问接口方法二:http://localhost:8080/cxfRestSpringService/rest/item/shop/getShopList/JAVA%E7%BC%96%E7%A8%8B%E6%80%9D%E6%83%B3
如果能出现上述的页面,说明 已经 发布功能了,至于客户端……这不须要客户端了,因为它已经返回了数据,你只需要将数据进行解析就行了。你可以在网页上通过AJAX、http直接访问获取数据。。。
相关文章推荐
- webservice--CXF+Spring整合发布REST的服务
- 8、CXF与Spring整合发布http rest 风格的WebService服务
- Spring整合CXF 发布webservice接口服务器(普通及REST)和客户端,WSDL简单解析
- SpringBoot整合cxf发布WebService服务和客户端调用WebService服务
- spring boot整合cxf发布webservice服务和spring boot整合cxf客户端调用webservice服务
- Spring整合CXF之发布WebService服务
- Spring整合CXF之发布WebService服务
- java开发中使用CXF发布rest风格的webservice服务
- spring boot整合cxf发布webservice服务和cxf客户端调用
- 使用cxf发布rest服务接口,和spring的整合
- spring整合cxf方式发布的rest webservice,调用接口
- (四)CXF整合Spring发布WebService服务
- webservice--CXF+Spring整合发布SOAP协议的服务
- cxfspring boot整合cxf发布webservice服务和cxf客户端调用
- spring boot整合cxf发布webservice服务和cxf客户端调用
- Spring整合CXF配置WebService服务发布
- Maven+SpringMVC+CXF整合实现webservice服务的发布与调用
- WebService的CXF框架与Spring的整合发布服务入门
- webservice--cxf和spring结合,发布restFull风格的服务
- CXF+Spring+REST发布webservice服务