使用Java读取xlxs文件和写入txt文件,并将数据写入到本地文件
2017-10-11 15:31
337 查看
小编今天要给大家分享的是从xlxs文件中将数据读取出来,并将数据写入到本地txt文件中。
那么为啥有这个分享呢,来看看背景介绍:
背景:在实际开发中,通常会用到使用xlxs来提取业务的需求,同时在xlxs文件中会有大量的数据用于业务开发的使用中,应趋势所取,便有了现在这个分享。
好了,一起走进小编的代码,一个简单的小应用,解决手动导入的麻烦.
我要从xlxs中将数据取出,并将数据存放到有一定格式的txt文件中,
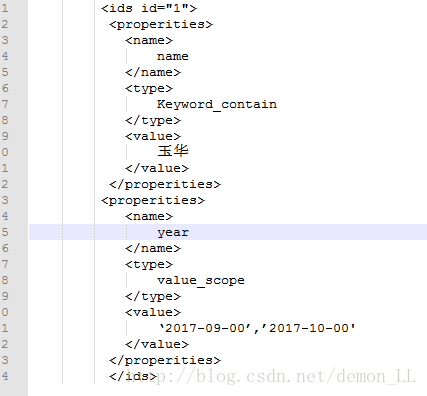
从xlxs文件中将数据取出,作为IT人员不能手动弄吧,这也太对不起自己的专业了吧,O(∩_∩)O哈哈~
使用代码之前,小编用的是idea maven项目,当然要用pom文件,所需maven-pom文件,下载地址:
http://download.csdn.net/download/demon_ll/10016057
代码所示
读取xlsx文件:
那么为啥有这个分享呢,来看看背景介绍:
背景:在实际开发中,通常会用到使用xlxs来提取业务的需求,同时在xlxs文件中会有大量的数据用于业务开发的使用中,应趋势所取,便有了现在这个分享。
好了,一起走进小编的代码,一个简单的小应用,解决手动导入的麻烦.
我要从xlxs中将数据取出,并将数据存放到有一定格式的txt文件中,
从xlxs文件中将数据取出,作为IT人员不能手动弄吧,这也太对不起自己的专业了吧,O(∩_∩)O哈哈~
使用代码之前,小编用的是idea maven项目,当然要用pom文件,所需maven-pom文件,下载地址:
http://download.csdn.net/download/demon_ll/10016057
代码所示
读取xlsx文件:
import java.io.FileInputStream; import java.io.FileNotFoundException; import java.io.IOException; import java.io.InputStream; import java.util.ArrayList; import java.util.List; import org.apache.log4j.Logger; import org.apache.poi.hssf.usermodel.HSSFWorkbook; import org.apache.poi.ss.usermodel.Cell; import org.apache.poi.ss.usermodel.Row; import org.apache.poi.ss.usermodel.Sheet; import org.apache.poi.ss.usermodel.Workbook; import org.apache.poi.xssf.usermodel.XSSFWorkbook; /** * excel读写工具类 */ public class POIUtils { private static Logger logger = Logger.getLogger(POIUtil.class); private final static String xls = "xls"; private final static String xlsx = "xlsx"; /** * 读入excel文件,解析后返回 * @param file * @throws IOException */ public static List<String[]> readExcel(String file) throws IOException{ checkFile(file); Workbook workbook = getWorkBook(file); List<String[]> list = new ArrayList<String[]>(); if(workbook != null){ for(int sheetNum = 0;sheetNum < workbook.getNumberOfSheets();sheetNum++){ Sheet sheet = workbook.getSheetAt(sheetNum); if(sheet == null){ continue; } int firstRowNum = sheet.getFirstRowNum(); int lastRowNum = sheet.getLastRowNum(); for(int rowNum = firstRowNum+1;rowNum <= lastRowNum;rowNum++){ Row row = sheet.getRow(rowNum); if(row == null){ continue; } int firstCellNum = row.getFirstCellNum(); int lastCellNum = row.getPhysicalNumberOfCells(); String[] cells = new String[row.getPhysicalNumberOfCells()]; for(int cellNum = firstCellNum; cellNum < lastCellNum;cellNum++){ Cell cell = row.getCell(cellNum); cells[cellNum] = getCellValue(cell); } list.add(cells); } } workbook.close(); } return list; } public static void checkFile(String file) throws IOException{ if(null == file){ logger.error("文件不存在!"); throw new FileNotFoundException("文件不存在!"); } String fileName = file; if(!fileName.endsWith(xls) && !fileName.endsWith(xlsx)){ logger.error(fileName + "不是excel文件"); throw new IOException(fileName + "不是excel文件"); } } public static Workbook getWorkBook(String file) { String fileName = file; Workbook workbook = null; try { InputStream is = new FileInputStream(file); if(fileName.endsWith(xls)){ workbook = new HSSFWorkbook(is); }else if(fileName.endsWith(xlsx)){ workbook = new XSSFWorkbook(is); } } catch (IOException e) { logger.info(e.getMessage()); } return workbook; } public static String getCellValue(Cell cell){ String cellValue = ""; if(cell == null){ return cellValue; } if(cell.getCellType() == Cell.CELL_TYPE_NUMERIC){ cell.setCellType(Cell.CELL_TYPE_STRING); } switch (cell.getCellType()){ case Cell.CELL_TYPE_NUMERIC: //数字 cellValue = String.valueOf(cell.getNumericCellValue()); break; case Cell.CELL_TYPE_STRING: //字符串 cellValue = String.valueOf(cell.getStringCellValue()); break; case Cell.CELL_TYPE_BOOLEAN: //Boolean cellValue = String.valueOf(cell.getBooleanCellValue()); break; case Cell.CELL_TYPE_FORMULA: //公式 cellValue = String.valueOf(cell.getCellFormula()); break; case Cell.CELL_TYPE_BLANK: //空值 cellValue = ""; break; case Cell.CELL_TYPE_ERROR: //故障 cellValue = "非法字符"; break; default: cellValue = "未知类型"; break; } return cellValue; } }
/** * Created by Administrator on 2017/10/11. */ import java.io.BufferedWriter; import java.io.File; import java.io.FileWriter; import java.io.IOException; import java.util.List; public class ReadXlsx { public static void main(String[] args){ try { //本地绝对路径 String readFile = "D:/zling/users.xlsx"; String writeFile="D:/zling/textFile.txt"; List<String[]> userList = POIUtil.readExcel(readFile); String outfiles=""; for (int i = 0; i < userList.size(); i++) { String[] users = userList.get(i); //users[1]为第二列 outfiles+="<ids id=\""+users[1]+"\">\n" + " <properities>\n" + "\t\t\t<name>\n" + "\t\t\t\tname\n" + "\t\t\t</name>\n" + " <type>\n" + "\t\t\t\tKeyword_contain\n" + "\t\t\t</type>\n" + " <value>\n" + "\t\t\t\t"+users[4]+"\n" + "\t\t\t</value>\n" + "\t\t </properities>\n" + " <properities>\n" + "\t\t\t<name>\n" + "\t\t\t\tyear\n" + "\t\t\t</name>\n" + " <type>\n" + "\t\t\t\tvalue_scope\n" + "\t\t\t</type>\n" + " <value>\n" + "\t\t\t\t‘2017-09-00’,’2017-10-00’\n" + "\t\t\t</value>\n" + "\t\t </properities>\n" + "\t\t</ids>"; } /* 写入Txt文件 */ File writename = new File(writeFile); // 相对路径,如果没有则要建立一个新的output。txt文件 writename.createNewFile(); // 创建新文件 BufferedWriter out = new BufferedWriter(new FileWriter(writename)); out.write(outfiles); // \r\n即为换行 out.flush(); // 把缓存区内容压入文件 out.close(); // 最后记得关闭文件 System.out.print("保存文件成功"); } catch (IOException e) { System.out.print("异常"); } } }
这样就ok了,很容易。
小编所分享的到此结束了
相关文章推荐
- 【java IO】使用Java输入输出流 读取txt文件内数据,进行拼接后写入到另一个文件中
- java中如何获取远程计算机post提交的数据并把这些数据写入本地的txt文件中
- java读取数据写入txt文件并将读取txt文件写入另外一个表
- Java 使用DataInputStream将数据写入文件,使用FileReader读取演示
- 使用java语言将数组中的数据写入txt文件,然后读取
- java读取Excel数据,然后写入到txt文件,并批量保存到oracle数据库中
- 如何使用JavaExcel(jxl)读取一个文件并写入一个新文件
- 读取、回收和重用:使用 Excel、XML 和 Java 技术轻松搞定报告使用 Java 和 XML 技术读取 Excel 文件并写入新文件(1)
- Java从.CSV文件中读取数据和写入
- java向txt文件中写入数据时换行
- JAVA 创建TXT文件,写入文件内容,读取文件内容
- 通过java的io流将本地文件读取到控制台,并将文件内容再次写入另一个文件中
- Java读取txt文件 copy文件 写入文件
- FBReader打开txt优化方案,加入本地格式好后得数据写入和读取
- Java 读取本地 UTF8 txt文件乱码处理
- JAVA 创建TXT文件,写入文件内容,读取文件内容
- Java使用URLConnection写入和读取数据
- java中使用JXL对Excel文件进行数据的写入、导出操作
- Java-流的简单使用:读取文件、写入文件(面试题:删除注释代码)
- 使用java读取txt里边的文件内容并获取大小(M).txt