JavaSE基础练习
2017-10-09 17:54
543 查看
1.考试成绩已经保存在数组scores中,依次为89,23,64,91,119,52,73,-23.要求根据通过自定义方法来找出其中前三名,将数组成绩作为参数传入,要求判断成绩的有效性(0-100)如果成绩无效则忽略此成绩。
测试结果:

2.用数组来实现,定义并初始化一个(1-100)保存100个数的数组,从第一个元素开始,依次数(1,2,3循环往复),每次数到3的元素淘汰掉,当达到数组末尾的时候再从头开始,直到最后剩余一个元素,写出算法,并输出最后保留的元素所在的最初位置。

3.用数组来实现对于一个整型数组,分别通过冒泡排序和快速排序,实现对于任意一个数组进行由小到大的排序。
测试结果:


4.判断101到200之间有多少个素数,并输出所有的素数。
测试结果:

5.输入一行字符,分别统计出其中英文字母,空格,数字和其他字符的个数。
测试结果:
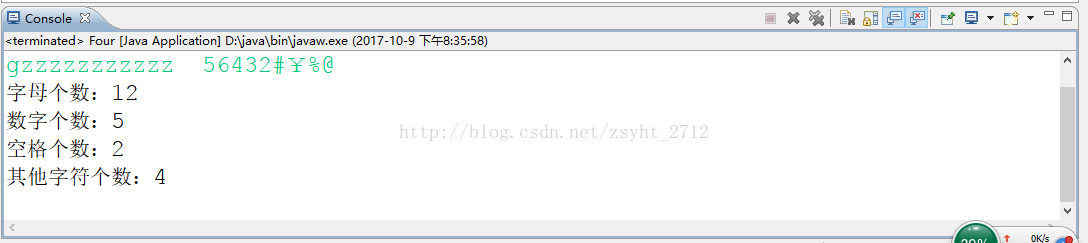
6.企业发放的奖金根据利润提成,利润低于或等于10万元时,奖金可提10%;利润高于10万元时低于20万元时,低于10万元的部分按10%提成,高于十万元的部分可提成7.5%;20万到40万之间时,高于20万元的部分可提成5%;40万到60万之间时高于40万元的部分可提成3%;60万到100万之间时,高于60万的部分可提成1.5%,超过100万元的部分按1%提成,从键盘输入当月利润,求应发放奖金总数。
测试结果:

7.分别定义用户类,订单类,产品类,其中订单类至少要具有下订单的行为(入参为一个产品名称),产品类中至少有一个成员变量为产品名称,至少有一个方法用来获取产品的名称,用户类中持有一个产品类型的成员变量,持有一个订单类型的 并且调用成员变量订单的对象成员变量。在我们的用户类中定义main函数,当执行的时候,构造一个用户类的对象,并且通过手段可以拿到产品成员变量中的产品名称,,进行下单,最后输出下单成功。
package task; import java.util.Arrays; //引用数组类默认排序 public class one { public static void main(String [] args){ one test = new one(); //创建一个对象 //定义数组 int [] scores ={89,23,64,91,119,52,73,-23}; System.out.println("考试前三名为:"); //调用类的方法输出结果 test.methods(scores); } void methods (int [] scores){ //使用数组的sort()方法排序 Arrays.sort(scores); int count = 0; //初始化变量 for(int i =scores.length-1;i>=0;i--){ //判断成绩是否有效,若无效则跳过该成绩 if(scores[i]<0||scores[i]>100){ continue; } count++; System.out.print(scores[i]+" "); //累计输出成绩数为三,终止循环 if(count == 3){ break; } } } }
测试结果:
2.用数组来实现,定义并初始化一个(1-100)保存100个数的数组,从第一个元素开始,依次数(1,2,3循环往复),每次数到3的元素淘汰掉,当达到数组末尾的时候再从头开始,直到最后剩余一个元素,写出算法,并输出最后保留的元素所在的最初位置。
package task; public class Seven { static void Arr(int[] num) { for(int i = 0;i < num.length;i++) num[i] = i + 1; } static void methods(int[] num) { //计数器 int a = 0; //被淘汰的数的位置 int s = 0; //下标 int i = 0; //每次的循环的数字 int loop = 0; while(a != 100) { //通过该数是否为0 判断该数是否已经被淘汰 if(num[i] != 0) { //判断循环的数字是否到3 if(loop == 2) { //如果达到3 num[i] = 0; //该数置为0 a++; //计数器自加 s = i; //记录位置 loop = 0; //循环计数器 重置为0 } else { loop++; } } i++; if (i == 100) { i = 0; } } System.out.println("最终淘汰的元素的下标为 " + s); } public static void main(String[] args) { int[] num = new int[100]; Arr(num); methods(num); } }
3.用数组来实现对于一个整型数组,分别通过冒泡排序和快速排序,实现对于任意一个数组进行由小到大的排序。
package task; public class Five { public static void main(String [] args){ //冒泡排序 int []arr = new int[]{5,89,46,3,445,122,30,18}; for(int i=0;i<arr.length-1;i++) for( int j=i+1;j<arr.length;j++){ if(arr[i]>arr[j]){ int temp; temp=arr[i]; arr[i]=arr[j]; arr[j]=temp; } } for(int i=0;i<arr.length;i++){ System.out.print(arr[i]+" "); } } }
测试结果:
package task; public class Three2 { public static void quickSort(int[] num,int low, int high) { int left = low; int right = high; int key = num[low]; int temp; //快速排序 while (left < right) { //从有边往左边找,把大的数放到右边 while (left < right && num[right] >= key) { right--; } if (left < right) { temp = num[right]; num[right] = num[left]; num[left] = temp; left++; } //从左边往右边找 while (left < right && num[left] <= key) { left++; } if(left < right) { temp = num[right]; num[right] = num[left]; num[left] = temp; right--; } } if(left > low) { quickSort(num,low,left - 1); } if(right < high) { quickSort(num,right + 1,num.length - 1); } } public static void printArr(int[] num) { for(int i = 0;i < num.length;i++) { System.out.print(num[i] + " "); } } public static void main(String[] args) { int[] num = new int[] {5,89,46,3,445,122,30,18}; quickSort(num,0,num.length - 1); printArr(num); } }
4.判断101到200之间有多少个素数,并输出所有的素数。
package task; public class Three { public static void main(String[] args){ int num = 0; for(int i= 101;i<200;i++){ for(int j = 2;j<i;j++){ int m = i%j; if(j == i-1){ ++num; System.out.print(i+" "); }else if(m==0){ break; } } } System.out.println(); System.out.println("101到200之间素数共有"+num+"个"); } }
测试结果:
5.输入一行字符,分别统计出其中英文字母,空格,数字和其他字符的个数。
package task; import java.util.*; public class Four { public static void main(String[] args) { int abcCount=0;//英文字母个数 int spaceCount=0;//空格键个数 int numCount=0;//数字个数 int otherCount=0;//其他字符个数 System.out.println("请输入一串任意字符:"); Scanner scan=new Scanner(System.in); String str=scan.nextLine(); char[] ch = str.toCharArray(); for(int i=0;i<ch.length;i++){ if(Character.isLetter(ch[i])){ //判断是否字母 abcCount++; } else if(Character.isDigit(ch[i])){ //判断是否数字 numCount++; } else if(Character.isSpaceChar(ch[i])){ //判断是否空格键 spaceCount++; } else{ //以上都不是则认为是其他字符 otherCount++; } } System.out.println("字母个数:"+abcCount); System.out.println("数字个数:"+numCount); System.out.println("空格个数:"+spaceCount); System.out.println("其他字符个数:"+otherCount); } }
测试结果:
6.企业发放的奖金根据利润提成,利润低于或等于10万元时,奖金可提10%;利润高于10万元时低于20万元时,低于10万元的部分按10%提成,高于十万元的部分可提成7.5%;20万到40万之间时,高于20万元的部分可提成5%;40万到60万之间时高于40万元的部分可提成3%;60万到100万之间时,高于60万的部分可提成1.5%,超过100万元的部分按1%提成,从键盘输入当月利润,求应发放奖金总数。
package task; import java.util.*; public class Six { //计算提成的方法 public static double getResult (double profit) { double commission = 0; if(profit<=10){ commission = profit*0.1; }else if(profit>10 && profit<=20){ commission = (profit -10)*0.075+getResult(10); }else if(profit>20 && profit<=40){ commission = (profit -20)*0.05+getResult(20); }else if(profit>40 && profit<=60){ commission = (profit -40)*0.03+getResult(40); }else if(profit>60 && profit<=100){ commission = (profit -60)*0.015+getResult(60); }else if(profit>100){ commission = (profit -100)*0.01+getResult(100); } return commission; } public static void main(String [] args){ System.out.print("请输入当月利润:"); Scanner sc = new Scanner(System.in); double profit = sc.nextDouble(); double commission = getResult(profit); System.out.println("当月可提成"+commission); } }
测试结果:
7.分别定义用户类,订单类,产品类,其中订单类至少要具有下订单的行为(入参为一个产品名称),产品类中至少有一个成员变量为产品名称,至少有一个方法用来获取产品的名称,用户类中持有一个产品类型的成员变量,持有一个订单类型的 并且调用成员变量订单的对象成员变量。在我们的用户类中定义main函数,当执行的时候,构造一个用户类的对象,并且通过手段可以拿到产品成员变量中的产品名称,,进行下单,最后输出下单成功。
package task; public class Eight { public static void main(String[] args){ User user=new User(); user.productName="酸奶"; user.productprice=8.5; user.getProductName(); user.getProductprice(); user.order.getOrder(); } } class Product{ String productName; double productprice; String productType; void getProductName(){ System.out.println("产品:"+productName); } void getProductprice(){ System.out.println("价格:"+productprice); } } class Order extends Product{ String orderName; String orderTime; void getOrder(){ System.out.println("恭喜,下单成功!"); } } class User extends Order{ String userName; Order order=new Order(); }
相关文章推荐
- 黑马程序员----【javaSE基础】代码练习--重载与重写
- 黑马程序员----【javaSE基础】代码练习--内部类
- 03_JAVASE_语法基础下.ppt_练习
- JAVASE基础 Item -- IO流综合练习
- JAVASE基础-day23(递归练习)
- 基础练习 杨辉三角形
- 基础练习 十六进制转八进制
- 蓝桥杯 基础练习 特殊的数字
- 快速学习javaSE基础4---面向对象的编程
- JavaSe基础(6)-- 方法
- JavaSE阶段1_Java基础
- linux基础练习(六)
- js基础for循环练习
- JavaSE练习2
- python基础练习之几个简单的游戏
- 【算法学习笔记】08.数据结构基础 二叉树初步练习1
- JavaSE 拾遗(2)——JavaSE 面向对象程序设计语言基础(2)...变量
- 基础练习 FJ的字符串
- JAVASE基础(一)
- 蓝桥杯 基础练习 回文数 JAVA