使用Struts实现简单的登陆
2017-09-28 17:30
387 查看
引入jar包
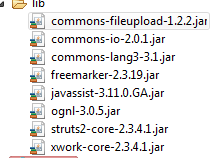
文件结构图如下:
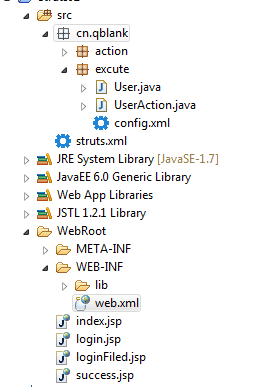
首先在web.xml文件中设置核心过滤器
<?xml version="1.0" encoding="UTF-8"?>
<web-app version="3.0"
xmlns="http://java.sun.com/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd"> <!-- 引入struts核心过滤器 -->
<filter>
<filter-name>struts2</filter-name>
<filter-class>org.apache.struts2.dispatcher.ng.filter.StrutsPrepareAndExecuteFilter</filter-class>
</filter>
<filter-mapping>
<filter-name>struts2</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
</web-app>
然后创建一个实体类对象User,省略getter和setter方法
private String userName;
private String pwd;
然后写对应的UserAction类继承ActionSupport方法
package cn.qblank.excute;
import java.util.Map;
import com.opensymphony.xwork2.ActionContext;
import com.opensymphony.xwork2.ActionSupport;
@SuppressWarnings("serial")
public class UserAction extends ActionSupport {
private User user = new User();
public void setUser(User user) {
this.user = user;
}
public User getUser() {
return user;
}
//登陆
public String login() {
if ("admin".equals(user.getUserName()) && "123".equals(user.getPwd())) {
//将数据存入域中
ActionContext ac = ActionContext.getContext();
//获取request的Map集合
Map<String, Object> request = ac.getContextMap();
//获取session的Map集合
@SuppressWarnings("unused")
Map<String, Object> session = ac.getSession();
//获取ServletContext的Map集合
@SuppressWarn
4000
ings("unused")
Map<String, Object> application = ac.getApplication();
//request域中存储数据
request.put("req_username", user.getUserName());
request.put("req_pwd", user.getPwd());
return "success";
}else{
return "loginFiled";
}
}
}
在同级目录中写下config.xml进行配置
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE struts PUBLIC
"-//Apache Software Foundation//DTD Struts Configuration 2.0//EN"
"http://struts.apache.org/dtds/struts-2.0.dtd">
<struts>
<!--
package 定义一个包。 包作用,管理action。
(通常,一个业务模板用一个包)
name 包的名字; 包名不能重复;
extends 当前包继承自哪个包
在struts中,包一定要继承struts-default
struts-default在struts-default.xml中定的包
abstract
表示当前包为抽象包; 抽象包中不能有action的定义,否则运行时期报错
abstract=true 只有当当前的包被其他包继承时候才用!
如:
<package name="basePackage" extends="struts-default" abstract="true"></package>
<package name="user" extends="basePackage">
namespace 名称空间,默认为"/"
作为路径的一部分
访问路径= http://localhost:8080/项目/名称空间/ActionName action 配置请求路径与Action类的映射关系
name 请求路径名称
class 请求处理的aciton类的全名
method 请求处理方法
result
name action处理方法返回值
type 跳转的结果类型
标签体中指定跳转的页面
-->
<!-- 子配置文件 -->
<package name="user" extends="struts-default" namespace="/">
<!-- 动作 -->
<action name="login" class="cn.qblank.excute.UserAction" method="login">
<result name="success">/success.jsp</result>
<result name="loginFiled">/loginFiled.jsp</result>
</action>
</package>
</struts>
在主配置文件中引入config.xml文件
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE struts PUBLIC
"-//Apache Software Foundation//DTD Struts Configuration 2.0//EN"
"http://struts.apache.org/dtds/struts-2.0.dtd">
<struts>
<!-- 总配置文件 负责引入各个子配置文件 -->
<include file="cn/qblank/excute/config.xml"></include>
</struts>
对应的登陆界面login.jsp
<%@ page language="java" import="java.util.*" pageEncoding="UTF-8"%>
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
<html>
<head>
<title>login</title>
<meta http-equiv="pragma" content="no-cache">
<meta http-equiv="cache-control" content="no-cache">
<meta http-equiv="expires" content="0">
<meta http-equiv="keywords" content="keyword1,keyword2,keyword3">
<meta http-equiv="description" content="This is my page">
</head>
<body>
<form action="${pageContext.request.contextPath }/login" name="frmLogin" method="post">
用户名: <input type="text" name="user.userName"> <br/>
密码: <input type="text" name="user.pwd"> <br/>
<input type="submit" value="登陆"> <br/>
</form>
</body>
</html>
登陆成功界面success.jsp
<%@ page language="java" import="java.util.*" pageEncoding="UTF-8"%>
<%
String path = request.getContextPath();
String basePath = request.getScheme()+"://"+request.getServerName()+":"+request.getServerPort()+path+"/";
%>
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
<html>
<head>
<base href="<%=basePath%>">
<title>访问成功</title>
<meta http-equiv="pragma" content="no-cache">
<meta http-equiv="cache-control" content="no-cache">
<meta http-equiv="expires" content="0">
<meta http-equiv="keywords" content="keyword1,keyword2,keyword3">
<meta http-equiv="description" content="This is my page">
</head>
<body>
<h3>访问成功</h3>
欢迎 ${user.userName}来到本页面!!!
</body>
</html>
登陆失败界面loginFiled.jsp
<%@ page language="java" import="java.util.*" pageEncoding="UTF-8"%>
<%
String path = request.getContextPath();
String basePath = request.getScheme()+"://"+request.getServerName()+":"+request.getServerPort()+path+"/";
%>
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
<html>
<head>
<base href="<%=basePath%>">
<title>My JSP 'loginFiled.jsp' starting page</title>
<meta http-equiv="pragma" content="no-cache">
<meta http-equiv="cache-control" content="no-cache">
<meta http-equiv="expires" content="0">
<meta http-equiv="keywords" content="keyword1,keyword2,keyword3">
<meta http-equiv="description" content="This is my page">
</head>
<body>
<h3>登陆失败</h3>
</body>
</html>
登陆成功:
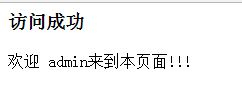
登陆失败:
文件结构图如下:
首先在web.xml文件中设置核心过滤器
<?xml version="1.0" encoding="UTF-8"?>
<web-app version="3.0"
xmlns="http://java.sun.com/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd"> <!-- 引入struts核心过滤器 -->
<filter>
<filter-name>struts2</filter-name>
<filter-class>org.apache.struts2.dispatcher.ng.filter.StrutsPrepareAndExecuteFilter</filter-class>
</filter>
<filter-mapping>
<filter-name>struts2</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
</web-app>
然后创建一个实体类对象User,省略getter和setter方法
private String userName;
private String pwd;
然后写对应的UserAction类继承ActionSupport方法
package cn.qblank.excute;
import java.util.Map;
import com.opensymphony.xwork2.ActionContext;
import com.opensymphony.xwork2.ActionSupport;
@SuppressWarnings("serial")
public class UserAction extends ActionSupport {
private User user = new User();
public void setUser(User user) {
this.user = user;
}
public User getUser() {
return user;
}
//登陆
public String login() {
if ("admin".equals(user.getUserName()) && "123".equals(user.getPwd())) {
//将数据存入域中
ActionContext ac = ActionContext.getContext();
//获取request的Map集合
Map<String, Object> request = ac.getContextMap();
//获取session的Map集合
@SuppressWarnings("unused")
Map<String, Object> session = ac.getSession();
//获取ServletContext的Map集合
@SuppressWarn
4000
ings("unused")
Map<String, Object> application = ac.getApplication();
//request域中存储数据
request.put("req_username", user.getUserName());
request.put("req_pwd", user.getPwd());
return "success";
}else{
return "loginFiled";
}
}
}
在同级目录中写下config.xml进行配置
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE struts PUBLIC
"-//Apache Software Foundation//DTD Struts Configuration 2.0//EN"
"http://struts.apache.org/dtds/struts-2.0.dtd">
<struts>
<!--
package 定义一个包。 包作用,管理action。
(通常,一个业务模板用一个包)
name 包的名字; 包名不能重复;
extends 当前包继承自哪个包
在struts中,包一定要继承struts-default
struts-default在struts-default.xml中定的包
abstract
表示当前包为抽象包; 抽象包中不能有action的定义,否则运行时期报错
abstract=true 只有当当前的包被其他包继承时候才用!
如:
<package name="basePackage" extends="struts-default" abstract="true"></package>
<package name="user" extends="basePackage">
namespace 名称空间,默认为"/"
作为路径的一部分
访问路径= http://localhost:8080/项目/名称空间/ActionName action 配置请求路径与Action类的映射关系
name 请求路径名称
class 请求处理的aciton类的全名
method 请求处理方法
result
name action处理方法返回值
type 跳转的结果类型
标签体中指定跳转的页面
-->
<!-- 子配置文件 -->
<package name="user" extends="struts-default" namespace="/">
<!-- 动作 -->
<action name="login" class="cn.qblank.excute.UserAction" method="login">
<result name="success">/success.jsp</result>
<result name="loginFiled">/loginFiled.jsp</result>
</action>
</package>
</struts>
在主配置文件中引入config.xml文件
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE struts PUBLIC
"-//Apache Software Foundation//DTD Struts Configuration 2.0//EN"
"http://struts.apache.org/dtds/struts-2.0.dtd">
<struts>
<!-- 总配置文件 负责引入各个子配置文件 -->
<include file="cn/qblank/excute/config.xml"></include>
</struts>
对应的登陆界面login.jsp
<%@ page language="java" import="java.util.*" pageEncoding="UTF-8"%>
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
<html>
<head>
<title>login</title>
<meta http-equiv="pragma" content="no-cache">
<meta http-equiv="cache-control" content="no-cache">
<meta http-equiv="expires" content="0">
<meta http-equiv="keywords" content="keyword1,keyword2,keyword3">
<meta http-equiv="description" content="This is my page">
</head>
<body>
<form action="${pageContext.request.contextPath }/login" name="frmLogin" method="post">
用户名: <input type="text" name="user.userName"> <br/>
密码: <input type="text" name="user.pwd"> <br/>
<input type="submit" value="登陆"> <br/>
</form>
</body>
</html>
登陆成功界面success.jsp
<%@ page language="java" import="java.util.*" pageEncoding="UTF-8"%>
<%
String path = request.getContextPath();
String basePath = request.getScheme()+"://"+request.getServerName()+":"+request.getServerPort()+path+"/";
%>
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
<html>
<head>
<base href="<%=basePath%>">
<title>访问成功</title>
<meta http-equiv="pragma" content="no-cache">
<meta http-equiv="cache-control" content="no-cache">
<meta http-equiv="expires" content="0">
<meta http-equiv="keywords" content="keyword1,keyword2,keyword3">
<meta http-equiv="description" content="This is my page">
</head>
<body>
<h3>访问成功</h3>
欢迎 ${user.userName}来到本页面!!!
</body>
</html>
登陆失败界面loginFiled.jsp
<%@ page language="java" import="java.util.*" pageEncoding="UTF-8"%>
<%
String path = request.getContextPath();
String basePath = request.getScheme()+"://"+request.getServerName()+":"+request.getServerPort()+path+"/";
%>
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
<html>
<head>
<base href="<%=basePath%>">
<title>My JSP 'loginFiled.jsp' starting page</title>
<meta http-equiv="pragma" content="no-cache">
<meta http-equiv="cache-control" content="no-cache">
<meta http-equiv="expires" content="0">
<meta http-equiv="keywords" content="keyword1,keyword2,keyword3">
<meta http-equiv="description" content="This is my page">
</head>
<body>
<h3>登陆失败</h3>
</body>
</html>
登陆成功:
登陆失败:
相关文章推荐
- 使用Struts2和jQuery EasyUI实现简单CRUD系统(八)——Struts与EasyUI使用JSON进行交互
- 使用三层架构实现简单的MVC登陆操作
- 使用Python登陆QQ邮箱发送垃圾邮件 简单实现
- 运用Struts1框架来简单实现登陆跳转
- SpringMVC(13):使用springmvc优化订单管理系统的示例(登陆和注销的简单实现)
- java使用JFrame连接mysql数据库,实现简单登陆。
- 使用Struts2和jQuery EasyUI实现简单CRUD系统(八)——Struts与EasyUI使用JSON进行交互
- j2ee使用struts实现用户的登陆及注册
- ASP.NET使用Cookie简单实现记住登陆状态功能
- Android 使用 intent 实现简单登陆页面
- 使用线性布局实现简单登陆界面的实例
- ASP.NET使用Cookie简单实现记住登陆状态功能
- 使用三层架构实现简单的MVC登陆操作!并实现基本的增删改查功能!!
- Eclipse使用(七)—— 使用Eclipse创建JavaWeb项目并使用JDBC连接数据库实现简单的登陆注册功能
- JavaWeb_使用Servlet + HttpSession实现简单用户登陆
- j2ee使用struts实现用户的登陆及注册
- 【MVC】使用Servlet 作为控制器实现一个简单的登陆验证
- .NET中使用datagrid实现的简单分页效果
- 使用异或进行简单的密码加密(JAVA实现)
- 使用 HttpWebRequest 轻松实现站外提交(可用于自动登陆,自动网上投票等)