AngularJS+Bootstrap 分页功能实现,同时支持模糊查询分页
2017-09-11 20:18
1171 查看
因为项目的需要需要实现页面的分页功能,需要用到AngularJS+Bootstrap来实现页面的分页功能,同时支持模糊查询分页。
思考了一下需要确定要完成以下步骤:
一、完成页面的页码数显示
1、获取总条数
2、根据页数获取对应当前显示的页数
二、按照页码数点击读取对应的数据
1、传入对应的起始条数来获取对应的数据
三、获取模糊查询结果的页码数显示
1、获取模糊查询的总条数
2、获取对应可以显示的页数
四、按照页码数和模糊查询来获取对应数据
1、对应传入起始的获取条数和模糊查询的字符来获取数据
以下是javascript代码:
对应的html代码:
<div class="modal fade" tabindex="-1" role="dialog" id="myModal">
<div class="modal-dialog" role="document">
<div class="modal-content">
<div class="modal-header"&g
4000
t;
<button type="button" class="close" data-dismiss="modal" aria-label="Close"><span aria-hidden="true">×</span></button>
<h4 class="modal-title">公司资料</h4>
</div>
<div class="modal-body">
<div class="table_box jlp-scroll-table">
<div class="searchBox has-feedback">
<input type="text" class="form-control" placeholder="公司名称、层级" ng-model="searchText" />
<span class="glyphicon glyphicon-search form-control-feedback"></span>
</div>
<table class="table table-hover">
<thead class="jlp-table-thcolor">
<tr>
<th>#</th>
<th>公司名称</th>
<th>公司层级</th>
</tr>
</thead>
<tbody>
<tr ng-dblclick="showRowData($index)" ng-class="{selectStyle:$index==selectRow}" ng-repeat="item in allListData">
<th scope="row">{{item.number}}</th>
<td ng-bind='item.CorpName'></td>
<td ng-bind='item.CorpLevel'></td>
</tr>
</tbody>
</table>
</div>
</div>
<div class="modal-footer">
<center>
<nav aria-label="Page navigation">
<ul class="pagination" style="margin: 0px;">
<li>
<a aria-label="Previous" ng-click="changePage(1)">
<span aria-hidden="true">« 第1页</span>
</a>
</li>
<li ng-repeat="d in PageData" ng-class="{true: 'active', false: ''}[d.active]">
<a ng-click="changePage(d.page)">{{d.page}}</a>
</li>
<li>
<a aria-label="Next" ng-click="changePage(PageLength)">
<span aria-hidden="true">第{{PageLength}}页 »</span>
</a>
</li>
</ul>
</nav>
</center>
</div>
</div><!-- /.modal-content -->
</div><!-- /.modal-dialog -->
</div>
效果:
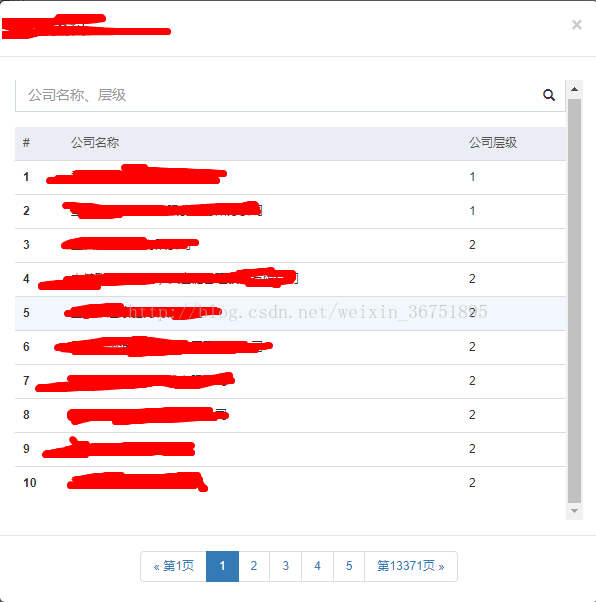
思考了一下需要确定要完成以下步骤:
一、完成页面的页码数显示
1、获取总条数
2、根据页数获取对应当前显示的页数
二、按照页码数点击读取对应的数据
1、传入对应的起始条数来获取对应的数据
三、获取模糊查询结果的页码数显示
1、获取模糊查询的总条数
2、获取对应可以显示的页数
四、按照页码数和模糊查询来获取对应数据
1、对应传入起始的获取条数和模糊查询的字符来获取数据
以下是javascript代码:
/* *传入数据获取对应的页面的数据 *@param {int} star 开始读取的点 *@param {int} size 需要读取的条数 */ $scope.getCopInfo = function (star,size) { //这个是封装的ajax函数的调用,详细的过程不表,传入一个回掉函数 Utils.AjaxInterfaceService('getCorporations', function (res) { if (res.Flag) { var dat = res.MsgInfo; var list = star+1; $scope.allListData = angular.copy(dat); //遍历添加页面显示条数 for (var i = 0; i < $scope.allListData.length; i++) { $scope.allListData[i].number = list++; } } else { alert(res.ErrInfo); } //传入对应的用户id,需要安全过滤用户权限,后台详细不表 }, { userid: userInfo.USERID, pageStar: star, pageSize: size }, "get", false) } /* *传入数据获取对应的页面的数据,获取模糊查询的结果 *@param {int} star 开始读取的点 *@param {int} size 需要读取的条数 */ $scope.getCopInfoLike = function (star, size) { Utils.AjaxInterfaceService('getCorporationsLike', function (res) { if (res.Flag) { var dat = res.MsgInfo; var list = star + 1; $scope.allListData = angular.copy(dat); //遍历添加条数 for (var i = 0; i < $scope.allListData.length; i++) { $scope.allListData[i].number = list++; } } else { alert(res.ErrInfo); } //封装了具体输入框绑定的数据,进行模糊查询,后台详细sql不表 }, { userid: userInfo.USERID, pageStar: star, pageSize: size, likeData: $scope.searchText }, "get", false) } //初始化显示的总页数 $scope.PageLength = null; /* *获取查询的结果的总条数,然后按每页十条来显示数据 */ $scope.getCopLength = function () { Utils.AjaxInterfaceService('getCorporationsLength', function (res) { if (res.Flag) { var dat = angular.copy(res.MsgInfo); $scope.PageLength = Math.ceil(parseFloat(dat[0].info) / 10); } else { alert(res.ErrInfo); } }, { userid: userInfo.USERID }, "get", false) } /* *获取模糊查询的结果的总条数,然后按每页十条来显示数据 */ $scope.getCopLengthLike = function () { Utils.AjaxInterfaceService('getCorporationsLengthLike', function (res) { if (res.Flag) { var dat = angular.copy(res.MsgInfo); $scope.PageLength = Math.ceil(parseFloat(dat[0].info) / 10); } else { alert(res.ErrInfo); } }, { userid: userInfo.USERID, likeData: $scope.searchText }, "get", false) } //需要显示的页码数据初始化 $scope.PageData = []; /* *点击页数改变,完成相应的页数的改变 *@param {int} page 传入需要显示的页数 */ $scope.changePage = function (page) { //初始化显示的条数,和sql读取的真实起始 var size = 10; var star = (size * (page - 1)) - 1; if (star < 0) { star = 0; } //获取对应页数的数据.要是有模糊查询的词就要模糊查询 if ($scope.searchText) {//模糊查询 $scope.getCopInfoLike(star, 10); } else {//正常查询 $scope.getCopInfo(star, 10); } //获取对应页数的数据,显示对应5个页码 var forStar = 0; $scope.PageData = []; if ($scope.PageLength < 5) { forStar = $scope.PageLength; } else { forStar = 5; } //选中的页数显示在中间,要是当前的页数不能中间显示也需要判断 var starpage = page - 2; var endPage = page + 2; if (endPage > $scope.PageLength) { starpage -= endPage - $scope.PageLength; } if (starpage <= 0) { starpage = 1; } //循环出对应的页码数 for (; forStar > 0; forStar--) { var namber = starpage++; var actve = false; //对应的页码选中当前页 if (namber === page) { actve = true; } var pageInfo = { page: namber, active: actve }; $scope.PageData.push(pageInfo); } } /* *点击加载显示对应的弹出框的绑定事件 *这个函数完成正常的数据展示 */ $scope.showCopInfo = function () { //清空搜索数据 $scope.searchText = ""; //默认正常查询 $scope.getCopLength(); $scope.changePage(1); $('#myModal').modal('show'); } //搜索框数据初始化 $scope.searchText = ""; /* *监听搜索框绑定的数据,要是数据变化查询对应的数据 *缺点就是只要有改变就会请求,可以使用change时间来触发,或者使用按钮的click事件来触发 *这个函数完成模糊搜索的实现 */ $scope.$watch('searchText', function () { //传入数据查询模糊查询结果 if ($scope.searchText) { $scope.getCopLengthLike(); $scope.changePage(1); } });
对应的html代码:
<div class="modal fade" tabindex="-1" role="dialog" id="myModal">
<div class="modal-dialog" role="document">
<div class="modal-content">
<div class="modal-header"&g
4000
t;
<button type="button" class="close" data-dismiss="modal" aria-label="Close"><span aria-hidden="true">×</span></button>
<h4 class="modal-title">公司资料</h4>
</div>
<div class="modal-body">
<div class="table_box jlp-scroll-table">
<div class="searchBox has-feedback">
<input type="text" class="form-control" placeholder="公司名称、层级" ng-model="searchText" />
<span class="glyphicon glyphicon-search form-control-feedback"></span>
</div>
<table class="table table-hover">
<thead class="jlp-table-thcolor">
<tr>
<th>#</th>
<th>公司名称</th>
<th>公司层级</th>
</tr>
</thead>
<tbody>
<tr ng-dblclick="showRowData($index)" ng-class="{selectStyle:$index==selectRow}" ng-repeat="item in allListData">
<th scope="row">{{item.number}}</th>
<td ng-bind='item.CorpName'></td>
<td ng-bind='item.CorpLevel'></td>
</tr>
</tbody>
</table>
</div>
</div>
<div class="modal-footer">
<center>
<nav aria-label="Page navigation">
<ul class="pagination" style="margin: 0px;">
<li>
<a aria-label="Previous" ng-click="changePage(1)">
<span aria-hidden="true">« 第1页</span>
</a>
</li>
<li ng-repeat="d in PageData" ng-class="{true: 'active', false: ''}[d.active]">
<a ng-click="changePage(d.page)">{{d.page}}</a>
</li>
<li>
<a aria-label="Next" ng-click="changePage(PageLength)">
<span aria-hidden="true">第{{PageLength}}页 »</span>
</a>
</li>
</ul>
</nav>
</center>
</div>
</div><!-- /.modal-content -->
</div><!-- /.modal-dialog -->
</div>
效果:
相关文章推荐
- 基于HTML5 Bootstrap搭建的后台模板,分页,模糊查询已经全部JS实现,无需编码,嵌入数据即可开发,内置8款皮肤,欧美风格,非常好用!
- Angular.js与Bootstrap相结合实现表格分页代码
- Angular实现的自定义模糊查询、排序及三角箭头标注功能示例
- Angularjs+Bootstrap实现分页指令
- jQuery基于xml格式数据实现模糊查询及分页功能的方法
- html+css+angularjs 实现商品库存信息管理页面 可删除/批量删除/可模糊查询/可升序降序
- AngularJS+bootstrap实现动态选择商品功能示例
- html+css+angularjs 实现商品库存信息管理页面 可删除/批量删除/可模糊查询/可升序降序
- Angular实现的内置过滤器orderBy排序与模糊查询功能示例
- 基于SpringMVC+Bootstrap+DataTables实现表格服务端分页、模糊查询
- Vue.js实现分页查询功能
- Angular.js+Bootstrap实现表格分页
- Bootstrap + AngularJS 实现简单的数据过滤字符查找功能
- Angular+Bootstrap+Spring Boot实现分页功能实例代码
- Angular.js+Bootstrap实现表格分页
- 使用bootstrap-select实现下拉菜单的模糊搜索,支持单选和多选功能
- Hibernate分页的实现(支持模糊查询)
- bootstrap typeahead实现模糊查询功能
- mybatis分页及模糊查询功能实现
- Angular.js与Bootstrap相结合实现表格分页代码