jdk中队列的实现--阻塞队列和无阻塞队列
2017-08-28 12:10
295 查看
队列有先进先出FIFO和后进先出LIFO两种模式
队列一般都采用链表实现
无阻塞队列:
阻塞队列:
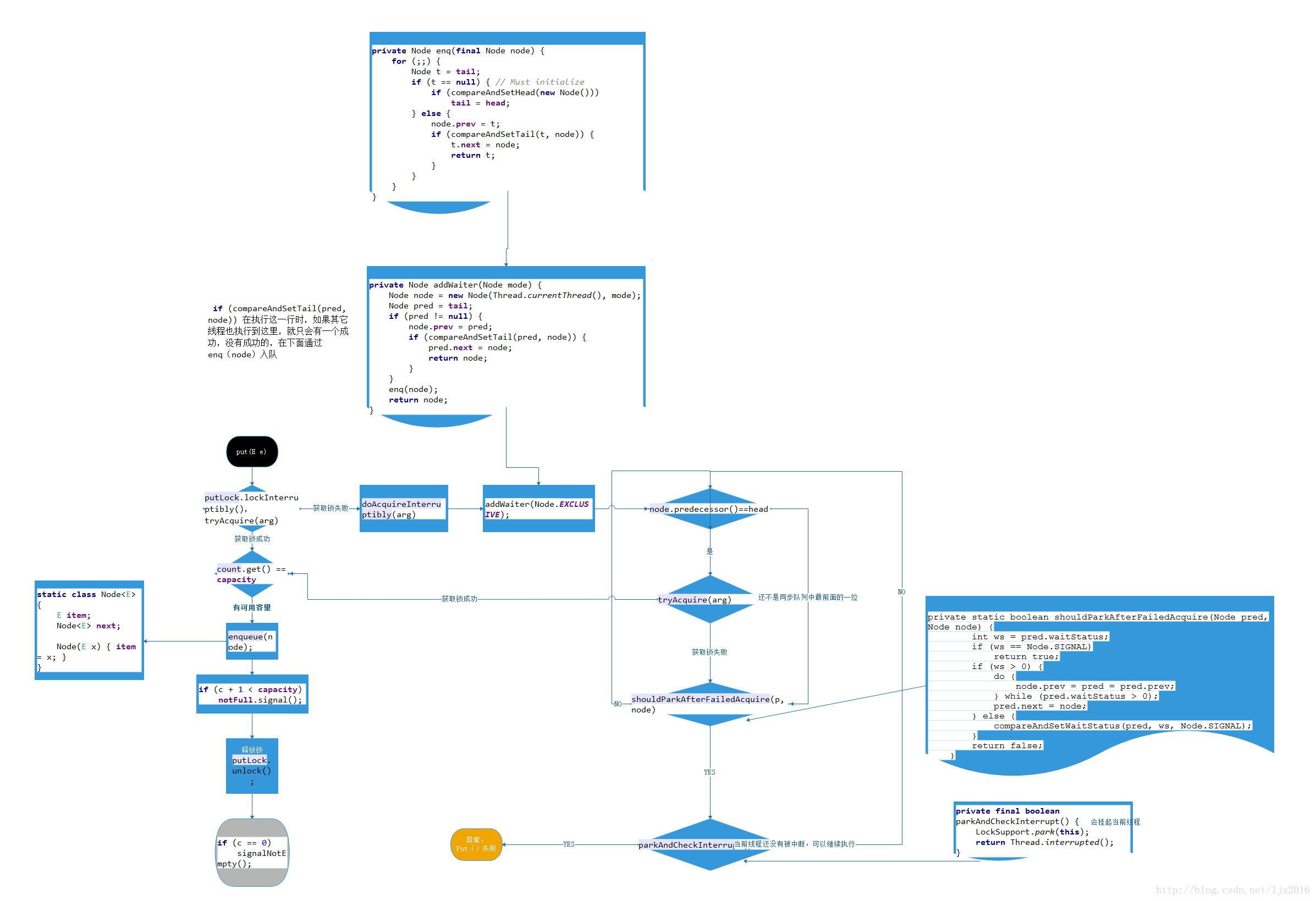
队列一般都采用链表实现
无阻塞队列:
class QueueElement<T>{//队列节点 QueueElement<T> next=null; QueueElement<T> prev=null; T obj=null; QueueElement(T var1){ this.obj=var1; } } public class Queue<T>{//这是一个双向链表,是一个FIFO模型的队列 int length=0; QueueElement<T> head=null;//头部 QueueElement<T> tail=null;//尾部 public Queue(){} public synchronized void enqueue(T var1){//入队 QueueElement var2=new QueueElement(var1); if(this.head==null){//队列为空 this.head=var2; this.tail=var2; this.length=1; } else { var2.next=this.head; this.head.prev=var2; this.head=var2; this.length++; } this.notify();//唤醒其它线程 } public T dequeue() throws InterruptedException(){//出队 return this.dequeue(0L); } public synchronized T dequeue(long var1){ while(this.tail==null){//队列为空时,等待 this.wait(var1); } QueueElement var3=this.tail; this.tail=var3.prev; if(this.tail==null){//队列只有一个元素 this.head=null; } else { this.tail.next=null;//释放这个节点 } --this.length; return var3.obj; } public synchronized boolean isEmpty(){ return this.tail==null; } public final synchronized Enumeration<T> elements(){ return new FIFOQueueElemerator(this);//获取一个枚举器 } public final synchronized Enumeration<T> reverseElements(){ return new LIFOQueueElemerator(this);//获取一个后进先出的枚举器 } } final class FIFOQueueEnumerator<T> implements Enumeration<T>{ Queue<T> queue; QueueElement<T> cursor; FIFOQueueEnumerator(Queue queue){ this.queue=queue; this.cursor=queue.tail; } public boolean hasMoreElements(){ return this.cursor!=null; } public T nextElement(){ Queue queue=this.queue; synchronized(this.queue){ if(this.cursor!=null){ QueueElement qe=this.cursor; this.cursor=this.cursor.prev; return qe.obj; } } } } final class LIFOQueueEnumerator<T> implements Enumeration<T> { Queue<T> queue; QueueElement<T> cursor; LIFOQueueEnumerator(Queue<T> var1) { this.queue = var1; this.cursor = var1.head; } public boolean hasMoreElements() { return this.cursor != null; } public T nextElement() { Queue var1 = this.queue; synchronized(this.queue) { if(this.cursor != null) { QueueElement var2 = this.cursor; this.cursor = this.cursor.next; return var2.obj; } } throw new NoSuchElementException("LIFOQueueEnumerator"); } }
阻塞队列:
static class Node<E> { E item; Node<E> next; Node(E x) { item = x; } } public void put(E e) throws InterruptException{//入队 if(e ==null){ throw new NullPointerException(); } int c=-1; Node<E> node =new Node(e); final ReentrantLock putLock=this.putLock;//private final ReentrantLock putLock = new ReentrantLock();默认是非公平锁 final AtomicInteger count=this.count;//线程安全的一个类,用于计数 putLock.lockInterruptibly();//获取锁,如果获取失败,则线程中断,等待机会被唤醒。 try{ while(count.get()==capacity){//private final int capacity;是一个成员变量,表示队列容量,默认为Integer.MAX_VALUE; notFull.await();//当队列满了时,线程等。。待 } enqueuw(node);//入队 c=count.getAndIncrement();//队列中元素数量自增1; if(c+1<capacity){ notFull.signal();//还有可用容量 } }finally{ putLock.unlock();//释放锁 } if(c==0) signalNotEmpty(); } 对于ReentrantLock,在并发编程中另外一文中详解。
相关文章推荐
- JDK源码分析之主要阻塞队列实现类ArrayBlockingQueue -- java消息队列/java并发编程/阻塞队列
- java消费者生产者模式及JDK之阻塞队列LinkedBlockingQueue实现
- JDK源码分析之主要阻塞队列实现类LinkedBlockingQueue
- JDK源码分析之主要阻塞队列实现类PriorityBlockingQueue
- Java阻塞队列线程集控制的实现
- Linux C++ 使用condition实现阻塞队列的方法
- Java阻塞队列的实现
- 聊聊高并发(十四)理解Java中的管程,条件队列,Condition以及实现一个阻塞队列
- Java阻塞队列BlockingQueue实现生产者消费者-只有代码-不讲原理
- notify和notifyAll的区别及阻塞队列模拟实现
- 手写阻塞队列(Condition实现)
- 什么是阻塞队列? 如何使用阻塞队列来实现生产者-消费者模型?
- Java阻塞队列ArrayBlockingQueue和LinkedBlockingQueue实现原理分析(还没看,先马)
- 基于阻塞队列实现消费者和生产者
- Java并发编程-阻塞队列(BlockingQueue)的实现原理
- javaweb简单实现前台请求的非阻塞消息队列(ConcurrentLinkedQueue)
- Java阻塞队列线程集控制的实现
- c++ 阻塞队列的实现
- java阻塞队列的实现
- 使用阻塞队列实现生产者和消费者问题