Java设计模式之--装饰模式
2017-08-26 11:24
489 查看
装饰模式(Decorator),动态地给一个对象添加一些额外的职责,就增加功能来说,装饰模式比生成子类更为灵活。
何时用:当系统需要新功能的时候,是向旧的类种添加新的代码,这些新加的代码通常装饰了原有类的核心职责和或主要行为。而这些新加入的东西仅仅是为了满足一些只在某种特定情况下才会执行的特殊行为的需要。而装饰模式提供了很好的解决方案,把每个要装饰的功能放在单独的类中,并让这个类包装它所要装饰的对象。因此,当需要执行特殊行为时,客户代码就可以在运行时根据需要有选择地、按顺序地使用装饰功能包装对象了。这样有效的把类的核心职责和装饰功能从类中搬移取出,简化原来的类。
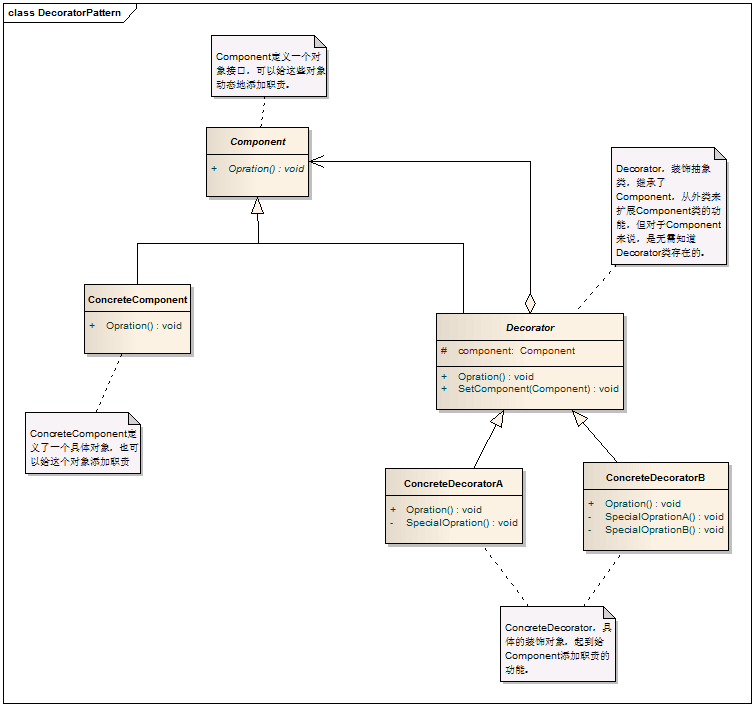
[csharp]
view plain
copy
using System;
using System.Collections.Generic;
using System.Text;
namespace 装饰模式
{
class Program
{
static void Main(string[] args)
{
Person xc = new Person("小菜");//定义的具体对象ConcreteComponent,为其添加职责,例:Person类,为其搭配服饰
Console.WriteLine("\n第一种装扮:");
Sneakers pqx = new Sneakers();
BigTrouser kk = new BigTrouser();
TShirts dtx = new TShirts();
//装饰的过程:
pqx.Decorate(xc);//先用ConcreteComponent实例化Person类为xc,
kk.Decorate(pqx);//按顺序再用Sneaker鞋来搭配BigTrouser垮裤
dtx.Decorate(kk);//最后用搭配过的垮裤BigTrouser来搭配TShirtsT恤
dtx.Show();//显示第一种
Console.WriteLine("\n第二种装扮:");
LeatherShoes px = new LeatherShoes();
Tie ld = new Tie();
Suit xz = new Suit();
px.Decorate(xc);
ld.Decorate(px);
xz.Decorate(ld);
xz.Show();
Console.WriteLine("\n第三种装扮:");
Sneakers pqx2 = new Sneakers();
LeatherShoes px2 = new LeatherShoes();
BigTrouser kk2 = new BigTrouser();
Tie ld2 = new Tie();
pqx2.Decorate(xc);
px2.Decorate(pqx);
kk2.Decorate(px2);
ld2.Decorate(kk2);
ld2.Show();
Console.Read();
}
}
class Person //定义的具体对象ConcreteComponent,为其添加职责,例:Person类,为其搭配服饰
{
public Person()
{ }
private string name;
public Person(string name)
{
this.name = name;
}
public virtual void Show()
{
Console.WriteLine("装扮的{0}", name);
}
}
class Finery : Person //装饰抽象类(Decorator),继承抽象父类,并玩去取代Component
{
protected Person component;
//打扮
public void Decorate(Person component)
{
this.component = component;
}
public override void Show()
{
if (component != null)
{
component.Show();
}
}
}
class TShirts : Finery //具体的装饰对象
{
pub
bb05
lic override void Show()
{
Console.Write("大T恤 ");
base.Show(); //调用基类Finery上已被其他方法重写的方法
}
}
class BigTrouser : Finery
{
public override void Show()
{
Console.Write("垮裤 ");
base.Show();
}
}
class Sneakers : Finery
{
public override void Show()
{
Console.Write("破球鞋 ");
base.Show();
}
}
class Suit : Finery
{
public override void Show()
{
Console.Write("西装 ");
base.Show();
}
}
class Tie : Finery
{
public override void Show()
{
Console.Write("领带 ");
base.Show();
}
}
class LeatherShoes : Finery
{
public override void Show()
{
Console.Write("皮鞋 ");
base.Show();
}
}
}
注意如果只有一个ConcreteComponent类而没有抽象的Component类,那么Decorator类可以是ConcreteComponent的一个子类。同理,如果只有一个ConcreteDecorator类,那么就没必要建立一个单独的Decorator类,而可以把Decorator类和ConcreteDecorator类的责任合并为一个类。
何时用:当系统需要新功能的时候,是向旧的类种添加新的代码,这些新加的代码通常装饰了原有类的核心职责和或主要行为。而这些新加入的东西仅仅是为了满足一些只在某种特定情况下才会执行的特殊行为的需要。而装饰模式提供了很好的解决方案,把每个要装饰的功能放在单独的类中,并让这个类包装它所要装饰的对象。因此,当需要执行特殊行为时,客户代码就可以在运行时根据需要有选择地、按顺序地使用装饰功能包装对象了。这样有效的把类的核心职责和装饰功能从类中搬移取出,简化原来的类。
[csharp]
view plain
copy
using System;
using System.Collections.Generic;
using System.Text;
namespace 装饰模式
{
class Program
{
static void Main(string[] args)
{
Person xc = new Person("小菜");//定义的具体对象ConcreteComponent,为其添加职责,例:Person类,为其搭配服饰
Console.WriteLine("\n第一种装扮:");
Sneakers pqx = new Sneakers();
BigTrouser kk = new BigTrouser();
TShirts dtx = new TShirts();
//装饰的过程:
pqx.Decorate(xc);//先用ConcreteComponent实例化Person类为xc,
kk.Decorate(pqx);//按顺序再用Sneaker鞋来搭配BigTrouser垮裤
dtx.Decorate(kk);//最后用搭配过的垮裤BigTrouser来搭配TShirtsT恤
dtx.Show();//显示第一种
Console.WriteLine("\n第二种装扮:");
LeatherShoes px = new LeatherShoes();
Tie ld = new Tie();
Suit xz = new Suit();
px.Decorate(xc);
ld.Decorate(px);
xz.Decorate(ld);
xz.Show();
Console.WriteLine("\n第三种装扮:");
Sneakers pqx2 = new Sneakers();
LeatherShoes px2 = new LeatherShoes();
BigTrouser kk2 = new BigTrouser();
Tie ld2 = new Tie();
pqx2.Decorate(xc);
px2.Decorate(pqx);
kk2.Decorate(px2);
ld2.Decorate(kk2);
ld2.Show();
Console.Read();
}
}
class Person //定义的具体对象ConcreteComponent,为其添加职责,例:Person类,为其搭配服饰
{
public Person()
{ }
private string name;
public Person(string name)
{
this.name = name;
}
public virtual void Show()
{
Console.WriteLine("装扮的{0}", name);
}
}
class Finery : Person //装饰抽象类(Decorator),继承抽象父类,并玩去取代Component
{
protected Person component;
//打扮
public void Decorate(Person component)
{
this.component = component;
}
public override void Show()
{
if (component != null)
{
component.Show();
}
}
}
class TShirts : Finery //具体的装饰对象
{
pub
bb05
lic override void Show()
{
Console.Write("大T恤 ");
base.Show(); //调用基类Finery上已被其他方法重写的方法
}
}
class BigTrouser : Finery
{
public override void Show()
{
Console.Write("垮裤 ");
base.Show();
}
}
class Sneakers : Finery
{
public override void Show()
{
Console.Write("破球鞋 ");
base.Show();
}
}
class Suit : Finery
{
public override void Show()
{
Console.Write("西装 ");
base.Show();
}
}
class Tie : Finery
{
public override void Show()
{
Console.Write("领带 ");
base.Show();
}
}
class LeatherShoes : Finery
{
public override void Show()
{
Console.Write("皮鞋 ");
base.Show();
}
}
}
注意如果只有一个ConcreteComponent类而没有抽象的Component类,那么Decorator类可以是ConcreteComponent的一个子类。同理,如果只有一个ConcreteDecorator类,那么就没必要建立一个单独的Decorator类,而可以把Decorator类和ConcreteDecorator类的责任合并为一个类。
相关文章推荐
- Java设计模式之------装饰模式
- (三)Java设计模式--装饰器模式
- JAVA IO之装饰器模式(Head first 设计模式之装饰器模式)
- (九)Java设计模式之装饰模式
- JAVA设计模式-装饰模式
- java设计模式之装饰模式(decorator)
- java中的设计模式一 装饰模式
- java设计模式(九)--装饰器模式
- Java基础知识IO流(装饰设计模式)
- java之装饰设计模式和继承的简单区别
- java设计模式-装饰模式
- Java设计模式十九:装饰模式(Decorator Pattern)
- Java设计模式之装饰模式
- Java设计模式—装饰模式
- java--IO流缓冲区,装饰设计模式
- IO_打印流_装饰设计模式JAVA161-162
- java-设计模式(结构型)-【装饰模式】
- JAVA设计模式之装饰模式
- java设计模式之装饰模式(装饰器模式/装饰者模式)
- java二十三种设计模式------(一)装饰模式 装饰类VS子类继承