Java Swing中添加图标、对话框、分割线、信息提示、面板的使用
2017-08-24 12:30
585 查看
(一).JLabel、JButton、JFrame、JPane等可以设置图标,接下来代码演示一下
输出
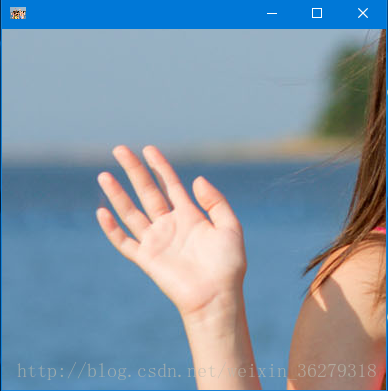
(二).对话框常用方法的使用
showMessageDialog(Component parentComponent, Object message)
showMessageDialog(Component parentComponent, Object message, String title, int messageType)
showConfirmDialog(Component parentComponent, Object message)
showInputDialog(Component parentComponent, Object message)
(三)代码演示
1.比如登录账号后的提示
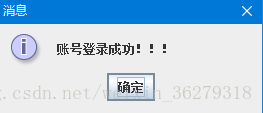
2.比如登录有误的提示
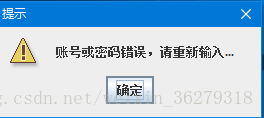
3.输入对话框
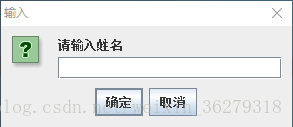
4.确认对话框的使用
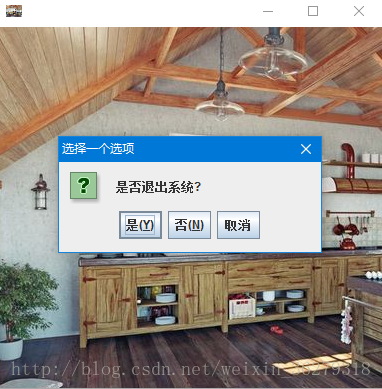
(四)分割线在菜单项中的使用
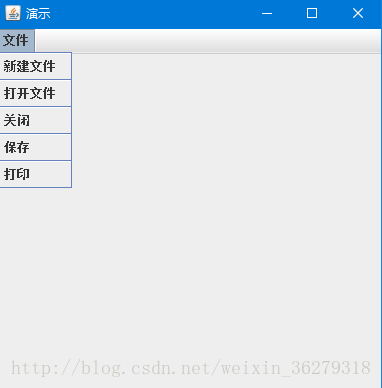
(五)信息提示
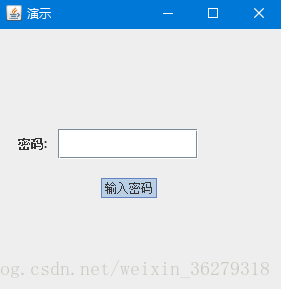
(六)面板的使用
面板可以添加组件,设置组件在面板上的位置和大小,设置好以后再将面板添加到JFrame中。
输出:
package com.Swing; import java.awt.*; import javax.swing.ImageIcon; import javax.swing.JFrame; import javax.swing.JLabel; class IconDemo { IconDemo() { JFrame f = new JFrame(); //使用ImageIO也可以获取image Image image = Toolkit.getDefaultToolkit().getImage("D:\\1.jpg"); JLabel label=new JLabel(); label.setSize(390,390); label.setIcon( new ImageIcon(image)); f.add(label); f.setIconImage(image); f.setLayout(null); f.setSize(400, 400); f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); f.setVisible(true); } public static void main(String args[]) { new IconDemo(); } }
输出
(二).对话框常用方法的使用
showMessageDialog(Component parentComponent, Object message)
showMessageDialog(Component parentComponent, Object message, String title, int messageType)
showConfirmDialog(Component parentComponent, Object message)
showInputDialog(Component parentComponent, Object message)
(三)代码演示
1.比如登录账号后的提示
package com.Swing; import javax.swing.*; public class OptionPaneDemo1 { JFrame f; OptionPaneDemo1(){ f=new JFrame(); JOptionPane.showMessageDialog(f,"账号登录成功!!!"); } public static void main(String[] args) { new OptionPaneDemo1(); } }
2.比如登录有误的提示
package com.Swing; import javax.swing.*; public class OptionPaneDemo2{ JFrame f; OptionPaneDemo1() { f = new JFrame(); JOptionPane.showMessageDialog(f, "账号或密码错误,请重新输入...", "Alert", JOptionPane.WARNING_MESSAGE); } public static void main(String[] args) { new OptionPaneDemo1(); } }
3.输入对话框
package com.Swing; import javax.swing.*; public class OptionPaneDemo3 { JFrame f; OptionPaneDemo1() { f = new JFrame(); String name = JOptionPane.showInputDialog(f,"请输入姓名"); } public static void main(String[] args) { new OptionPaneDemo1(); } }
4.确认对话框的使用
package com.Swing; import javax.imageio.ImageIO; import javax.swing.*; import java.awt.Image; import java.awt.event.*; import java.io.File; import java.io.IOException; public class OptionPaneDemo4 extends WindowAdapter { JFrame f; JLabel label; ImageIO imageIO; Image image; OptionPaneDemo1() throws IOException { f = new JFrame(); label=new JLabel(); label.setSize(400, 400); image=ImageIO.read(new File("D:\\4.jpg")); label.setIcon(new ImageIcon(image)); f.setIconImage(image); f.add(label); f.addWindowListener(this); f.setSize(400, 400); f.setLayout(null); f.setLocationRelativeTo(null); f.setDefaultCloseOperation(JFrame.DO_NOTHING_ON_CLOSE); f.setVisible(true); } public void windowClosing(WindowEvent e) { int a = JOptionPane.showConfirmDialog(f, "是否退出系统?"); if (a == JOptionPane.YES_OPTION) { f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); } } public static void main(String[] args) throws IOException { new OptionPaneDemo1(); } }
(四)分割线在菜单项中的使用
package com.Swing; import javax.swing.*; class SeparatorDemo { JMenu menu, submenu; JMenuItem i1, i2, i3, i4, i5; SeparatorDemo() { JFrame f = new JFrame("演示"); JMenuBar mb = new JMenuBar(); menu = new JMenu("文件"); i1 = new JMenuItem("新建文件"); i2 = new JMenuItem("打开文件"); i3 = new JMenuItem("关闭"); i4 = new JMenuItem("保存"); i5 = new JMenuItem("打印"); menu.add(i1); menu.addSeparator(); menu.add(i2); menu.addSeparator(); menu.add(i3); menu.addSeparator(); menu.add(i4); menu.addSeparator(); menu.add(i5); mb.add(menu); f.setJMenuBar(mb); f.setSize(400, 400); f.setLocationRelativeTo(null); f.setLayout(null); f.setVisible(true); } public static void main(String args[]) { new SeparatorDemo(); } }
(五)信息提示
package com.Swing; import javax.swing.*; public class TooltipsDemo { public static void main(String[] args) { JFrame f = new JFrame("演示"); JPasswordField value = new JPasswordField(); value.setBounds(60, 100, 140, 30); value.setToolTipText("输入密码"); JLabel l1 = new JLabel("密码:"); l1.setBounds(20, 100, 80, 30); f.add(value); f.add(l1); f.setSize(300, 300); f.setLocationRelativeTo(null); f.setLayout(null); f.setVisible(true); } }
(六)面板的使用
面板可以添加组件,设置组件在面板上的位置和大小,设置好以后再将面板添加到JFrame中。
package com.Swing; import java.awt.*; import javax.swing.*; public class JPaneDemo { JPaneDemo() { JFrame f = new JFrame("演示"); JPanel panel = new JPanel(); panel.setBounds(90, 80, 200, 200); panel.setBackground(Color.gray); JButton b1 = new JButton("登录"); b1.setBounds(50, 100, 80, 30); b1.setBackground(Color.GREEN); JButton b2 = new JButton("退出"); b2.setBounds(100, 100, 80, 30); b2.setBackground(Color.RED); panel.add(b1); panel.add(b2); f.add(panel); f.setSize(400, 400); f.setLocationRelativeTo(null); f.setLayout(null); f.setVisible(true); } public static void main(String args[]) { new JPaneDemo(); } }
输出:
相关文章推荐
- 在基于对话框的MFC中添加工具栏以及工具栏提示信息并改变图标支持256色
- VS2010 MFC中在对话框上添加工具栏以及工具栏提示信息并改变图标支持256色
- java swing 之 JScrollPane(滚动面板)的使用
- vs2008 对话框上的工具栏添加提示信息
- 使用dialog插件弹出提示和确定信息对话框8-8
- 使用dialog插件弹出提示和确定信息对话框8-8
- java.swing 容器与面板之间的关系以及正确使用方法
- Java swing 之 标签控件 和 图标控件的使用
- Java swing创建按钮并添加到面板中
- jquery validate插件的参考使用 checkbox、radio提示信息如何添加
- Sqoop-1.4.6 mysql数据导出到HDFS提示;注: java使用或覆盖了已过时的 API。关详细信息, 请使用 -Xlint:deprecation 重新编译。
- 现在已经获得了软件的大小、图标等信息,想得到软件的使用频率,就像控制面板中添加或删除程序那样
- 使用HashSet和TreeSet存储多个商品信息,遍历并输出;其中商品属性:编号,名称,单价,出版社;要求向其中添加多个相同的商品,验证集合中元素的唯一性。 提示:向HashSet中添加自定义
- Java中给按钮等控件添加图标(Swing)
- 使用pyinstaller 2.1将python打包并添加版本信息和图标
- java swing 之 JScrollPane(滚动面板)的使用
- JAVA学习Swing章节标签JLabel中图标的使用
- MFC对话框添加菜单、状态栏,并在状态栏显示菜单提示信息
- Java Swing Ribbon(Flamingo)的使用06:添加JComponent组件
- JAVA学习Swing章节标签JLabel中图标的使用