java hashmap的一些分析记录
2017-08-19 19:24
288 查看
最近朋友去面试被问了些hashmap相关的问题,hashmap的初始容量啊,什么操作最耗时等,之前看过hashmap的源码,正好这里也在总结下。
主要围绕下面几个点:
HashMap是由数组+链表(jdk8 升级为红黑树)结构实现
HashMap 在第一次put的时候才会去分配内存(ArrayList也是在第一次add的时候)
HashMap 默认数组大小是16
HashMap 每次扩容之后大小都为2的倍数
HashMap在达到容量阀值(threshold=capacity*loadFactor)时候会进行扩容
HashMap如何进行扩容
代码参考jdk1.7
HashMap 在第一次put的时候才会去分配内存(ArrayList也是在第一次add的时候)
这是HashMap的构造函数,看一看到这里只是初始化了loadFactor跟threshold 这两个参数,而在上一个分析中第一次put元素的时候会执行inflateTable函数来初始化数组:
在调用addEntry添加新元素的方法时,会判定size是否达到了临界值,如果到了则进行数组扩容,回调用resize方法可以看到新的容量为原始容量*2
HashMap如何进行扩容
这段代码是进行数组下标计算的
resize代码如下
JDK1.8中这里会涉及到HashMap涉及很巧妙的一个点。由于 table.length 也就是capacity 肯定是2的N次方,使用 & 位运算意味着只是多了最高位,这样就不用重新计算 index,元素要么在原位置,要么在原位置+ oldCapacity
如果增加的高位为0,resize 后 index 不变,如图所示:
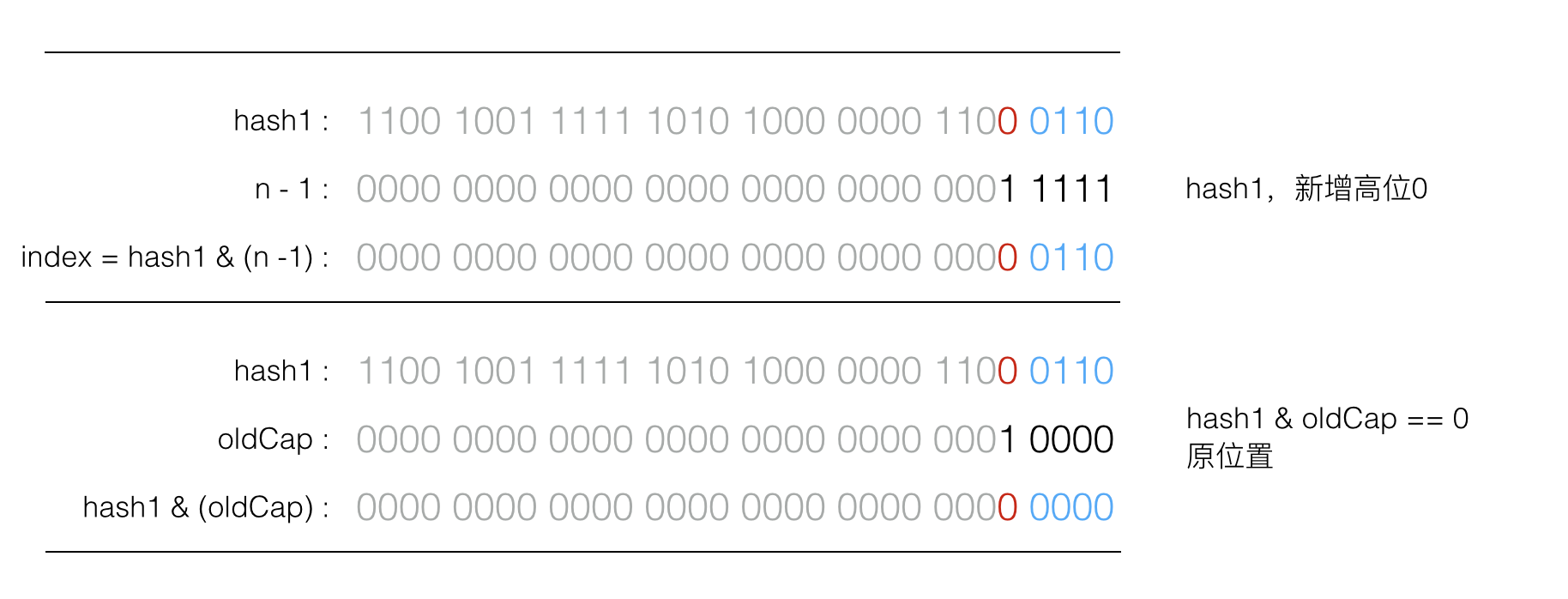
如果增加的高位为1,resize 后 index 增加 oldCap,如图所示:

这个设计的巧妙之处在于,节省了一部分重新计算hash的时间,同时新增的一位为0或1的概率可以认为是均等的,所以在resize 的过程中就将原来碰撞的节点又均匀分布到了两个bucket里。
主要围绕下面几个点:
HashMap是由数组+链表(jdk8 升级为红黑树)结构实现
HashMap 在第一次put的时候才会去分配内存(ArrayList也是在第一次add的时候)
HashMap 默认数组大小是16
HashMap 每次扩容之后大小都为2的倍数
HashMap在达到容量阀值(threshold=capacity*loadFactor)时候会进行扩容
HashMap如何进行扩容
代码参考jdk1.7
HashMap是由数组+链表(jdk8 升级为红黑树)结构实现
这个是再put时候会初始化一个数组,在key hash冲突时候同一bucket加入新value// 初始化数组 private void inflateTable(int toSize) { int capacity = roundUpToPowerOf2(toSize); threshold = (int) Math.min(capacity * loadFactor, MAXIMUM_CAPACITY + 1); table = new Entry[capacity]; initHashSeedAsNeeded(capacity); }
public V put(K key, V value) { if (table == EMPTY_TABLE) { inflateTable(threshold); } // key 为null时候直接put if (key == null) return putForNullKey(value); int hash = hash(key); int i = indexFor(hash, table.length); for (Entry<K,V> e = table[i]; e != null; e = e.next) { Object k; // 在hash值相同&&key equals时候进行值替换 if (e.hash == hash && ((k = e.key) == key || key.equals(k))) { V oldValue = e.value; e.value = value; e.recordAccess(this); return oldValue; } } // 否则添加新value modCount++; addEntry(hash, key, value, i); return null; }
HashMap 在第一次put的时候才会去分配内存(ArrayList也是在第一次add的时候)
public HashMap(int initialCapacity, float loadFactor) { if (initialCapacity < 0) throw new IllegalArgumentException("Illegal initial capacity: " + initialCapacity); if (initialCapacity > MAXIMUM_CAPACITY) initialCapacity = MAXIMUM_CAPACITY; if (loadFactor <= 0 || Float.isNaN(loadFactor)) throw new IllegalArgumentException("Illegal load factor: " + loadFactor); this.loadFactor = loadFactor; threshold = initialCapacity; init(); }
这是HashMap的构造函数,看一看到这里只是初始化了loadFactor跟threshold 这两个参数,而在上一个分析中第一次put元素的时候会执行inflateTable函数来初始化数组:
table = new Entry[capacity];进行内存分配 HashMap 默认数组大小是16 static final int DEFAULT_INITIAL_CAPACITY = 1 << 4; // aka 16 HashMap 每次扩容之后大小都为2的倍数
void addEntry(int hash, K key, V value, int bucketIndex) { if ((size >= threshold) && (null != table[bucketIndex])) { resize(2 * table.length); hash = (null != key) ? hash(key) : 0; bucketIndex = indexFor(hash, table.length); } createEntry(hash, key, value, bucketIndex); }
在调用addEntry添加新元素的方法时,会判定size是否达到了临界值,如果到了则进行数组扩容,回调用resize方法可以看到新的容量为原始容量*2
HashMap如何进行扩容
static int indexFor(int h, int length) { // assert Integer.bitCount(length) == 1 : "length must be a non-zero power of 2"; return h & (length-1); }
这段代码是进行数组下标计算的
resize代码如下
JKD1.7 void resize(int newCapacity) { Entry[] oldTable = table; int oldCapacity = oldTable.length; if (oldCapacity == MAXIMUM_CAPACITY) { threshold = Integer.MAX_VALUE; return; } Entry[] newTable = new Entry[newCapacity]; transfer(newTable, initHashSeedAsNeeded(newCapacity)); table = newTable; threshold = (int)Math.min(newCapacity * loadFactor, MAXIMUM_CAPACITY + 1); } /** * Transfers all entries from current table to newTable. */ void transfer(Entry[] newTable, boolean rehash) { int newCapacity = newTable.length; for (Entry<K,V> e : table) { while(null != e) { Entry<K,V> next = e.next; if (rehash) { e.hash = null == e.key ? 0 : hash(e.key); } int i = indexFor(e.hash, newCapacity); e.next = newTable[i]; newTable[i] = e; e = next; } } }
JDK1.8中这里会涉及到HashMap涉及很巧妙的一个点。由于 table.length 也就是capacity 肯定是2的N次方,使用 & 位运算意味着只是多了最高位,这样就不用重新计算 index,元素要么在原位置,要么在原位置+ oldCapacity
如果增加的高位为0,resize 后 index 不变,如图所示:
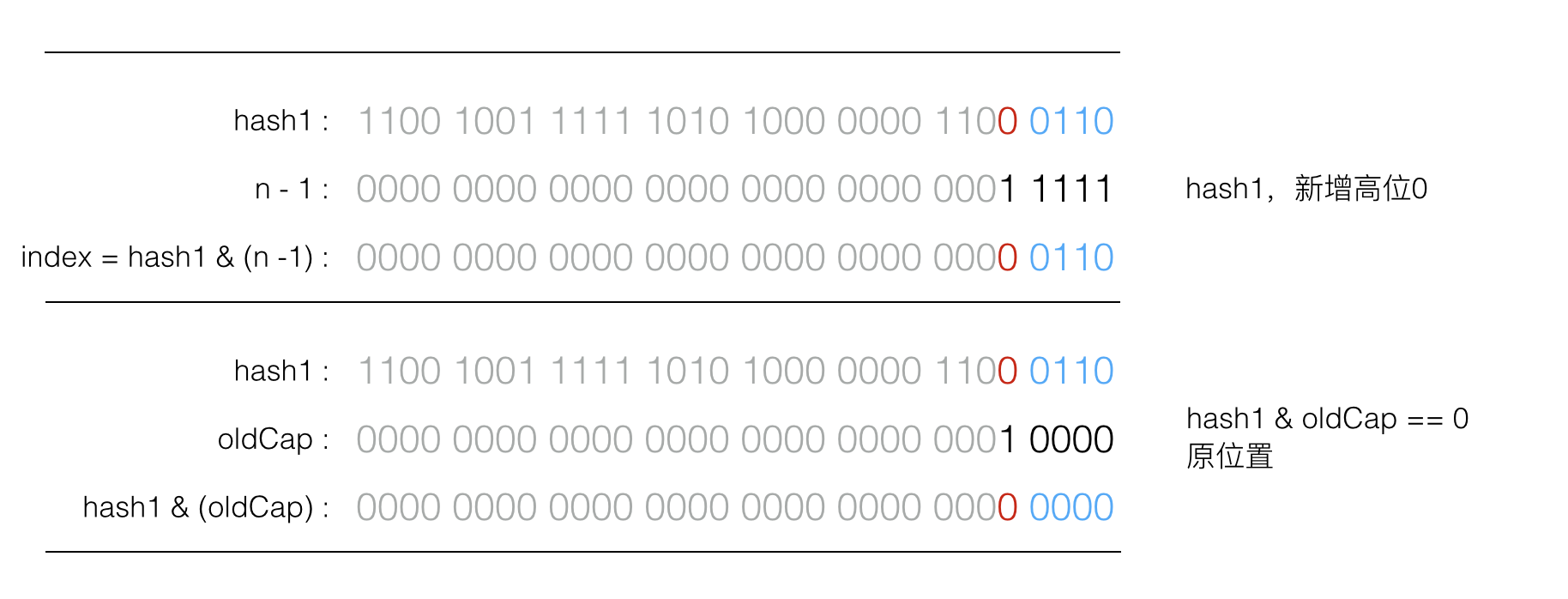
如果增加的高位为1,resize 后 index 增加 oldCap,如图所示:

这个设计的巧妙之处在于,节省了一部分重新计算hash的时间,同时新增的一位为0或1的概率可以认为是均等的,所以在resize 的过程中就将原来碰撞的节点又均匀分布到了两个bucket里。
do { next = e.next; if ((e.hash & oldCap) == 0) { if (loTail == null) loHead = e; else loTail.next = e; loTail = e; } else { if (hiTail == null) hiHead = e; else hiTail.next = e; hiTail = e; } } while ((e = next) != null);
相关文章推荐
- java集合-HashMap的一些记录
- Java Collections---HashMap深度分析与比较(zt)
- Java中对HashMap的深度分析
- Java中对HashMap的深度分析与比较
- (转载)Java语言中对HashMap的深度分析与比较
- Java中对HashMap的深度分析与比较
- Java Collections---HashMap深度分析与比较
- Java中对HashMap的深度分析与比较
- 关于fedora10、ORACLE11、JAVA的一些记录
- Java中对HashMap的深度分析
- Java HashMap集合深度分析
- Java HashMap集合深度分析
- JAVA HASHMAP的原理分析
- Java语言中对HashMap的深度分析与比较
- Java中对HashMap的深度分析
- Java中对HashMap的深度分析
- Java中对HashMap的深度分析
- Java中对HashMap的深度分析与比较Java中对HashMap的深度分析与比较
- Java容器类(List、ArrayList、Vector、map、HashTable、HashMap)的分析比较
- Java中对HashMap的深度分析与比较