java8传递代码例子
2017-08-13 22:57
211 查看
案例:
List中存放Apple实体类,找出color属性为green,重量大于150的apple(Java8实战第一章笔记)
Apple:
package unitOne;
/**
* author : chengshanyunduo
* create : 2017-08-14 0:00
* desc :
**/
public class Apple {
private String color;
private Integer weight;
public String getColor() {
return color;
}
public void setColor(String color) {
this.color = color;
}
public Integer getWeight() {
return weight;
}
public void setWeight(Integer weight) {
this.weight = weight;
}
//筛选color为green的方法
public static boolean isGreenApple(Apple apple){
return "green".equals(apple.getColor());
}
//筛选weight大于150的方法
public static boolean isHeavyApple(Apple apple){
return apple.getWeight() > 150;
}
public interface Predicate<T>{
boolean test(T t);
}
}
运行代码:
package unitOne;
import java.util.ArrayList;
import java.util.LinkedList;
import java.util.List;
/**
* author : chengshanyunduo
* create : 2017-08-14 0:00
* desc :
**/
public class testUnitOne {
public static void main(String[] args){
List<Apple> apples = new LinkedList<Apple>();
for (int i=0; i<9; i++){
apples.add(new Apple());
}
apples.get(0).setColor("green");
apples.get(1).setColor("red");
apples.get(2).setColor("yellow");
apples.get(3).setColor("green");
apples.get(4).setColor("green");
apples.get(5).setColor("red");
apples.get(6).setColor("green");
apples.get(7).setColor("red");
apples.get(8).setColor("green");
apples.get(0).setWeight(120);
apples.get(1).setWeight(150);
apples.get(2).setWeight(160);
apples.get(3).setWeight(110);
apples.get(4).setWeight(170);
apples.get(5).setWeight(100);
apples.get(6).setWeight(110);
apples.get(7).setWeight(190);
apples.get(8).setWeight(200);
//将Apple中的方法作为变量传进方法中
List<Apple> resultColor = filterApples(apples, Apple::isGreenApple);
List<Apple> resultWeight = filterApples(apples, Apple::isHeavyApple);
//检验结果
for (int i=0; i<resultColor.size(); i++){
System.out.println(i + ":" + resultColor.get(i).getColor());
}
for (int i=0; i<resultWeight.size(); i++){
System.out.println(i + ":" + resultWeight.get(i).getWeight());
}
}
/**
* 筛选方法
* @param inventory
* @param p
* @return
*/
static List<Apple> filterApples(List<Apple> inventory, Apple.Predicate<Apple> p){
List<Apple> result = new ArrayList<>();
for (Apple apple : inventory){
if (p.test(apple)){
result.add(apple);
}
}
return result;
}
}
运行结果:
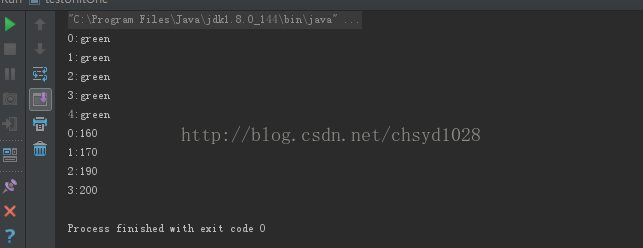
优点:
避免代码重复,方便代码修。
List中存放Apple实体类,找出color属性为green,重量大于150的apple(Java8实战第一章笔记)
Apple:
package unitOne;
/**
* author : chengshanyunduo
* create : 2017-08-14 0:00
* desc :
**/
public class Apple {
private String color;
private Integer weight;
public String getColor() {
return color;
}
public void setColor(String color) {
this.color = color;
}
public Integer getWeight() {
return weight;
}
public void setWeight(Integer weight) {
this.weight = weight;
}
//筛选color为green的方法
public static boolean isGreenApple(Apple apple){
return "green".equals(apple.getColor());
}
//筛选weight大于150的方法
public static boolean isHeavyApple(Apple apple){
return apple.getWeight() > 150;
}
public interface Predicate<T>{
boolean test(T t);
}
}
运行代码:
package unitOne;
import java.util.ArrayList;
import java.util.LinkedList;
import java.util.List;
/**
* author : chengshanyunduo
* create : 2017-08-14 0:00
* desc :
**/
public class testUnitOne {
public static void main(String[] args){
List<Apple> apples = new LinkedList<Apple>();
for (int i=0; i<9; i++){
apples.add(new Apple());
}
apples.get(0).setColor("green");
apples.get(1).setColor("red");
apples.get(2).setColor("yellow");
apples.get(3).setColor("green");
apples.get(4).setColor("green");
apples.get(5).setColor("red");
apples.get(6).setColor("green");
apples.get(7).setColor("red");
apples.get(8).setColor("green");
apples.get(0).setWeight(120);
apples.get(1).setWeight(150);
apples.get(2).setWeight(160);
apples.get(3).setWeight(110);
apples.get(4).setWeight(170);
apples.get(5).setWeight(100);
apples.get(6).setWeight(110);
apples.get(7).setWeight(190);
apples.get(8).setWeight(200);
//将Apple中的方法作为变量传进方法中
List<Apple> resultColor = filterApples(apples, Apple::isGreenApple);
List<Apple> resultWeight = filterApples(apples, Apple::isHeavyApple);
//检验结果
for (int i=0; i<resultColor.size(); i++){
System.out.println(i + ":" + resultColor.get(i).getColor());
}
for (int i=0; i<resultWeight.size(); i++){
System.out.println(i + ":" + resultWeight.get(i).getWeight());
}
}
/**
* 筛选方法
* @param inventory
* @param p
* @return
*/
static List<Apple> filterApples(List<Apple> inventory, Apple.Predicate<Apple> p){
List<Apple> result = new ArrayList<>();
for (Apple apple : inventory){
if (p.test(apple)){
result.add(apple);
}
}
return result;
}
}
运行结果:
优点:
避免代码重复,方便代码修。
相关文章推荐
- Java8--传递代码:一个例子
- Java代码规范--排版,命名---以及一个例子
- java bufferreader 例子代码
- 如下代码中greater10函数调用时实参是如何传递的呢? 代码是MSDN中的一个例子
- 一个使用自定义命名空间的Schema文件,xml文件和castor生成的java代码的例子
- Java8Map示例:一个略复杂的数据映射聚合例子及代码重构
- [转]JSTL 与 JSP 或者 Java 相互传递变量的代码
- Unity3D之打开Activity与调用JAVA代码传递参数
- UML中类之间的关系及其Java代码例子 -转载
- openSSL学习例子(代码含c与java两版本)
- 一个简单的例子,证明JAVA参数是通过值传递
- Java代码规范--排版,命名---以及一个例子
- JNI 调用C++代码 并在C++代码中调用Java传递进来的接口
- Android中WebView载入本地HTML代码并实现Java与JavaScript交互的例子
- java lucene实现近实时搜索及高亮显示的代码例子下载
- Unity3D研究院之打开Activity与调用JAVA代码传递参数(十八)
- 反编译:java代码中含有同步和异常的反编译前后代码对照的一个例子(xiongjy)
- java多线程stop,suspend使用代码实际例子
- java 下载文件功能代码例子
- cocos2dx 之 android java 与 c++ 互相调用 代码(以百度定位为例子)