python网络爬虫实战--重点整理
2017-07-12 11:30
531 查看
第四章--python爬虫常用模块
urllib2.urlopen(url,timeout)请求返回响应,timeout是超时时间设置
使用代理服务器来访问url
有免费的代理服务器,但是使用urlopen的使用很容易出现<urlopen error timed out>,所以需要循环调用urlopen()。
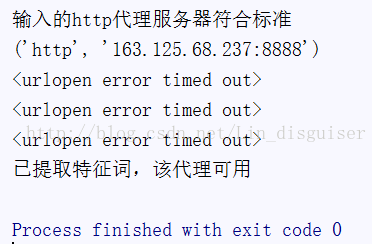
修改header
网站是通过浏览器发送过来的User-Agent的值来确认浏览器的身份的,所以可能有些网站不允许被程序访问,所以我们发送请求时需要修改User-Agent欺骗网站,利用add_header()可以添加头部。同一网站会给不同的浏览器访问不同的内容。
下面程序是用IE和手机版的UC访问有道翻译。
getpass.getuser()
返回当前用户名。这个函数会按顺序检查环境变量LOGNAME, USER, LNAME和USERNAME。返回第一个非空的值。如果检查不到非空的值,模块会尝试导入pwd模块,如果系统支持pwd模块,会返回通过pwd模块获取的用户名,否则报错。
re模块
\A:仅匹配字符串开头,如\Aabc
\Z:仅匹配字符串结尾,如abc\Z
urllib2.urlopen(url,timeout)请求返回响应,timeout是超时时间设置
#! python2.7 #-*- coding:utf-8 -*- import urllib2 def linkBaidu(): url='http://www.baidu.com' try: response=urllib2.urlopen(url,timeout=4) except urllib2.URLError: print("网络地址错误") exit() with open('baiduResponse.txt','w') as fp: #写入文档 fp.write(response.read()) print(response.geturl()) #获取url信息 print(response.getcode()) #返回状态码 print(response.info()) #返回信息 if __name__=='__main__': linkBaidu()
使用代理服务器来访问url
有免费的代理服务器,但是使用urlopen的使用很容易出现<urlopen error timed out>,所以需要循环调用urlopen()。
#-*-coding:utf-8 -*- ''' 测试代理proxy是否有效 ''' import urllib2,re class TestProxy(): def __init__(self,proxy): self.proxy=proxy self.checkProxyFormat(self.proxy) self.url='http://www.baidu.com' self.timeout=4 self.keyword='百度' #在网页返回的数据中查找这个词 self.useProxy(proxy) def checkProxyFormat(self,proxy): try: match=re.compile(r'^http[s]?://[\d]{1,3}.[\d]{1,3}.[\d]{1,3}.[\d]{1,3}:[\d]{1,5}$') match.search(proxy).group() except AttributeError: print("你输入的代理地址格式不正确") exit() flag=1 proxy=proxy.replace('//','') try: protocol=proxy.split(':')[0] ip=proxy.split(':')[1] port=proxy.split(':')[2] except IndexError: print('下标出界') exit() flag=flag and ip.split('.')[0] in map(str,xrange(1,256)) #map对每个数应用到str函数 flag=flag and ip.split('.')[1] in map(str,xrange(256)) flag=flag and ip.split('.')[2] in map(str,xrange(256)) flag=flag and ip.split('.')[3] in map(str,xrange(1,255)) flag=flag and protocol in ['http','https'] flag=flag and port in map(str,xrange(1,65535)) if flag: print('输入的http代理服务器符合标准') else: exit() def useProxy(self,proxy): protocol=proxy.split('//')[0].replace(':','') ip=proxy.split('//')[1] print(protocol,ip) ''' build_opener ()返回的对象具有open()方法,与urlopen()函数的功能相同, install_opener 用来创建(全局)默认opener。这个表示调用urlopen将使用你安装的opener ''' opener=urllib2.build_opener(urllib2.ProxyHandler({protocol:ip})) #protocol:http ip:163.125.68.237:8888 urllib2.install_opener(opener) for i in range(10): try: response=urllib2.urlopen(self.url,timeout=5) break except Exception as e: print(e) str=response.read() if re.search(self.keyword,str): print("已提取特征词,该代理可用") else: print('该代理不可用') if __name__=='__main__': proxy=r'http://163.125.68.237:8888' TestProxy(proxy)
修改header
网站是通过浏览器发送过来的User-Agent的值来确认浏览器的身份的,所以可能有些网站不允许被程序访问,所以我们发送请求时需要修改User-Agent欺骗网站,利用add_header()可以添加头部。同一网站会给不同的浏览器访问不同的内容。
下面程序是用IE和手机版的UC访问有道翻译。
#-*-coding:utf-8 -*- import userAgents import urllib2 class ModifyHeader(): def __init__(self): piua=userAgents.pcUserAgent.get('IE 9.0') muua=userAgents.mobileUserAgent.get('UC standard') print('piua: '+piua) self.url='http://fanyi.youdao.com' self.userAgent(piua,1) self.userAgent(muua,2) def userAgent(self,agent,name): request=urllib2.Request(self.url) request.add_header(agent.split(':')[0],agent.split(':')[1]) response=urllib2.urlopen(request) filename=str(name)+'.html' with open(filename,'w') as fp: fp.write('%s\n\n'%agent) fp.write(response.read()) if __name__=='__main__': ModifyHeader()
getpass.getuser()
返回当前用户名。这个函数会按顺序检查环境变量LOGNAME, USER, LNAME和USERNAME。返回第一个非空的值。如果检查不到非空的值,模块会尝试导入pwd模块,如果系统支持pwd模块,会返回通过pwd模块获取的用户名,否则报错。
re模块
\A:仅匹配字符串开头,如\Aabc
\Z:仅匹配字符串结尾,如abc\Z
相关文章推荐
- Python3 大型网络爬虫实战 002 --- scrapy 爬虫项目的创建及爬虫的创建 --- 实例:爬取百度标题和CSDN博客
- 【Python爬虫9】Python网络爬虫实例实战
- 自己动手,丰衣足食!Python3网络爬虫实战案例
- Python3网络爬虫快速入门实战解析
- Python爬虫入门实战系列(一)--爬取网络小说并存放至txt文件
- Python3 大型网络爬虫实战 — 给 scrapy 爬虫项目设置为防反爬
- Python3网络爬虫:Scrapy入门实战之爬取动态网页图片
- python实战之网络爬虫
- 大数据实战课程第一季Python基础和网络爬虫数据分析
- 【备忘】最新Python3网络爬虫实战案例高清视频教程
- Python3 大型网络爬虫实战 004 — scrapy 大型静态商城网站爬虫项目编写及数据写入数据库实战 — 实战:爬取淘宝
- Python 编写新浪新闻网络爬虫(学习整理)
- Python网络爬虫与信息提取(三):网络爬虫之实战
- Python3网络爬虫快速入门实战解析(一小时入门 Python 3 网络爬虫)
- Python大型网络爬虫项目开发实战
- Python爬虫实战三之实现山东大学无线网络掉线自动重连
- Python实战:网络爬虫都能干什么?
- Python3 大型网络爬虫实战 001 --- 搭建开发环境
- Python网络爬虫实战项目大全!
- Python3网络爬虫实战案例这套教程太全面了,真得收藏一下!