数据结构之栈的定义及python实现
2017-07-10 19:15
302 查看
栈是一个逻辑结构,通俗的讲,是一个有“纪律”的线性表,栈只允许一端进行插入和删除操作,即“先进后出”规则。如下图所示:
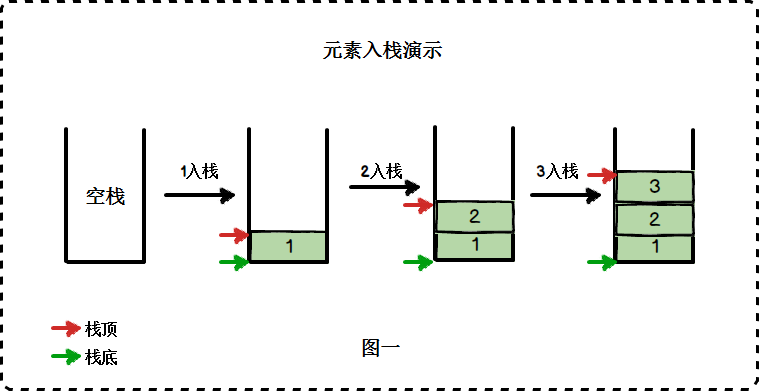
红箭头代表栈顶,即只允许插入和删除的那一端。绿箭头代表栈底,是固定的,即不允许进行插入和删除的另一端。当这个栈内没有元素时,则此时该栈被称为空栈。同样的,栈也有初始化,判定是否为空,插入(进栈),删除(出栈)等操作。下面是关于栈的python实现。
顺序栈实现:
#coding:utf-8
'''
author:xzfreewind
'''
#顺序存储
class Stack(object):
#初始化一个栈
def __init__(self,size):
self.size = size
self.num = 0
self.stack = []
#获取栈的长度
def getSize(self):
return self.num
#输出栈
def outStack(self):
for l in self.stack:
print l
#进栈
def appendStack(self,value):
if self.num >= self.size:
print "the stack is full"
return
else:
self.stack.append(value)
self.num += 1
#出栈
def popStack(self):
if self.isEmpty():
print "the stack is empty,no element can be output"
return
else:
topElement = self.stack[-1]
self.stack.remove(topElement)
return topElement
def isEmpty(self):
if self.num == 0:
return True
else:
return False
def isFull(self):
if self.num == self.size:
return True
else:
return False
链栈实现:
#链式对象建立
class Node(object):
def __init__(self,vaule,q=0):
self.value = vaule
self.next = q
#链式存储
class chainStack(object):
#初始化链栈
def __init__(self):
self.head = 0
#判断栈是否为空
def isEmpty(self):
if self.head == 0:
return True
else:
return False
#计算栈的长度
def getSize(self):
length = 0
p = self.head
while p != 0:
length += 1
p = p.next
return length
#将数组初始化成栈存储
def initStack(self,list):
self.head = Node(list[0])
node = self.head
for i in list[1:]:
p = Node(i)
p.next = node
node = p
#进栈
def insert(self,value):
if self.head == 0: #如果为空栈,直接插入
self.head = Node(value)
else:
node = Node(value)
node.next = self.head
self.head = node
#出栈
def pop(self):
if self.head == 0: #如果为空栈,提示栈为空
print "the stack is empty"
return
else:
l = self.head.value
self.head = self.head.next
return l
#输出栈
def outStack(self):
if self.head == 0: #如果为空栈,提示栈为空
print "the stack is empty"
return
p = self.head
while p != 0:
print p.value
p = p.next
红箭头代表栈顶,即只允许插入和删除的那一端。绿箭头代表栈底,是固定的,即不允许进行插入和删除的另一端。当这个栈内没有元素时,则此时该栈被称为空栈。同样的,栈也有初始化,判定是否为空,插入(进栈),删除(出栈)等操作。下面是关于栈的python实现。
顺序栈实现:
#coding:utf-8
'''
author:xzfreewind
'''
#顺序存储
class Stack(object):
#初始化一个栈
def __init__(self,size):
self.size = size
self.num = 0
self.stack = []
#获取栈的长度
def getSize(self):
return self.num
#输出栈
def outStack(self):
for l in self.stack:
print l
#进栈
def appendStack(self,value):
if self.num >= self.size:
print "the stack is full"
return
else:
self.stack.append(value)
self.num += 1
#出栈
def popStack(self):
if self.isEmpty():
print "the stack is empty,no element can be output"
return
else:
topElement = self.stack[-1]
self.stack.remove(topElement)
return topElement
def isEmpty(self):
if self.num == 0:
return True
else:
return False
def isFull(self):
if self.num == self.size:
return True
else:
return False
链栈实现:
#链式对象建立
class Node(object):
def __init__(self,vaule,q=0):
self.value = vaule
self.next = q
#链式存储
class chainStack(object):
#初始化链栈
def __init__(self):
self.head = 0
#判断栈是否为空
def isEmpty(self):
if self.head == 0:
return True
else:
return False
#计算栈的长度
def getSize(self):
length = 0
p = self.head
while p != 0:
length += 1
p = p.next
return length
#将数组初始化成栈存储
def initStack(self,list):
self.head = Node(list[0])
node = self.head
for i in list[1:]:
p = Node(i)
p.next = node
node = p
#进栈
def insert(self,value):
if self.head == 0: #如果为空栈,直接插入
self.head = Node(value)
else:
node = Node(value)
node.next = self.head
self.head = node
#出栈
def pop(self):
if self.head == 0: #如果为空栈,提示栈为空
print "the stack is empty"
return
else:
l = self.head.value
self.head = self.head.next
return l
#输出栈
def outStack(self):
if self.head == 0: #如果为空栈,提示栈为空
print "the stack is empty"
return
p = self.head
while p != 0:
print p.value
p = p.next
相关文章推荐
- C++和python利用struct结构传输二进制数据实现
- 打印python的ctype定义的结构中的数据
- Python数据结构之单链表实现
- 常用查找数据结构及算法(Python实现)
- c++ 数据结构 用单链表实现多项式的类定义
- 详解常用查找数据结构及算法(Python实现)
- python数据结构与算法 4栈的实现
- 详解常用查找数据结构及算法(Python实现)
- Python 数据结构与算法——二叉搜索树的实现
- 数据结构之单链表及python实现
- Python实现常用的逻辑数据结构
- 数据结构顺序表定义和实现
- 数据结构之队列11循环队列定义及实现
- python——数据结构之单链表的实现
- 常用查找数据结构及算法(Python实现)
- python 数据结构之顺序列表的实现
- python 数据结构之单链表的实现
- 数据结构(5)--栈的定义以及相关操作的实现
- ZH奶酪:【数据结构与算法】基础排序算法总结与Python实现
- 数据结构——用python实现排序算法