spring初级入门一(Spring介绍+启动Spring容器+Spring容器创建对象)
2017-07-10 15:56
555 查看
一、Spring介绍
1、概念
开源
分层
轻量级
一站式(full-stack)
简化企业级开发框架
2、特点
(1)简化开发
spring对一些常见API(比如JDBC)做封装,使用这些封装后的代码会大大简化
(如:使用springjdbc来访问数据库,就不用考虑如何获取连接和关闭连接)
(2)管理对象
spring管理对象之间的依赖关系,维护起来方便
(3)集成其他框架
spring将一些框架进行集成,方便使用框架
(如:spring集成mybatis,这样mybatis操作起来简单方便)
3、特征
(1)控制反转IoC:依赖注入
(2)面向切面AOP:分离业务逻辑与系统级服务
(3)容器:管理对象的配置和生命周期
二、Spring容器
1、概念
Spring容器---是Spring框架中的一个核心模块
用来管理对象
2、Spring容器类型有2种
(1)BeanFactory
(2)ApplicationContext:ApplicationContext继承BeanFactory接口,拥有更多企业级方法,推荐使用(本例子采用这种类型)
3、启动Spring容器
步骤:
(1)导包:spring-webmvc 3.2.8.RELEASE(这里随便选的版本)

(2)添加配置文件:applicationContext.xml(应该放置在java Resources项目资源文件中)
位置:
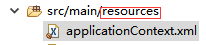
(3)启动Spring容器:java代码中编写
3、Spring容器创建对象(bean的实例化)
(1)无参构造器
创建一个无参构造器的java类(如:Student.java)
(2)静态工厂方法
配置applicatinContext.xml
(3)实例工厂方法
配置applicatinContext.xml
1、概念
开源
分层
轻量级
一站式(full-stack)
简化企业级开发框架
2、特点
(1)简化开发
spring对一些常见API(比如JDBC)做封装,使用这些封装后的代码会大大简化
(如:使用springjdbc来访问数据库,就不用考虑如何获取连接和关闭连接)
(2)管理对象
spring管理对象之间的依赖关系,维护起来方便
(3)集成其他框架
spring将一些框架进行集成,方便使用框架
(如:spring集成mybatis,这样mybatis操作起来简单方便)
3、特征
(1)控制反转IoC:依赖注入
(2)面向切面AOP:分离业务逻辑与系统级服务
(3)容器:管理对象的配置和生命周期
二、Spring容器
1、概念
Spring容器---是Spring框架中的一个核心模块
用来管理对象
2、Spring容器类型有2种
(1)BeanFactory
(2)ApplicationContext:ApplicationContext继承BeanFactory接口,拥有更多企业级方法,推荐使用(本例子采用这种类型)
3、启动Spring容器
步骤:
(1)导包:spring-webmvc 3.2.8.RELEASE(这里随便选的版本)
(2)添加配置文件:applicationContext.xml(应该放置在java Resources项目资源文件中)
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:context="http://www.springframework.org/schema/context" xmlns:jdbc="http://www.springframework.org/schema/jdbc" xmlns:jee="http://www.springframework.org/schema/jee" xmlns:tx="http://www.springframework.org/schema/tx" xmlns:aop="http://www.springframework.org/schema/aop" xmlns:mvc="http://www.springframework.org/schema/mvc" xmlns:util="http://www.springframework.org/schema/util" xmlns:jpa="http://www.springframework.org/schema/data/jpa" xsi:schemaLocation=" http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.2.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-3.2.xsd http://www.springframework.org/schema/jdbc http://www.springframework.org/schema/jdbc/spring-jdbc-3.2.xsd http://www.springframework.org/schema/jee http://www.springframework.org/schema/jee/spring-jee-3.2.xsd http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx-3.2.xsd http://www.springframework.org/schema/data/jpa http://www.springframework.org/schema/data/jpa/spring-jpa-1.3.xsd http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-3.2.xsd http://www.springframework.org/schema/mvc http://www.springframework.org/schema/mvc/spring-mvc-3.2.xsd http://www.springframework.org/schema/util http://www.springframework.org/schema/util/spring-util-3.2.xsd"> </beans>
位置:
(3)启动Spring容器:java代码中编写
import org.springframework.context.ApplicationContext; import org.springframework.context.support.ClassPathXmlApplicationContext; public class FirstSpring { public static void main(String[] args) { /* * 此行代码负责启动spring容器 * a. ApplicationContext:是继承BeanFactory一个接口 * b. ClassPathXmlApplicationContext是 ApplicationContext接口的一个实现类(该类依据类路径去查找配置文件) */ //加载工程classpath下的配置文件(即applicationContext.xml)实例化 ApplicationContext ac = new ClassPathXmlApplicationContext("applicationContext.xml"); }
3、Spring容器创建对象(bean的实例化)
(1)无参构造器
创建一个无参构造器的java类(如:Student.java)
package first; public class Student { //默认构造器 public Student() { System.out.println("打桩Student()"); } }配置applicatinContext.xml
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:context="http://www.springframework.org/schema/context" xmlns:jdbc="http://www.springframework.org/schema/jdbc" xmlns:jee="http://www.springframework.org/schema/jee" xmlns:tx="http://www.springframework.org/schema/tx" xmlns:aop="http://www.springframework.org/schema/aop" xmlns:mvc="http://www.springframework.org/schema/mvc" xmlns:util="http://www.springframework.org/schema/util" xmlns:jpa="http://www.springframework.org/schema/data/jpa" xsi:schemaLocation=" http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.2.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-3.2.xsd http://www.springframework.org/schema/jdbc http://www.springframework.org/schema/jdbc/spring-jdbc-3.2.xsd http://www.springframework.org/schema/jee http://www.springframework.org/schema/jee/spring-jee-3.2.xsd http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx-3.2.xsd http://www.springframework.org/schema/data/jpa http://www.springframework.org/schema/data/jpa/spring-jpa-1.3.xsd http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-3.2.xsd http://www.springframework.org/schema/mvc http://www.springframework.org/schema/mvc/spring-mvc-3.2.xsd http://www.springframework.org/schema/util http://www.springframework.org/schema/util/spring-util-3.2.xsd"> <!-- 1、无参构造器创建对象 id属性:要求唯一。 class属性:需要一个默认的空构造器,要求写类的全限定名。 --> <bean id="stu1" class="first.Student"></bean> </beans>创建一个java实例化验证(使用getBean方法获取)
package first; import org.springframework.context.ApplicationContext; import org.springframework.context.support.ClassPathXmlApplicationContext; public class FirstSpring { public static void main(String[] args) { //启动容器 ApplicationContext ac = new ClassPathXmlApplicationContext("applicationContext.xml"); /* * 此行代码的作用:通过容器获得要创建的对象(注意,getBean方法的第一个参数是bean的id)。 * Student.class:是Student在方法区中对应的class对象。 */ Student stu1 =ac.getBean("stu1",Student.class); System.out.println("无参构造器创建对象:"+stu1); } }
(2)静态工厂方法
配置applicatinContext.xml
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:context="http://www.springframework.org/schema/context" xmlns:jdbc="http://www.springframework.org/schema/jdbc" xmlns:jee="http://www.springframework.org/schema/jee" xmlns:tx="http://www.springframework.org/schema/tx" xmlns:aop="http://www.springframework.org/schema/aop" xmlns:mvc="http://www.springframework.org/schema/mvc" xmlns:util="http://www.springframework.org/schema/util" xmlns:jpa="http://www.springframework.org/schema/data/jpa" xsi:schemaLocation=" http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.2.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-3.2.xsd http://www.springframew 4000 ork.org/schema/jdbc http://www.springframework.org/schema/jdbc/spring-jdbc-3.2.xsd http://www.springframework.org/schema/jee http://www.springframework.org/schema/jee/spring-jee-3.2.xsd http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx-3.2.xsd http://www.springframework.org/schema/data/jpa http://www.springframework.org/schema/data/jpa/spring-jpa-1.3.xsd http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-3.2.xsd http://www.springframework.org/schema/mvc http://www.springframework.org/schema/mvc/spring-mvc-3.2.xsd http://www.springframework.org/schema/util http://www.springframework.org/schema/util/spring-util-3.2.xsd"> <!-- 2、静态工厂方法创建对象 factory-method属性:指定创建bean实例的静态工厂方法。 spring容器会调用该方法来创建对象。 --> <bean id="cal1" class="java.util.Calendar" factory-method="getInstance"></bean> </beans>创建一个java实例化验证(使用getBean方法获取)
package first; import java.util.Calendar; import org.springframework.context.ApplicationContext; import org.springframework.context.support.ClassPathXmlApplicationContext; public class FirstSpring { public static void main(String[] args) { //启动容器 ApplicationContext ac = new ClassPathXmlApplicationContext("applicationContext.xml"); //getBean方法获取 Calendar cal1 =ac.getBean("cal1",Calendar.class); System.out.println("静态工厂方法创建对象:"+cal1); } }
(3)实例工厂方法
配置applicatinContext.xml
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:context="http://www.springframework.org/schema/context" xmlns:jdbc="http://www.springframework.org/schema/jdbc" xmlns:jee="http://www.springframework.org/schema/jee" xmlns:tx="http://www.springframework.org/schema/tx" xmlns:aop="http://www.springframework.org/schema/aop" xmlns:mvc="http://www.springframework.org/schema/mvc" xmlns:util="http://www.springframework.org/schema/util" xmlns:jpa="http://www.springframework.org/schema/data/jpa" xsi:schemaLocation=" http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.2.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-3.2.xsd http://www.springframework.org/schema/jdbc http://www.springframework.org/schema/jdbc/spring-jdbc-3.2.xsd http://www.springframework.org/schema/jee http://www.springframework.org/schema/jee/spring-jee-3.2.xsd http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx-3.2.xsd http://www.springframework.org/schema/data/jpa http://www.springframework.org/schema/data/jpa/spring-jpa-1.3.xsd http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-3.2.xsd http://www.springframework.org/schema/mvc http://www.springframework.org/schema/mvc/spring-mvc-3.2.xsd http://www.springframework.org/schema/util http://www.springframework.org/schema/util/spring-util-3.2.xsd"> <!-- 静态工厂方法配合下面实例工厂方法创建对象 --> <bean id="cal1" class="java.util.Calendar" factory-method="getInstance"></bean> <!-- 3、实例工厂方法创建对象 factory-bean属性:指定要调用的bean的id。 spring容器会调用该bean的方法来创建对象。 --> <bean id="date1" factory-bean="cal1" factory-method="getTime"></bean> <bean id="date2" class="java.util.Date"></bean> </beans>创建一个java实例化验证(使用getBean方法获取)
package first; import java.util.Date; import java.util.Calendar; import org.springframework.context.ApplicationContext; import org.springframework.context.support.ClassPathXmlApplicationContext; public class FirstSpring { public static void main(String[] args) { //启动容器 ApplicationContext ac = new ClassPathXmlApplicationContext("applicationContext.xml"); //getBean方法 //第一种 // Date date1 =ac.getBean("date1",Date.class); // System.out.println(date1); //第二种 Date date2 = ac.getBean("date2", Date.class); System.out.println(date2); } }Maven管理结构
相关文章推荐
- Spring 为了将spring容器和对象的创建在服务器启动时创建(并且只创建一次),将其放在servletContext的监听器内执行。参数名contextConfigLocation哪里找?
- 手动创建(new)对象,使用spring容器中的bean(ServletContext)。
- SpringIOC容器-创建对象
- spring学习教程7-spring容器创建bean对象的方式以及如何处理多个spring配置文件
- spring容器创建对象时抛错解决办法
- spring之IOC容器创建对象
- spring IOC容器创建对象的三种方式
- spring启动后保证创建的对象不被垃圾回收器回收
- Spring容器创建对象的三种方式
- spring容器创建对象的3种方式(bean的实例化)
- spring之IOC容器创建对象
- spring容器创建对象的时机
- Spring的核心之IoC容器创建对象
- Spring创建容器对象
- 两种方式创建docker镜像的启动容器时区别介绍(总结篇)
- spring容器创建对象的3种方式(bean的实例化)
- 云星数据---Scala实战系列(精品版)】:Scala入门教程017-Scala实战源码-Scala 创建对象 以及类的介绍
- spring容器IOC创建对象<二>
- spring容器创建对象的时机
- spring 之脱离容器管理创建的对象进行依赖注入