Spring Boot 使用 Junit
2017-07-06 00:00
267 查看
JUnit是一个Java语言的单元测试框架。它由Kent Beck和Erich Gamma建立,逐渐成为源于Kent Beck的sUnit的xUnit家族中最为成功的一个。 JUnit有它自己的JUnit扩展生态圈。多数Java的开发环境都已经集成了JUnit作为单元测试的工具。
通过观看https://my.oschina.net/sdlvzg/blog/1154281创建项目,再执行以下操作
在pom.xml中引入依赖包
编写代码:
编写Service
编写Controller
测试类编写及运行:
测试Service
在上面类中右击==》Run As ==》1Junit Test ,执行测试类,结果如下:
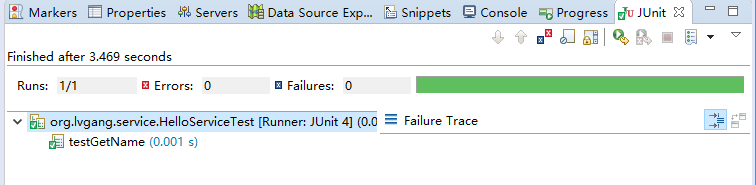
测试Controller(两个方法)
方法一:
在上面类中右击==》Run As ==》1Junit Test ,执行测试类,结果如下:

方法二:
在上面类中右击==》Run As ==》1Junit Test ,执行测试类,结果如下:
通过观看https://my.oschina.net/sdlvzg/blog/1154281创建项目,再执行以下操作
在pom.xml中引入依赖包
<!-- 测试依赖包 --> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> </dependency>
编写代码:
编写Service
package org.lvgang.service; import org.springframework.stereotype.Service; /** * 测试类 * @author Administrator */ @Service public class HelloService { public String getName() { return "hello"; } }
编写Controller
package org.lvgang.controller; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RestController; /** * Hello测试类 * @author Administrator * */ @RestController // 等价于@Controller+@RequstMapping public class HelloController { @RequestMapping("/hello") public String hello(){ return "Hello world test!"; } }
测试类编写及运行:
测试Service
package org.lvgang.service; import org.junit.Assert; import org.junit.Test; import org.junit.runner.RunWith; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.boot.test.context.SpringBootTest; import org.springframework.test.context.junit4.SpringRunner; /** * 测试类 * @author Administrator * */ //SpringBoot1.4版本之前用的是SpringJUnit4ClassRunner.class @RunWith(SpringRunner.class) //SpringBoot1.4版本之前用的是@SpringApplicationConfiguration(classes = Application.class) @SpringBootTest public class HelloServiceTest { @Autowired private HelloService helloService; /** * 测试HelloService类的getName方法 */ @Test public void testGetName() { Assert.assertEquals("hello", helloService.getName()); } }
在上面类中右击==》Run As ==》1Junit Test ,执行测试类,结果如下:
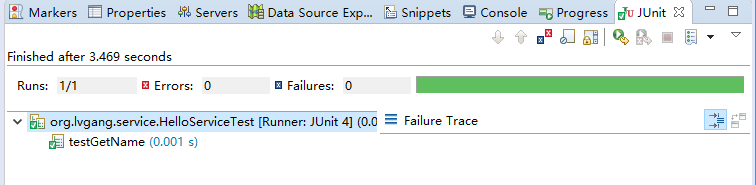
测试Controller(两个方法)
方法一:
package org.lvgang.controller; import static org.hamcrest.Matchers.equalTo; import static org.junit.Assert.assertEquals; import static org.junit.Assert.assertThat; import org.junit.Test; import org.junit.runner.RunWith; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.boot.test.autoconfigure.web.servlet.AutoConfigureMockMvc; import org.springframework.boot.test.context.SpringBootTest; import org.springframework.http.MediaType; import org.springframework.test.context.junit4.SpringRunner; import org.springframework.test.web.servlet.MockMvc; import org.springframework.test.web.servlet.MvcResult; import org.springframework.test.web.servlet.request.MockMvcRequestBuilders; /** * 测试类 * @author Administrator * */ @RunWith(SpringRunner.class) @SpringBootTest(webEnvironment = SpringBootTest.WebEnvironment.RANDOM_PORT) @AutoConfigureMockMvc public class HelloControllerTest { @Autowired private MockMvc mvc; @Test public void testHello() throws Exception { String uri = "/hello"; MvcResult mvcResult = mvc.perform(MockMvcRequestBuilders.get(uri).accept(MediaType.APPLICATION_JSON)) .andReturn(); int status = mvcResult.getResponse().getStatus(); String content = mvcResult.getResponse().getContentAsString(); System.out.println("content:"+content); System.out.println("status:"+status); assertEquals("错误,正确的返回值为200", status, 200); assertThat(content, equalTo("\"Hello world test!\"")); } }
在上面类中右击==》Run As ==》1Junit Test ,执行测试类,结果如下:

方法二:
package org.lvgang.controller; import static org.hamcrest.Matchers.equalTo; import static org.junit.Assert.assertEquals; import static org.junit.Assert.assertThat; import java.net.URL; import org.junit.Before; import org.junit.Test; import org.junit.runner.RunWith; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.boot.context.embedded.LocalServerPort; import org.springframework.boot.test.context.SpringBootTest; import org.springframework.boot.test.web.client.TestRestTemplate; import org.springframework.http.ResponseEntity; import org.springframework.test.context.junit4.SpringRunner; /** * 测试类 * * @author Administrator * */ @RunWith(SpringRunner.class) @SpringBootTest(webEnvironment = SpringBootTest.WebEnvironment.RANDOM_PORT) public class HelloControllerTest2 { @LocalServerPort private int port; private URL base; @Autowired private TestRestTemplate template; @Before public void setUp() throws Exception { this.base = new URL("http://localhost:" + port + "/hello"); } @Test public void testHello() throws Exception { System.out.println("base.toString():"+base.toString()); ResponseEntity<String> response = template.getForEntity(base.toString(), String.class); int status = response.getStatusCodeValue(); String content = response.getBody(); assertEquals("错误,正确的返回值为200", status, 200); assertThat(content, equalTo("Hello world test!")); } }
在上面类中右击==》Run As ==》1Junit Test ,执行测试类,结果如下:
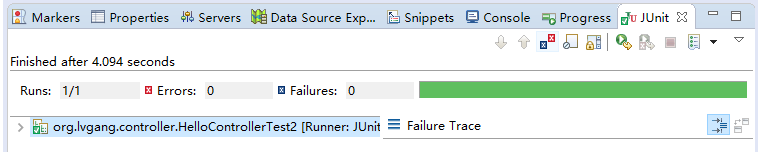
相关文章推荐
- Spring Boot学习之旅:(五)Spring Boot 使用 junit 单元测试
- 使用JUnit 对 Spring Boot REST API 执行单元测试
- Spring Boot---(11)SpringBoot使用Junit单元测试
- SpringBoot Web项目中中如何使用Junit
- 使用spring boot +Junit 测试 spring mvc 配置中心项目
- Spring Boot使用HandlerInterceptorAdapter和WebMvcConfigurerAdapter实现原始的登录验证
- Spring Boot 官方文档学习(一)入门及使用
- Spring Boot 之FilterRegistrationBean --支持web Filter 排序的使用
- Spring Boot 1.2.5使用redis做数据缓存
- 使用Spring Boot与否,初始化Spring应用的对比
- Spring Boot Admin的使用
- 使用Spring Boot开发 “Hello World” Web应用
- SpringBoot 使用properties配置文件实现多环境配置
- spring boot优雅的使用mybatis
- 使用IDEA在Spring Boot中集成JSP
- Spring Boot中使用Swagger2构建强大的RESTful API文档
- springBoot中Logback日志的使用
- 二十二、Spring Boot中使用Redis缓存
- spring boot @Configuration、@ImportResource使用
- Intellij IDEA 使用Spring-boot-devTools无效解决办法