C语言实现单向链表
2017-06-22 17:59
931 查看
结合我所写代码,pplist是一个二级指针,**pplist是首元素内的内容,*pplist可以表示整个链表的地址,类似于数组首元素地址与数组地址,这里用一个pplist二级指针,可以将链表地址,链表首元素地址.链表的结构大体如下图所示:
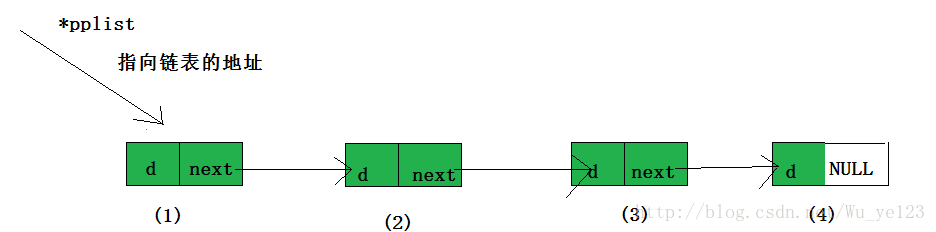
下面就是链表的各个函数代码了:
一个头文件(各个函数的声明),两个源文件(一个函数功能的实现,一个是测试函数功能的正确性)
头文件代码:
函数功能的实现:
函数测试源文件是调用各个函数.
下面就是链表的各个函数代码了:
一个头文件(各个函数的声明),两个源文件(一个函数功能的实现,一个是测试函数功能的正确性)
头文件代码:
#define _CRT_SECURE_NO_WARNINGS 1 #ifndef __LIST_H__ #define __LIST_H__ #include <stdio.h> #include <assert.h> #include <stdlib.h> typedef int Datatype; typedef struct Node { Datatype d;//结构体内所存数据 struct Node* next;//后半部分所存下个数据地址 }Node, *pNode,*pList; void InitList(pList *pplist);//初始化链表 void PushBack(pList *pplist,Datatype x);//尾插 void PopBack(pList *pplist);//尾删 void PushFront(pList *pplist,Datatype x);//头插 void PopFront(pList *pplist);头删 void Print(const pList *ppplist);//打印链表 void DistroyList(pList *pplist);//销毁链表 pNode Find(pList *pplist, Datatype x);查找指定数据元素 void Remove(pList *pplist, Datatype x);//删除指定元素 void RemoveAll(pList *pplist, Datatype x);//删除链表中所有的指定元素 void Insert(pList *pplist,pList pos, Datatype x);//指定位置插入 void Erase(pList *pplist, pNode pos);//指定位置删除 void Sort(pList *pplist);//冒泡排序 void ReverseList(pList *pplist);//倒序链表 #endif
函数功能的实现:
#define _CRT_SECURE_NO_WARNINGS 1 #include "list.h" void InitList(pList *pplist) { assert(pplist); *pplist = NULL; } void DistroyList(pList *pplist) { pNode cur = *pplist; assert(pplist); while (cur != NULL) { pNode p = cur; cur = cur->next; free(p); p = NULL; } *pplist = NULL; } pNode BuyNode(Datatype x) { pNode newNode = (pNode)malloc(sizeof(Node)); newNode->d = x; newNode->next = NULL; return newNode; } void Print(const pList *pplist) { pNode cur = *pplist; while(cur) { printf("%d----> ",cur->d); cur = cur->next; } printf("NULL\n"); } void PushBack(pList *pplist,Datatype x) { pNode newNode = BuyNode(x); pNode cur = *pplist; assert(pplist != NULL); if (*pplist == NULL) //无结点 { *pplist = newNode; return; } //有结点 while(cur->next != NULL) { cur = cur->next; } cur->next = newNode; } void PopBack(pList *pplist) { pNode cur = *pplist; assert(pplist); if (*pplist == NULL) { return; } if ((*pplist)->next == NULL) { free(*pplist); *pplist = NULL; return; } while(cur->next->next != NULL) { cur = cur->next; } free(cur->next); cur->next = NULL; } void PushFront(pList *pplist,Datatype x) { pNode newNode = BuyNode(x); assert(pplist); if (*pplist == NULL) { *pplist = newNode; } else { newNode->next = *pplist; *pplist = newNode; } } void PopFront(pList *pplist) { pNode cur = *pplist; assert(pplist); if (*pplist == NULL) { return; } else if((*pplist)->next == NULL) { free(*pplist); *pplist = NULL; return; } *pplist = cur->next; free(cur); cur = NULL; } pNode Find(pList *pplist, Datatype x) { pNode newNode = *pplist; assert(pplist); while(newNode!=NULL) { if (newNode->d == x) { return newNode; } newNode = newNode->next; } return NULL; } void Remove(pList *pplist, Datatype x) { pNode newNode = Find(pplist,x); assert(pplist); //未找到 if (newNode == NULL) { return; } if (newNode->next == NULL) //元素在尾部 { PopBack(pplist); return; } if (newNode == *pplist) //元素在头部 { PopFront(pplist); return; } newNode->d = newNode->next->d; //将newNode内的数据与next指针变量改为下个结构体元素内的数据 newNode->next = newNode->next->next; } void RemoveAll(pList *pplist, Datatype x) { pNode newNode = Find(pplist,x); assert(pplist); while (newNode!=NULL) { Remove(pplist,x); newNode = Find(pplist,x); //ERROR② //newNode = Find(&newNode,x); //ERROR① //newNode->d= newNode->next->d; //newNode->next = newNode->next->next; //newNode = Fine(pplist,x); } } //定点插入 void Insert(pList *pplist,pList pos, Datatype x) { pNode newNode = BuyNode(x); assert(pplist); assert(pos); if (*pplist == NULL) { PushBack(pplist,x); return; } newNode->next = pos->next; pos->next = newNode; } //定点删除 void Erase(pList *pplist, pNode pos) { pNode cur = *pplist; assert(pplist); assert(pos); if (*pplist == NULL) { return; } while (cur && (cur->next != pos)) { cur = cur->next; } if (cur != NULL) { cur->next = pos->next; free(pos); } } //冒泡 void Sort(pList *pplist) { Datatype tmp; pNode p = NULL; pNode q = NULL; assert(pplist); for (p = *pplist; p != NULL; p = p->next) { int flag = 1; for (q = p->next; q != NULL; q = q->next) { if (p->d > q->d) { flag = 0; tmp = p->d; p->d = q->d; q->d = tmp; } } if (flag == 1) { return; } } } //逆序 void ReverseList(pList *pplist) { pNode cur = *pplist; pNode newhead = *pplist; assert(pplist); if ((*pplist == NULL)||((*pplist)->next == NULL)) { return; } /*while(cur->next != NULL) { pNode tmp = cur; cur = cur->next; cur->next = tmp; }*/ while (cur->next) { pNode tmp = cur->next; cur->next = tmp->next; tmp->next = newhead; newhead = tmp; } *pplist = newhead; }
函数测试源文件是调用各个函数.
相关文章推荐
- 带表头的单向链表实现(C语言)
- c语言单向链表的简单实现,隐藏head节点
- C语言实现链表之单向链表(二)结点内存申请及数据初始化
- C语言实现一个简单的单向链表list
- C语言实现链表之单向链表(六)删除头结点
- C语言实现的单向链表
- C语言单向链表的实现
- C语言实现链表之单向链表(四)清空链表
- C语言实现链表之单向链表(三)创建链表
- C语言实现链表之单向链表(五)头结点前插入结点
- C语言数据结构之单向链表(已经调试可以实现相应的功能了,可是还是有几个问题现在还是不大理解,希望大家能够一起探讨)
- C语言实现单向链表及其各种排序(含快排,选择,插入,冒泡)
- C语言实现单向链表
- C语言单向动态链表程序,实现链表的建立,合并,重新排序,链表元素的插入与删除,以及根据元素成员的值进行元素删除。
- list.c - A linked list by C --- C语言实现的单向链表
- 单向链表的C语言实现
- 自己实现C语言单向链表
- C语言实现单向链表及其各种排序(含快排,选择,插入,冒泡)
- C语言实现链表之单向链表(七)尾结点后插入结点
- C语言实现链表之单向链表(一)头文件