C语言实现单链表(不带头结点)
2017-06-22 16:19
435 查看
头文件
测试程序:
test 1;
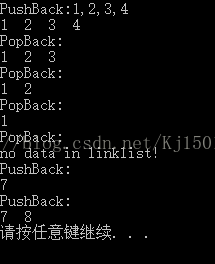
test 2:
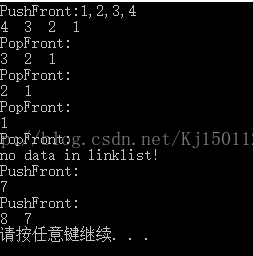
test 3:
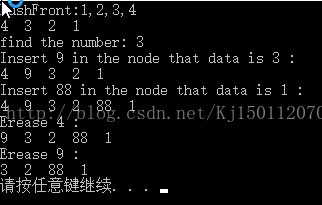
test 4:
#ifndef _LINK_LIST_H_ #define _LINK_LIST_H_ #include<stdio.h> #include<stdlib.h> #include<assert.h> typedef int DataType; //the type of the data in the linklist typedef struct Node //the struct of the of the node in the linklist { DataType _data; //Data domain struct Node *_next; //Pointer domain }Node,*pNode,*pList; //Init the linklist void InitLinkList(pList *pplist); //insert a new node in the back of the linklist void PushBack(pList *pplist,DataType x); //delete a node from the back of the linklist void PopBack(pList *pplist); //display the linklist void Display(pList pplist); //insert a new node in the head of the linklist void PushFront(pList *pplist,DataType x); //delete a node from the head of the linklis void PopFront(pList *pplist); //destroy the linklist void DestroyLinklist(pList *pplist); //find the pos of the data in the linklist pNode Find(const pList * pplist, DataType x); //insert new node in the Specify location int Insert(pList* plist, pNode pos, DataType x); //delete a node in the Specify location int Erase(pList *plist, pNode pos); //delete the first appearance node that it's data is x int Remove(pList *plist, DataType x); //delete the all appearance node that it's data is x void RemoveAll(pList *pplist, DataType x); //sort of the linklist from small to big void Sort(pList *pplist); #endif//_LINK_LIST_H_单链表功能的实现:
#include"LinkList.h" //Init the linklist void InitLinkList(pList *pplist) { *pplist=NULL; } //Create a new node pNode BuyNode(DataType x) { pNode p=(pNode)malloc(sizeof(Node));//create a new node if(p==NULL)//create failed { perror("BuyNode():malloc"); } //create successful p->_data=x; p->_next=NULL; return p; } //insert a new node in the back of the linklist void PushBack(pList *pplist,DataType x) { pNode pnode=NULL; pNode p=*pplist; assert(pplist!=NULL); pnode=BuyNode(x);//create a new node if(*pplist==NULL)//null linklist,don't have any number { *pplist=pnode; return; } //at least have two or more data in this linklist while(p->_next)//find the last node { p=p->_next; } p->_next=pnode; } //delete a node from the back of the linklist void PopBack(pList *pplist) { pNode p=*pplist; assert(pplist!=NULL&&*pplist!=NULL); if(p->_next==NULL)//only have a node inlinklist { *pplist=NULL; return; } //at least have two node in linklist while(p->_next->_next)//find the last node { p=p->_next; } free(p->_next); p->_next=NULL; } //display the linklist void Display(pList pplist) { pNode p=pplist; if(p==NULL)//while the linklist is empty { printf("no data in linklist!\n"); return; } 4000 while(p) { printf("%d ",p->_data); p=p->_next; } printf("\n"); } //insert a new node in the head of the linklist void PushFront(pList *pplist,DataType x) { pNode pnode=NULL; pNode p = NULL; assert(pplist!=NULL); pnode=BuyNode(x); if(*pplist==NULL)//if the linklist is NULL; { *pplist=pnode; return; } //at least have two numbers in the linklist p=*pplist; *pplist=pnode; (*pplist)->_next=p; } //delete a node from the head of the linklist void PopFront(pList *pplist) { pNode p=*pplist; assert(pplist!=NULL&&*pplist!=NULL); if((*pplist)->_next==NULL)//only have one node in the linklist { *pplist=NULL; return; } //at least have two node in the linklist *pplist=(*pplist)->_next; free(p); p=NULL; } //destroy the linklist void DestroyLinklist(pList *pplist) { assert(pplist!=NULL); while(*pplist) { pNode p=*pplist; *pplist=(*pplist)->_next; free(p); } *pplist=NULL; } //find the pos of the data in the linklist pNode Find(const pList *pplist, DataType x) { pNode p=*pplist; assert(pplist!=NULL); while(p) { if(p->_data==x) { return p; } p=p->_next; } return NULL; } //insert new node in the Specify location int Insert(pList* pplist, pNode pos, DataType x) { pNode pnode=*pplist; pNode new_node=NULL; assert(pplist!=NULL); new_node=BuyNode(x); if((*pplist)->_next==NULL)//only have one node in the linklist { if(*pplist==pos)//insert the pos node in the front of the first node { new_node->_next=*pplist; *pplist=new_node; return 1; } return -1; } //at least have two node on the linklist if(*pplist==pos)//if the pos node is the first node { new_node->_next=*pplist; *pplist=new_node; return 1; } else{//the pos node isn't the first node while(pnode->_next) { if(pnode->_next==pos)//find the frontal node of the pos node { new_node->_next=pnode->_next; pnode->_next=new_node; return 1; } pnode=pnode->_next; } return -1; } } //delete a node in the Specify location int Erase(pList *pplist, pNode pos) { pNode p=*pplist; assert(pplist!=NULL); if((*pplist)->_next==NULL)//only have one node in the linklist { if(pos==*pplist) { *pplist=NULL; return 1; } return -1; } if(*pplist==pos)//if the pos node is the first node in the linklist { pNode ret=*pplist; *pplist=(*pplist)->_next; free(ret); ret=NULL; return 1; } else//the pos node isn't the first node in the linklist { while(p->_next) { if(p->_next==pos) { pNode ret=p->_next; p->_next=p->_next->_next; free(ret); ret=NULL; return 1; } p=p->_next; } return -1; } } //delete the first appearance node that it's data is x int Remove(pList *pplist, DataType x) { pNode p_find=NULL; assert(pplist!=NULL); p_find=Find(pplist,x); if(p_find==NULL) { printf("This data isn't exist in the linklist or the linklist is empty!\n"); return -1; } //the pos must found in the linklist return Erase(pplist,p_find); } //delete the all appearance node that it's data is x void RemoveAll(pList *pplist, DataType x) { pNode p_find=*pplist; assert(pplist!=NULL); while((p_find=Find(pplist,x)))//find the data in the linklist { Erase(pplist,p_find);//Erase the node that we find in the linklist } } //sort of the linklist from small to big void Sort(pList *pplist) { pNode p_one=*pplist; pNode p_two=NULL; assert(pplist!=NULL); if((*pplist)->_next==NULL) { return; } while(p_one->_next)//First cycle { p_two=p_one->_next; while(p_two)//Second cycle { if(p_one->_data>p_two->_data)//swap { DataType tmp=p_one->_data; p_one->_data=p_two->_data; p_two->_data=tmp; } p_two=p_two->_next; } p_one=p_one->_next; } }
测试程序:
#include"linklist.h" //testing of PopBack and PushBack void test1() { pList pplist; InitLinkList(&pplist); printf("PushBack:1,2,3,4\n"); PushBack(&pplist,1); PushBack(&pplist,2); PushBack(&pplist,3); PushBack(&pplist,4); Display(pplist); printf("PopBack:\n"); PopBack(&pplist); Display(pplist); printf("PopBack:\n"); PopBack(&pplist); Display(pplist); printf("PopBack:\n"); PopBack(&pplist); Display(pplist); printf("PopBack:\n"); PopBack(&pplist); Display(pplist); printf("PushBack:\n"); PushBack(&pplist,7); Display(pplist); printf("PushBack:\n"); PushBack(&pplist,8); Display(pplist); DestroyLinklist(&pplist); } //testing of PopFront and PushFront void test2() { pList pplist; InitLinkList(&pplist); printf("PushFront:1,2,3,4\n"); PushFront(&pplist,1); PushFront(&pplist,2); PushFront(&pplist,3); PushFront(&pplist,4); Display(pplist); printf("PopFront:\n"); PopFront(&pplist); Display(pplist); printf("PopFront:\n"); PopFront(&pplist); Display(pplist); printf("PopFront:\n"); PopFront(&pplist); Display(pplist); printf("PopFront:\n"); PopFront(&pplist); Display(pplist); printf("PushFront:\n"); PushFront(&pplist,7); Display(pplist); printf("PushFront:\n"); PushFront(&pplist,8); Display(pplist); DestroyLinklist(&pplist); } //testing Find,Insert and Erase void test3() { pNode p_find=NULL; pList pplist; InitLinkList(&pplist); printf("PushFront:1,2,3,4\n"); PushFront(&pplist,1); PushFront(&pplist,2); PushFront(&pplist,3); PushFront(&pplist,4); Display(pplist); p_find=Find(&pplist,3); if(p_find) { printf("find the number: %d\n",p_find->_data); }else{ printf("no this number in the linklist or the linklist is empty!\n"); } //Insert the 9 to the pos of p_find in the linklist printf("Insert 9 in the node that data is 3 :\n"); Insert(&pplist,p_find,9); Display(pplist); //Insert the 88 to the pos of p_find in the linklist p_find=Find(&pplist,1); printf("Insert 88 in the node that data is 1 :\n"); Insert(&pplist,p_find,88); Display(pplist); //Erease the 4 to the pos of p_find in the linklist p_find=Find(&pplist,4); printf("Erease 4 :\n"); Erase(&pplist,p_find); Display(pplist); //Erease the 9 to the pos of p_find in the linklist p_find=Find(&pplist,9); printf("Erease 9 :\n"); Erase(&pplist,p_find); Display(pplist); DestroyLinklist(&pplist); } //testing Remove ,RemoveAll and Sort void test4() { pNode p_find=NULL; pList pplist; InitLinkList(&pplist); printf("PushFront 8,2,6,4:\n"); PushFront(&pplist,8); PushFront(&pplist,2); PushFront(&pplist,6); PushFront(&pplist,4); Display(pplist); //Remove 3 printf("Remove 3:\n"); Remove(&pplist,3); Display(pplist); //Remove 2 printf("Remove 2:\n"); Remove(&pplist,2); Display(pplist); printf("PushBack 12,3,5,12,5,12 :\n"); PushBack(&pplist,12); PushBack(&pplist,3); PushBack(&pplist,5); PushBack(&pplist,12); PushBack(&pplist,5); PushBack(&pplist,12); Display(pplist); printf("RemoveAll 12:\n"); RemoveAll(&pplist,12); Display(pplist); printf("Sort :\n"); Sort(&pplist); Display(pplist); DestroyLinklist(&pplist); } int main() { //test1(); //test2(); //test3(); //test4(); return 0; }
test 1;
test 2:
test 3:
test 4:
相关文章推荐
- C语言实现双向非循环链表(带头结点尾结点)的基本操作
- 不带头结点的单链表的实现(C语言)
- C语言实现单链表(带头结点)的基本操作(创建,头插法,尾插法,删除结点,打印链表)
- C语言实现单链表节点的删除(带头结点)
- c语言实现--带头结点单链表操作
- 数据结构模版----单链表SimpleLinkList[不带头结点&&伪OO](C语言实现)
- C语言实现带头结点的链表的创建、查找、插入、删除操作
- C语言实现单链表(带头结点)的基本操作(创建,头插法,尾插法,删除结点,打印链表)
- c语言实现--不带头结点的单链表操作
- C语言实现单链表(带头结点)的基本操作
- C语言实现单链表节点的删除(带头结点)
- C语言实现单链表(不带头结点)节点的插入
- C语言实现使用带头结点的单链表来构造栈结构
- C语言实现单链表-不带头结点
- C语言实现双向非循环链表(带头结点尾结点)的节点插入
- 数据结构模版----单链表SimpleLinkList[不带头结点](C语言实现)
- 链表中每个结点的data域存放一个二进制位。并在此链表上实现对二进制数加1的运算。 用C语言编写 用以存放输入的二进制数 建立 一个带头结点的线性链表
- 学习笔记——C语言实现单链表的基本操作:创建、输出、插入结点、删除结点、逆序链表
- 带头结点的单链表实现就地逆置的更优方法
- 单链表、带头结点的单链表、循环单链表 以及其操作实现