qt下的时钟程序(简单美丽,继承自QWidget的Clock,用timer调用update刷新,然后使用paintEvent作画就行了,超详细中文注释)good
2017-06-14 18:40
501 查看
最近抽空又看了下qt,发现用它来实现一些东西真的很容易
比如下面这个例子,绘制了个圆形的时钟,
但代码却清晰易懂[例子源自奇趣科技提供的例子]
因为清晰,所以就只写注释了,吼吼
其实也就这么几行代码
头文件
//clock.h
#ifndef CLOCK_H
#define CLOCK_H
#include <QWidget>
class Clock : public QWidget
{
//对于具有signal,slot机制的类需要声明
Q_OBJECT
public:
Clock(QWidget *parent = 0);
protected:
//重绘用的事件处理函式
void paintEvent(QPaintEvent *event);
};
#endif // CLOCK_H
cpp文件
1 #include "clock.h"
2
3
4 #include <QtGui>
5
6 #include "clock.h"
7
8 Clock::Clock(QWidget *parent): QWidget(parent)
9 {
10 //声明一个定时器
11 QTimer *timer = new QTimer(this);
12 //连接信号与槽
13 connect(timer, SIGNAL(timeout()), this, SLOT(update()));
14 timer->start(1000);
15 //设置窗体名称与大小
16 setWindowTitle(tr("Clock"));
17 resize(200, 200);
18
19 }
20
21
22 void Clock::paintEvent(QPaintEvent *)
23
24 {
25 //下面三个数组用来定义表针的三个顶点,以便后面的填充
26 static const QPoint hourHand[3] = {
27 QPoint(3, 8),
28 QPoint(-3, 8),
29 QPoint(0, -40)
30 };
31 static const QPoint minuteHand[3] = {
32 QPoint(3, 8),
33 QPoint(-3, 8),
34 QPoint(0, -70)
35 };
36 static const QPoint secondHand[3] = {
37 QPoint(3, 8),
38 QPoint(-3, 8),
39 QPoint(0, -90)
40 };
41
42 //填充表针的颜色
43 QColor hourColor(127, 0, 127);
44 QColor minuteColor(0, 127, 127, 191);
45 QColor secondColor(127, 127,0,120);
46 //绘制的范围
47 int side = qMin(width(), height());
48 //获取当前的时间
49 QTime time = QTime::currentTime();
50 //声明用来绘图用的“画家”
51 QPainter painter(this);
52
53 painter.setRenderHint(QPainter::Antialiasing);
54 //重新定位坐标起始点点
55 painter.translate(width() / 2, height() / 2);
56 //设定花布的边界
57 painter.scale(side / 200.0, side / 200.0);
58 //填充时针,不需要边线所以NoPen
59 painter.setPen(Qt::NoPen);
60 //画刷颜色设定
61 painter.setBrush(hourColor);
62 //保存“画家”的状态
63 painter.save();
64 //将“画家”(的”视角“)根据时间参数转移
65 painter.rotate(30.0 * ((time.hour() + time.minute() / 60.0)));
66 //填充时针的区域
67 painter.drawConvexPolygon(hourHand, 3);
68 //恢复填充前“画家”的状态
69 painter.restore();
70
71 //下面画表示小时的刻度,此时要用到画笔(因为要划线)
72 painter.setPen(hourColor);
73 //十二个刻度,循环下就好了
74 for (int i = 0; i < 12; ++i) {
75 //没次都是这样,先画跳线,再转个角
76 painter.drawLine(88, 0, 96, 0);
77 painter.rotate(30.0);
78 }
79
80 //后面的跟前面的类似,分别绘制了分针和秒针,及相应的刻度,我就不废话了
81
82 painter.setPen(Qt::NoPen);
83
84 painter.setBrush(minuteColor);
85
86
87 painter.save();
88 painter.rotate(6.0 * (time.minute() + time.second() / 60.0));
89 painter.drawConvexPolygon(minuteHand, 3);
90 painter.restore();
91
92 painter.setPen(minuteColor);
93
94 for (int j = 0; j < 60; ++j) {
95 if ((j % 5) != 0)
96 painter.drawLine(92, 0, 96, 0);
97 painter.rotate(6.0);
98 }
99
100
101 painter.setPen(Qt::NoPen);
102
103 painter.setBrush(secondColor);
104
105 painter.save();
106 painter.rotate(6.0*time.second());
107 painter.drawConvexPolygon(secondHand,3);
108 painter.restore();
109
110 }
111
112
main文件
#include <QApplication>
#include "clock.h"
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
//声明下,再show出来就可以了
Clock clock;
clock.show();
return app.exec();
}
pro文件
HEADERS = clock.h
SOURCES = clock.cpp \
main.cpp
下面是运行时的截图,开发环境为qtcreator
在奇趣提供的例子中还将其做成了控件,有时间在写点关于那个例子的东西。
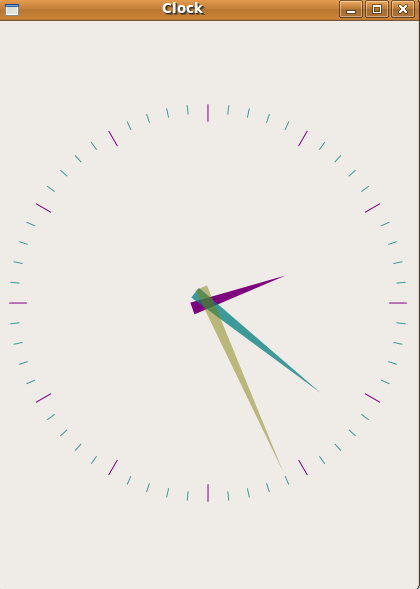
http://www.cnblogs.com/pingf/archive/2009/08/06/1540374.html
比如下面这个例子,绘制了个圆形的时钟,
但代码却清晰易懂[例子源自奇趣科技提供的例子]
因为清晰,所以就只写注释了,吼吼
其实也就这么几行代码
头文件
//clock.h
#ifndef CLOCK_H
#define CLOCK_H
#include <QWidget>
class Clock : public QWidget
{
//对于具有signal,slot机制的类需要声明
Q_OBJECT
public:
Clock(QWidget *parent = 0);
protected:
//重绘用的事件处理函式
void paintEvent(QPaintEvent *event);
};
#endif // CLOCK_H
cpp文件
1 #include "clock.h"
2
3
4 #include <QtGui>
5
6 #include "clock.h"
7
8 Clock::Clock(QWidget *parent): QWidget(parent)
9 {
10 //声明一个定时器
11 QTimer *timer = new QTimer(this);
12 //连接信号与槽
13 connect(timer, SIGNAL(timeout()), this, SLOT(update()));
14 timer->start(1000);
15 //设置窗体名称与大小
16 setWindowTitle(tr("Clock"));
17 resize(200, 200);
18
19 }
20
21
22 void Clock::paintEvent(QPaintEvent *)
23
24 {
25 //下面三个数组用来定义表针的三个顶点,以便后面的填充
26 static const QPoint hourHand[3] = {
27 QPoint(3, 8),
28 QPoint(-3, 8),
29 QPoint(0, -40)
30 };
31 static const QPoint minuteHand[3] = {
32 QPoint(3, 8),
33 QPoint(-3, 8),
34 QPoint(0, -70)
35 };
36 static const QPoint secondHand[3] = {
37 QPoint(3, 8),
38 QPoint(-3, 8),
39 QPoint(0, -90)
40 };
41
42 //填充表针的颜色
43 QColor hourColor(127, 0, 127);
44 QColor minuteColor(0, 127, 127, 191);
45 QColor secondColor(127, 127,0,120);
46 //绘制的范围
47 int side = qMin(width(), height());
48 //获取当前的时间
49 QTime time = QTime::currentTime();
50 //声明用来绘图用的“画家”
51 QPainter painter(this);
52
53 painter.setRenderHint(QPainter::Antialiasing);
54 //重新定位坐标起始点点
55 painter.translate(width() / 2, height() / 2);
56 //设定花布的边界
57 painter.scale(side / 200.0, side / 200.0);
58 //填充时针,不需要边线所以NoPen
59 painter.setPen(Qt::NoPen);
60 //画刷颜色设定
61 painter.setBrush(hourColor);
62 //保存“画家”的状态
63 painter.save();
64 //将“画家”(的”视角“)根据时间参数转移
65 painter.rotate(30.0 * ((time.hour() + time.minute() / 60.0)));
66 //填充时针的区域
67 painter.drawConvexPolygon(hourHand, 3);
68 //恢复填充前“画家”的状态
69 painter.restore();
70
71 //下面画表示小时的刻度,此时要用到画笔(因为要划线)
72 painter.setPen(hourColor);
73 //十二个刻度,循环下就好了
74 for (int i = 0; i < 12; ++i) {
75 //没次都是这样,先画跳线,再转个角
76 painter.drawLine(88, 0, 96, 0);
77 painter.rotate(30.0);
78 }
79
80 //后面的跟前面的类似,分别绘制了分针和秒针,及相应的刻度,我就不废话了
81
82 painter.setPen(Qt::NoPen);
83
84 painter.setBrush(minuteColor);
85
86
87 painter.save();
88 painter.rotate(6.0 * (time.minute() + time.second() / 60.0));
89 painter.drawConvexPolygon(minuteHand, 3);
90 painter.restore();
91
92 painter.setPen(minuteColor);
93
94 for (int j = 0; j < 60; ++j) {
95 if ((j % 5) != 0)
96 painter.drawLine(92, 0, 96, 0);
97 painter.rotate(6.0);
98 }
99
100
101 painter.setPen(Qt::NoPen);
102
103 painter.setBrush(secondColor);
104
105 painter.save();
106 painter.rotate(6.0*time.second());
107 painter.drawConvexPolygon(secondHand,3);
108 painter.restore();
109
110 }
111
112
main文件
#include <QApplication>
#include "clock.h"
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
//声明下,再show出来就可以了
Clock clock;
clock.show();
return app.exec();
}
pro文件
HEADERS = clock.h
SOURCES = clock.cpp \
main.cpp
下面是运行时的截图,开发环境为qtcreator
在奇趣提供的例子中还将其做成了控件,有时间在写点关于那个例子的东西。
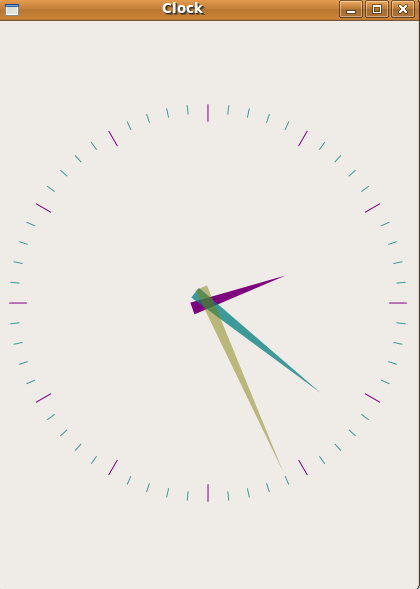
http://www.cnblogs.com/pingf/archive/2009/08/06/1540374.html
相关文章推荐
- 简单的QT绘图程序(把全部的点都记录下来,然后在paintEvent里使用drawLine函数进行绘制,貌似效率很低。。。)
- 以不同用户身份运行程序,/savecred只需要输入一次密码(GetTokenByName取得EXPLORER.EXE的令牌,然后调用CreateProcessAsUser,而且使用LoadUserProfile解决另存文件的问题)good
- 简单的QT绘图程序(把全部的点都记录下来,然后在paintEvent里使用drawLine函数进行绘制,貌似效率很低。。。)
- 一个 Qt 显示图片的控件(继承QWidget,使用QPixmap记录图像,最后在paintEvent进行绘制,可缩放)
- 使用QT调用外部程序-小心linux中的命令陷阱
- Qt之ui在程序中的使用——(2)多继承法
- TGraphicControl(自绘就2步,直接自绘自己,不需要调用VCL框架提供的函数重绘所有子控件,也不需要自己来提供PaintWindow函数让管理框架来调用)与TControl关键属性方法速记(Repaint要求父控件执行详细代码来重绘自己,还是直接要求Invalidate无效后Update刷新父控件,就看透明不透明这个属性,因为计算显示的区域有所不同)
- QT 使用QProcess 调用外部程序并截取输出流
- 使用Trigger让UpdatePanel外部的控件也支持无刷新异步调用
- 在使用Arduino中遇到的问题(无法使用中文注释、程序无法下载)
- Qt之ui在程序中的使用——(1)单继承法
- 使用Trigger让UpdatePanel外部的控件也支持无刷新异步调用
- 使用Trigger让UpdatePanel外部的控件也支持无刷新异步调用
- QT中调用外部程序:QProcess的使用
- C#使用timer实现的简单闹钟程序
- Ubuntu下使用Java调用IKAnalyzer中文分词程序失效的解决方法
- 使用 timer 来创建一个简单的报警程序
- QT中调用外部程序:QProcess的使用
- 汇编打字游戏程序(别人写的,我修改了部分,然后写了详细的注释)
- 使用VC2005编译真正的静态Qt程序-Qt中文论坛-夏威夷雪人