网络编程之新闻案例
2017-05-28 12:27
274 查看
我们要用到两个开源的框架,SmartImageView,AsyncHttpClient.
我们要做到这个效果。
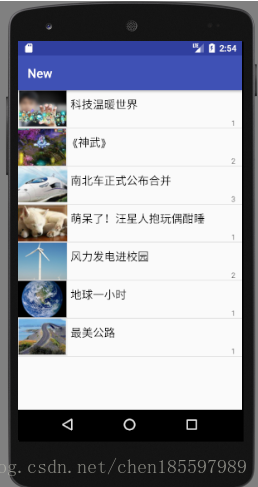
图片是用SmartImageView。
1.建一个新闻的实体类。
2主布局文件
3.子布局
4.自定义一个adapter
5.MainActivity中
5.这里用到了json数据解析。
你在运行前,需将你的json数据和图片放到你Tomcat的webapp的ROOT文件夹下。
我们要做到这个效果。
图片是用SmartImageView。
1.建一个新闻的实体类。
public class NewsInfo { private String icon; private String title; private String description; private int type; private long comment; public NewsInfo(String icon, String title, String description, int type, long comment) { this.icon = icon; this.title = title; this.description = description; this.type = type; this.comment = comment; } public String getIcon() { return icon; } public void setIcon(String icon) { this.icon = icon; } public String getTitle() { return title; } public void setTitle(String title) { this.title = title; } public String getDescription() { return description; } public void setDescription(String description) { this.description = description; } public int getType() { return type; } public void setType(int type) { this.type = type; } public long getComment() { return comment; } public void setComment(long comment) { this.comment = comment; }
2主布局文件
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:id="@+id/activity_main" android:layout_width="match_parent" android:layout_height="match_parent" tools:context="cn.edu.bzu.anew.MainActivity"> <FrameLayout android:layout_width="match_parent" android:layout_height="match_parent"> <LinearLayout android:id="@+id/loading" android:layout_width="match_parent" android:layout_height="match_parent" android:gravity="center" android:orientation="vertical" android:visibility="invisible"> <ProgressBar android:layout_width="wrap_content" android:layout_height="wrap_content" /> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="正在加载信息..." /> </LinearLayout> <ListView android:id="@+id/lv_news" android:layout_width="match_parent" android:layout_height="match_parent" /> </FrameLayout> </RelativeLayout>
3.子布局
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="65dp"> <com.loopj.android.image.SmartImageView android:id="@+id/siv_icon" android:layout_width="80dp" android:layout_height="60dp" android:scaleType="centerCrop" android:src="@mipmap/ic_launcher" android:layout_alignParentLeft="true" android:layout_alignParentStart="true"></com.loopj.android.image.SmartImageView> <TextView android:id="@+id/tv_title" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginLeft="5dp" android:layout_marginTop="10dp" android:layout_toRightOf="@id/siv_icon" android:ellipsize="end" android:maxLength="20" android:singleLine="true" android:text="我是标题" android:textColor="#000000" android:textSize="18sp" /> <TextView android:id="@+id/tv_description" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_below="@id/tv_title" android:layout_marginLeft="5dp" android:layout_marginTop="5dp" android:layout_toRightOf="@id/siv_icon" android:ellipsize="end" android:maxLength="16" android:maxLines="1" android:text="我是描述" android:textColor="#99000000" android:textSize="14sp" /> <TextView android:id="@+id/tv_type" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_alignParentBottom="true" android:layout_alignParentRight="true" android:layout_marginBottom="5dp" android:layout_marginRight="10dp" android:text="评论" android:textColor="#99000000" android:textSize="12sp" /> </RelativeLayout>
4.自定义一个adapter
public class NewsAdapter extends ArrayAdapter<NewsInfo>{ public NewsAdapter(Context context, List<NewsInfo> objects) { super(context, R.layout.news_item, objects); } @NonNull @Override public View getView(int position, View convertView, ViewGroup parent) { NewsInfo newsinfo= getItem(position);//传递position,获取当前位置对应的newsinfo新闻信息 View view=null; viewHolder viewHolder; if(convertView==null){ //判断convertView中是否加载了布局,有没有缓存。为空说明没有缓存 view=LayoutInflater.from(getContext()).inflate(R.layout.news_item,null); viewHolder=new viewHolder(); viewHolder.siv= (SmartImageView) view.findViewById(R.id.siv_icon); viewHolder.tv_title= (TextView) view.findViewById(R.id.tv_title); viewHolder.tv_description= (TextView) view.findViewById(R.id.tv_description); viewHolder.tv_type= (TextView) view.findViewById(R.id.tv_type); view.setTag(viewHolder); //保存 }else{ view=convertView; viewHolder=(viewHolder) view.getTag(); } viewHolder.siv.setImageUrl(newsinfo.getIcon()); viewHolder.tv_title.setText(newsinfo.getTitle());//传递题目 viewHolder.tv_description.setText(newsinfo.getDescription()); viewHolder.tv_type.setText(newsinfo.getType()+""); return view; } class viewHolder{//添加类,封装需要查找的控件 TextView tv_title; TextView tv_description; TextView tv_type; SmartImageView siv; } }
5.MainActivity中
public class MainActivity extends AppCompatActivity { private ListView lvNews; private List<NewsInfo> newsInfos; private LinearLayout loading; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); lvNews=(ListView)findViewById(R.id.lv_news); loading=(LinearLayout)findViewById(R.id.loading); fillData(); } private void fillData(){ AsyncHttpClient client =new AsyncHttpClient(); client.get("http://10.51.9.254:8080/NewsInfo.json",new AsyncHttpResponseHandler(){ @Override public void onSuccess(int i, org.apache.http.Header[] headers, byte[] bytes) { try{ String json=new String(bytes,"utf-8"); newsInfos= JsonParse.getNewsInfo(json); if(newsInfos==null){ Toast.makeText(MainActivity.this,"解析失败", Toast.LENGTH_LONG).show(); }else{ loading .setVisibility(View.INVISIBLE); lvNews.setAdapter(new NewsAdapter(MainActivity.this,newsInfos)); } } catch (UnsupportedEncodingException e) { e.printStackTrace(); } } @Override public void onFailure(int i, org.apache.http.Header[] headers, byte[] bytes, Throwable throwable) { } } ); } }
5.这里用到了json数据解析。
public class JsonParse { public static List<NewsInfo>getNewsInfo(String json){ Gson gson =new Gson(); Type listType=new TypeToken<List<NewsInfo>> (){ }.getType(); List<NewsInfo>newsInfos=gson.fromJson(json,listType); return newsInfos; } }
你在运行前,需将你的json数据和图片放到你Tomcat的webapp的ROOT文件夹下。
相关文章推荐
- 网络编程案例---新闻发布系统
- 网络编程案例简易新闻客户端
- 案例三:java网络编程(对象流传输)
- Java网络编程之组播小案例
- Java网络编程案例--CS模型的简单实现
- Python案例-网络编程-异步解耦
- Python案例-网络编程-socket入门-server&client
- linux 网络编程典型案例(proxy.c) - linux 操作
- JAVA网络编程UDP案例
- JAVA网络编程UDP案例
- Python案例-网络编程-socket-解决ssh消息粘包问题
- Java网络编程之组播小案例
- Linux网络编程案例
- java网络编程和io,多线程结合完成文件上传和下载案例
- Android网络编程-1.4 网络应用实战案例
- 案例四:java网络编程 双工通讯(带界面)
- JAVA 网络编程(包含案例)
- Java基础知识强化之网络编程笔记05:UDP之多线程实现聊天室案例
- 《java入门第一季》之UDP协议下的网络编程小案例
- Python案例-网络编程-线程池