【JavaWeb-30】SpringMVC原理、入门程序、参数传递
2017-05-27 21:03
671 查看
1、springmvc类似于struts,核心就是进行请求响应的处理,但是这里和struts不同的是,springmvc的前端控制器就是一个servlet类,只不过继承了之后做了些改变,所以效率上比struts高,因为struts是封装成了过滤器filter。
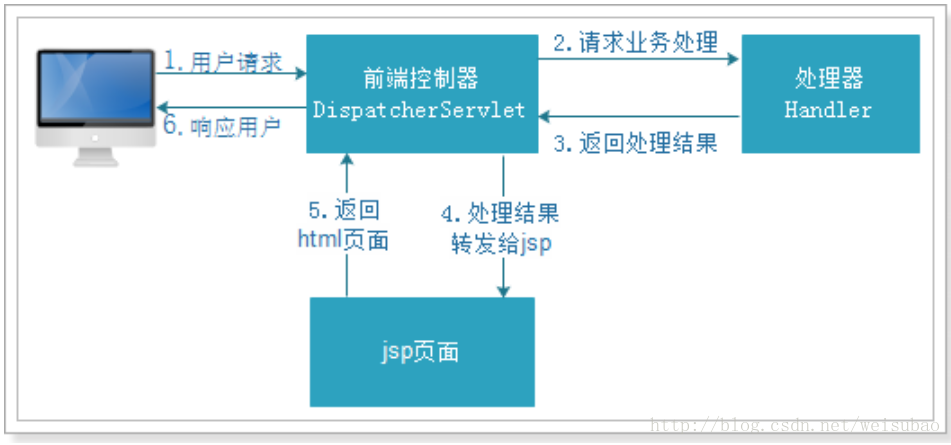
2、我们创建一个web项目。
——第一步,就是导入springmvc的jar包。
——第二步,就是在web.xml中进行前端控制器的配置,其实就是配置一个servlet。
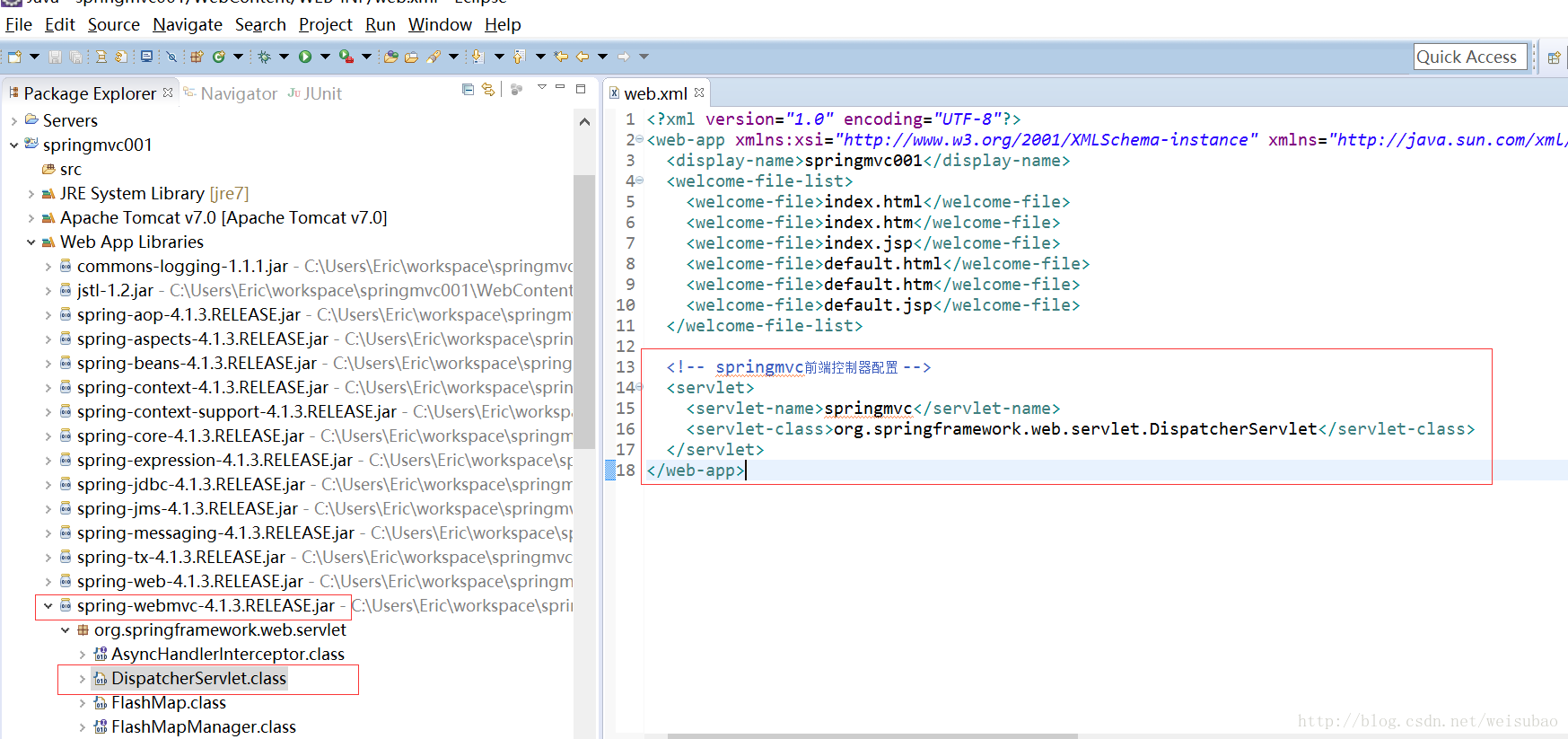
——在src下新建springmvc.xml,然后添加约束。
——下面我们就需要写handler了,也就是Controller。我们在放Controller的package下新建一个ItemController,我们需要把这个类注解成
——接下来,我们就可以在Controller中写代码了。这里面,我们是用的ModelAndView,Model用的死数据,view用的jsp路径名字。
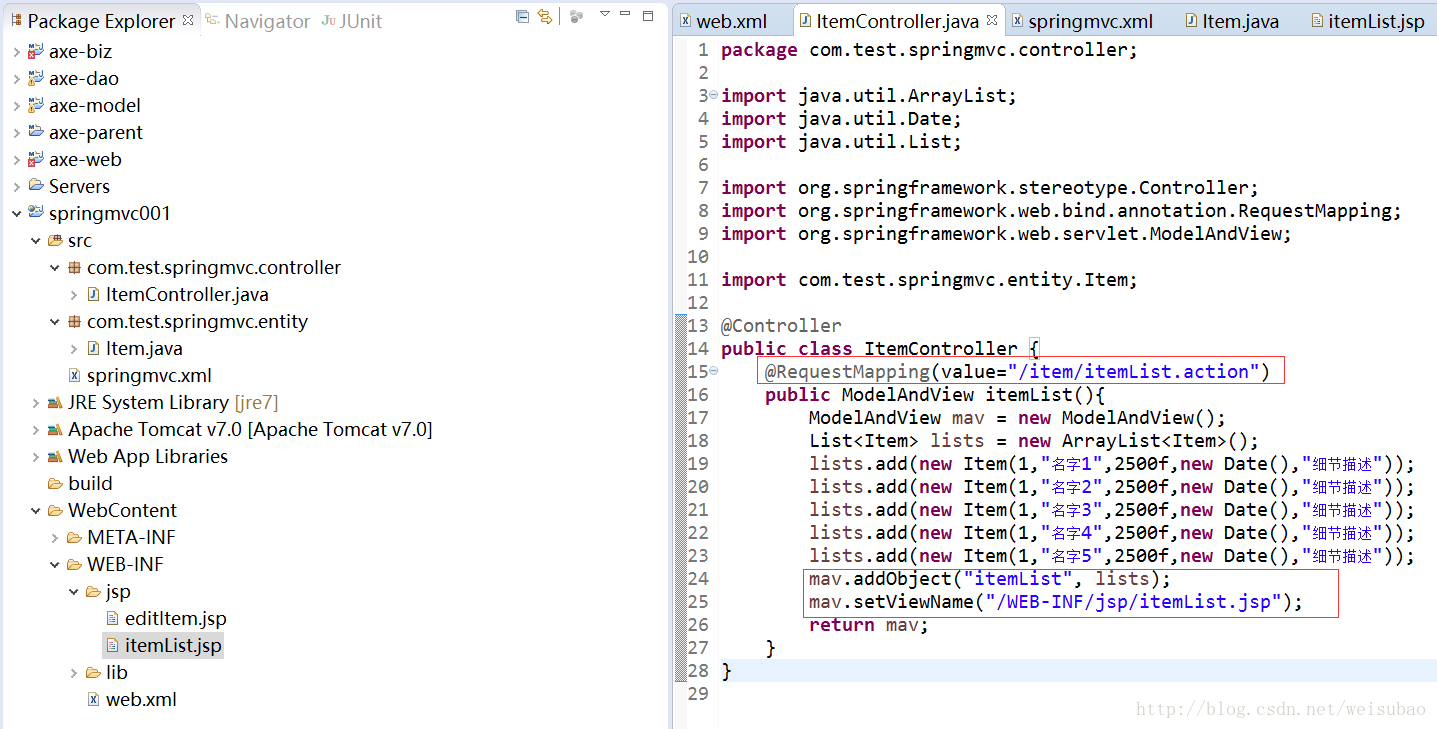
3、详细流程和原理。
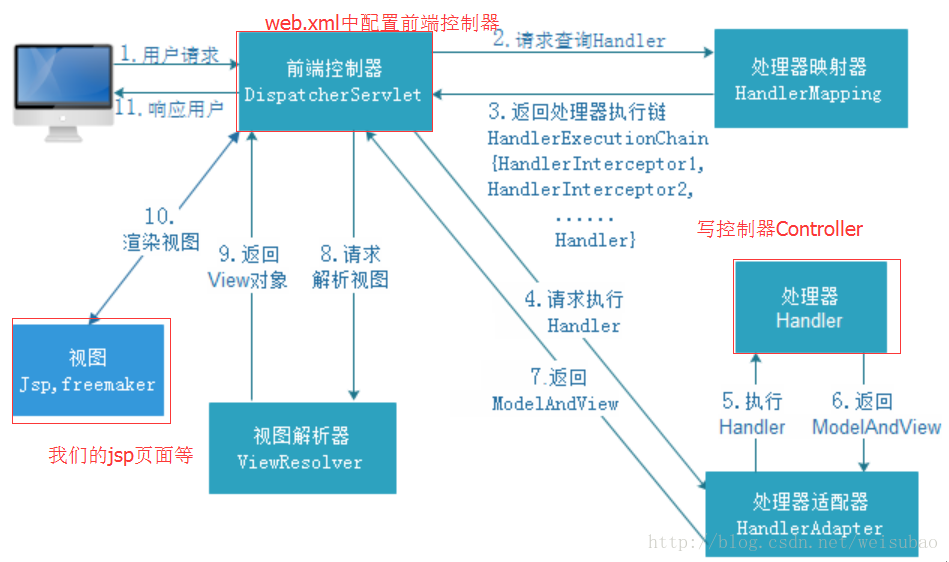
4、我们可以看看上面的那个原理图,我们发现其中一些映射器和适配器、解析器我们都没有配置,因为这是springmvc帮我们配置好了。我们可以打开jar包看一下。发现映射器类不止一个,因为是针对不同的方式的,有针对xml配置的,有针对注解的,我们用不同的方式,它就会调用不同的类来执行。
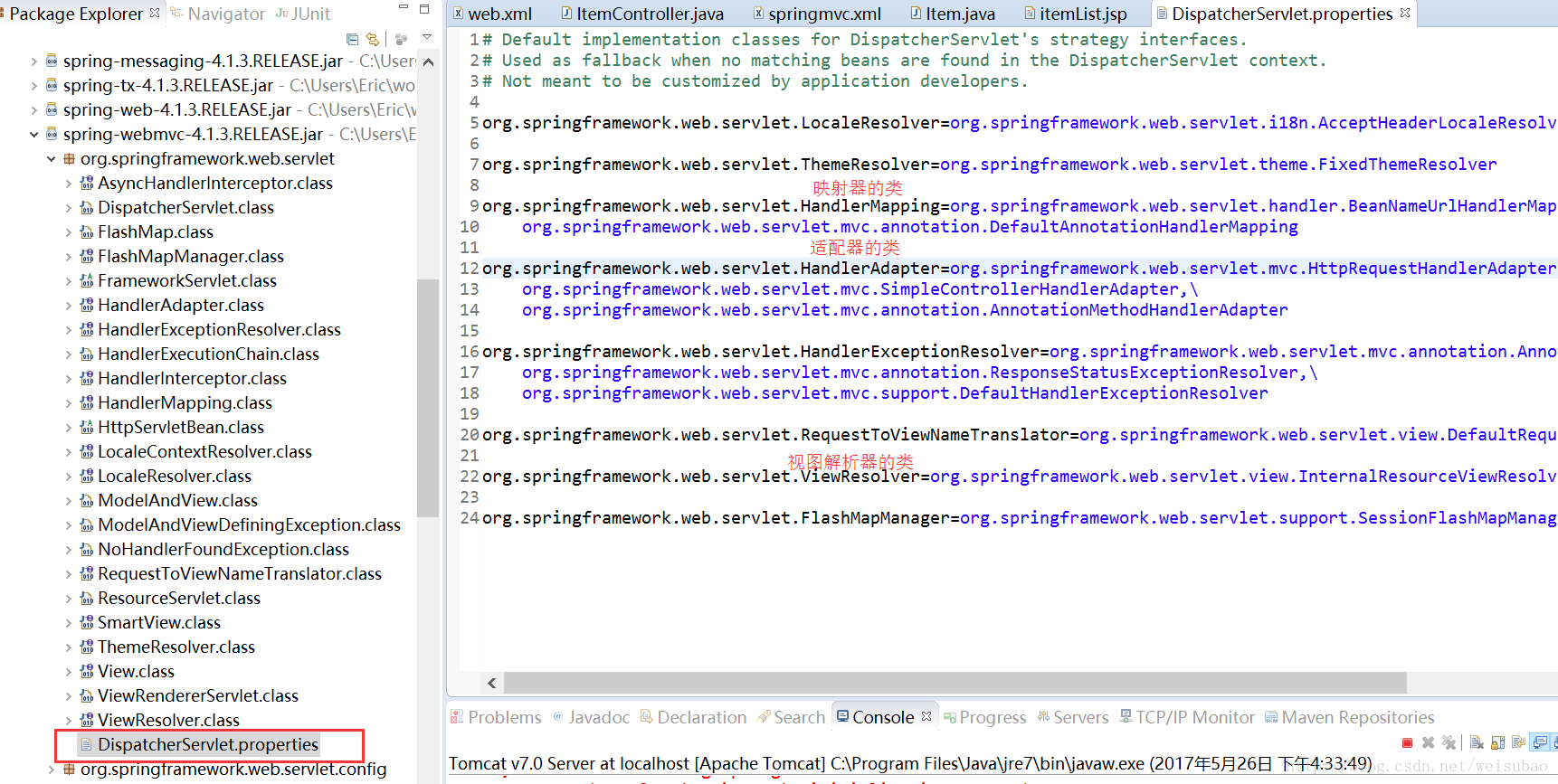
我们可以打开源码看看这些映射器的类(比如注解类吧
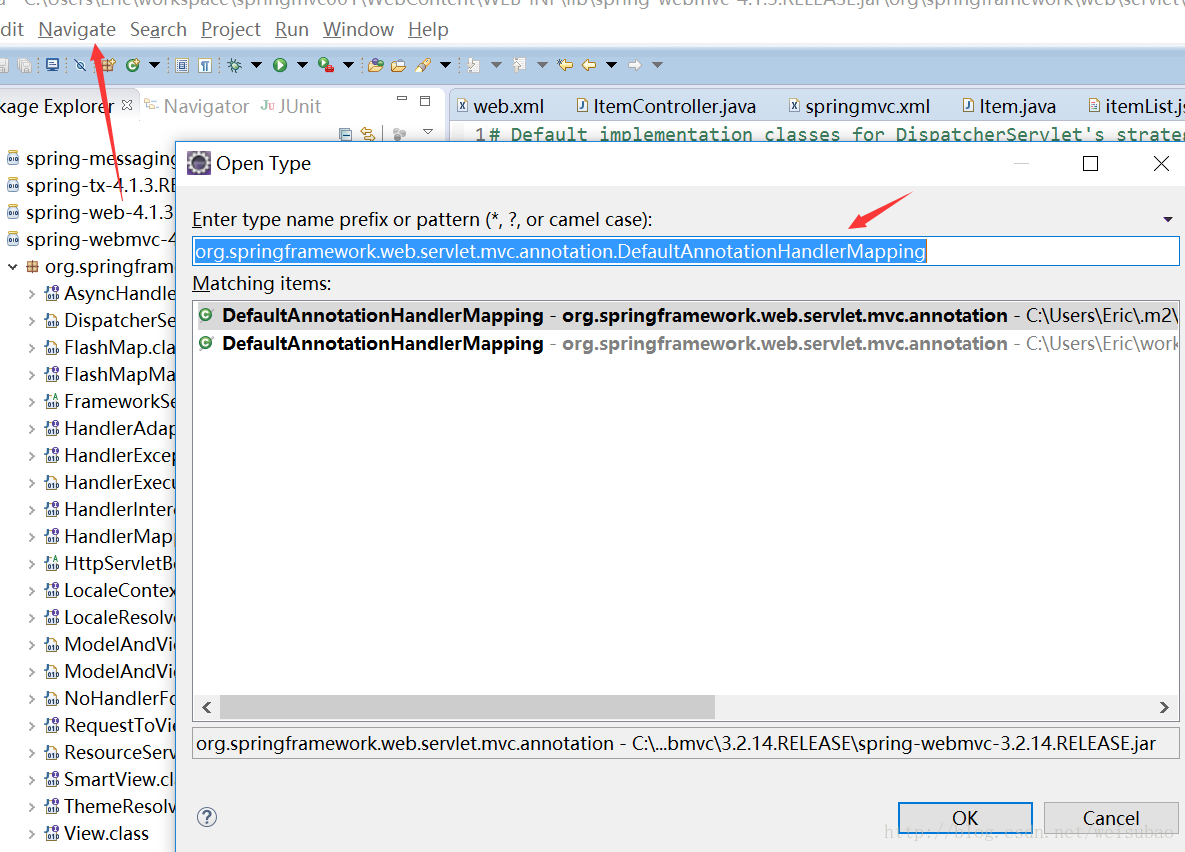
打开源码,发现这个类已经不建议使用了,有新的类。
其实,适配器类的注解类也是有新的了。所以,我们可以不管,因为这个默认的能继续使用,但如果我们要换成最新的话,那就在我们的springmvc.xml中进行配置:
——这个样子,其实就已经可以了,但是这个太长了,我们可以用下面的一行代码来代替:
——其实,这个样子也就可以了。但是我们还有视图解析器,这个貌似没动过,其实不需要动。但是我们写视图路径的时候觉得太长,如果我只想写成下面这样:
那么就需要在springmvc中设置:
5、传递数据。比如在url上绑定一个数据传递给下一个界面的话,在下一个界面的Controller中,可以用HttpServletRequest来获取。
或者直接根据url中的name设置形参,直接使用,它会自动接收参数值:
2、我们创建一个web项目。
——第一步,就是导入springmvc的jar包。
——第二步,就是在web.xml中进行前端控制器的配置,其实就是配置一个servlet。
<!-- springmvc前端控制器配置 --> <servlet> <servlet-name>springmvc</servlet-name> <!-- 这个值是在jar包中的,见下图 --> <servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class> <!-- 这是加载配置文件的,这里的param-name和spring的一样,param-value的值就是我们一会要在src下新建的文件 --> <init-param> <param-name>contextConfigLocation</param-name> <param-value>classpath:springmvc.xml</param-value> </init-param> </servlet> <servlet-mapping> <servlet-name>springmvc</servlet-name> <!-- .action 就是拦截所有以action结尾的,.do一样的原理 / 就是拦截所有的除了jsp。png、css这些静态的也拦截,建议使用 /* 这是真拦截所有的,包括jsp,不建议使用 --> <url-pattern>*.action</url-pattern> </servlet-mapping>
——在src下新建springmvc.xml,然后添加约束。
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:p="http://www.springframework.org/schema/p" xmlns:context="http://www.springframework.org/schema/context" xmlns:mvc="http://www.springframework.org/schema/mvc" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-4.0.xsd http://www.springframework.org/schema/mvc http://www.springframework.org/schema/mvc/spring-mvc-4.0.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-4.0.xsd"> </beans>
——下面我们就需要写handler了,也就是Controller。我们在放Controller的package下新建一个ItemController,我们需要把这个类注解成
@Controller,但是这个注解没有用啊,需要我们在springmvc.xml中扫描这个文件。下面是直接扫描com.test下,这样不管是Controller还是后面我们写的service等都能被扫描。
<context:component-scan base-package="com.test"></context:component-scan>
——接下来,我们就可以在Controller中写代码了。这里面,我们是用的ModelAndView,Model用的死数据,view用的jsp路径名字。
@Controller public class ItemController { @RequestMapping(value="/item/itemList.action") public ModelAndView itemList(){ ModelAndView mav = new ModelAndView(); List<Item> lists = new ArrayList<Item>(); lists.add(new Item(1,"名字1",2500f,new Date(),"细节描述")); lists.add(new Item(1,"名字2",2500f,new Date(),"细节描述")); lists.add(new Item(1,"名字3",2500f,new Date(),"细节描述")); lists.add(new Item(1,"名字4",2500f,new Date(),"细节描述")); lists.add(new Item(1,"名字5",2500f,new Date(),"细节描述")); mav.addObject("itemList", lists); mav.setViewName("/WEB-INF/jsp/itemList.jsp"); return mav; } }
3、详细流程和原理。
4、我们可以看看上面的那个原理图,我们发现其中一些映射器和适配器、解析器我们都没有配置,因为这是springmvc帮我们配置好了。我们可以打开jar包看一下。发现映射器类不止一个,因为是针对不同的方式的,有针对xml配置的,有针对注解的,我们用不同的方式,它就会调用不同的类来执行。
我们可以打开源码看看这些映射器的类(比如注解类吧
org.springframework.web.servlet.mvc.annotation.DefaultAnnotationHandlerMapping)。快捷键ctrl+shift+T。搜索我们要查找的类。
打开源码,发现这个类已经不建议使用了,有新的类。
/** * @deprecated in Spring 3.2 in favor of * {@link org.springframework.web.servlet.mvc.method.annotation.RequestMappingHandlerMapping RequestMappingHandlerMapping} */
其实,适配器类的注解类也是有新的了。所以,我们可以不管,因为这个默认的能继续使用,但如果我们要换成最新的话,那就在我们的springmvc.xml中进行配置:
<!-- 配置映射器 --> <bean class="org.springframework.web.servlet.mvc.method.annotation.RequestMappingHandlerMapping"></bean> <!-- 配置适配器 --> <bean class="org.springframework.web.servlet.mvc.method.annotation.RequestMappingHandlerMapping"></bean>
——这个样子,其实就已经可以了,但是这个太长了,我们可以用下面的一行代码来代替:
<!-- 注解驱动 --> <mvc:annotation-driven></mvc:annotation-driven>
——其实,这个样子也就可以了。但是我们还有视图解析器,这个貌似没动过,其实不需要动。但是我们写视图路径的时候觉得太长,如果我只想写成下面这样:
//mav.setViewName("/WEB-INF/jsp/itemList.jsp"); mav.setViewName("itemList");
那么就需要在springmvc中设置:
<!-- 视图解析器的配置 --> <bean class="org.springframework.web.servlet.view.InternalResourceViewResolver"> <property name="prefix" value="/WEB-INF/jsp/"></property> <property name="suffix" value=".jsp"></property> </bean>
5、传递数据。比如在url上绑定一个数据传递给下一个界面的话,在下一个界面的Controller中,可以用HttpServletRequest来获取。
@RequestMapping(value="xxxx") public ModelAndView itemEdit(HttpServletRequest request){ String id = request.getParameter("id"); ... }
或者直接根据url中的name设置形参,直接使用,它会自动接收参数值:
@RequestMapping(value="xxxx") public ModelAndView itemEdit(String id,HttpServletRequest request){ ... }
相关文章推荐
- JavaWeb——springMVC入门程序
- JavaWeb——MyBatis入门程序
- JAVAWEB开发之SpringMVC详解(一)——SpringMVC的框架原理、架构简介、与mybatis整合和注解方式的使用、
- Java springmvc web项目,基于maven的hello world入门级项目使用IntelliJ IDEA 2017版本
- Java Web编程入门--SpringMVC首页
- JAVAWEB开发之redis学习(九)——redis主从复制入门及原理
- 【JavaWeb-26】MyBatis快速入门程序+一些扩展
- JAVAWEB开发之SpringMVC详解(一)——SpringMVC的框架原理、架构简介、与mybatis整合和注解方式的使用、
- Java springmvc web项目,基于maven的hello world入门级项目使用IntelliJ IDEA 2017版本
- Java - SpringMVC基础入门,创建一个HelloWorld程序
- 我的第十个java程序--(其实是修改别人的web代码{springmvc+mybatis},知道了原理后其实一切都变的很简单)
- java-web程序的运行原理
- [ASP.NET入门随想三]抽象的力量——WEB程序编程思想的演进
- 面向 Java 开发人员的 Ajax: Google Web Toolkit 入门
- Google Web Toolkit--用Java编写AJAX程序
- java程序-编译原理实验
- Swing 写的客户端程序在java web start 运行,多语言过程中,JOptionPane.showMessageDialog() 按钮多语言问题
- 编译原理课程设计---用java写的SNLCompiler(简单嵌套语言SNL的编译程序)
- java web三层架构配置入门
- 一些入门的java小程序