Spring中基于AOP的XML架构
2017-05-25 16:25
295 查看
以下内容引用自http://wiki.jikexueyuan.com/project/spring/aop-with-spring-framenwork/xml-schema-based-aop-with-spring.html:
为了使用aop命名空间标签,需要导入spring-aop架构,如下所示:
还需要增加而外的jar包用于支持AspectJ:
集成步骤:
1、声明一个aspect(方面)
一个aspect是使用<aop:aspect>元素声明的,并使用ref属性引用bean,如下所示:
这个“aBean”将被配置和依赖注入就像其他Spring bean一样。
2、声明一个pointcut(切入点)
切入点有助于确定用不同建议执行的关联点(即方法)。在使用基于XML Schema的配置时,切入点将定义如下:
以下示例定义了一个名为“businessService”的切入点,该切入点将匹配在com.tutorialspoint包中的Student类中可用的getName()方法:
提示:
①类似:“execution(*com.tutorialspoint.Student.getName(..))”这样的语法叫做AspectJ切入点语法,参考:http://www.cnblogs.com/EasonJim/p/6901806.html
②官方文档关于AspectJ的介绍:https://docs.spring.io/spring/docs/current/spring-framework-reference/html/aop.html#aop-ataspectj
3、声明建议(通知类型)
可以使用<aop:{ADVICE NAME}>五个建议中的任何一个元素在<aop:aspect>内声明,如下所示:
可以对不同的建议使用相同的doRequiredTask或不同的方法。这些方法将被定义为方面模块的一部分。
至此集成完毕。
基于AOP的XML架构的示例:
pom.xml:
Logging.java:
Student.java:
beans.xml:
App.java:
测试结果:
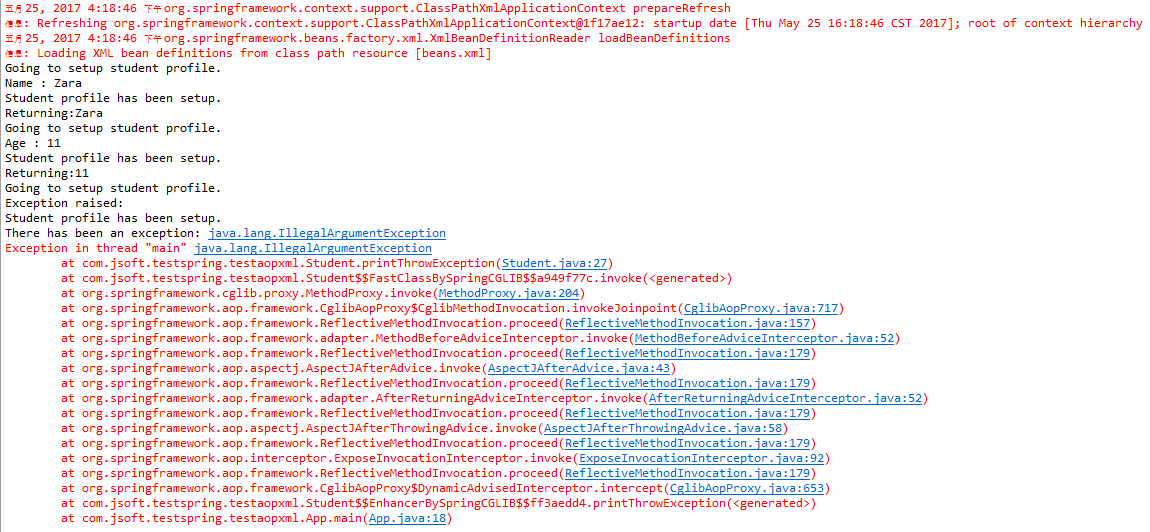
测试工程:https://github.com/easonjim/5_java_example/tree/master/springtest/test16/testaopxml
为了使用aop命名空间标签,需要导入spring-aop架构,如下所示:
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:aop="http://www.springframework.org/schema/aop" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop.xsd"> </beans>
还需要增加而外的jar包用于支持AspectJ:
<!-- aspectjrt.jar --> <!-- https://mvnrepository.com/artifact/org.aspectj/aspectjrt --> <dependency> <groupId>org.aspectj</groupId> <artifactId>aspectjrt</artifactId> <version>1.8.10</version> </dependency> <!-- aspectjweaver.jar --> <!-- https://mvnrepository.com/artifact/org.aspectj/aspectjweaver --> <dependency> <groupId>org.aspectj</groupId> <artifactId>aspectjweaver</artifactId> <version>1.8.10</version> </dependency>
集成步骤:
1、声明一个aspect(方面)
一个aspect是使用<aop:aspect>元素声明的,并使用ref属性引用bean,如下所示:
<aop:config> <aop:aspect id="myAspect" ref="aBean"> ... </aop:aspect> </aop:config> <bean id="aBean" class="..."> ... </bean>
这个“aBean”将被配置和依赖注入就像其他Spring bean一样。
2、声明一个pointcut(切入点)
切入点有助于确定用不同建议执行的关联点(即方法)。在使用基于XML Schema的配置时,切入点将定义如下:
<aop:config> <aop:aspect id="myAspect" ref="aBean"> <aop:pointcut id="businessService" expression="execution(* com.xyz.myapp.service.*.*(..))"/> ... </aop:aspect> </aop:config> <bean id="aBean" class="..."> ... </bean>
以下示例定义了一个名为“businessService”的切入点,该切入点将匹配在com.tutorialspoint包中的Student类中可用的getName()方法:
<aop:config> <aop:aspect id="myAspect" ref="aBean"> <aop:pointcut id="businessService" expression="execution(*com.tutorialspoint.Student.getName(..))"/> ... </aop:aspect> </aop:config> <bean id="aBean" class="..."> ... </bean>
提示:
①类似:“execution(*com.tutorialspoint.Student.getName(..))”这样的语法叫做AspectJ切入点语法,参考:http://www.cnblogs.com/EasonJim/p/6901806.html
②官方文档关于AspectJ的介绍:https://docs.spring.io/spring/docs/current/spring-framework-reference/html/aop.html#aop-ataspectj
3、声明建议(通知类型)
可以使用<aop:{ADVICE NAME}>五个建议中的任何一个元素在<aop:aspect>内声明,如下所示:
<aop:config> <aop:aspect id="myAspect" ref="aBean"> <aop:pointcut id="businessService" expression="execution(* com.xyz.myapp.service.*.*(..))"/> <!-- a before advice definition --> <aop:before pointcut-ref="businessService" method="doRequiredTask"/> <!-- an after advice definition --> <aop:after pointcut-ref="businessService" method="doRequiredTask"/> <!-- an after-returning advice definition --> <!--The doRequiredTask method must have parameter named retVal --> <aop:after-returning pointcut-ref="businessService" returning="retVal" method="doRequiredTask"/> <!-- an after-throwing advice definition --> <!--The doRequiredTask method must have parameter named ex --> <aop:after-throwing pointcut-ref="businessService" throwing="ex" method="doRequiredTask"/> <!-- an around advice definition --> <aop:around pointcut-ref="businessService" method="doRequiredTask"/> ... </aop:aspect> </aop:config> <bean id="aBean" class="..."> ... </bean>
可以对不同的建议使用相同的doRequiredTask或不同的方法。这些方法将被定义为方面模块的一部分。
至此集成完毕。
基于AOP的XML架构的示例:
pom.xml:
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.jsoft.testspring</groupId> <artifactId>testaopxml</artifactId> <version>0.0.1-SNAPSHOT</version> <packaging>jar</packaging> <name>testaopxml</name> <url>http://maven.apache.org</url> <properties> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> </properties> <dependencies> <dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>3.8.1</version> <scope>test</scope> </dependency> <!-- Spring Core --> <!-- http://mvnrepository.com/artifact/org.springframework/spring-core --> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-core</artifactId> <version>4.1.4.RELEASE</version> </dependency> <!-- Spring Context --> <!-- http://mvnrepository.com/artifact/org.springframework/spring-context --> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-context</artifactId> <version>4.1.4.RELEASE</version> </dependency> <!-- String AOP --> <!-- http://mvnrepository.com/artifact/org.springframework/spring-aop --> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-aop</artifactId> <version>4.1.4.RELEASE</version> </dependency> <!-- aspectjrt.jar --> <!-- https://mvnrepository.com/artifact/org.aspectj/aspectjrt --> <dependency> <groupId>org.aspectj</groupId> <artifactId>aspectjrt</artifactId> <version>1.8.10</version> </dependency> <!-- aspectjweaver.jar --> <!-- https://mvnrepository.com/artifact/org.aspectj/aspectjweaver --> <dependency> <groupId>org.aspectj</groupId> <artifactId>aspectjweaver</artifactId> <version>1.8.10</version> </dependency> </dependencies> </project>
Logging.java:
package com.jsoft.testspring.testaopxml; public class Logging { public void beforeAdvice() { System.out.println("Going to setup student profile."); } public void afterAdvice() { System.out.println("Student profile has been setup."); } public void afterReturningAdvice(Object retVal) { System.out.println("Returning:" + retVal.toString()); } public void AfterThrowingAdvice(IllegalArgumentException ex) { System.out.println("There has been an exception: " + ex.toString()); } }
Student.java:
package com.jsoft.testspring.testaopxml; public class Student { private Integer age; private String name; public void setAge(Integer age) { this.age = age; } public Integer getAge() { System.out.println("Age : " + age); return age; } public void setName(String name) { this.name = name; } public String getName() { System.out.println("Name : " + name); return name; } public void printThrowException() { System.out.println("Exception raised:"); throw new IllegalArgumentException(); } }
beans.xml:
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:aop="http://www.springframework.org/schema/aop" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop.xsd"> <aop:config> <aop:aspect id="log" ref="logging"> <aop:pointcut id="selectAll" expression="execution(* com.jsoft..*.*(..))"/> <aop:before pointcut-ref="selectAll" method="beforeAdvice"/> <aop:after pointcut-ref="selectAll" method="afterAdvice"/> <aop:after-returning pointcut-ref="selectAll" returning="retVal" method="afterReturningAdvice"/> <aop:after-throwing pointcut-ref="selectAll" throwing="ex" method="AfterThrowingAdvice"/> </aop:aspect> </aop:config> <bean id="student" class="com.jsoft.testspring.testaopxml.Student"> <property name="name" value="Zara" /> <property name="age" value="11"/> </bean> <bean id="logging" class="com.jsoft.testspring.testaopxml.Logging"/> </beans>
App.java:
package com.jsoft.testspring.testaopxml; import org.springframework.context.ApplicationContext; import org.springframework.context.support.ClassPathXmlApplicationContext; /** * Hello world! * */ public class App { public static void main( String[] args ) { ApplicationContext context = new ClassPathXmlApplicationContext("beans.xml"); Student student = (Student) context.getBean("student"); student.getName(); student.getAge(); student.printThrowException(); } }
测试结果:
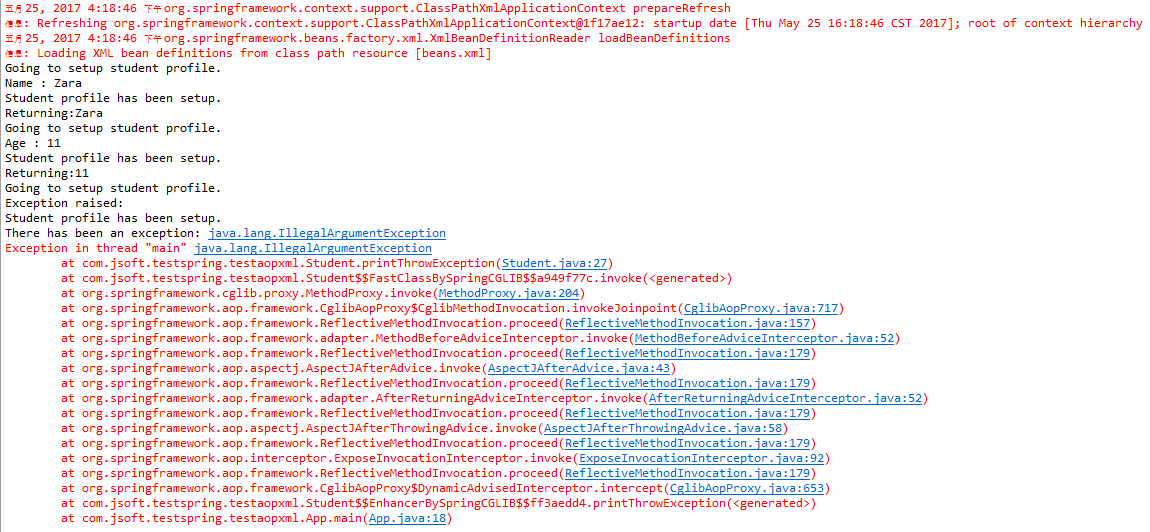
测试工程:https://github.com/easonjim/5_java_example/tree/master/springtest/test16/testaopxml
相关文章推荐
- spring aop的使用(注解方式以及基于xml配置方式)
- 8.4.6: Spring的AOP---基于XML配置文件的管理方式
- Spring中的AOP(六)——基于XML配置文件方式的AOP
- Spring AOP - 基于XML配置文件
- spring添加AOP切面-基于XML配置
- 一步一步深入spring(6)--使用基于XML配置的spring实现的AOP
- Spring -- 基于XML的AOP通知配置
- Spring学习总结7(AOP-基于XML)
- 基于XML的Spring AOP配置
- Spring AOP(3)基于XML配置实现的示例
- Spring3.0 入门进阶(3):基于XML方式的AOP使用
- (一)Spring AOP:基于XML配置文件
- Spring的AOP配置(基于xml)
- spring -- aop基于xml的简单实现
- spring aop的使用(注解方式以及基于xml配置方式)
- Spring中AOP基于XML的两种配置方法
- springAOP基于xml的配置前后环绕通知
- Spring 基于XML配置的AOP入门案例
- Spring 基于xml配置方式的AOP
- Spring AOP使用Aspectj基于xml方式,初始化Bean参数