MyBatis整合到Spring 配置文件总结与分析
2017-05-12 17:31
489 查看
上文参考了myBatis的开发文档后,对mybatis的配置文件做了较为详细的分析,但实际上在工作中Mybatis更多的是与Spring整合在一起使用。
我们先看看还没整合到Spring的Mybatis配置文件
下面我们可以把里面大多数的配置内容删除
关于数据库的连接我们可以放到spring的配置文件中
接着,开始书写spring的配置文件,名字叫做spring-datasource.xml
在最开始写mapper扫描器的时候,我会这么写
MapperFactoryBean 创建的代理类实现了 UserMapper 接口,并且注入到应用程序中。 但是同时又会带来一个,如果我有上百个mapper文件,我是否就要写上百次呢
举个例子
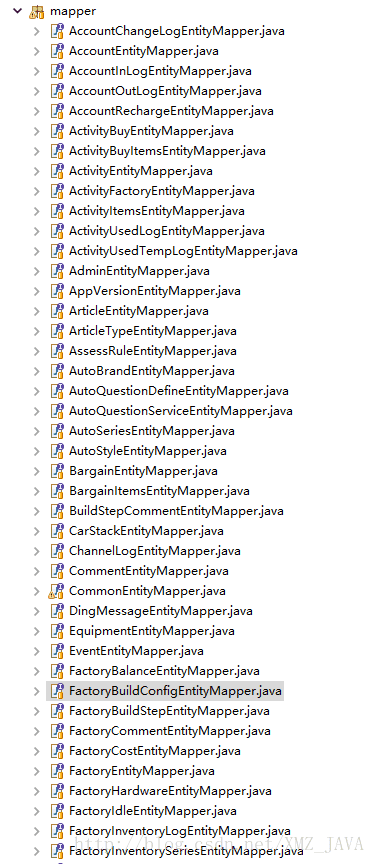
所以在实际生产环境,我们一般采用下面的配置方式
我们可以使用分号或逗号 作为分隔符设置多于一个的包路径。每个映射器将会在指定的包路径中递归地被搜索到
没有必要去指定SqlSessionFactory 或 SqlSessionTemplate,因为MapperScannerConfigurer 将会创建MapperFactoryBean,之后自动装配。但是,如果你使 用了一个以上的DataSource ,那自动装配可能会失效。这种 情况下 ,你可以使用 sqlSessionFactoryBeanName 或 sqlSessionTemplateBeanName 属性来设置正确的bean名称来使用。这就是它如何来配置的,注意bean的名称是必须的,而不是bean的引用,因 此,value属性在这里替代通常的ref:
使用基于注解方式配置事务
配置事务的传播特性
配置事务拦截器拦截哪些类的哪些方法,一般设置成拦截Service
我们先看看还没整合到Spring的Mybatis配置文件
<?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE configuration PUBLIC "-//mybatis.org//DTD Config 3.0//EN" "http://mybatis.org/dtd/mybatis-3-config.dtd"> <configuration> <typeAliases> <typeAlias alias="Message" type="com.xmz.entity.MessageEntity"/> </typeAliases> <environments default="sql1"> <environment id="sql1"> <transactionManager type="JDBC" /> <dataSource type="POOLED"> <property name="driver" value="com.mysql.jdbc.Driver" /> <property name="url" value="jdbc:mysql://localhost:3306/test?useUnicode=true&characterEncoding=UTF-8&zeroDateTimeBehavior=convertToNull" /> <property name="username" value="root" /> <property name="password" value="123" /> </dataSource> </environment> </environments> <mappers> <mapper resource="com/xmz/mapper/mapper.xml"></mapper> </mappers> </configuration>
下面我们可以把里面大多数的配置内容删除
<?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE configuration PUBLIC "-//mybatis.org//DTD Config 3.0//EN" "http://mybatis.org/dtd/mybatis-3-config.dtd"> <configuration> <typeAliases> <typeAlias alias="Message" type="com.xmz.entity.MessageEntity"/> </typeAliases> </configuration>
关于数据库的连接我们可以放到spring的配置文件中
接着,开始书写spring的配置文件,名字叫做spring-datasource.xml
引入配置文件
<bean class="org.springframework.beans.factory.config.PropertyPlaceholderConfigurer"> <property name="locations"> <list> <value>classpath:/conf/db-config.properties</value> <value>classpath:/conf/common.properties</value> </list> </property> </bean>
数据库配置
这里使用数据库连接池,dbcp、c3p0都可以使用,我这里使用的是阿里的druid<bean id="dataSourceDDS" class="com.alibaba.druid.pool.DruidDataSource" init-method="init" destroy-method="close"> <!-- 基本属性 url、user、password --> <property name="url" value="${dataSource.url}" /> <property name="username" value="${dataSource.username}" /> <property name="password" value="${dataSource.password}" /> <property name="connectionProperties" value="${dataSource.driver}"></property> <!--<property name="passwordCallback">--> <!--<bean class="com.hxyz.datasource.security.DBPasswordCallback"/>--> <!--</property>--> <!-- 数据库监控,及日志配置 --> <property name="filters" value="log4j"/> <!-- 配置监控统计拦截的filters --> <!-- <property name="proxyFilters"> <list> <ref bean="stat-filter"/> <ref bean="wall-filter"/> <ref bean="log-filter"/> </list> </property> --> <!-- 初始化连接大小 --> <property name="initialSize" value="1"/> <!-- 连接池最大使用连接数量 --> <property name="maxActive" value="300"/> <!-- 获取连接最大等待时间 --> <property name="maxWait" value="60000"/> <!-- 连接池最小空闲 --> <property name="minIdle" value="5"/> <!-- 配置间隔多久才进行一次检测,检测需要关闭的空闲连接,单位是毫秒 --> <property name="timeBetweenEvictionRunsMillis" value="3000"/> <!-- 配置一个连接在池中最小生存的时间,单位是毫秒 --> <property name="minEvictableIdleTimeMillis" value="300000"/> <property name="validationQuery" value="SELECT 'x'"/> <property name="testWhileIdle" value="true"/> <property name="testOnBorrow" value="false"/> <property name="testOnReturn" value="false"/> <property name="poolPreparedStatements" value="true"/> <property name="maxPoolPreparedStatementPerConnectionSize" value="20"/> <property name="removeAbandoned" value="true"/> <!-- 打开removeAbandoned功能 --> <property name="removeAbandonedTimeout" value="1800"/> <!-- 1800秒,也就是30分钟 --> <property name="logAbandoned" value="true"/> <!-- 关闭abanded连接时输出错误日志 --> </bean>
SqlSessionFactory
SqlSessionFactory,使用过MyBatis的朋友们一定对这个类不陌生,它是用于配置MyBatis环境的,SqlSessionFactory里面有两个属性configLocation、mapperLocations,顾名思义分别代表配置文件的位置和映射文件的位置,这里只要路径配置正确,Spring便会自动去加载这两个配置文件了。<bean id="sqlSessionFactory" class="org.mybatis.spring.SqlSessionFactoryBean"> <property name="dataSource" ref="dataSourceDDS"/> <property name="typeAliasesPackage" value="com.hxyz.domain.entity"/> <property name="mapperLocations" value="classpath*:mapper/*.xml"/> <property name="plugins"> <ref bean="paginationInterceptor"/> </property> </bean>
MapperScannerConfigurer
MapperScannerConfigurer 自动扫描 将Mapper接口生成代理注入到Spring,所以我们一般在dao层无需添加注解在最开始写mapper扫描器的时候,我会这么写
<bean id="userMapper" class="org.mybatis.spring.mapper.MapperFactoryBean"> <property name="mapperInterface" value="org.mybatis.spring.sample.mapper.UserMapper" /> <property name="sqlSessionFactory" ref="sqlSessionFactory" /> </bean>
MapperFactoryBean 创建的代理类实现了 UserMapper 接口,并且注入到应用程序中。 但是同时又会带来一个,如果我有上百个mapper文件,我是否就要写上百次呢
举个例子
所以在实际生产环境,我们一般采用下面的配置方式
<bean class="org.mybatis.spring.mapper.MapperScannerConfigurer"> <property name="sqlSessionFactoryBeanName" value="sqlSessionFactory"/> <property name="basePackage" value="com.hxyz.domain.mapper"/> </bean>
我们可以使用分号或逗号 作为分隔符设置多于一个的包路径。每个映射器将会在指定的包路径中递归地被搜索到
没有必要去指定SqlSessionFactory 或 SqlSessionTemplate,因为MapperScannerConfigurer 将会创建MapperFactoryBean,之后自动装配。但是,如果你使 用了一个以上的DataSource ,那自动装配可能会失效。这种 情况下 ,你可以使用 sqlSessionFactoryBeanName 或 sqlSessionTemplateBeanName 属性来设置正确的bean名称来使用。这就是它如何来配置的,注意bean的名称是必须的,而不是bean的引用,因 此,value属性在这里替代通常的ref:
配置事务
配置事务管理器<bean id="transactionManager" class="org.springframework.jdbc.datasource.DataSourceTransactionManager"> <property name="dataSource" ref="dataSourceDDS"/> </bean>
使用基于注解方式配置事务
<tx:annotation-driven transaction-manager="transactionManager" proxy-target-class="true"/>
配置事务的传播特性
<tx:advice id="txAdvice" transaction-manager="transactionManager"> <tx:attributes> <!--<tx:method name="get*" isolation="DEFAULT" propagation="REQUIRED" read-only="true"/>--> <tx:method name="load*" isolation="DEFAULT" propagation="REQUIRED" read-only="false"/> <tx:method name="list*" isolation="DEFAULT" propagation="REQUIRED" read-only="false"/> <tx:method name="find*" isolation="DEFAULT" propagation="REQUIRED" read-only="false"/> <tx:method name="select*" isolation="DEFAULT" propagation="REQUIRED" read-only="false"/> <tx:method name="query*" isolation="DEFAULT" propagation="REQUIRED" read-only="false"/> <tx:method name="criteria*" isolation="DEFAULT" propagation="REQUIRED" read-only="false"/> <tx:method name="onSuccessfulAuthentication" read-only="false"/> <tx:method name="onUnsuccessfulAuthentication" read-only="false"/> <tx:method name="insert*" isolation="DEFAULT" read-only="false" propagation="REQUIRED" rollback-for="Exception"/> <tx:method name="add*" isolation="DEFAULT" read-only="false" propagation="REQUIRED" rollback-for="Exception"/> <tx:method name="create*" isolation="DEFAULT" read-only="false" propagation="REQUIRED" rollback-for="Exception"/> <tx:method name="update*" isolation="DEFAULT" read-only="false" propagation="REQUIRED" rollback-for="Exception"/> <tx:method name="modify*" isolation="DEFAULT" read-only="false" propagation="REQUIRED" rollback-for="Exception"/> <tx:method name="save*" isolation="DEFAULT" read-only="false" propagation="REQUIRED" rollback-for="Exception"/> <tx:method name="commit*" isolation="DEFAULT" read-only="false" propagation="REQUIRED" rollback-for="Exception"/> <tx:method name="del*" isolation="DEFAULT" read-only="false" propagation="REQUIRED" rollback-for="Exception"/> <tx:method name="remove*" isolation="DEFAULT" read-only="false" propagation="REQUIRED" rollback-for="Exception"/> <tx:method name="cancel*" isolation="DEFAULT" read-only="false" propagation="REQUIRED" rollback-for="Exception"/> <tx:method name="approve*" isolation="DEFAULT" read-only="false" propagation="REQUIRED" rollback-for="Exception"/> <tx:method name="propose*" isolation="DEFAULT" read-only="false" propagation="REQUIRED" rollback-for="Exception"/> <tx:method name="reback*" isolation="DEFAULT" read-only="false" propagation="REQUIRED" rollback-for="Exception"/> <tx:method name="receive*" isolation="DEFAULT" read-only="false" propagation="REQUIRED" rollback-for="Exception"/> <tx:method name="post*" isolation="DEFAULT" read-only="false" propagation="REQUIRED" rollback-for="Exception"/> <tx:method name="mark*" isolation="DEFAULT" read-only="false" propagation="REQUIRED" rollback-for="Exception"/> <tx:method name="execute*" isolation="DEFAULT" read-only="false" propagation="REQUIRED" rollback-for="Exception"/> <tx:method name="set*" isolation="DEFAULT" read-only="false" propagation="REQUIRED" rollback-for="Exception"/> <tx:method name="register*" isolation="DEFAULT" read-only="false" propagation="REQUIRED" rollback-for="Exception"/> <tx:method name="batch*" isolation="DEFAULT" read-only="false" propagation="REQUIRED" rollback-for="Exception"/> <tx:method name="confirm" isolation="DEFAULT" read-only="false" propagation="REQUIRED" rollback-for="Exception"/> <tx:method name="account*" isolation="DEFAULT" read-only="false" propagation="REQUIRED" rollback-for="Exception"/> <tx:method name="relieveGuard" isolation="DEFAULT" read-only="false" propagation="REQUIRED" rollback-for="Exception"/> <tx:method name="runAway" isolation="DEFAULT" read-only="false" propagation="REQUIRED" rollback-for="Exception"/> <tx:method name="revertFactory" isolation="DEFAULT" read-only="false" propagation="REQUIRED" rollback-for="Exception"/> </tx:attributes> </tx:advice>
配置事务拦截器拦截哪些类的哪些方法,一般设置成拦截Service
<aop:config> <aop:pointcut id="frontPointcut" expression="execution(* com.hxyz.*.service..*.*(..))"/> <aop:advisor pointcut-ref="frontPointcut" advice-ref="txAdvice"/> </aop:config>
相关文章推荐
- mybatis与spring整合(基于配置文件)
- mybatis与spring整合(基于配置文件)
- javaWeb项目SpringMVC3.2.1与Mybatis3.0.4整合实例(Mybaits-spring配置文件的几种方式)之一SqlSessionDaoSupport方式
- mybatis与spring整合(基于配置文件)(转)
- Spring整合Struts2 wel.xml中写spring核心配置文件路径问题,顺便总结下我学习SSH整合的过程
- spring mvc +mybatis整合时要配置的连接文件
- mybatis与spring整合(基于配置文件)
- Spring、Spring MVC、MyBatis整合文件配置详解
- SSM整合配置文件(Springmvc+Spring+Mybatis)
- springmvc + spring + mybatis + maven整合配置文件
- Spring、MyBatis的整合数据映射器类(UserMapper->iocContext.xml)配置文件详解
- mybatis与spring整合(基于配置文件)
- mybatis与spring整合(基于配置文件)
- spring与mybatis整合配置文件
- Struts2 Spring3 Mybatis 3 整合 相关配置文件
- spring与mybatis整合(基于配置文件)
- SSM整合配置文件(Springmvc+Spring+Mybatis)
- mybatis与spring整合(基于配置文件)
- spring-mybatis.xml配置文件,spring与mybatis整合在一起
- mybatis与spring整合(基于配置文件)