C#操作Redis存储基础
2017-05-08 14:46
375 查看
什么是Redis?
官方定义:Redis is an open source (BSD licensed), in-memory data structure store, used as a database, cache and message broker. It supports data structures such as strings, hashes, lists, sets, sorted sets with range queries, bitmaps, hyperloglogs and geospatial indexes with radius queries. Redis has built-in replication, Lua scripting, LRU eviction, transactions and different levels of on-disk persistence, and provides high availability via Redis Sentinel and automatic partitioning with Redis Cluster
中文网定义:Redis 是一个开源(BSD许可)的,内存中的数据结构存储系统,它可以用作数据库、缓存和消息中间件。 它支持多种类型的数据结构,如 字符串(strings), 散列(hashes), 列表(lists), 集合(sets), 有序集合(sorted sets) 与范围查询, bitmaps, hyperloglogs 和地理空间(geospatial) 索引半径查询。 Redis 内置了 复制(replication),LUA脚本(Lua scripting), LRU驱动事件(LRU eviction),事务(transactions) 和不同级别的 磁盘持久化(persistence), 并通过 Redis哨兵(Sentinel)和自动 分区(Cluster)提供高可用性(high availability)。
简单来说,就是开源的 先进的 Key-value形式存储的数据结构服务器。
Key可以是:string hashes lists sets sortedsets
为了保证效率,数据都是缓存在内存中,也可以周期性的把更新的数据写入到磁盘或者把修改操作写入到追加的记录文件。
我是在我的windows系统上部署的Redis服务,步骤如下:
1、下载地址:https://github.com/ServiceStack/redis-windows
解压后:
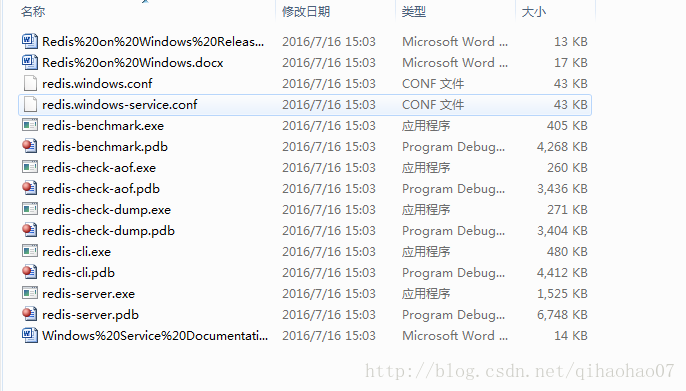
解压之后我拷到另一个我新建的文件夹MyRedis下了,
在MyRedis文件夹下 Shift+右键 选择在此处打开命令窗口 ,
输入 redis-server redis.windows.conf
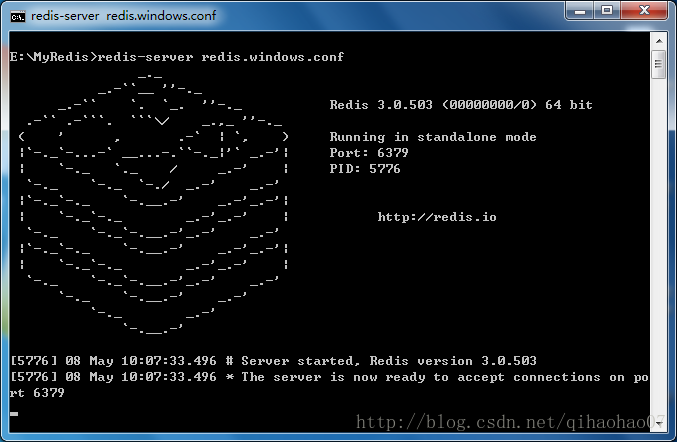
这样就启动了,但是问题又是关闭cmd窗口就会关闭Redis。
解决办法:安装到咱们系统中,作为服务运行着。
关闭这个cmd命令窗口,按上面说的重新打开这个窗口,输入命令
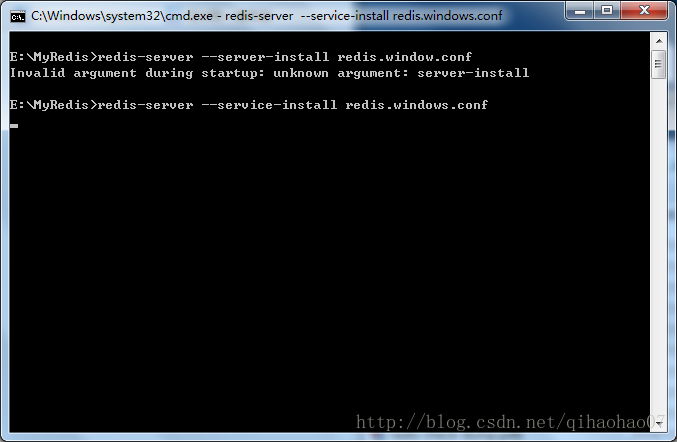
回车 提示安装,确定。
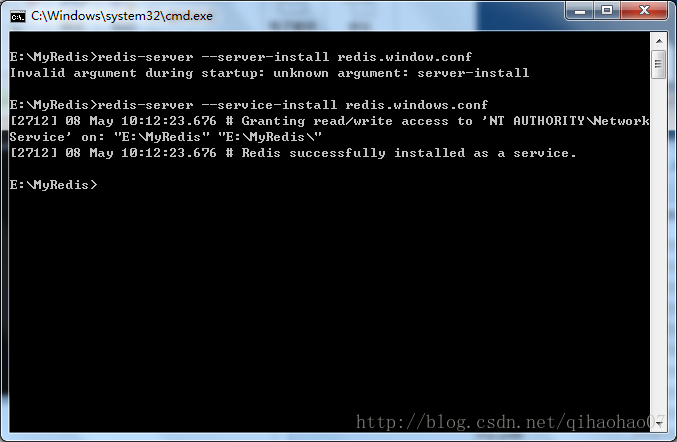
这样服务中有了,自己设置启动方式以及是否启动。
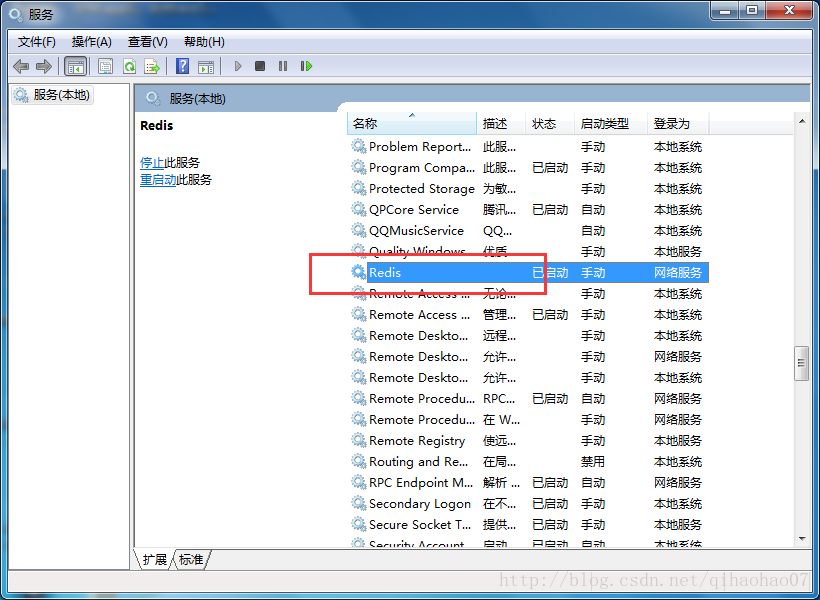
Redis启动命令如下:
1. redis-server –service-start
停止命令:
2. redis-server –service-stop
还可以安装多个实例
3. redis-server –service-install –service-name redisService1 –port 10001
4. redis-server –service-start –service-name redisService1
5. redis-server –service-install –service-name redisService2 –port 10002
6. redis-server –service-start –service-name redisService2
7. redis-server –service-install –service-name redisService3 –port 10003
8. redis-server –service-start –service-name redisService3
卸载命令:
9. redis-server –service-uninstall
安装完毕,C#操作代码用winform写的,非常简单如下:
官方定义:Redis is an open source (BSD licensed), in-memory data structure store, used as a database, cache and message broker. It supports data structures such as strings, hashes, lists, sets, sorted sets with range queries, bitmaps, hyperloglogs and geospatial indexes with radius queries. Redis has built-in replication, Lua scripting, LRU eviction, transactions and different levels of on-disk persistence, and provides high availability via Redis Sentinel and automatic partitioning with Redis Cluster
中文网定义:Redis 是一个开源(BSD许可)的,内存中的数据结构存储系统,它可以用作数据库、缓存和消息中间件。 它支持多种类型的数据结构,如 字符串(strings), 散列(hashes), 列表(lists), 集合(sets), 有序集合(sorted sets) 与范围查询, bitmaps, hyperloglogs 和地理空间(geospatial) 索引半径查询。 Redis 内置了 复制(replication),LUA脚本(Lua scripting), LRU驱动事件(LRU eviction),事务(transactions) 和不同级别的 磁盘持久化(persistence), 并通过 Redis哨兵(Sentinel)和自动 分区(Cluster)提供高可用性(high availability)。
简单来说,就是开源的 先进的 Key-value形式存储的数据结构服务器。
Key可以是:string hashes lists sets sortedsets
为了保证效率,数据都是缓存在内存中,也可以周期性的把更新的数据写入到磁盘或者把修改操作写入到追加的记录文件。
我是在我的windows系统上部署的Redis服务,步骤如下:
1、下载地址:https://github.com/ServiceStack/redis-windows
解压后:
解压之后我拷到另一个我新建的文件夹MyRedis下了,
在MyRedis文件夹下 Shift+右键 选择在此处打开命令窗口 ,
输入 redis-server redis.windows.conf
这样就启动了,但是问题又是关闭cmd窗口就会关闭Redis。
解决办法:安装到咱们系统中,作为服务运行着。
关闭这个cmd命令窗口,按上面说的重新打开这个窗口,输入命令
回车 提示安装,确定。
这样服务中有了,自己设置启动方式以及是否启动。
Redis启动命令如下:
1. redis-server –service-start
停止命令:
2. redis-server –service-stop
还可以安装多个实例
3. redis-server –service-install –service-name redisService1 –port 10001
4. redis-server –service-start –service-name redisService1
5. redis-server –service-install –service-name redisService2 –port 10002
6. redis-server –service-start –service-name redisService2
7. redis-server –service-install –service-name redisService3 –port 10003
8. redis-server –service-start –service-name redisService3
卸载命令:
9. redis-server –service-uninstall
安装完毕,C#操作代码用winform写的,非常简单如下:
using ServiceStack.Redis; using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Windows.Forms; namespace Demo01 { public partial class Form1 : Form { public Form1() { InitializeComponent(); } private void button1_Click(object sender, EventArgs e) { RedisClient client = new RedisClient("127.0.0.1", 6379); var timeOut = new TimeSpan(0, 0, 30); //AddString(client); //AddPerson(client); //Addhash(client); //AddQueue(client); //AddStack(client); //AddSet(client); //AddSortSet(client); //Union(client); //JJ(client); //CJ(client); } private static void CJ(RedisClient client) { var hashD = client.GetDifferencesFromSet("Set8001", new string[] { "Set8002" }); //[返回存在于第一个集合,但是不存在于其他集合的数据。差集] foreach (var item in hashD) { MessageBox.Show(item); } } private static void JJ(RedisClient client) { var hashG = client.GetIntersectFromSets(new string[] { "Set8001", "Set8002" }); foreach (var item in hashG) { MessageBox.Show(item); } } private static void Union(RedisClient client) { client.AddItemToSet("Set8001", "A"); client.AddItemToSet("Set8001", "B"); client.AddItemToSet("Set8001", "C"); client.AddItemToSet("Set8001", "D"); client.AddItemToSet("Set8002", "E"); client.AddItemToSet("Set8002", "F"); client.AddItemToSet("Set8002", "G"); client.AddItemToSet("Set8002", "D"); var setunion = client.GetUnionFromSets("Set8001", "Set8002"); foreach (var item in setunion) { MessageBox.Show(item); } } private static void AddSortSet(RedisClient client) { client.AddItemToSortedSet("SetSorted1001", "1.qhh"); client.AddItemToSortedSet("SetSorted1001", "2.qihaohao"); client.AddItemToSortedSet("SetSorted1001", "3.qihh"); var sortset = client.GetAllItemsFromSortedSet("SetSorted1001"); foreach (var item in sortset) { MessageBox.Show(item); } var sortset1 = client.GetRangeFromSortedSet("SetSorted1001",0,0); foreach (var item in sortset1) { MessageBox.Show(item); } var list = client.GetRangeFromSortedSetDesc("SetSorted1001", 0, 0); foreach (var item in list) { MessageBox.Show(item); } } private static void AddSet(RedisClient client) { client.AddItemToSet("Set1001", "qhh"); client.AddItemToSet("Set1001", "qihaohao"); client.AddItemToSet("Set1001", "qihh"); var set = client.GetAllItemsFromSet("Set1001"); foreach (var item in set) { MessageBox.Show(item); } } private static void AddStack(RedisClient client) { client.PushItemToList("StackListId", "1.qhh"); //入栈 client.PushItemToList("StackListId", "2.qihaohao"); client.PushItemToList("StackListId", "3.qihh"); var stackCount = client.GetListCount("StackListId"); for (int i = 0; i < stackCount; i++) { MessageBox.Show(client.PopItemFromList("StackListId")); } } private static void AddQueue(RedisClient client) { client.EnqueueItemOnList("QueueListId", "1.qhh"); client.EnqueueItemOnList("QueueListId", "2.qihaohao"); client.EnqueueItemOnList("QueueListId", "3.qihh"); var queue = client.GetListCount("QueueListId"); for (int i = 0; i < queue; i++) { MessageBox.Show(client.DequeueItemFromList("QueueListId")); } } private static void Addhash(RedisClient client) { client.SetEntryInHash("HashId", "Name", "QHH"); client.SetEntryInHash("HashId", "Age", "26"); var hash = client.GetHashValues("HashId"); foreach (var item in hash) { MessageBox.Show(item); } } private static void AddPerson(RedisClient client) { var person = new Person() { Name = "qhh", Age = 26 }; client.Add("person", person); var cachePerson = client.Get<Person>("person"); MessageBox.Show("name=" + cachePerson.Name + "----age=" + cachePerson.Age); } private static void AddString(RedisClient client) { client.Add("qhh", "qihaohao"); MessageBox.Show(client.Get<string>("qhh")); } } public class Person { public string Name { get; set; } public int Age { get; set; } } }
相关文章推荐
- C#操作Redis存储基础(续一)
- VSTS 2005 写SQL Server存储过程基础 (C#)
- c#线程基础之原子操作
- C#连接Oracle数据库通过存储过程操作数据库
- C#操作数据库,分页、执行存储过程等 [二] - ADO.NET入门之中
- C#操作数据库,分页、执行存储过程等 [一] - ADO.NET入门之中
- c#存储过程基础
- c# WinForm开发 有关DataGridView控件数据库连接(存储和删除)的操作
- C#基础系列(5)-- 第一部分 基础数据类型与操作 -- 反码操作符(5)
- .Net基础:C#中对DatagridView部分常用操作
- C#文件操作基础(未完)
- C#操作Excel基础知识
- C#基础系列(4)-- 第一部分 基础数据类型与操作 -- 枚举类型与位标志(4)
- C#基础系列(3)-- 第一部分 基础数据类型与操作 -- 位运算(3)
- <转载>在C#中操作XML(基础操作)
- c#线程基础之原子操作
- C#基础系列(2)-- 第一部分 基础数据类型与操作 -- 位运算(2)
- .NET多线程编程(11)——c#线程基础的原子操作
- c#学习之基础篇(Windows应用程序 文件操作)
- 『C#基础』C#调用存储过程