【Java学习20170427】Servlet过滤器和监听器
2017-04-27 18:52
375 查看
JavaWeb
Servlet过滤器和监听器
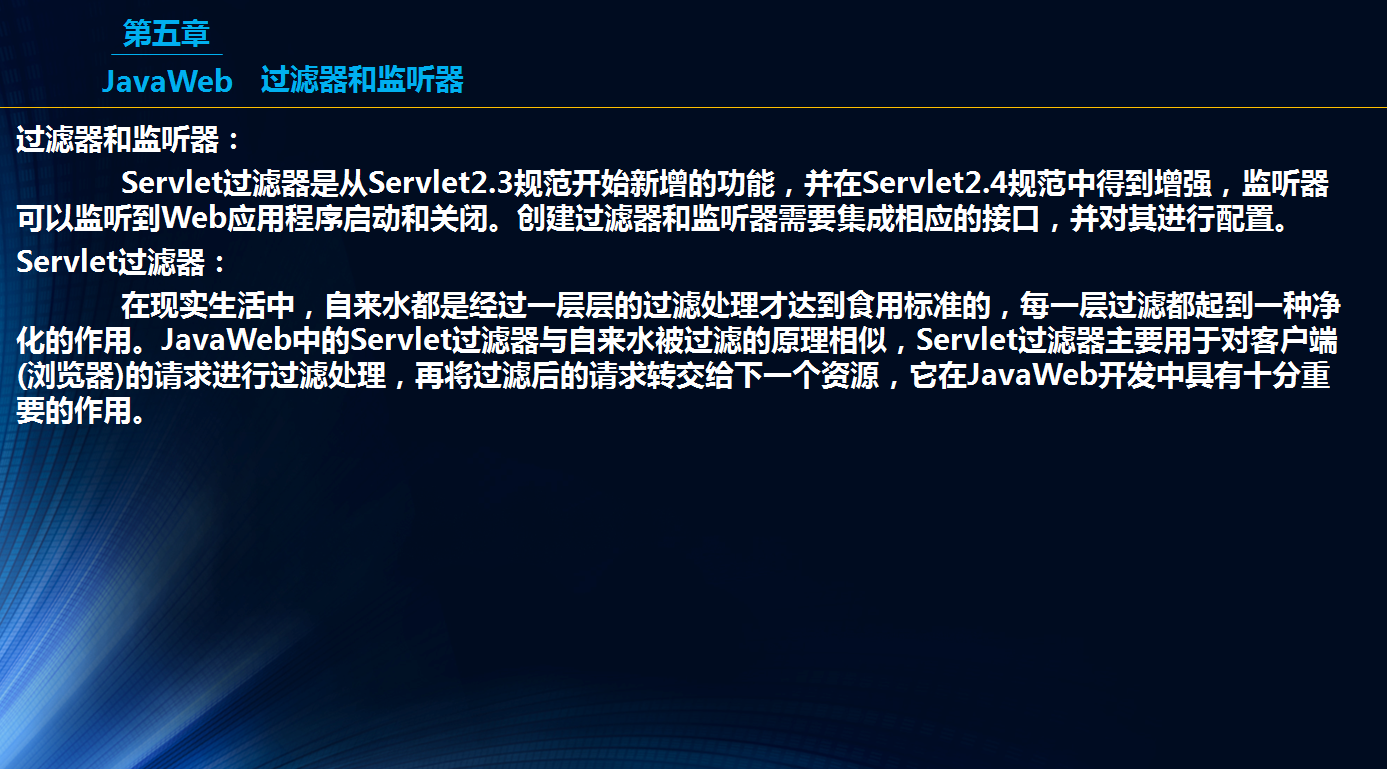
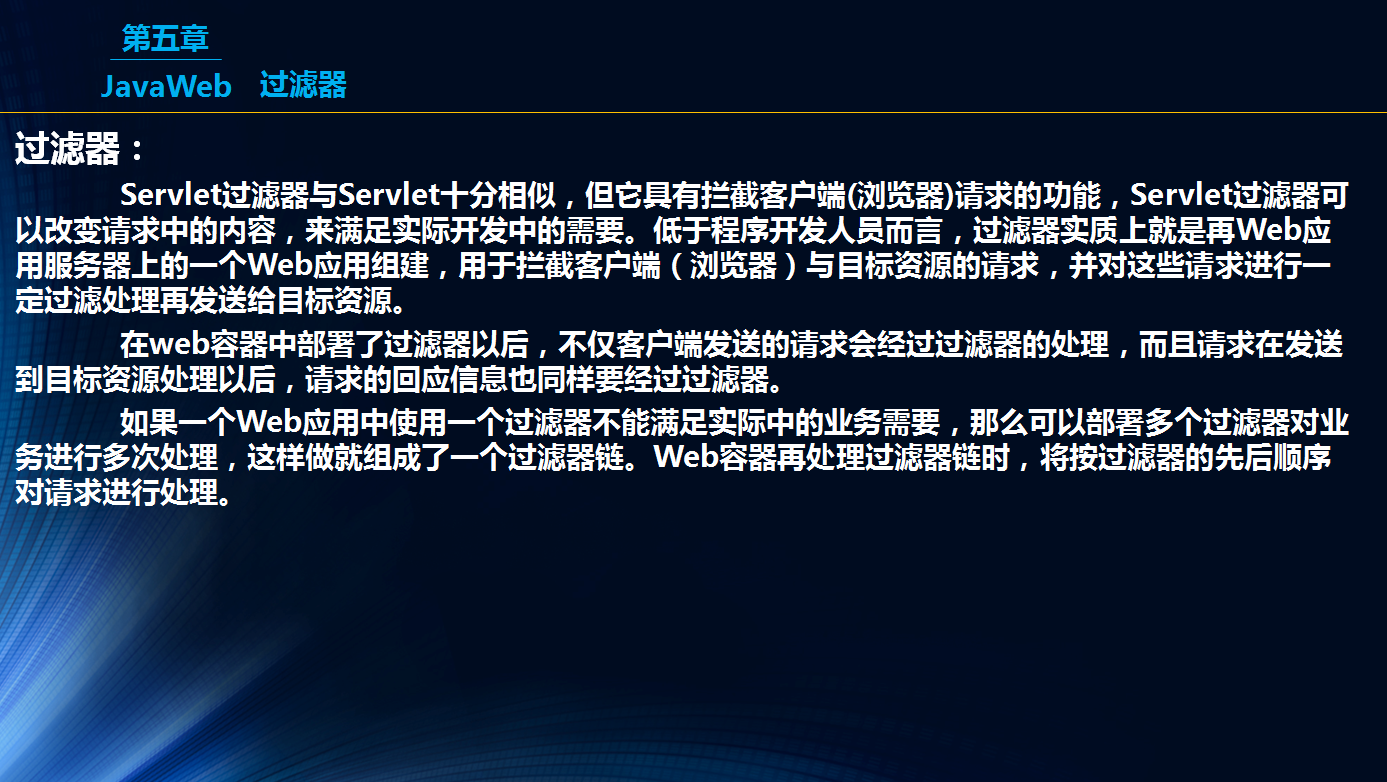
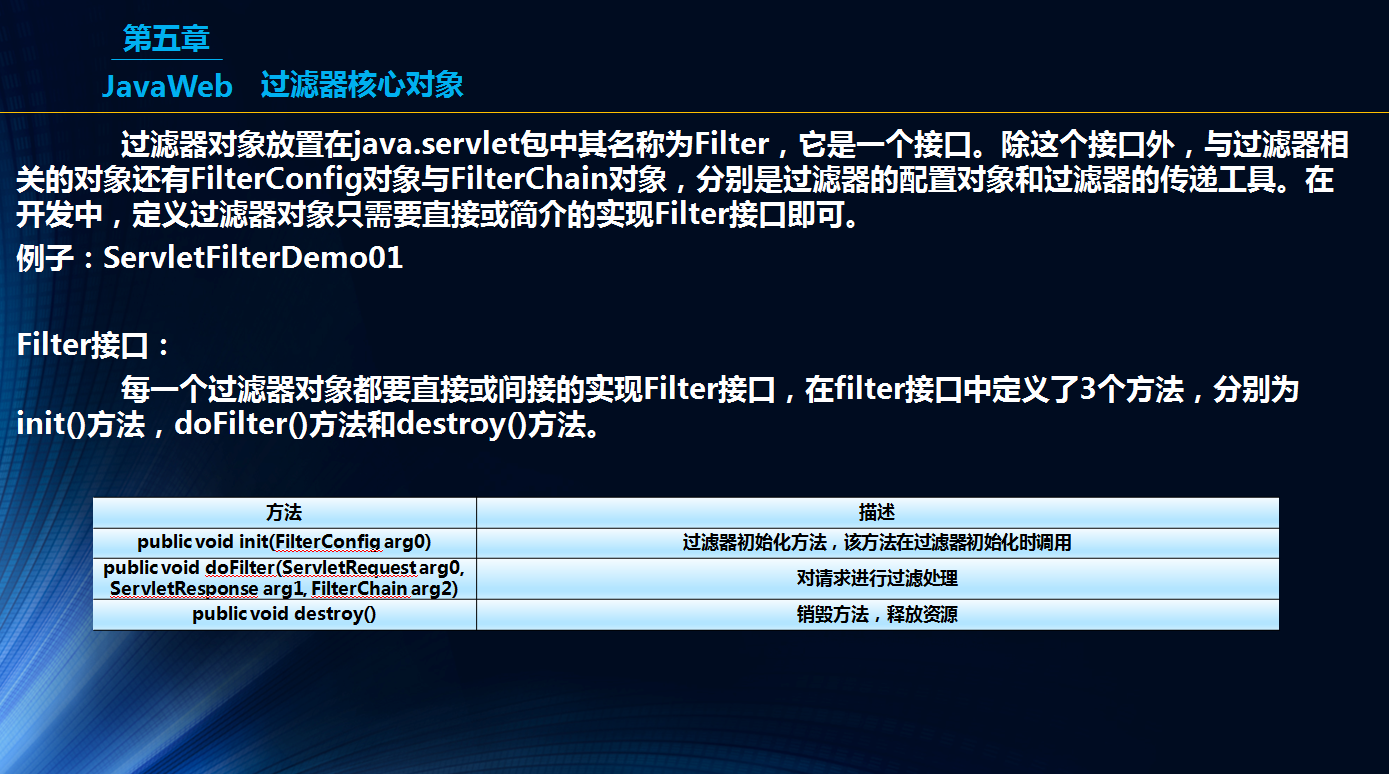
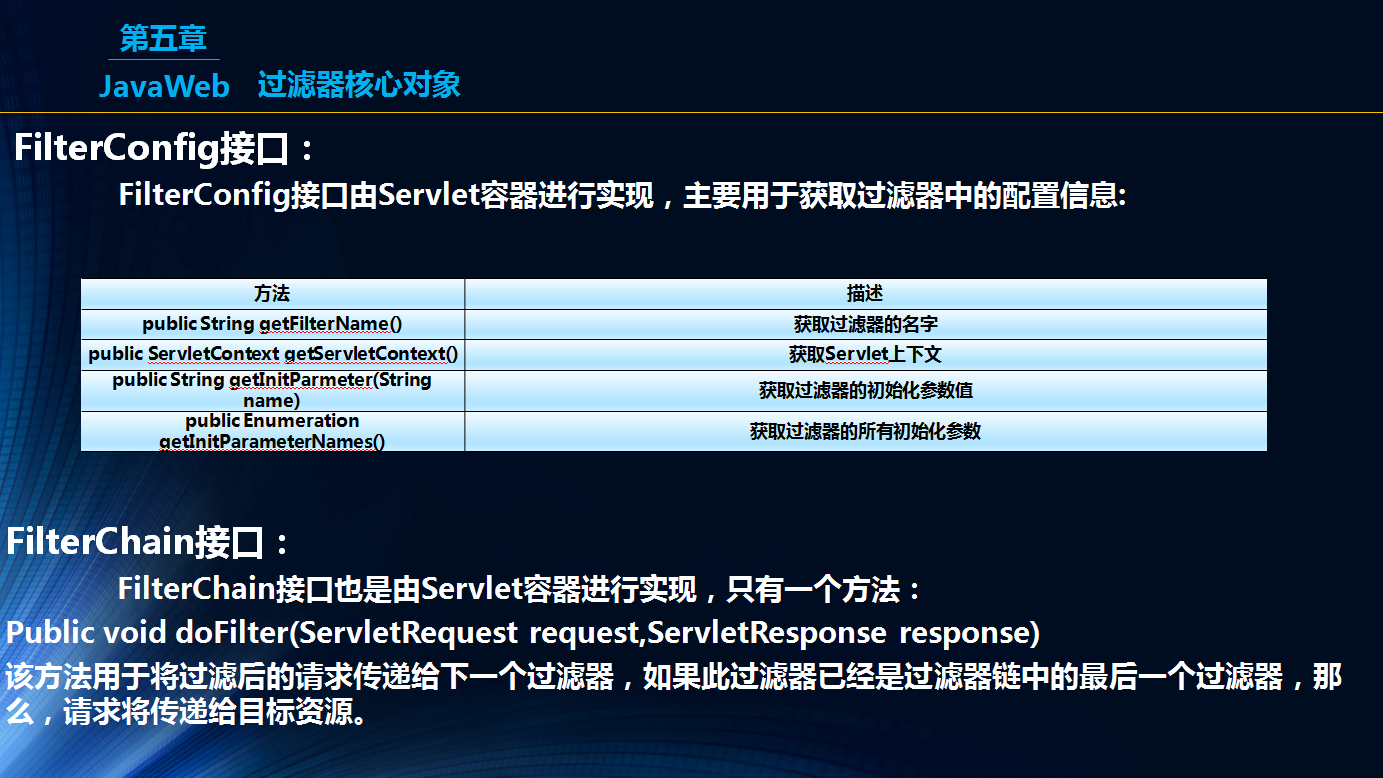
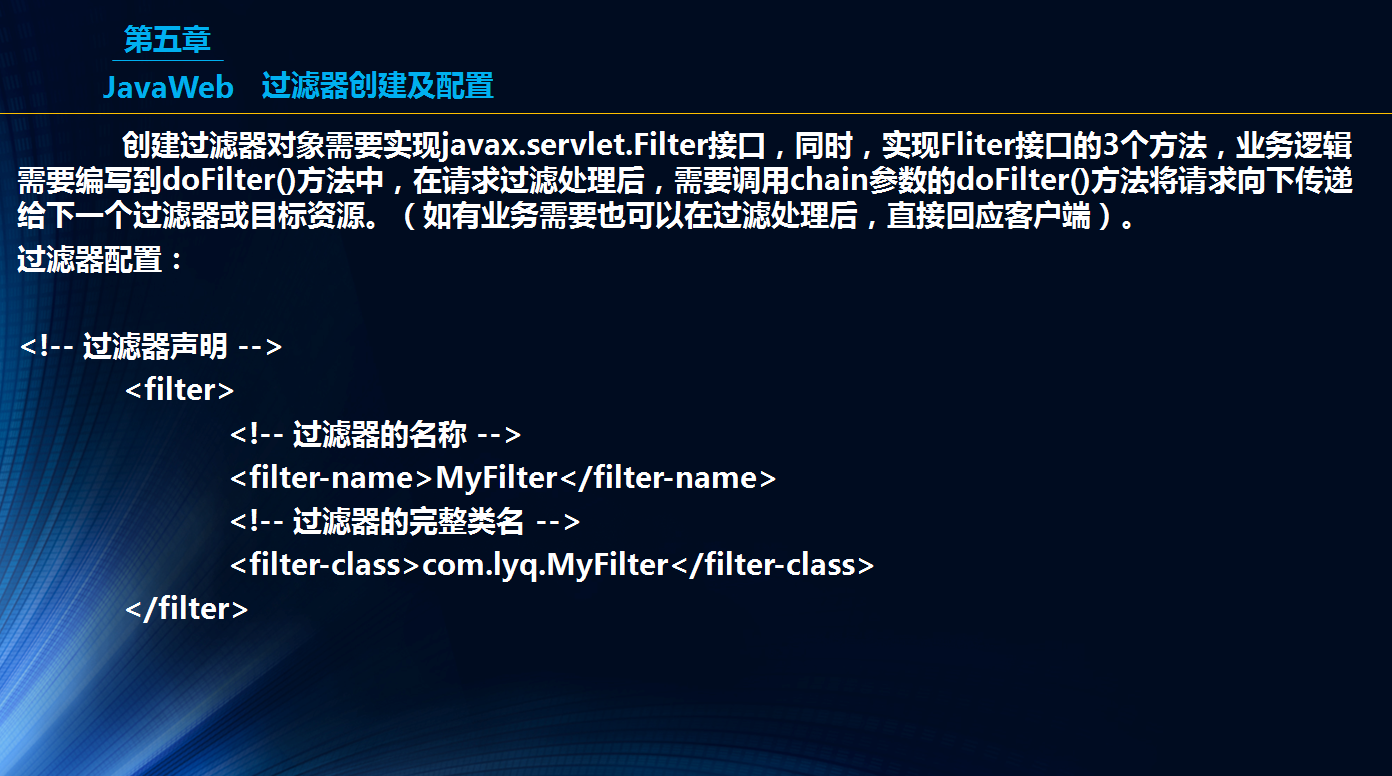
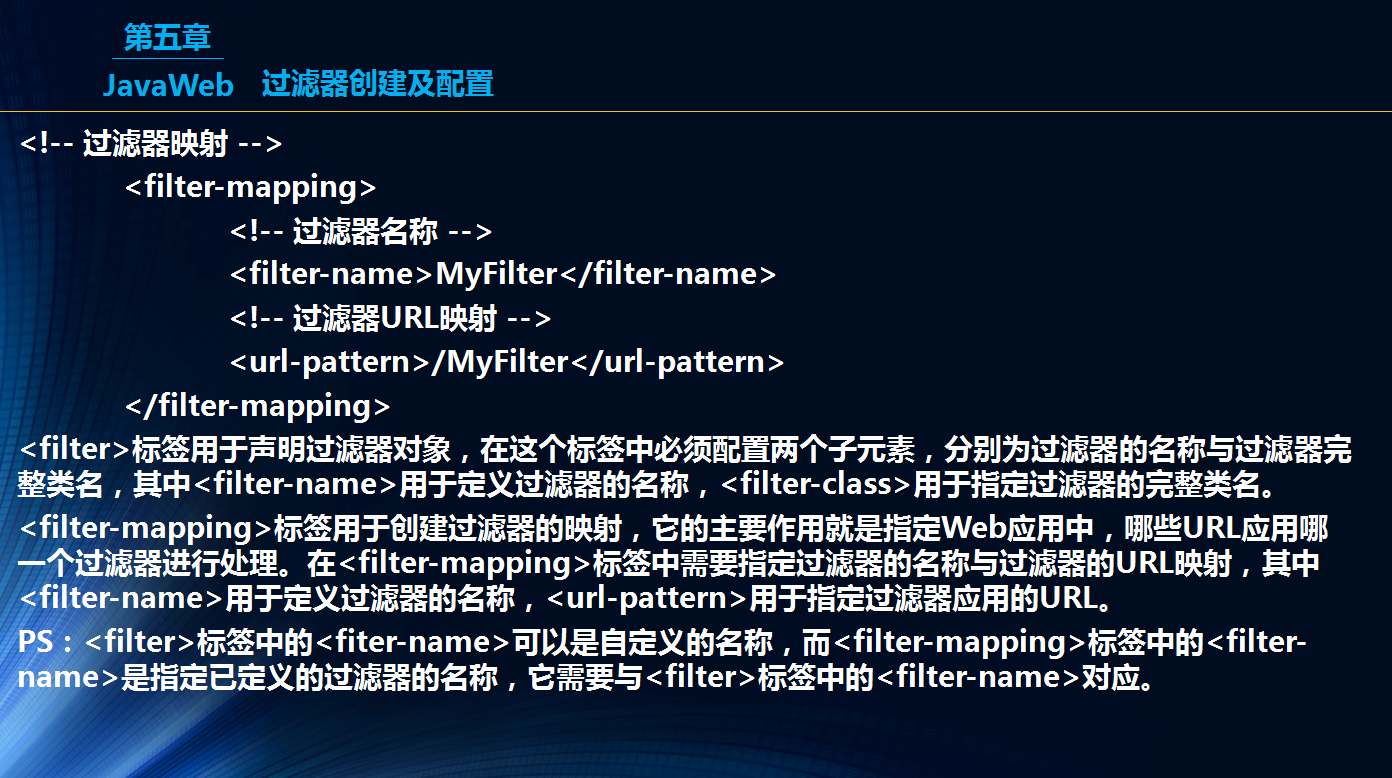
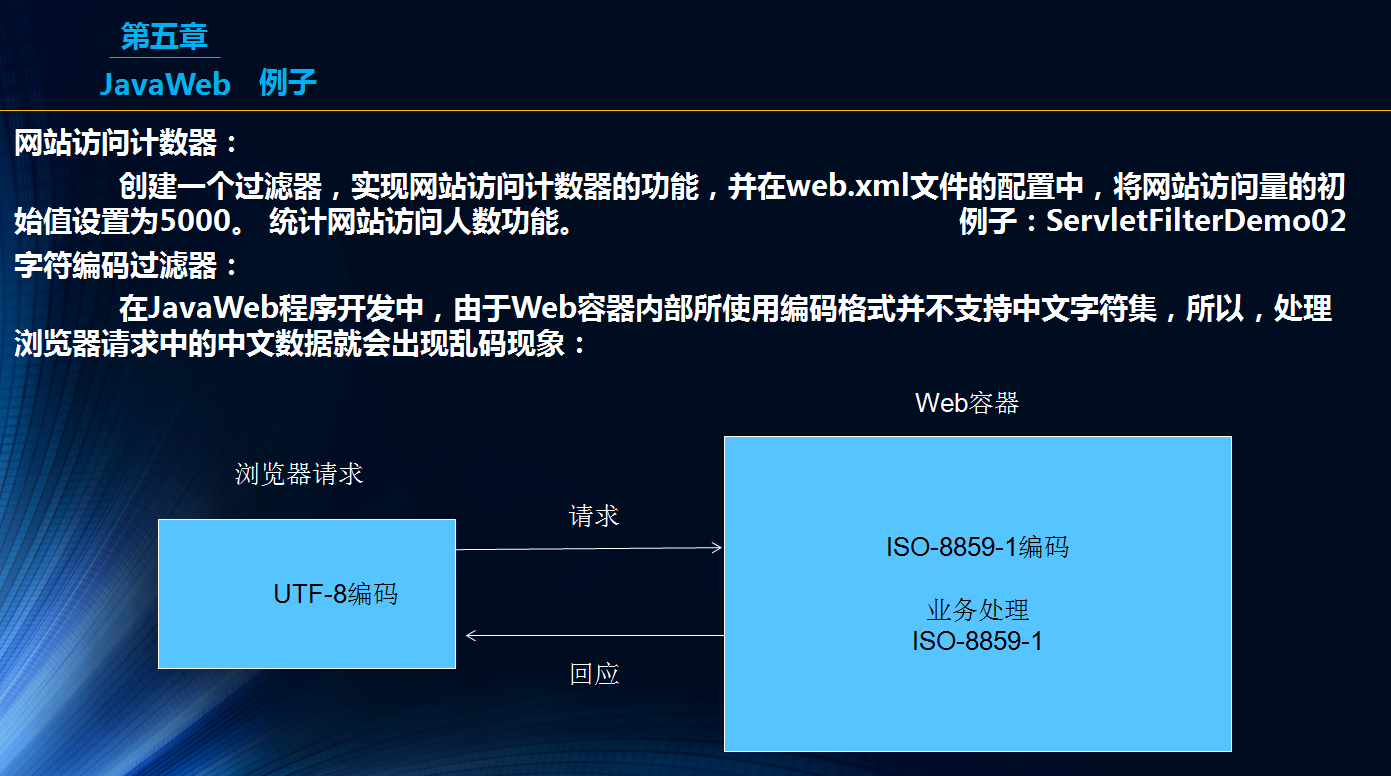
package com.tx.demo2;
import java.io.IOException;
import javax.servlet.Filter;
import javax.servlet.FilterChain;
import javax.servlet.FilterConfig;
import javax.servlet.ServletContext;
import javax.servlet.ServletException;
import javax.servlet.ServletRequest;
import javax.servlet.ServletResponse;
import javax.servlet.http.HttpServletRequest;
/**
* 统计过滤器
*/
public class CountFilter implements Filter {
// 来访数量
private int count;
@Override
public void init(FilterConfig filterConfig) throws ServletException {
// 获取初始化参数
String param = filterConfig.getInitParameter("count");
// 将字符串转换为int
count = Integer.valueOf(param);
}
@Override
public void doFilter(ServletRequest request, ServletResponse response, FilterChain chain)
throws IOException, ServletException {
// 访问数量自增
count++;
// 将ServletRequest转换成HttpServletRequest
HttpServletRequest req = (HttpServletRequest) request;
// 获取ServletContext
ServletContext context = req.getSession().getServletContext();
// 将来访数量值放入到ServletContext中
context.setAttribute("count", count);
// 向下传递过滤器
chain.doFilter(request, response);
}
@Override
public void destroy() {
}
}
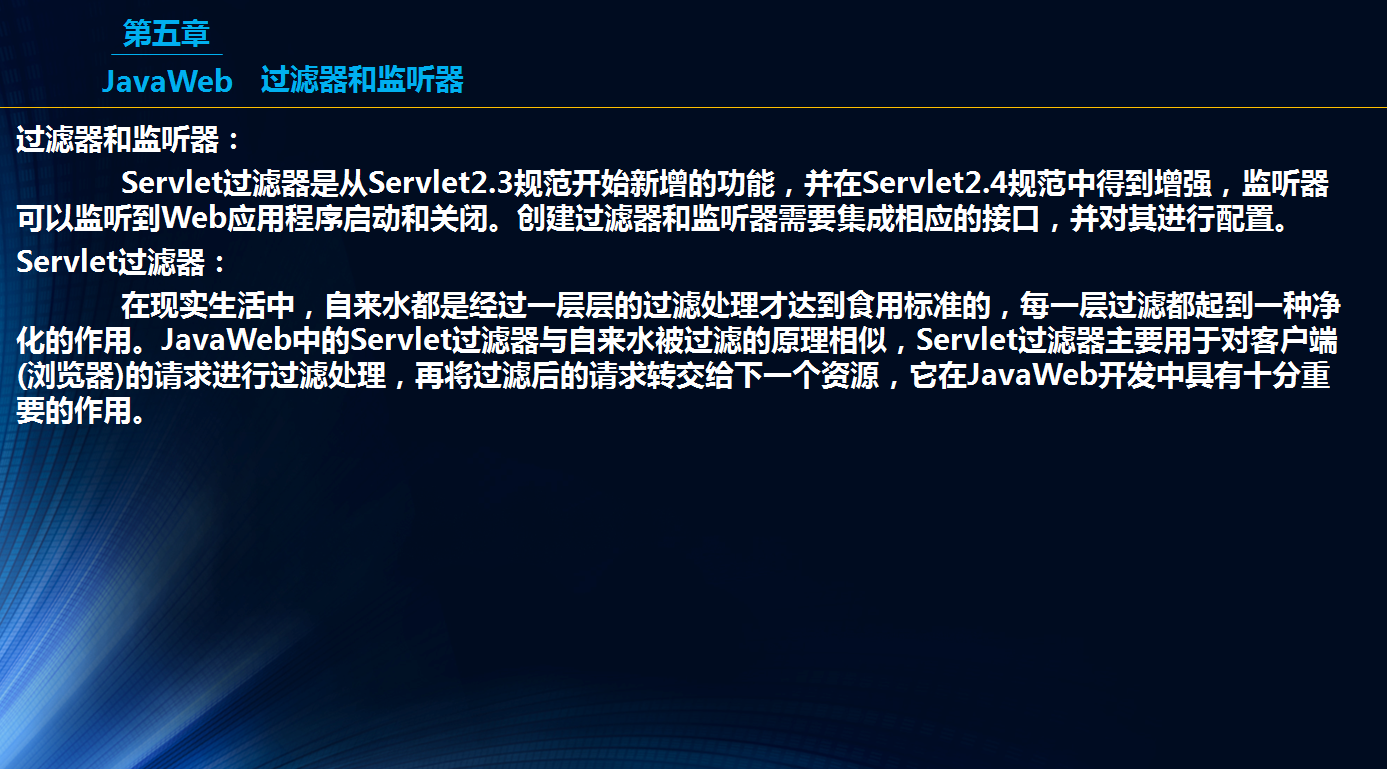
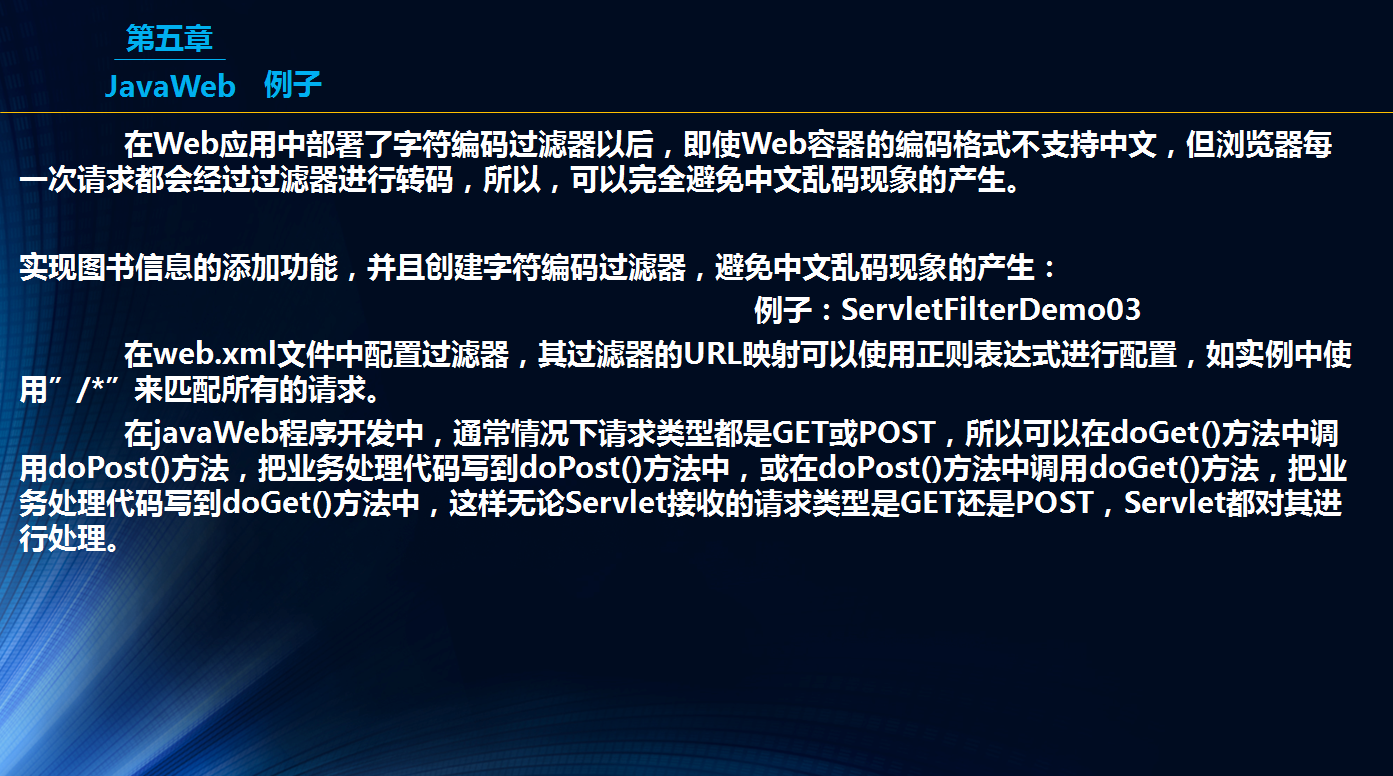
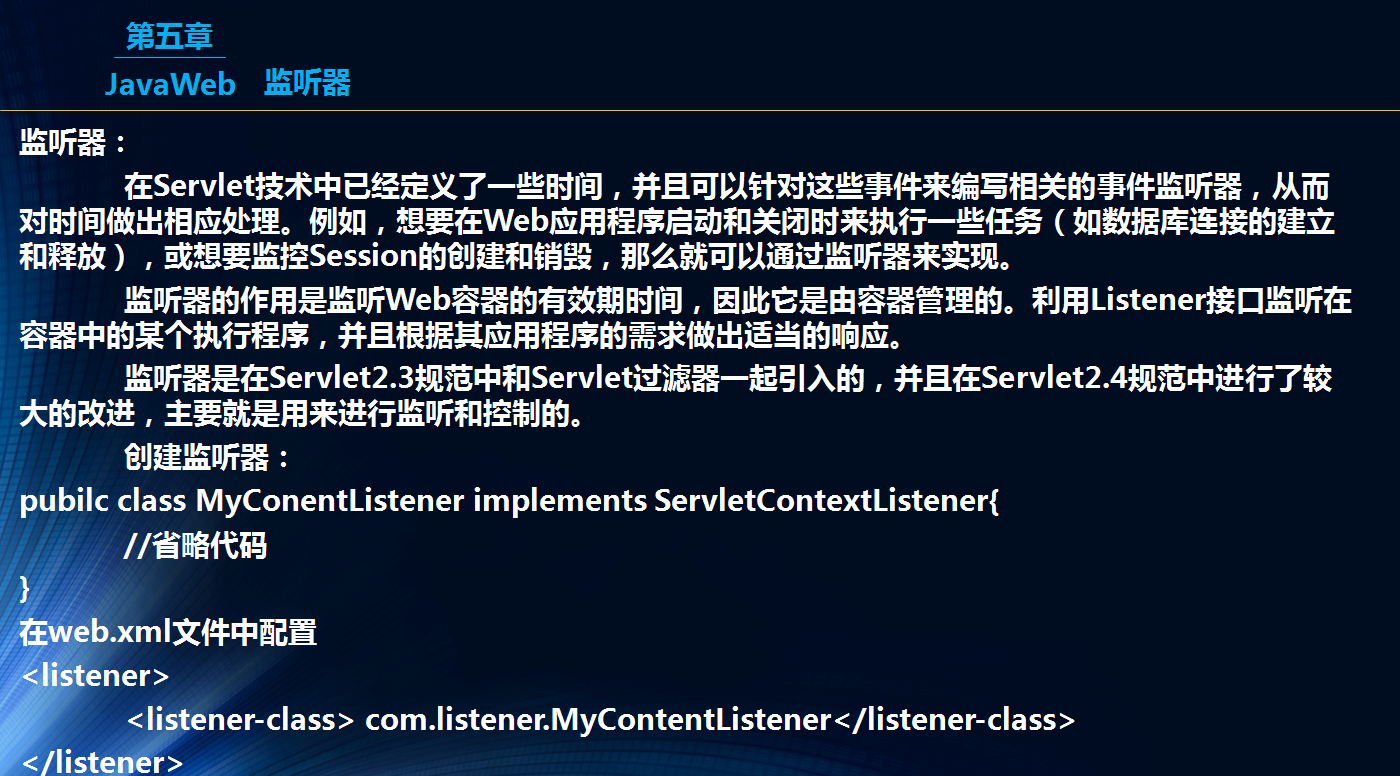
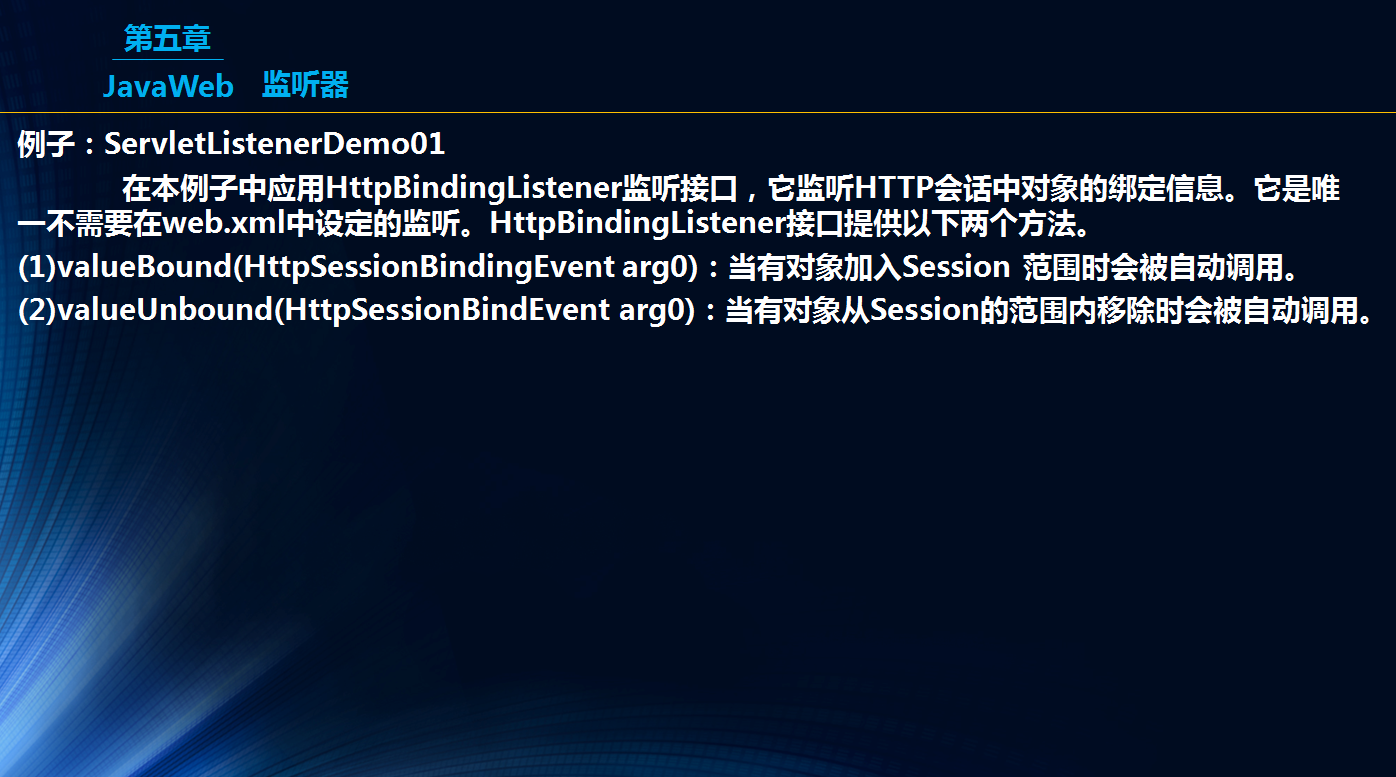
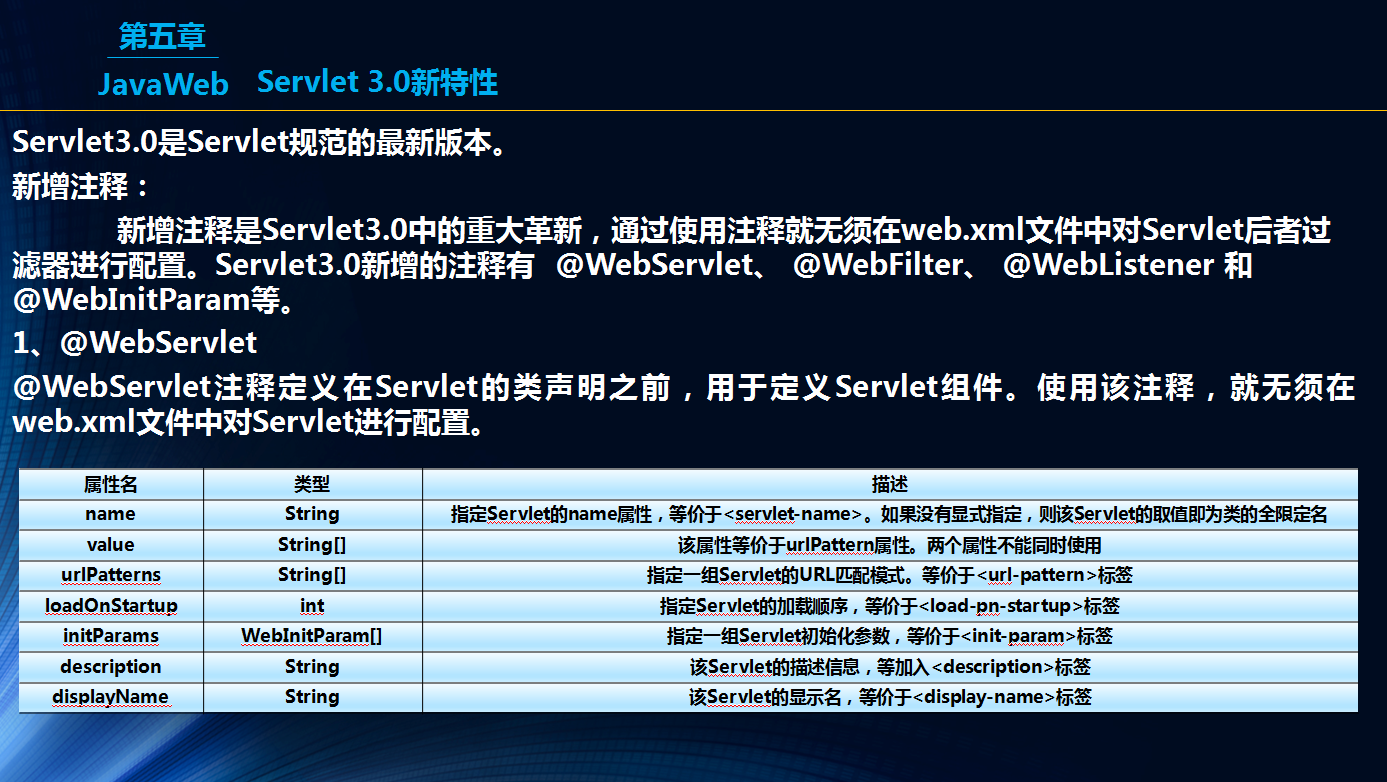
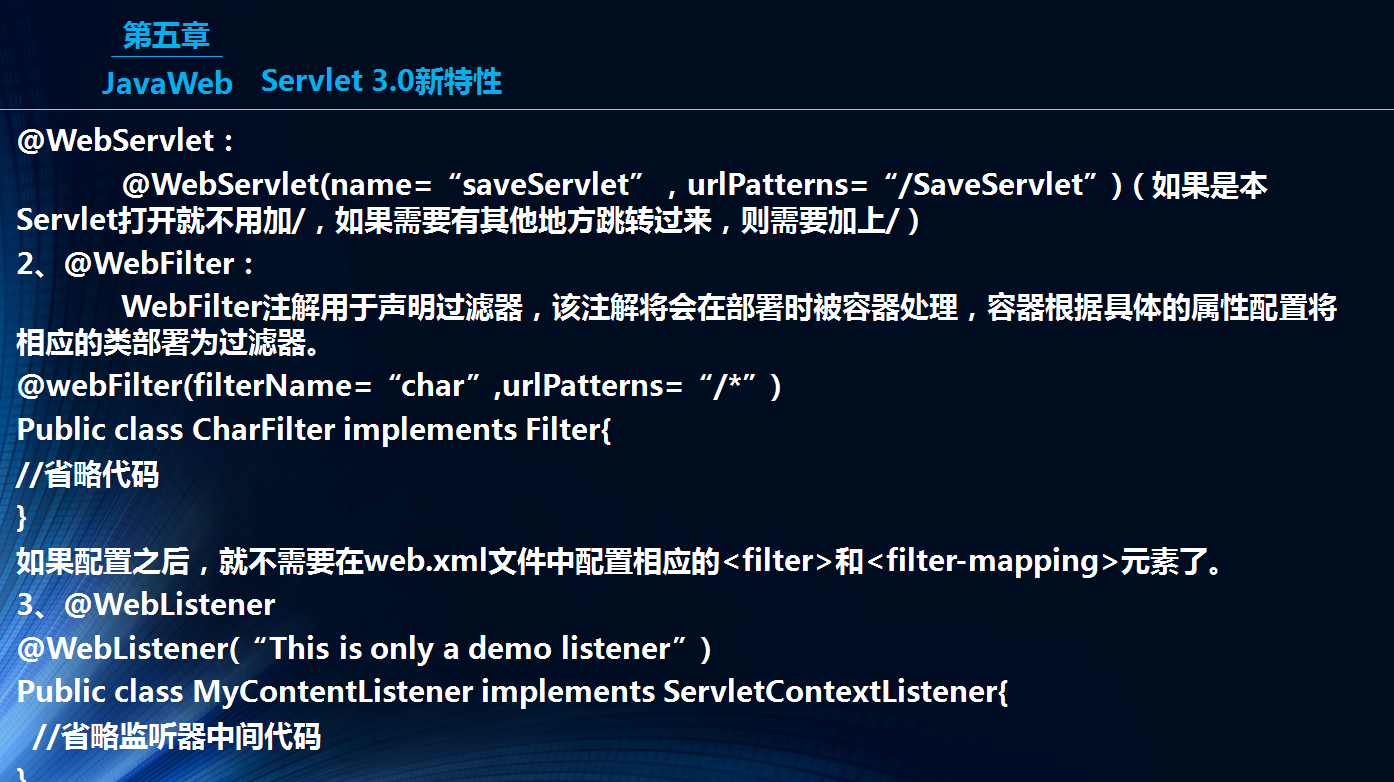
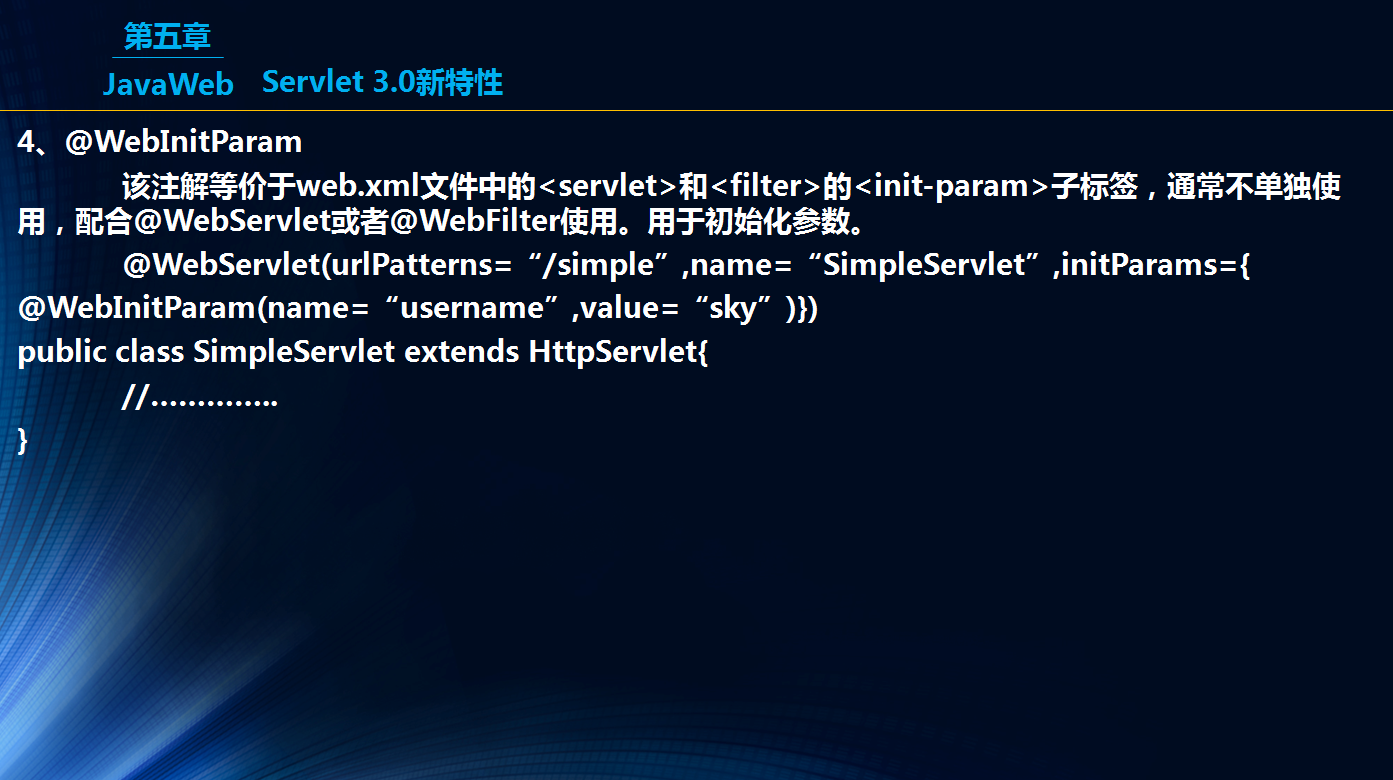
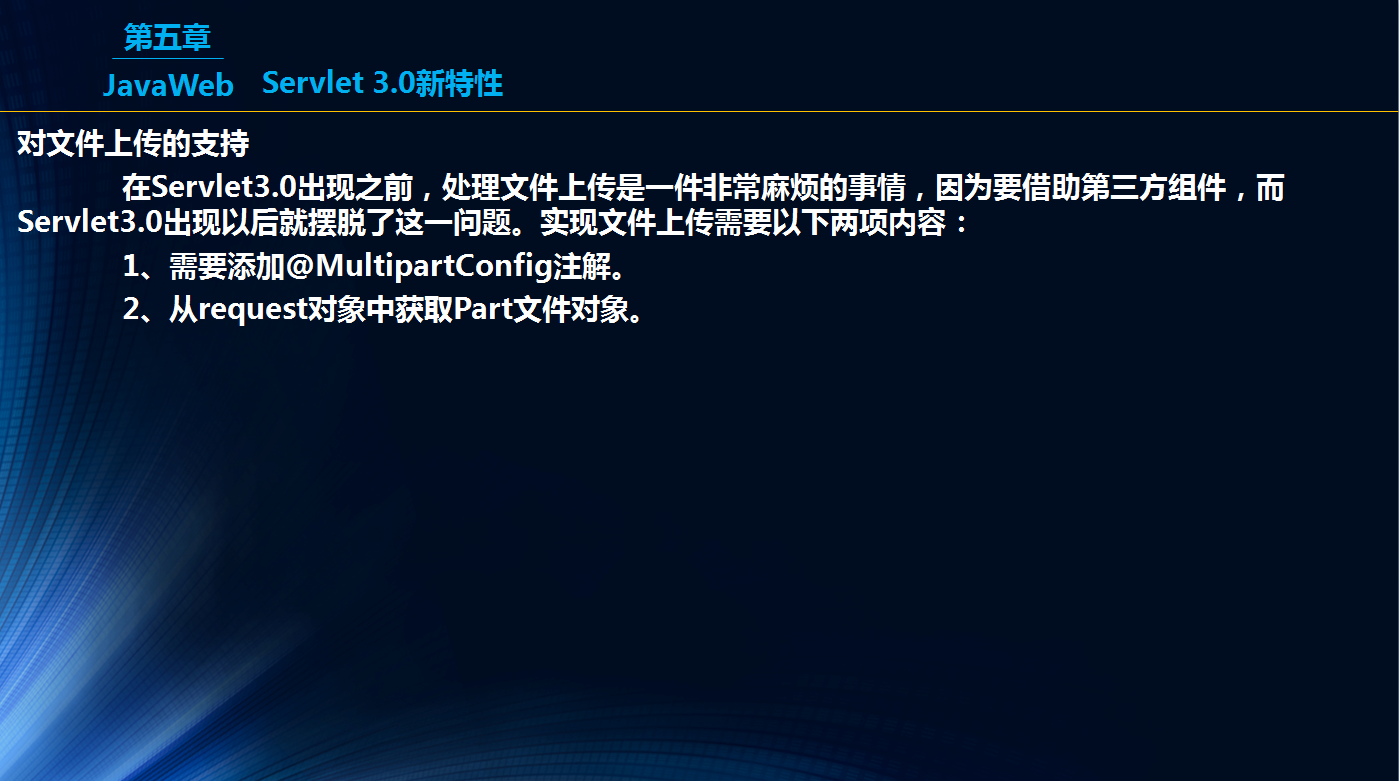
文件上传
package com.share.putfile;
import java.io.File;
import java.io.IOException;
import java.io.PrintWriter;
import javax.servlet.ServletException;
import javax.servlet.annotation.MultipartConfig;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.Part;
import org.apache.catalina.core.ApplicationPart;
/**
* Servlet implementation class UploadServlet
*/
@WebServlet("/UploadServlet")
@MultipartConfig()
public class UploadServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
protected void doPost(HttpServletRequest request,
HttpServletResponse response) throws ServletException, IOException {
request.setCharacterEncoding("UTF-8");
response.setContentType("text/html;charset=UTF-8");
PrintWriter out = response.getWriter();
String path = this.getServletContext().getRealPath("/"); //获取服务器地址
Part p = request.getPart("file1"); //获取用户选择的上传文件
if (p.getContentType().contains("image")) { // 仅处理上传的图像文件
ApplicationPart ap = (ApplicationPart) p;
System.out.println(ap.getHeaderNames());
System.out.println(ap.getHeader("content-disposition"));
// String fname1 = ap.getFilename(); //获取上传文件名
// int path_idx = fname1.lastIndexOf("\\") + 1; //对上传文件名进行截取
// String fname2 = fname1.substring(path_idx, fname1.length());
String content = ap.getHeader("content-disposition");
String fname1 = content.substring(content.lastIndexOf("\\")+1);
fname1 = fname1.substring(0, fname1.length()-1);
File file = new File(path + "/upload/" + fname1);
p.write(file.getPath()); // 写入 web 项目根路径下的upload文件夹中
out.write("文件上传成功");
}
else{
out.write("请选择图片文件!!!");
}
}
}
package com.tx.control;
import java.io.IOException;
import javax.servlet.Filter;
import javax.servlet.FilterChain;
import javax.servlet.FilterConfig;
import javax.servlet.ServletException;
import javax.servlet.ServletRequest;
import javax.servlet.ServletResponse;
import javax.servlet.annotation.WebFilter;
import javax.servlet.http.HttpServletResponse;
@WebFilter(value="/tx")
public class MyFile_1 implements Filter {
@Override
public void destroy() {
// TODO Auto-generated method stub
}
@Override
public void doFilter(ServletRequest request, ServletResponse response, FilterChain chain)
throws IOException, ServletException {
// TODO Auto-generated method stub
String people = request.getParameter("user");
if("teacher".equals(people)){
chain.doFilter(request, response);//放行
}else{
((HttpServletResponse)response).sendRedirect("/FilerWebTest3/error.html");
}
}
@Override
public void init(FilterConfig arg0) throws ServletException {
// TODO Auto-generated method stub
}
}
Filter 和Servlet实现用户权限角色验证
package com.tx.util;
import java.util.Hashtable;
public class Jurisdiction {
/** 管理员*/
private Hashtable<String,String> administrator;
/** 开发人员*/
private Hashtable<String,String> engineer;
/** 游客*/
private Hashtable<String,String> visitor;
private Hashtable<String,String> people;
//初始化代码块
{
administrator = new Hashtable<String,String>();
engineer = new Hashtable<String,String>();
visitor = new Hashtable<String,String>();
people = new Hashtable<String,String>();
init();
}
private static Jurisdiction
aeff
j;
static {
j = new Jurisdiction();
}
public static Jurisdiction getnewInstance(){
return j;
}
private Jurisdiction(){}
/**
* 初始化
*/
public void init(){
administrator.put("王二狗", "123465");
engineer.put("帅哥", "123");
visitor.put("user", "112233");
people.putAll(administrator);
people.putAll(engineer);
people.putAll(visitor);
}
/**
* 检查权限等级
* @param username
* @return
*/
public int examine(String username){
if(administrator.containsKey(username)){
return 1;
}else if(engineer.containsKey(username)){
return 2;
}else if(visitor.containsKey(username)){
return 3;
}else {
return 4;
}
}
public Hashtable<String, String> getAdministrator() {
return administrator;
}
public Hashtable<String, String> getEngineer() {
return engineer;
}
public Hashtable<String, String> getVisitor() {
return visitor;
}
public Hashtable<String, String> getPeople() {
return people;
}
}
package com.tx.action;
import java.io.IOException;
import javax.servlet.Filter;
import javax.servlet.FilterChain;
import javax.servlet.FilterConfig;
import javax.servlet.ServletException;
import javax.servlet.ServletRequest;
import javax.servlet.ServletResponse;
import javax.servlet.annotation.WebFilter;
@WebFilter(value = "/*")
public class CharactorFilter implements Filter {
@Override
public void doFilter(ServletRequest request, ServletResponse response, FilterChain chain)
throws IOException, ServletException {
System.out.println("DoFilter");
// 设置request的编码格式
request.setCharacterEncoding("UTF-8");
// 设置response字符编码
response.setContentType("text/html; charset=UTF-8");
// 传递给下一过滤器
chain.doFilter(request, response);
}
@Override
public void init(FilterConfig filterConfig) throws ServletException {
}
@Override
public void destroy() {
}
}
以下文件放在WebRoot---WEB-INF(为了防止用户根据链接拼接接入)
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
<h1 align="center">无访问权限,请联系管理员</h1>
</body>
</html>
Servlet过滤器和监听器
package com.tx.demo2;
import java.io.IOException;
import javax.servlet.Filter;
import javax.servlet.FilterChain;
import javax.servlet.FilterConfig;
import javax.servlet.ServletContext;
import javax.servlet.ServletException;
import javax.servlet.ServletRequest;
import javax.servlet.ServletResponse;
import javax.servlet.http.HttpServletRequest;
/**
* 统计过滤器
*/
public class CountFilter implements Filter {
// 来访数量
private int count;
@Override
public void init(FilterConfig filterConfig) throws ServletException {
// 获取初始化参数
String param = filterConfig.getInitParameter("count");
// 将字符串转换为int
count = Integer.valueOf(param);
}
@Override
public void doFilter(ServletRequest request, ServletResponse response, FilterChain chain)
throws IOException, ServletException {
// 访问数量自增
count++;
// 将ServletRequest转换成HttpServletRequest
HttpServletRequest req = (HttpServletRequest) request;
// 获取ServletContext
ServletContext context = req.getSession().getServletContext();
// 将来访数量值放入到ServletContext中
context.setAttribute("count", count);
// 向下传递过滤器
chain.doFilter(request, response);
}
@Override
public void destroy() {
}
}
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>人数统计</title> </head> <body> <h2> 欢迎光临,<br> 您是本站的第【 <%=application.getAttribute("count") %> 】位访客! </h2> </body> </html>
<?xml version="1.0" encoding="UTF-8"?> <web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://xmlns.jcp.org/xml/ns/javaee" xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee http://xmlns.jcp.org/xml/ns/javaee/web-app_3_1.xsd" id="WebApp_ID" version="3.1"> <display-name>FilerTest</display-name> <welcome-file-list> <welcome-file>index.html</welcome-file> <welcome-file>index.htm</welcome-file> <welcome-file>index.jsp</welcome-file> <welcome-f 4000 ile>default.html</welcome-file> <welcome-file>default.htm</welcome-file> <welcome-file>default.jsp</welcome-file> </welcome-file-list> <!-- 过滤器声明 --> <filter> <!-- 过滤器的名称 --> <filter-name>CountFilter</filter-name> <!-- 过滤器的完整类名 --> <filter-class>com.tx.demo2.CountFilter</filter-class> <!-- 设置初始化参数 --> <init-param> <!-- 参数名 --> <param-name>count</param-name> <!-- 参数值 --> <param-value>5000</param-value> </init-param> </filter> <!-- 过滤器映射 --> <filter-mapping> <!-- 过滤器名称 --> <filter-name>CountFilter</filter-name> <!-- 过滤器URL映射 --> <url-pattern>/index.jsp</url-pattern> </filter-mapping> </web-app>
package com.tx.demo3; import java.io.IOException; import java.io.PrintWriter; import javax.servlet.ServletException; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; /** * 添加图书信息的Servlet */ public class AddServlet extends HttpServlet { private static final long serialVersionUID = 1L; // 处理GET请求 protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { doPost(request, response); } // 处理POST请求 protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { // 获取 PrintWriter PrintWriter out = response.getWriter(); // 获取图书编号 String id = request.getParameter("id"); // 获取名称 String name = request.getParameter("name"); // 获取作者 String author = request.getParameter("author"); // 获取价格 String price = request.getParameter("price"); // 输出图书信息 out.print("<h2>图书信息添加成功</h2><hr>"); out.print("图书编号:" + id + "<br>"); out.print("图书名称:" + name + "<br>"); out.print("作者:" + author + "<br>"); out.print("价格:" + price + "<br>"); // 刷新流 out.flush(); // 关闭流 out.close(); } }
package com.tx.demo3; import java.io.IOException; import javax.servlet.Filter; import javax.servlet.FilterChain; import javax.servlet.FilterConfig; import javax.servlet.ServletException; import javax.servlet.ServletRequest; import javax.servlet.ServletResponse; // 字符编码过滤器 public class CharactorFilter implements Filter { // 字符编码 String encoding = null; @Override public void destroy() { encoding = null; } @Override public void doFilter(ServletRequest request, ServletResponse response, FilterChain chain) throws IOException, ServletException { // 判断字符编码是否为空 if(encoding != null){ // 设置request的编码格式 request.setCharacterEncoding(encoding); // 设置response字符编码 response.setContentType("text/html; charset="+encoding); } // 传递给下一过滤器 chain.doFilter(request, response); } @Override public void init(FilterConfig filterConfig) throws ServletException { // 获取初始化参数 encoding = filterConfig.getInitParameter("encoding"); } }
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>添加图书信息</title> </head> <body> <form action="AddServlet" method="get"> <table align="center" border="1" width="350"> <tr> <td class="2" align="center" colspan="2"> <h2>添加图书信息</h2> </td> </tr> <tr> <td align="right">图书编号:</td> <td> <input type="text" name="id"> </td> </tr> <tr> <td align="right">图书名称:</td> <td> <input type="text" name="name"> </td> </tr> <tr> <td align="right">作 者:</td> <td> <input type="text" name="author"> </td> </tr> <tr> <td align="right">价 格:</td> <td> <input type="text" name="price"> </td> </tr> <tr> <td class="2" align="center" colspan="2"> <input type="submit" value="添 加"> </td> </tr> </table> </form> </body> </html>
<?xml version="1.0" encoding="UTF-8"?> <web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://xmlns.jcp.org/xml/ns/javaee" xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee http://xmlns.jcp.org/xml/ns/javaee/web-app_3_1.xsd" id="WebApp_ID" version="3.1"> <display-name>FilerTest2</display-name> <welcome-file-list> <welcome-file>index.html</welcome-file> <welcome-file>index.htm</welcome-file> <welcome-file>index.jsp</welcome-file> <welcome-file>default.html</welcome-file> <welcome-file>default.htm</welcome-file> <welcome-file>default.jsp</welcome-file> </welcome-file-list> <!-- 声明过滤器 --> <filter> <!-- 过滤器名称 --> <filter-name>CharactorFilter</filter-name> <!-- 过滤器的完整类名 --> <filter-class>com.tx.demo3.CharactorFilter</filter-class> <!-- 初始化参数 --> <init-param> <!-- 参数名 --> <param-name>encoding</param-name> <!-- 参数值 --> <param-value>UTF-8</param-value> </init-param> </filter> <!-- 过滤器映射 --> <filter-mapping> <!-- 过滤器名称 --> <filter-name>CharactorFilter</filter-name> <!-- URL映射 --> <url-pattern>/*</url-pattern> </filter-mapping> <!-- 声明Servlet --> <servlet> <!-- Servlet名称 --> <servlet-name>AddServlet</servlet-name> <!-- Servlet完整类名 --> <servlet-class>com.tx.demo3.AddServlet</servlet-class> </servlet> <!-- Servlet映射 --> <servlet-mapping> <!-- Servlet名称 --> <servlet-name>AddServlet</servlet-name> <!-- URL映射 --> <url-pattern>/AddServlet</url-pattern> </servlet-mapping> </web-app>
package com.tx.listener; import java.util.*; public class LoginList { private static LoginList user = new LoginList(); private Vector<String> vector = null; // private调用构造函数, // 防止被外界类调用产生新的instance对象 private LoginList() { this.vector = new Vector<String>(); } // 外界使用的instance对象 public static LoginList getInstance() { return user; } // 用户登录 public boolean addLoginList(String user) { if (user != null) { this.vector.add(user); return true; } else { return false; } } // 获取用户列表 public Vector<String> getList() { return vector; } // 删除用户 public void removeLoginList(String user) { if (user != null) { vector.removeElement(user); } } }
package com.tx.listener; import javax.servlet.http.HttpSessionBindingEvent; import javax.servlet.http.HttpSessionBindingListener; public class LoginNote implements HttpSessionBindingListener { private String user; private LoginList container = LoginList.getInstance(); public LoginNote() { user = ""; } public void setUser(String user) { this.user = user; } public String getUser() { return this.user; } public void valueBound(HttpSessionBindingEvent arg0) { System.out.println(this.user + "该用户己经上线"); } public void valueUnbound(HttpSessionBindingEvent arg0) { System.out.println(this.user + "该用户己经下线"); if (user != "") { container.removeLoginList(user); } } }
文件上传
package com.share.putfile;
import java.io.File;
import java.io.IOException;
import java.io.PrintWriter;
import javax.servlet.ServletException;
import javax.servlet.annotation.MultipartConfig;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.Part;
import org.apache.catalina.core.ApplicationPart;
/**
* Servlet implementation class UploadServlet
*/
@WebServlet("/UploadServlet")
@MultipartConfig()
public class UploadServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
protected void doPost(HttpServletRequest request,
HttpServletResponse response) throws ServletException, IOException {
request.setCharacterEncoding("UTF-8");
response.setContentType("text/html;charset=UTF-8");
PrintWriter out = response.getWriter();
String path = this.getServletContext().getRealPath("/"); //获取服务器地址
Part p = request.getPart("file1"); //获取用户选择的上传文件
if (p.getContentType().contains("image")) { // 仅处理上传的图像文件
ApplicationPart ap = (ApplicationPart) p;
System.out.println(ap.getHeaderNames());
System.out.println(ap.getHeader("content-disposition"));
// String fname1 = ap.getFilename(); //获取上传文件名
// int path_idx = fname1.lastIndexOf("\\") + 1; //对上传文件名进行截取
// String fname2 = fname1.substring(path_idx, fname1.length());
String content = ap.getHeader("content-disposition");
String fname1 = content.substring(content.lastIndexOf("\\")+1);
fname1 = fname1.substring(0, fname1.length()-1);
File file = new File(path + "/upload/" + fname1);
p.write(file.getPath()); // 写入 web 项目根路径下的upload文件夹中
out.write("文件上传成功");
}
else{
out.write("请选择图片文件!!!");
}
}
}
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Insert title here</title> </head> <body> <form action="UploadServlet" enctype="multipart/form-data" method ="post" > 选择文件<input type="file" name="file1" /> <input type="submit" name="upload" value="上传" /> </form> </body> </html>Filter 和Servlet实现用户角色验证1
package com.tx.control;
import java.io.IOException;
import javax.servlet.Filter;
import javax.servlet.FilterChain;
import javax.servlet.FilterConfig;
import javax.servlet.ServletException;
import javax.servlet.ServletRequest;
import javax.servlet.ServletResponse;
import javax.servlet.annotation.WebFilter;
import javax.servlet.http.HttpServletResponse;
@WebFilter(value="/tx")
public class MyFile_1 implements Filter {
@Override
public void destroy() {
// TODO Auto-generated method stub
}
@Override
public void doFilter(ServletRequest request, ServletResponse response, FilterChain chain)
throws IOException, ServletException {
// TODO Auto-generated method stub
String people = request.getParameter("user");
if("teacher".equals(people)){
chain.doFilter(request, response);//放行
}else{
((HttpServletResponse)response).sendRedirect("/FilerWebTest3/error.html");
}
}
@Override
public void init(FilterConfig arg0) throws ServletException {
// TODO Auto-generated method stub
}
}
package com.tx.control; import java.io.IOException; import javax.servlet.Filter; import javax.servlet.FilterChain; import javax.servlet.FilterConfig; import javax.servlet.ServletException; import javax.servlet.ServletRequest; import javax.servlet.ServletResponse; import javax.servlet.annotation.WebFilter; import javax.servlet.http.HttpServletResponse; @WebFilter(value="/tx/test.do") public class MyFile_2 implements Filter{ @Override public void destroy() { // TODO Auto-generated method stub } @Override public void doFilter(ServletRequest request, ServletResponse response, FilterChain chain) throws IOException, ServletException { // TODO Auto-generated method stub String username = request.getParameter("username"); if("tx".equals(username)){ chain.doFilter(request, response); }else{ ((HttpServletResponse)response).sendRedirect("/FilerWebTest3/error.html"); } } @Override public void init(FilterConfig arg0) throws ServletException { // TODO Auto-generated method stub } }
package com.tx.control; import java.io.IOException; import java.io.PrintWriter; import javax.servlet.ServletException; import javax.servlet.annotation.WebServlet; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; @WebServlet(value="/tx/test.do") public class MyServlet extends HttpServlet{ @Override protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { // 设置request的编码 request.setCharacterEncoding("UTF-8"); response.setContentType("text/html; charset=utf-8"); String username = request.getParameter("username"); String password = request.getParameter("password"); PrintWriter pw = response.getWriter(); pw.println("账号:"+username+"密码:"+password); pw.flush(); pw.close(); } }
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>Insert title here</title> </head> <body> <form action="tx/test.do" method="post"> <select name="user"> <option value="student">学生</option> <option value="teacher">老师</option> </select> <br/> 用户名:<input name="username" type="text" > <br> 密码: <input name="password" type="text"> <br> <input type="submit" value="登录"> </form> </body> </html>
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>Insert title here</title> </head> <body> 没有访问权限 </body> </html>
Filter 和Servlet实现用户权限角色验证
package com.tx.util;
import java.util.Hashtable;
public class Jurisdiction {
/** 管理员*/
private Hashtable<String,String> administrator;
/** 开发人员*/
private Hashtable<String,String> engineer;
/** 游客*/
private Hashtable<String,String> visitor;
private Hashtable<String,String> people;
//初始化代码块
{
administrator = new Hashtable<String,String>();
engineer = new Hashtable<String,String>();
visitor = new Hashtable<String,String>();
people = new Hashtable<String,String>();
init();
}
private static Jurisdiction
aeff
j;
static {
j = new Jurisdiction();
}
public static Jurisdiction getnewInstance(){
return j;
}
private Jurisdiction(){}
/**
* 初始化
*/
public void init(){
administrator.put("王二狗", "123465");
engineer.put("帅哥", "123");
visitor.put("user", "112233");
people.putAll(administrator);
people.putAll(engineer);
people.putAll(visitor);
}
/**
* 检查权限等级
* @param username
* @return
*/
public int examine(String username){
if(administrator.containsKey(username)){
return 1;
}else if(engineer.containsKey(username)){
return 2;
}else if(visitor.containsKey(username)){
return 3;
}else {
return 4;
}
}
public Hashtable<String, String> getAdministrator() {
return administrator;
}
public Hashtable<String, String> getEngineer() {
return engineer;
}
public Hashtable<String, String> getVisitor() {
return visitor;
}
public Hashtable<String, String> getPeople() {
return people;
}
}
package com.tx.action;
import java.io.IOException;
import javax.servlet.Filter;
import javax.servlet.FilterChain;
import javax.servlet.FilterConfig;
import javax.servlet.ServletException;
import javax.servlet.ServletRequest;
import javax.servlet.ServletResponse;
import javax.servlet.annotation.WebFilter;
@WebFilter(value = "/*")
public class CharactorFilter implements Filter {
@Override
public void doFilter(ServletRequest request, ServletResponse response, FilterChain chain)
throws IOException, ServletException {
System.out.println("DoFilter");
// 设置request的编码格式
request.setCharacterEncoding("UTF-8");
// 设置response字符编码
response.setContentType("text/html; charset=UTF-8");
// 传递给下一过滤器
chain.doFilter(request, response);
}
@Override
public void init(FilterConfig filterConfig) throws ServletException {
}
@Override
public void destroy() {
}
}
package com.tx.action; import java.io.IOException; import javax.servlet.Filter; import javax.servlet.FilterChain; import javax.servlet.FilterConfig; import javax.servlet.ServletException; import javax.servlet.ServletRequest; import javax.servlet.ServletResponse; import javax.servlet.annotation.WebFilter; import javax.servlet.http.HttpServletResponse; import com.tx.util.Jurisdiction; @WebFilter(value = "/show.do") public class MyFilter implements Filter { @Override public void destroy() { // TODO Auto-generated method stub } @Override public void doFilter(ServletRequest request, ServletResponse response, FilterChain chain) throws IOException, ServletException { Jurisdiction j = Jurisdiction.getnewInstance(); String username = request.getParameter("user"); String password = request.getParameter("password"); switch (j.examine(username)) { case 1: if (j.getAdministrator().containsValue(password)) { request.getRequestDispatcher("WEB-INF/jsp/admin.jsp").forward(request, response); } else { request.getRequestDispatcher("WEB-INF/jsp/error.html").forward(request, response); } break; case 2: if (j.getEngineer().containsValue(password)) { request.getRequestDispatcher("WEB-INF/jsp/engineer.jsp").forward(request, response); } else { request.getRequestDispatcher("WEB-INF/jsp/error.html").forward(request, response); } break; case 3: if (j.getVisitor().containsValue(password)) { request.getRequestDispatcher("WEB-INF/jsp/visitor.jsp").forward(request, response); } else { request.getRequestDispatcher("WEB-INF/jsp/error.html").forward(request, response); } break; default: request.getRequestDispatcher("WEB-INF/jsp/warning.html").forward(request, response); break; } } @Override public void init(FilterConfig arg0) throws ServletException { // TODO Auto-generated method stub } }
package com.tx.action; import java.io.IOException; import java.io.PrintWriter; import javax.servlet.ServletException; import javax.servlet.annotation.WebServlet; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; @WebServlet(value="/show.do") public class MyServlet extends HttpServlet{ @Override protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException { // TODO Auto-generated method stub doPost(req,resp); } @Override protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException { // TODO Auto-generated method stub PrintWriter pw = resp.getWriter(); pw.println("逻辑出错"); pw.flush(); pw.close(); } }
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>Insert title here</title> </head> <body> <form action="show.do" method="post" > <table align=center border="1"> <caption>欢迎来到XXX后台系统</caption> <tr> <td>账号:</td> <td><input type="text" name="user"></td> </tr> <tr> <td>密码:</td> <td><input type="password" name="password"></td> </tr> <tr align="center"> <td colspan="2"><input type="submit" value="登录"></td> </tr> </table> </form> </body> </html>
以下文件放在WebRoot---WEB-INF(为了防止用户根据链接拼接接入)
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
<h1 align="center">无访问权限,请联系管理员</h1>
</body>
</html>
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>Insert title here</title> </head> <body> <h3 align="center">账号或密码错误</h3> </body> </html>
<%@page import="java.util.Map"%> <%@page import="java.util.Set"%> <%@page import="java.util.Hashtable"%> <%@page import="com.tx.util.Jurisdiction"%> <%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Insert title here</title> </head> <body> <table> <% Jurisdiction j = Jurisdiction.getnewInstance(); Hashtable<String, String> admin = j.getPeople(); Set<Map.Entry<String, String>> s = admin.entrySet(); for (Map.Entry<String, String> e : s) { out.println("<tr>"); out.println("<td>账号:" + e.getKey() + "</td><td>密码:" + e.getValue() + "</td>"); out.println("</tr>"); } %> <tr> <td><input type="button" value="删除"></td> </tr> </table> </body> </html>
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Insert title here</title> </head> <body> <% String name = request.getParameter("user"); String password = request.getParameter("password"); %> <table> <tr> <td> 账号:<%=name %> </td> </tr> <tr> <td> 密码:<%=password %> </td> </tr> <tr> <td> <input type="button" value="修改"> </td> </tr> </table> </body> </html>
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Insert title here</title> </head> <body> <% String name = request.getParameter("user"); String password = request.getParameter("password"); %> <table> <tr> <td> 账号:<%=name %> </td> </tr> <tr> <td> 密码:<%=password %> </td> </tr> </table> </body> </html>
相关文章推荐
- java 拦截器、过滤器、监听器 (转载,自己学习)
- 我的Java之旅 第八课 Servlet 进阶API、过滤器与监听器
- 传智播客java web 学习,Servlet事件监听器
- [学习笔记]Servlet开发(2)过滤器和监听器
- JavaServlet过滤器与监听器
- Java基础——Servlet(七)过滤器&监听器 相关
- 关于Servlet和过滤器、监听器的学习
- 我的Java之旅 第八课 Servlet 进阶API、过滤器与监听器
- Java-Web系列(四)--servlet,过滤器,监听器
- Java学习--过滤器与监听器
- java中拦截器 过滤器 监听器 、servlet原理
- J2EE与中间件 学习笔记2 Web组件 Servlet过滤器 监听器
- Java学习日记4(Servlet监听器)
- java中servlet过滤器Filter学习(看网上资料 算是自己copy理解一遍吧)
- Java学习(过滤器,监听器,拦截器)
- JavaWEB开发-Servlet监听器
- JAVA学习提高之---- FileUpload组件实现多文件上传(JSP+SERVLET)实现
- servlet监听器和过滤器实例
- Servlet,JSP,过滤器和监听器,四个作用域和九个内置对象,EL表达式语言,自定义标签,JSTL
- java--监听器、过滤器