(三)Spring 之AOP 详解
2017-04-26 18:12
501 查看
第一节:AOP 简介
AOP 简介:百度百科;
面向切面编程(也叫面向方面编程):Aspect Oriented Programming(AOP),是软件开发中的一个热点,也是Spring框架中的一个重要内容。利用AOP 可以对业务逻辑的各个部分进行隔离,从而使得业务逻辑各部分之间的耦合度降低,提高程序的可重用性,同时提高了开发的效率。
主要的功能是:日志记录,性能统计,安全控制,事务处理,异常处理等等。
--------
这里我先开头讲一个例子代码程序:
T.java:
package com.wishwzp.test; import org.junit.Before; import org.junit.Test; import org.springframework.context.ApplicationContext; import org.springframework.context.support.ClassPathXmlApplicationContext; import com.wishwzp.service.StudentService; public class T { private ApplicationContext ac; @Before public void setUp() throws Exception { ac=new ClassPathXmlApplicationContext("beans.xml"); } @Test public void test1() { StudentService studentService=(StudentService)ac.getBean("studentService"); studentService.addStudent("张三"); } }
beans.xml:
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd"> <bean id="studentService" class="com.wishwzp.service.impl.StudentServiceImpl"></bean> </beans>
StudentServiceImpl.java:
package com.wishwzp.service.impl; import com.wishwzp.service.StudentService; public class StudentServiceImpl implements StudentService{ @Override public void addStudent(String name) { System.out.println("开始添加学生"+name); System.out.println("添加学生"+name); System.out.println("完成学生"+name+"的添加"); } }
StudentService.java:
package com.wishwzp.service; public interface StudentService { public void addStudent(String name); }
运行结果显示:
开始添加学生张三
添加学生张三
完成学生张三的添加
--------------
第二节:Spring AOP 实例
1,前置通知;2,后置通知;
3,环绕通知;
4,返回通知;
5,异常通知;
1,前置通知;
T.java:
package com.wishwzp.test; import org.springframework.context.ApplicationContext; import org.springframework.context.support.ClassPathXmlApplicationContext; import com.wishwzp.service.StudentService; public class T { public static void main(String[] args) { ApplicationContext ac=new ClassPathXmlApplicationContext("beans.xml"); StudentService studentService=(StudentService)ac.getBean("studentService"); studentService.addStudent("张三"); } }
beans.xml:
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:aop="http://www.springframework.org/schema/aop" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop.xsd"> <!-- 注入bean,StudentServiceAspect --> <bean id="studentServiceAspect" class="com.wishwzp.advice.StudentServiceAspect"></bean> <!-- 注入bean,StudentServiceImpl --> <bean id="studentService" class="com.wishwzp.service.impl.StudentServiceImpl"></bean> <!-- 配置AOP --> <aop:config> <aop:aspect id="studentServiceAspect" ref="studentServiceAspect"> <aop:pointcut expression="execution(* com.wishwzp.service.*.*(..))" id="businessService"/> <aop:before method="doBefore" pointcut-ref="businessService"/> </aop:aspect> </aop:config> </beans>
StudentServiceAspect.java:
package com.wishwzp.advice; import org.aspectj.lang.JoinPoint; import org.aspectj.lang.ProceedingJoinPoint; public class StudentServiceAspect { public void doBefore(JoinPoint jp){ System.out.println("类名:"+jp.getTarget().getClass().getName()); System.out.println("方法名:"+jp.getSignature().getName()); System.out.println("开始添加学生:"+jp.getArgs()[0]); } }
StudentServiceImpl.java:
package com.wishwzp.service.impl; import com.wishwzp.service.StudentService; public class StudentServiceImpl implements StudentService{ @Override public void addStudent(String name) { // System.out.println("开始添加学生"+name); System.out.println("添加学生"+name); // System.out.println("完成学生"+name+"的添加"); } }
StudentService.java:
package com.wishwzp.service; public interface StudentService { public void addStudent(String name); }
运行结果显示:
类名:com.wishwzp.service.impl.StudentServiceImpl
方法名:addStudent
开始添加学生:张三
添加学生张三
2,后置通知;
T.java:
package com.wishwzp.test; import org.springframework.context.ApplicationContext; import org.springframework.context.support.ClassPathXmlApplicationContext; import com.wishwzp.service.StudentService; public class T { public static void main(String[] args) { ApplicationContext ac=new ClassPathXmlApplicationContext("beans.xml"); StudentService studentService=(StudentService)ac.getBean("studentService"); studentService.addStudent("张三"); } }
beans.xml:
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:aop="http://www.springframework.org/schema/aop" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop.xsd"> <!-- 注入bean,StudentServiceAspect --> <bean id="studentServiceAspect" class="com.wishwzp.advice.StudentServiceAspect"></bean> <!-- 注入bean,StudentServiceImpl --> <bean id="studentService" class="com.wishwzp.service.impl.StudentServiceImpl"></bean> <!-- 配置AOP --> <aop:config> <aop:aspect id="studentServiceAspect" ref="studentServiceAspect"> <!-- 配置执行切点路径和id --> <aop:pointcut expression="execution(* com.wishwzp.service.*.*(..))" id="businessService"/> <!-- 前置通知 --> <aop:before method="doBefore" pointcut-ref="businessService"/> <!-- 后置通知 --> <aop:after method="doAfter" pointcut-ref="businessService"/> </aop:aspect> </aop:config> </beans>
StudentServiceAspect.java:
package com.wishwzp.advice; import org.aspectj.lang.JoinPoint; import org.aspectj.lang.ProceedingJoinPoint; public class StudentServiceAspect { //前置通知 public void doBefore(JoinPoint jp){ System.out.println("类名:"+jp.getTarget().getClass().getName()); System.out.println("方法名:"+jp.getSignature().getName()); System.out.println("开始添加学生:"+jp.getArgs()[0]); } //后置通知 public void doAfter(JoinPoint jp){ System.out.println("类名:"+jp.getTarget().getClass().getName()); System.out.println("方法名:"+jp.getSignature().getName()); System.out.println("学生添加完成:"+jp.getArgs()[0]); } }
StudentServiceImpl.java:
package com.wishwzp.service.impl; import com.wishwzp.service.StudentService; public class StudentServiceImpl implements StudentService{ @Override public void addStudent(String name) { // System.out.println("开始添加学生"+name); System.out.println("添加学生"+name); // System.out.println("完成学生"+name+"的添加"); } }
StudentService.java:
package com.wishwzp.service; public interface StudentService { public void addStudent(String name); }
运行结果显示:
类名:com.wishwzp.service.impl.StudentServiceImpl
方法名:addStudent
开始添加学生:张三
添加学生张三
类名:com.wishwzp.service.impl.StudentServiceImpl
方法名:addStudent
学生添加完成:张三
3,环绕通知;
T.java:
package com.wishwzp.test; import org.springframework.context.ApplicationContext; import org.springframework.context.support.ClassPathXmlApplicationContext; import com.wishwzp.service.StudentService; public class T { public static void main(String[] args) { ApplicationContext ac=new ClassPathXmlApplicationContext("beans.xml"); StudentService studentService=(StudentService)ac.getBean("studentService"); studentService.addStudent("张三"); } }
beans.xml:
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:aop="http://www.springframework.org/schema/aop" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop.xsd"> <!-- 注入bean,StudentServiceAspect --> <bean id="studentServiceAspect" class="com.wishwzp.advice.StudentServiceAspect"></bean> <!-- 注入bean,StudentServiceImpl --> <bean id="studentService" class="com.wishwzp.service.impl.StudentServiceImpl"></bean> <!-- 配置AOP --> <aop:config> <aop:aspect id="studentServiceAspect" ref="studentServiceAspect"> <!-- 配置执行切点路径和id --> <aop:pointcut expression="execution(* com.wishwzp.service.*.*(..))" id="businessService"/> <!-- 前置通知 --> <aop:before method="doBefore" pointcut-ref="businessService"/> <!-- 后置通知 --> <aop:after method="doAfter" pointcut-ref="businessService"/> <!-- 环绕通知 --> <aop:around method="doAround" pointcut-ref="businessService"/> </aop:aspect> </aop:config> </beans>
StudentServiceAspect.java:
package com.wishwzp.advice; import org.aspectj.lang.JoinPoint; import org.aspectj.lang.ProceedingJoinPoint; public class StudentServiceAspect { //前置通知 public void doBefore(JoinPoint jp){ System.out.println("类名:"+jp.getTarget().getClass().getName()); System.out.println("方法名:"+jp.getSignature().getName()); System.out.println("开始添加学生:"+jp.getArgs()[0]); } //后置通知 public void doAfter(JoinPoint jp){ System.out.println("类名:"+jp.getTarget().getClass().getName()); System.out.println("方法名:"+jp.getSignature().getName()); System.out.println("学生添加完成:"+jp.getArgs()[0]); } //环绕通知,这里添加了返回值 public Object doAround(ProceedingJoinPoint pjp) throws Throwable{ System.out.println("添加学生前"); Object retVal=pjp.proceed(); System.out.println(retVal); System.out.println("添加学生后"); return retVal; } }
StudentServiceImpl.java:
package com.wishwzp.service.impl; import com.wishwzp.service.StudentService; public class StudentServiceImpl implements StudentService{ @Override public String addStudent(String name) { // System.out.println("开始添加学生"+name); System.out.println("添加学生"+name); // System.out.println("完成学生"+name+"的添加"); return name; } }
StudentService.java:
package com.wishwzp.service; public interface StudentService { public String addStudent(String name); }
运行结果显示:
类名:com.wishwzp.service.impl.StudentServiceImpl
方法名:addStudent
开始添加学生:张三
添加学生前
添加学生张三
张三
添加学生后
类名:com.wishwzp.service.impl.StudentServiceImpl
方法名:addStudent
学生添加完成:张三
4,返回通知;
T.java:
package com.wishwzp.test; import org.springframework.context.ApplicationContext; import org.springframework.context.support.ClassPathXmlApplicationContext; import com.wishwzp.service.StudentService; public class T { public static void main(String[] args) { ApplicationContext ac=new ClassPathXmlApplicationContext("beans.xml"); StudentService studentService=(StudentService)ac.getBean("studentService"); studentService.addStudent("张三"); } }
beans.xml:
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:aop="http://www.springframework.org/schema/aop" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop.xsd"> <!-- 注入bean,StudentServiceAspect --> <bean id="studentServiceAspect" class="com.wishwzp.advice.StudentServiceAspect"></bean> <!-- 注入bean,StudentServiceImpl --> <bean id="studentService" class="com.wishwzp.service.impl.StudentServiceImpl"></bean> <!-- 配置AOP --> <aop:config> <aop:aspect id="studentServiceAspect" ref="studentServiceAspect"> <!-- 配置执行切点路径和id --> <aop:pointcut expression="execution(* com.wishwzp.service.*.*(..))" id="businessService"/> <!-- 前置通知 --> <aop:before method="doBefore" pointcut-ref="businessService"/> <!-- 后置通知 --> <aop:after method="doAfter" pointcut-ref="businessService"/> <!-- 环绕通知 --> <aop:around method="doAround" pointcut-ref="businessService"/> <!-- 返回通知 --> <aop:after-returning method="doAfterReturning" pointcut-ref="businessService"/> </aop:aspect> </aop:config> </beans>
StudentServiceAspect.java:
package com.wishwzp.advice; import org.aspectj.lang.JoinPoint; import org.aspectj.lang.ProceedingJoinPoint; public class StudentServiceAspect { //前置通知 public void doBefore(JoinPoint jp){ System.out.println("类名:"+jp.getTarget().getClass().getName()); System.out.println("方法名:"+jp.getSignature().getName()); System.out.println("开始添加学生:"+jp.getArgs()[0]); } //后置通知 public void doAfter(JoinPoint jp){ System.out.println("类名:"+jp.getTarget().getClass().getName()); System.out.println("方法名:"+jp.getSignature().getName()); System.out.println("学生添加完成:"+jp.getArgs()[0]); } //环绕通知,这里添加了返回值 public Object doAround(ProceedingJoinPoint pjp) throws Throwable{ System.out.println("添加学生前"); Object retVal=pjp.proceed(); System.out.println(retVal); System.out.println("添加学生后"); return retVal; } //返回通知 public void doAfterReturning(JoinPoint jp){ System.out.println("返回通知"); } }
StudentServiceImpl.java:
package com.wishwzp.service.impl; import com.wishwzp.service.StudentService; public class StudentServiceImpl implements StudentService{ @Override public String addStudent(String name) { // System.out.println("开始添加学生"+name); System.out.println("添加学生"+name); // System.out.println("完成学生"+name+"的添加"); return name; } }
StudentService.java:
package com.wishwzp.service; public interface StudentService { public String addStudent(String name); }
运行结果显示:
类名:com.wishwzp.service.impl.StudentServiceImpl
方法名:addStudent
开始添加学生:张三
添加学生前
添加学生张三
返回通知
张三
添加学生后
类名:com.wishwzp.service.impl.StudentServiceImpl
方法名:addStudent
学生添加完成:张三
5,异常通知;
T.java:
package com.wishwzp.test; import org.springframework.context.ApplicationContext; import org.springframework.context.support.ClassPathXmlApplicationContext; import com.wishwzp.service.StudentService; public class T { public static void main(String[] args) { ApplicationContext ac=new ClassPathXmlApplicationContext("beans.xml"); StudentService studentService=(StudentService)ac.getBean("studentService"); studentService.addStudent("张三"); } }
beans.xml:
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:aop="http://www.springframework.org/schema/aop" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop.xsd"> <!-- 注入bean,StudentServiceAspect --> <bean id="studentServiceAspect" class="com.wishwzp.advice.StudentServiceAspect"></bean> <!-- 注入bean,StudentServiceImpl --> <bean id="studentService" class="com.wishwzp.service.impl.StudentServiceImpl"></bean> <!-- 配置AOP --> <aop:config> <aop:aspect id="studentServiceAspect" ref="studentServiceAspect"> <!-- 配置执行切点路径和id --> <aop:pointcut expression="execution(* com.wishwzp.service.*.*(..))" id="businessService"/> <!-- 前置通知 --> <aop:before method="doBefore" pointcut-ref="businessService"/> <!-- 后置通知 --> <aop:after method="doAfter" pointcut-ref="businessService"/> <!-- 环绕通知 --> <aop:around method="doAround" pointcut-ref="businessService"/> <!-- 返回通知 --> <aop:after-returning method="doAfterReturning" pointcut-ref="businessService"/> <!-- 异常通知 --> <aop:after-throwing method="doAfterThrowing" pointcut-ref="businessService" throwing="ex"/> </aop:aspect> </aop:config> </beans>
StudentServiceAspect.java:
package com.wishwzp.advice; import org.aspectj.lang.JoinPoint; import org.aspectj.lang.ProceedingJoinPoint; public class StudentServiceAspect { //前置通知 public void doBefore(JoinPoint jp){ System.out.println("类名:"+jp.getTarget().getClass().getName()); System.out.println("方法名:"+jp.getSignature().getName()); System.out.println("开始添加学生:"+jp.getArgs()[0]); } //后置通知 public void doAfter(JoinPoint jp){ System.out.println("类名:"+jp.getTarget().getClass().getName()); System.out.println("方法名:"+jp.getSignature().getName()); System.out.println("学生添加完成:"+jp.getArgs()[0]); } //环绕通知,这里添加了返回值 public Object doAround(ProceedingJoinPoint pjp) throws Throwable{ System.out.println("添加学生前"); Object retVal=pjp.proceed(); System.out.println(retVal); System.out.println("添加学生后"); return retVal; } //返回通知 public void doAfterReturning(JoinPoint jp){ System.out.println("返回通知"); } //异常通知 public void doAfterThrowing(JoinPoint jp,Throwable ex){ System.out.println("异常通知"); System.out.println("异常信息:"+ex.getMessage()); } }
StudentServiceImpl.java:
package com.wishwzp.service.impl; import com.wishwzp.service.StudentService; public class StudentServiceImpl implements StudentService{ @Override public String addStudent(String name) { // System.out.println("开始添加学生"+name); System.out.println("添加学生"+name); System.out.println(1/0); // System.out.println("完成学生"+name+"的添加"); return name; } }
StudentService.java:
package com.wishwzp.service; public interface StudentService { public String addStudent(String name); }
运行结果显示:
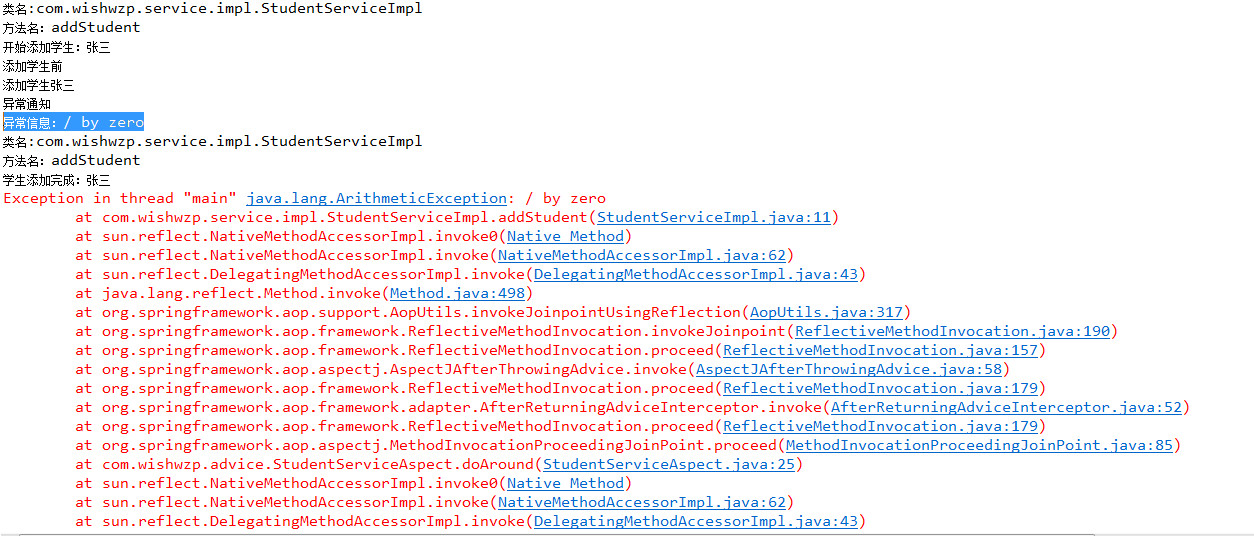
相关文章推荐
- Spring AOP注解通过@Autowired,@Resource,@Qualifier,@PostConstruct,@PreDestroy注入属性的配置文件详解
- Spring AOP 详解
- Spring AOP 详解
- Spring AOP详解 一.前言 在以前的项目中,很少去关注spring aop的具体实现与理论,只是简单了解了一下什么是aop具体怎么用,看到了一篇博文写得还不错,就转载来学习一下,博
- AOP 之 6.5 AspectJ切入点语法详解 ——跟我学spring3
- 细谈Spring(六)spring之AOP基本概念和配置详解
- Spring AOP 详解
- SSH中各个框架的作用以及Spring AOP,IOC,DI详解
- Spring AOP 详解
- 探秘SpringAop(一)_介绍以及使用详解
- Spring AOP详解
- »Spring 之AOP AspectJ切入点语法详解(最全了,不需要再去其他地找了)
- »Spring 之AOP AspectJ切入点语法详解(最全了,不需要再去其他地找了)
- Java程序员从笨鸟到菜鸟之(七十四)细谈Spring(六)spring之AOP基本概念和配置详解
- Spring3.0 AOP 详解
- Spring学习(2):SpringAOP基本概念详解
- 详解spring面向切面aop拦截器
- spring中的AOP编程思想详解
- Spring详解-----------AOP通知、代理
- Spring4之AOP注解配置详解