Page Object Model (Selenium + Python)(二)
2017-04-19 11:40
176 查看
Why this post?
Tutorials on the page object model usually show you how to implement the page object model using a cliched login page as an example. Most online tutorials rarely show you how to modify your existing tests to use the page object model. This post is a followup to our descriptive introduction to the page object model. In this tutorial, we provide a hands on example of how to revamp your existing test scripts
to use the page object model.
A refresher
Within your web app’s UI there are areas that your tests interact with. A Page Object simply models these as objects within the test code. This reduces the amount of duplicated code and means that if the UI changes, the fix need only be applied in one place.[1]The page object model allows us to do two things mainly:[2] 1) Encapsulate the low-level “internals” of the page – the
ids of the buttons, classes of element, etc.
2) Make the client (of the Page Object) test methods more elegant by taking them to a higher level.
Test scenario
I am going to test the chess viewer widget on Chessgames.com. I am choosing to check if Black’s 21st move in the Casino Royale Chess Game is executedcorrectly. Black’s queen moves from the b6 square to the f2 square. We will verify that the b6 square is empty and that the f2 square has a black queen on it.
PS: Black’s 21st move in this game is the reason behind our company name Qxf2.
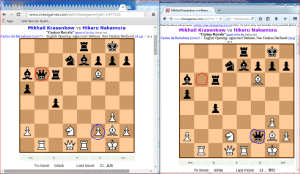
Implementing the page object model
For this tutorial, we will show the following:1. Write a working test
2. Prepare to move to the page object model
3. Implement the page objects
4. Run the test
5. Compare with the first implementation
1. Write a working test
I am going to implement a working test. We will use this as a starting point. Let’s pretend that this is your existing test. I have written the following qxf2move.py
qxf2move.py
import unittest from selenium import webdriver from selenium.webdriver.common.keys import Keys class qxf2move(unittest.TestCase): def setUp(self): self.driver=webdriver.Firefox() def testUserMove21Black(self): driver=self.driver driver.get("http://www.chessgames.com/perl/chessgame?gid=1477101") self.assertIn("Mikhail Krasenkow vs Hikaru Nakamura (2007) \"Casino Royale\"",driver.title) #Click on Black's 21st move driver.find_element_by_id("Var0Mv42").click() #Verify the squares b6 and f2 ([2,1], [6,5]) have the right pieces chess_square = driver.find_element_by_id("img_tcol5trow6") #Verify that the square f2 has a black queen (bq.png) self.assertEqual("http://www.chessgames.com/pgn4web-2.82/images/bq.png",chess_square.get_attribute('src')) chess_square = driver.find_element_by_id("img_tcol1trow2") #Verify that the square b6 is empty (clear.png) self.assertEqual("http://www.chessgames.com/pgn4web-2.82/images/clear.png",chess_square.get_attribute('src')) def tearDown(self): self.driver.close() if __name__=="__main__": unittest.main() |
by making a few changes.
2. Prepare to move to the page object model
– Identify the various objects and elements on the web page. Define them as objects and variables in the test script. E.g.: Title, Game_Id, Move_Str, Chess Board, Chess Piece, locators for the various objects
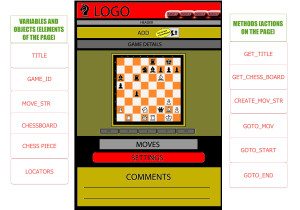
– Identify the various actions possible on these objects using the available elements. These actions can be defined as the methods in the test script. E.g.: get_Title, getCurrentBoard, create_mov_str, gotoMove, gotoStart, gotoEnd
3. Implement the different pages as page objects
-Separate the test case and the page objects, methods in different files. Here my test case is present in TestChessGamePage.py and the page objects, methods are present in ChessGamePage.py
TestChessGamePage.py
from selenium import webdriver from selenium.webdriver.common.by import By import unittest import ChessGamePage class TestChessGamePage(unittest.TestCase): def setUp(self): self.driver = webdriver.Firefox() def testUserMove21Black(self): "Test Black's 21st move (qxf2)" gameid = "1477101" player = "black" movenum = 21 """List of the board position in the format (Piece,row,col) where top left corner of the board is row =0, col= 0, white player = 'w',black player = 'b' pawn(p), rook(r), knight(n), bishop(b), queen(q), and king(k)""" lst = [('br',0,4), ('bk',0,6), ('bn',1,3), ('bb',1,4), ('bp',1,5), ('bp',1,6), ('bp',1,7), ('bb',2,0), ('br',2,2), ('wb',2,5), ('bp',3,0), ('bp',4,2), ('wp',5,6), ('wp',6,0), ('wn',6,3), ('bq',6,5), ('wb',6,6), ('wp',6,7), ('wr',7,1), ('wq',7,3), ('wr',7,4), ('wk',7,6)] chessgame_page = ChessGamePage.ChessGamePage(self.driver,gameid) chessgame_page.open() page_title = chessgame_page.get_Title() self.assertIn("Mikhail Krasenkow vs Hikaru Nakamura (2007) \"Casino Royale\"", page_title) #Goto Black's 21st move chessgame_page.goto_Move(player,movenum) actualBoardb21 = chessgame_page.getCurrentBoard() expectedBoardb21 = ChessGamePage.ChessBoard() expectedBoardb21.populate(lst) #Compare with the expected chess board self.assertEqual(actualBoardb21,expectedBoardb21) def tearDown(self): self.driver.close() #---START OF SCRIPT if __name__=="__main__": suite = unittest.TestLoader().loadTestsFromTestCase (TestChessGamePage) unittest.TextTestRunner(verbosity=2).run(suite) |
All the finer details are added to the files Base_Page_Object.py and ChessGamePage.py as shown below
Base_Page_Object.py
from selenium import webdriver from selenium.webdriver.common.by import By class Page(object): """ Base class that all page models can inherit from """ def __init__(self, selenium_driver, base_url='http://www.chessgames.com/'): self.base_url = base_url self.driver = selenium_driver self.timeout = 30 def find_element(self, *loc): return self.driver.find_element(*loc) def open(self,url): url = self.base_url + url self.driver.get(url) |
from selenium import webdriver from selenium.webdriver.common.by import By from Base_Page_Object import Page class ChessGamePage(Page): #Elements move_str = "" url = "" """ Var0Mv(N*2-1) is the id for Nth white move Var0Mv(N*2) is the id for Nth black move """ id_str = "Var0Mv" #Locators startButton_loc = (By.ID, 'startButton') backbButton_loc = (By.ID, 'backButton') autoplayButton_loc = (By.ID, 'autoplayButton') forwardButton_loc = (By.ID, 'forwardButton') endButton_loc = (By.ID, 'endButton') def __init__(self, selenium_driver,gameid,base_url='http://www.chessgames.com/'): Page.__init__(self, selenium_driver, base_url='http://www.chessgames.com/') self.url = 'perl/chessgame?gid=' + gameid # Actions def openpage(self): self.open(self.url) def get_Title(self): """Returns the page title""" return self.driver.title def getCurrentBoard(self): """Returns the current chessboard positions""" board = ChessBoard(self.driver) board.loadFromPage() return board def create_move_str(self,player,move): """Creates the value string using the player and move information""" if player.lower() == "white": self.move_str = self.id_str + str((move*2)-1) else: self.move_str = self.id_str + str(move*2) def goto_Move(self,player,move): """Clicks on the particular move""" self.create_move_str(player,move) move_loc = (By.ID,self.move_str) self.find_element(*move_loc).click() def goto_Start(self): """Clicks the start of the game button""" self.find_element(*self.startButton_loc).click() def goto_Back(self): """Clicks the ack one step bbutton""" self.find_element(*self.backButton_loc).click() def goto_AutoPlay(self): """Clicks the autoplay button""" self.find_element(*self.autoplayButton_loc).click() def goto_Forward(self): """Clicks the one step forward button""" self.find_element(*self.forwardButton_loc).click() def goto_End(self): """Clicks the end of game button""" self.find_element(*self.endButton_loc).click() """This class represents the actual chess board present on the page""" class ChessBoard(object): """This string is the id for the image(piece) present in each square(col,row) of the chess board""" id_str = "img_tcol%strow%s" def __init__(self,driver=None): self.driver = driver self.grid = [] def loadFromPage(self): """Creates the chess matrix from the page data""" for row in xrange(8): for col in xrange(8): name = self.getSquare(row,col) if name: piece = ChessPiece(name,row,col) self.grid.append(piece) def getSquare(self, row, col): """Returns the piece present at the particular row,col. None if the square is vacant""" elem = self.driver.find_element_by_id(self.id_str%(str(col),str(row))) src = elem.get_attribute('src') name = src.split("/")[-1].split(".")[0] return name if (name.lower() != 'clear') else None def populate(self,lst): """Creates the chess matrix as per the user data""" for entry in lst: name,row,col = entry piece = ChessPiece(name,row,col) self.grid.append(piece) def __str__(self): return str(self.grid) def __eq__(self,obj): return self.grid == obj.grid """This class represents the chessmen deployed on a chessboard for playing the game of chess""" class ChessPiece(object): def __init__(self,name,row,col): self.name = name self.row = row self.col = col def __eq__(self,obj): return (self.name == obj.name and self.row == obj.row and self.col == obj.col) def __hash__(self): tmp = 'row' + `self.row` + 'col' + `self.col` + self.name return hash(tmp) def __str__(self): return "(%s,%d,%d)" % (self.name, self.row, self.col) def __repr__(self): return "(%s,%d,%d)" % (self.name, self.row, self.col) |
Here is the snapshot of the test run
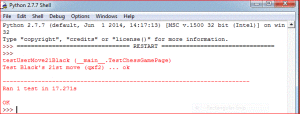
5. Compare with the first implementation
Let us compare the files qxf2move.py and TestChessGamePage.py
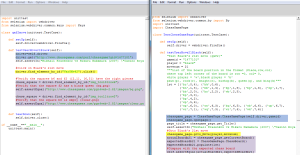
You’ll notice that using the page object model, it becomes easy to add new testcases without bothering about the details of the page elements. Also any changes to the page elements do not change the testcases – only the logic in ChessGamePage.py needs to be
modified.
原文:https://qxf2.com/blog/page-object-model-selenium/#comment-1147
相关文章推荐
- Selenium的PO模式(Page Object Model)|(Selenium Webdriver For Python)
- Selenium的PO模式(Page Object Model)[python版]
- Selenium的PO模式(Page Object Model)|(Selenium Webdriver For Python)
- Page Object Model (Selenium, Python)(一)
- Python+Selenium使用Page Object实现页面自动化测试
- Python+Selenium使用Page Object实现页面自动化测试
- Python+Selenium使用Page Object实现页面自动化测试
- Python+Selenium框架设计--- Page Object Model
- Selenium的PO模式(Page Object Model)[python版]
- Page Object Model (Selenium, Python)
- Page Object Model (Selenium + Python)(三)
- 搭建Python Selenium自动化测试环境
- Selenium + python的自动化框架搭建
- python model字段类型
- Running Selenium 'headless' with Chrome Driver in Python - YouTube
- Selenium Python bindings 文档二
- python glob model
- Selenium with Python
- python中使用selenium
- python学习(一)Use Python to Drive Selenium RC