[置顶] 数据库连接池c++ (msyql) mysql connector c++ 的使用
2017-04-02 21:41
453 查看
Mysql Connector/C++
使用介绍
一、下载mysql connector c++和 boost库
wget https://dev.mysql.com/get/Downloads/Connector-C++/mysql-connector-c++-1.1.8-linux-glibc2.5-x86-64bit.tar.gz
下载:根据自己的系统下载mysql connector c++版本,我们直接下载已经编译好压缩包,直接使用包里面的inlcude文件以及a文件或者so文件就可以了。当然你也可以下source源码文件自己编译安装。我是用官网的编译好的包,如下面所示。
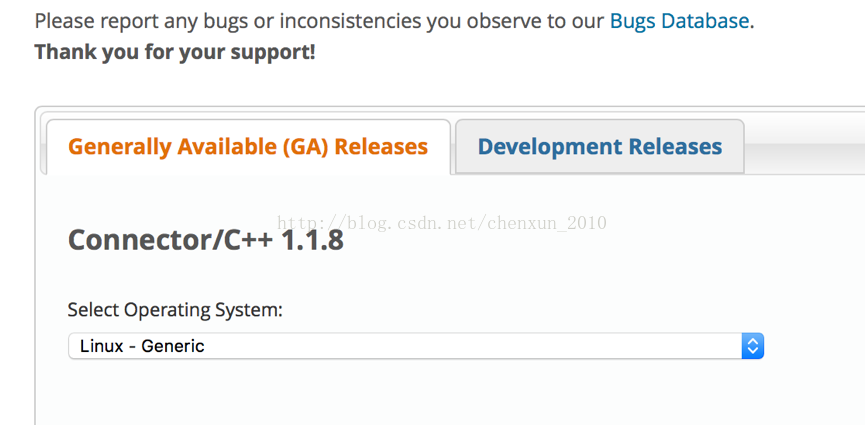
解压出来目录如下:(只要把include和lib目录包含到自己的项目目录就可以了)
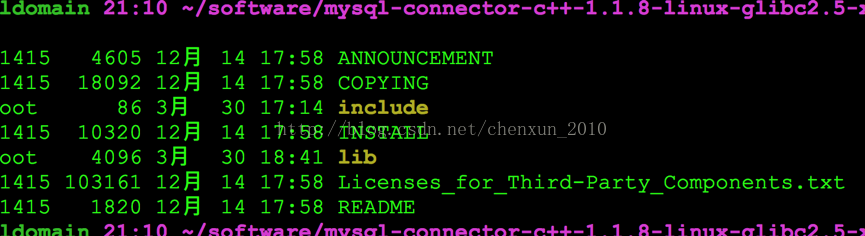
如果自己想从source源码编译出来:请按照下图页面下载就可以:
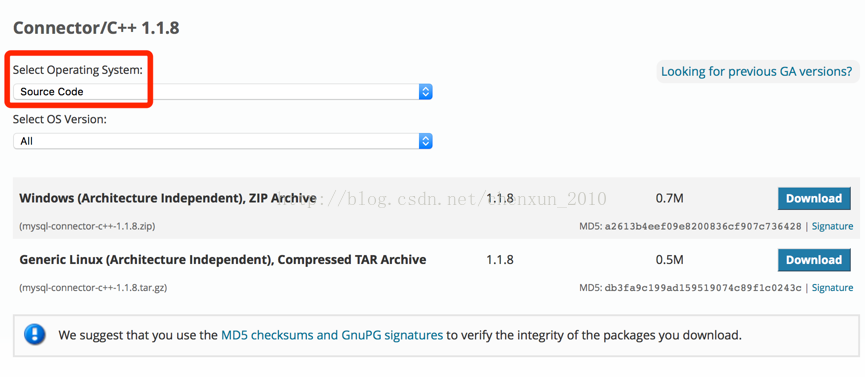
二、下载boost库编译安装boost库,因为mysql connector c++需要boost库的支持:(当然你可以直接使用yum安装)
wget https://sourceforge.net/projects/boost/files/boost/1.61.0/boost_1_61_0.tar.gz
然后解压安装,根据自己的实际情况选择安装路劲。我是默认安装的,安装步骤如下:
./bootstrap.sh
./b2
./b2 isntall
三.下面提供一个本人编写连接池,根据网上一些资料修改的。
cpp文件
main.cpp文件
自己根据项目写一个makefile,我这里就不提供了。运行情况如下
注意include文件和so文件路劲编译选项加上-lmysqlcppconn例如:
g++ main.cpp -o main -I... -L... –lmysqlcppconn
-I接你的mysqlconnector c++文头
-L接你的msyqlconnector c++ lib路劲
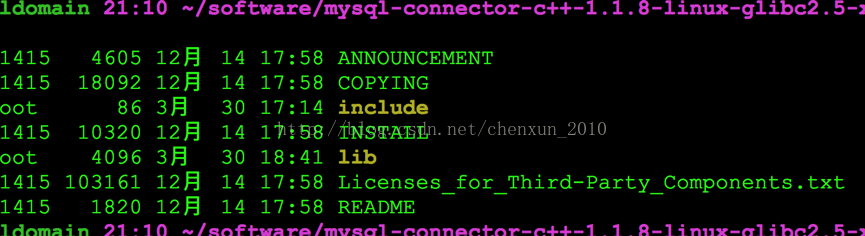
运行情况如下:
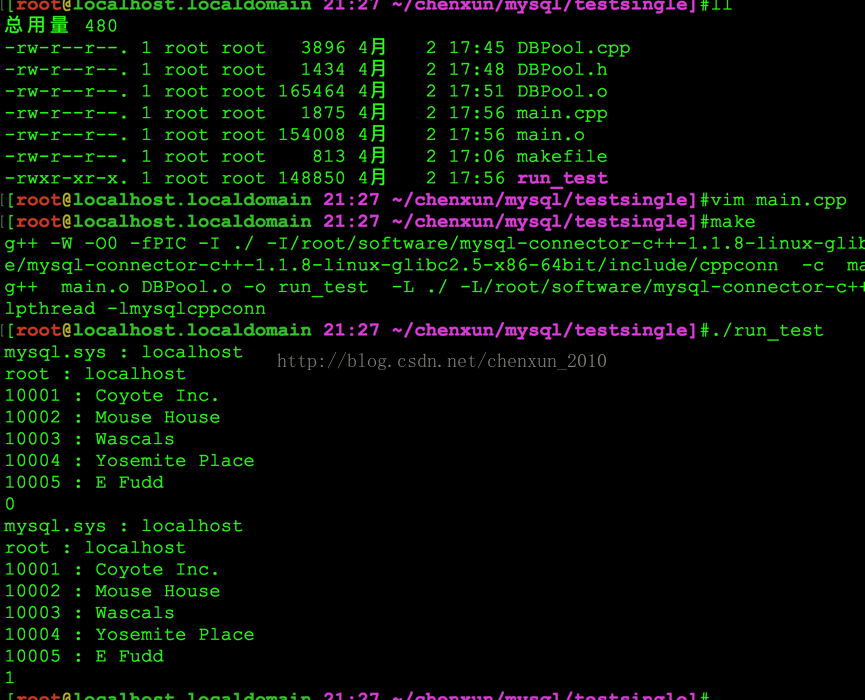
使用介绍
一、下载mysql connector c++和 boost库
wget https://dev.mysql.com/get/Downloads/Connector-C++/mysql-connector-c++-1.1.8-linux-glibc2.5-x86-64bit.tar.gz
下载:根据自己的系统下载mysql connector c++版本,我们直接下载已经编译好压缩包,直接使用包里面的inlcude文件以及a文件或者so文件就可以了。当然你也可以下source源码文件自己编译安装。我是用官网的编译好的包,如下面所示。
解压出来目录如下:(只要把include和lib目录包含到自己的项目目录就可以了)
如果自己想从source源码编译出来:请按照下图页面下载就可以:
二、下载boost库编译安装boost库,因为mysql connector c++需要boost库的支持:(当然你可以直接使用yum安装)
wget https://sourceforge.net/projects/boost/files/boost/1.61.0/boost_1_61_0.tar.gz
然后解压安装,根据自己的实际情况选择安装路劲。我是默认安装的,安装步骤如下:
./bootstrap.sh
./b2
./b2 isntall
三.下面提供一个本人编写连接池,根据网上一些资料修改的。
/* time: 2017.3.31 author: chenxun 275076730@qq.coom notice: MySql Connector C++ */ #ifndef _DB_POOL_H_ #define _BD_POOL_H_ #include <iostream> #include <mysql_connection.h> #include <mysql_driver.h> #include <cppconn/exception.h> #include <cppconn/driver.h> #include <cppconn/connection.h> #include <cppconn/resultset.h> #include <cppconn/prepared_statement.h> #include <cppconn/statement.h> #include <pthread.h> #include <list> using namespace std; using namespace sql; class DBPool { public: // Singleton static DBPool& GetInstance(); //init pool void initPool(std::string url_, std::string user_, std::string password_, int maxSize_); //get a conn from pool Connection* GetConnection(); //put the conn back to pool void ReleaseConnection(Connection *conn); ~DBPool(); private: DBPool(){} //init DB pool void InitConnection(int initSize); // create a connection Connection* CreateConnection(); //destory connection void DestoryConnection(Connection *conn); //destory db pool void DestoryConnPool(); private: string user; string password; string url; int maxSize; int curSize; Driver* driver; //sql driver (the sql will free it) list<Connection*> connList; //create conn list //thread lock mutex static pthread_mutex_t lock; }; #endif
cpp文件
/* time: 2017.3.31 author: chenxun 275076730@qq.coom notice: MySql Connector C++ */ #include <iostream> #include <stdexcept> #include <exception> #include <stdio.h> #include "DBPool.h" using namespace std; using namespace sql; pthread_mutex_t DBPool::lock = PTHREAD_MUTEX_INITIALIZER; //Singleton: get the single object DBPool& DBPool::GetInstance() { static DBPool instance_; return instance_; } void DBPool::initPool(std::string url_, std::string user_, std::string password_, int maxSize_) { this->user = user_; this->password = password_; this->url = url_; this->maxSize = maxSize_; this->curSize = 0; try{ this->driver=sql::mysql::get_driver_instance(); } catch(sql::SQLException& e) { perror("Get sql driver failed"); } catch(std::runtime_error& e) { perror("Run error"); } this->InitConnection(maxSize/2); } //init conn pool void DBPool::InitConnection(int initSize) { Connection* conn; pthread_mutex_lock(&lock); for(int i =0;i <initSize; i++) { conn= this->CreateConnection(); if(conn) { connList.push_back(conn); ++(this->curSize); } else { perror("create conn error"); } } pthread_mutex_unlock(&lock); } Connection* DBPool::CreateConnection() { Connection* conn; try{ conn = driver->connect(this->url,this->user,this->password); //create a conn return conn; } catch(sql::SQLException& e) { perror("link error"); return NULL; } catch(std::runtime_error& e) { perror("run error"); return NULL; } } Connection* DBPool::GetConnection() { Connection* conn; pthread_mutex_lock(&lock); if(connList.size()>0)//the pool have a conn { conn = connList.front(); connList.pop_front();//move the first conn if(conn->isClosed())//if the conn is closed, delete it and recreate it { delete conn; conn = this->CreateConnection(); } if(conn == NULL) { --curSize; } pthread_mutex_unlock(&lock); return conn; } else { if(curSize< maxSize)//the pool no conn { conn = this->CreateConnection(); if(conn) { ++curSize; pthread_mutex_unlock(&lock); return conn; } else { pthread_mutex_unlock(&lock); return NULL; } } else //the conn count > maxSize { pthread_mutex_unlock(&lock); return NULL; } } } //put conn back to pool void DBPool::ReleaseConnection(Connection *conn) { if(conn) { pthread_mutex_lock(&lock); connList.push_back(conn); pthread_mutex_unlock(&lock); } } void DBPool::DestoryConnPool() { list<Connection*>::iterator iter; pthread_mutex_lock(&lock); for(iter = connList.begin(); iter!=connList.end(); ++iter) { this->DestoryConnection(*iter); } curSize=0; connList.clear(); pthread_mutex_unlock(&lock); } void DBPool::DestoryConnection(Connection* conn) { if(conn) { try{ conn->close(); } catch(sql::SQLException&e) { perror(e.what()); } catch(std::exception& e) { perror(e.what()); } delete conn; } } DBPool::~DBPool() { this->DestoryConnPool(); }
main.cpp文件
/* * main.cpp * * Created on: 2013-3-26 * Author: chenxun */ #include "DBPool.h" #include <stdio.h> /*-------------------------------------------------------------- 单例模式,全局唯一 db pool,程序中使用onnpool中获取一个 db连接使用,使用完之后调用ReleaseConnection把conn放回pool中去. ----------------------------------------------------------------*/ DBPool connpool = DBPool::GetInstance(); int main(int argc, char* argv[]) { //初始化连接,创建参数中maxSize一半的连接 connpool.initPool("tcp://127.0.0.1:3306", "root", "123456", 100); Connection *con; Statement *state; ResultSet *result; con = connpool.GetConnection();//get a db conn for(int i = 0; i<1000; i++) { state = con->createStatement(); state->execute("use mysql"); // 查询 result = state->executeQuery("select host,user from user"); // 输出查询 while (result->next()) { try{ string user = result->getString("user"); string name = result->getString("host"); cout << user << " : " << name << endl; }catch(sql::SQLException& e){ std::cout << e.what() << std::endl; } } /*result = state->executeQuery("select cust_id,cust_name from customers"); while (result->next()) { try{ string user = result->getString("cust_id"); string name = result->getString("cust_name"); cout << user << " : " << name << endl; }catch(sql::SQLException& e){ std::cout << e.what() << std::endl; } } */ std::cout << i << std::endl; } delete result; delete state; connpool.ReleaseConnection(con); return 0; }
自己根据项目写一个makefile,我这里就不提供了。运行情况如下
注意include文件和so文件路劲编译选项加上-lmysqlcppconn例如:
g++ main.cpp -o main -I... -L... –lmysqlcppconn
-I接你的mysqlconnector c++文头
-L接你的msyqlconnector c++ lib路劲
运行情况如下:
相关文章推荐
- 使用MySQL connector/C++链接MySQL数据库
- 在CentOS里使用MySQL Connector/C++
- MySQL Connector/C++ 使用中出现的一个错误
- 编译和使用 MySQL C++ Connector
- 使用MySQL connector/C++链接MySQL数据库
- VS2010 64 位使用mysql-connector-c++-noinstall-1.1.3-winx64开发MySQL,供初学者
- 使用Visual Studio编译MYSQL CONNECTOR C/C++(MYSQLCPPCONN)
- MySQL connector c++使用笔记
- 在CentOS里使用MySQL Connector/C++
- 使用MySQL connector/C++链接MySQL数据库
- mysql connector c++与 visual studio 2012 联合使用
- 使用MySQL connector/C++链接MySQL数据库
- 在CentOS里使用MySQL Connector/C++
- 【转】使用Mysql-connector-c++插入数据时乱码解决
- C++使用MySQL-Connector/C++连接MySQL出现LNK2019错误
- 编译和使用 MySQL C++ Connector
- C++使用MySQL-Connector/C++连接MySQL出现LNK2019错误的解决方法
- 在CentOS里使用MySQL Connector/C++
- 使用MySQL connector/C++链接MySQL数据库
- VS 2010中使用MySQL Connector C/C++的问题