Spring Boot整合Mybatis
2017-03-21 17:31
260 查看
以往在使用Spring集成Mybatis都伴随着大量配置文件,而Spring Boot摒弃了繁琐的配置文件,可以快速的整合Mybatis,做到“2分钟”搭建web框架。
Mybatis官方文档:http://www.mybatis.org/spring-boot-starter
GitHub:https://github.com/mybatis/mybatis-spring-boot
Spring Boot整合Mybatis有两种方式,一种是基于注解的;另一种是使用xml,这也是官方推荐的一种,本文使用xml方式完成整合。
在上篇文章中已经使用Spring Boot搭建了一个简单的web项目,整合Mybatis很简单,只需要在pom文件中加入以下依赖即可
使用的是mysql数据库,加入依赖。
本人在整合mysql时候还遇到了一点小问题,因为Spring Boot会选用对应的依赖版本,所以刚开始没有加上版本标签,运行项目时候抛出这样一个异常。
后来发现Spring Boot默认加载的mysql版本是5.1.40的,而本人的是5.1.29版本,加上版本之后完美启动。
可以在命令窗口通过mysql -V查询自己的mysql版本
然后就可以写Mapper接口和对应Mapper.xml文件了,在写Mapper接口和Mapper.xml时尽量保持两者名称一致,以便方便查找,当然不一致完全没有问题。注意两者定义的方法名是需要保持一致的
StudentMapper.java
student.xml
实体Student.java
application.yml配置文件
StudentController.java
启动类
数据库表

启动项目,在浏览器访问:http://localhost:8080/student/getById/1显示结果如下
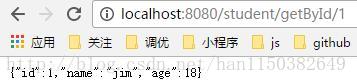
这样一个Spring Boot整合Mybatis项目就完成了,是不是非常简单
Mybatis官方文档:http://www.mybatis.org/spring-boot-starter
GitHub:https://github.com/mybatis/mybatis-spring-boot
Spring Boot整合Mybatis有两种方式,一种是基于注解的;另一种是使用xml,这也是官方推荐的一种,本文使用xml方式完成整合。
在上篇文章中已经使用Spring Boot搭建了一个简单的web项目,整合Mybatis很简单,只需要在pom文件中加入以下依赖即可
<dependency> <groupId>org.mybatis.spring.boot</groupId> <artifactId>mybatis-spring-boot-starter</artifactId> <version>1.2.0</version> </dependency>
使用的是mysql数据库,加入依赖。
<dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> <version>5.1.29</version> </dependency>
本人在整合mysql时候还遇到了一点小问题,因为Spring Boot会选用对应的依赖版本,所以刚开始没有加上版本标签,运行项目时候抛出这样一个异常。
org.springframework.jdbc.CannotGetJdbcConnectionException: Could not get JDBC Connection; nested exception is java.sql.SQLException: Unknown system variable 'language'
后来发现Spring Boot默认加载的mysql版本是5.1.40的,而本人的是5.1.29版本,加上版本之后完美启动。
可以在命令窗口通过mysql -V查询自己的mysql版本
然后就可以写Mapper接口和对应Mapper.xml文件了,在写Mapper接口和Mapper.xml时尽量保持两者名称一致,以便方便查找,当然不一致完全没有问题。注意两者定义的方法名是需要保持一致的
StudentMapper.java
package com.springboot.mapper; import org.apache.ibatis.annotations.Mapper; import com.springboot.enity.Student; /** * @Mapper 可以不写,只需要在启动类加上注解@MapperScan 然后指定Mapper接口所在包路径即可 */ //@Mapper public interface StudentMapper { Student getById(Integer id); }
student.xml
<?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd" > <mapper namespace="com.springboot.mapper.StudentMapper"> <select id="getById" parameterType="java.lang.Integer" resultType="com.springboot.enity.Student"> select id,name,age from student where id = #{id} </select> </mapper>
实体Student.java
public class Student { private Integer id; private String name; private Integer age; /* * getter and setter */ }
application.yml配置文件
spring: datasource: url : jdbc:mysql://127.0.0.1:3306/springboot_mybatis driver-class-name: com.mysql.jdbc.Driver username: root password: root mybatis: mapper-locations: - classpath:mybatis/*.xml #Mapper.xml文件位置 type-aliases-package: com.springboot.enity #实体位置
StudentController.java
package com.springboot.controller; import javax.annotation.Resource; import org.springframework.web.bind.annotation.PathVariable; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RestController; import com.springboot.enity.Student; import com.springboot.service.IStudentService; @RestController @RequestMapping("/student") public class StudentController { @Resource private StudentMapper studentMapper; @RequestMapping("/getById/{id}") public Student getById(@PathVariable Integer id){ Student s = studentMapper.getById(id); return s; } }
启动类
package com.springboot; import org.mybatis.spring.annotation.MapperScan; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; @SpringBootApplication //指定Mapper.java存放路径 @MapperScan(basePackages = "com.springboot.mapper") public class MybatisSpringbootApplication { public static void main(String[] args) { SpringApplication.run(MybatisSpringbootApplication.class, args); } }
数据库表
启动项目,在浏览器访问:http://localhost:8080/student/getById/1显示结果如下
这样一个Spring Boot整合Mybatis项目就完成了,是不是非常简单
相关文章推荐
- Spring boot +freemarker+mybatis整合
- Spring Boot 整合Mybatis(步骤讲解) 附源码
- springBoot整合mybatis
- springboot学习笔记-2 一些常用的配置以及整合mybatis
- springboot与mybatis整合实例详解(完美融合)
- springboot 整合mybatis
- Spring Boot学习第三篇:Spring Boot整合Mybatis、和其他
- springBoot与MyBatis整合示例
- spring-boot整合mybatis打包war包
- spring boot 学习笔记(2) 整合mybatis
- Spring boot 整合mybatis
- spring-boot与mybatis整合优化介绍
- springboot与mybatis整合实例详解(完美融合)
- SpringBoot入门之整合mybatis
- spring boot和mybatis整合
- Spring Boot学习记录(三)--整合Mybatis
- Spring Boot整合MyBatis
- SpringBoot整合Mybatis示例
- springboot整合mybatis中的坑
- Spring Boot整合MyBatis