Java,Socket&TCP编程 实现多线程端对端通信与文件传输
2017-03-12 12:22
603 查看
做了一个Java的项目,利用TCP实现了端对端通信与文件传输。下面是实现的效果:
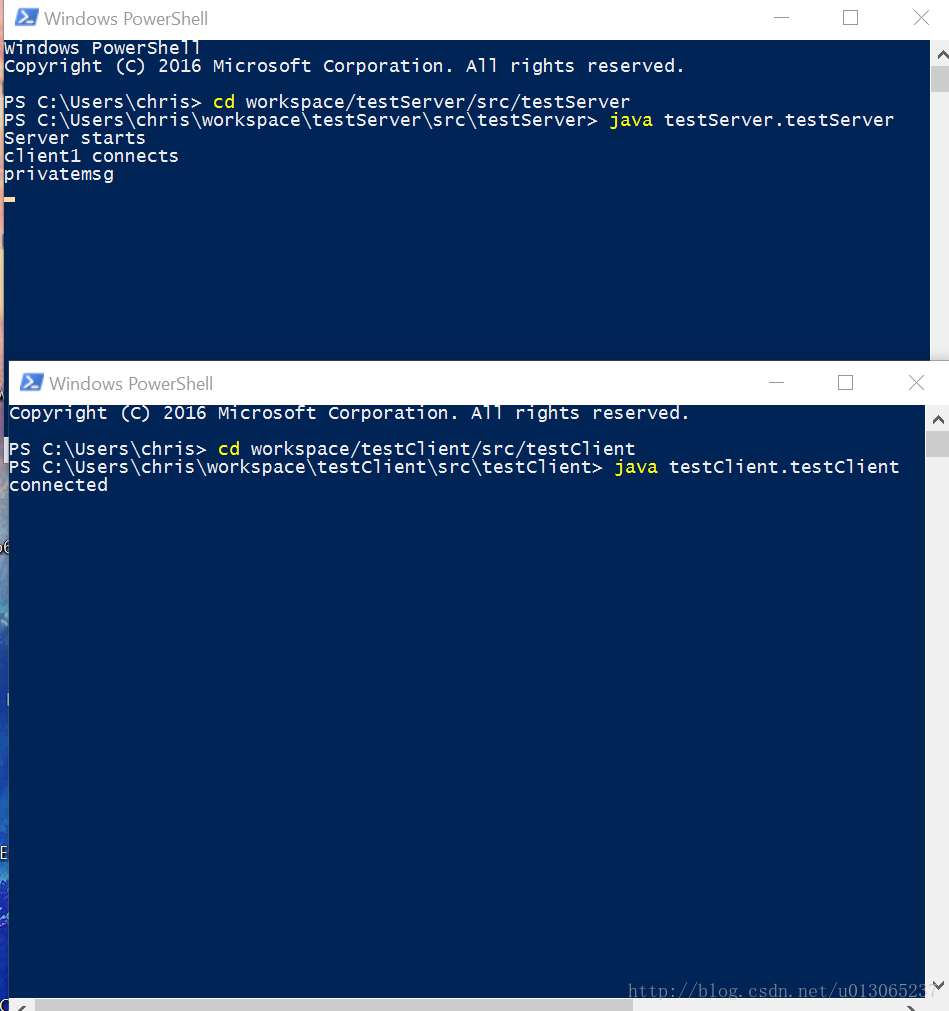
然后开启四个客户端:
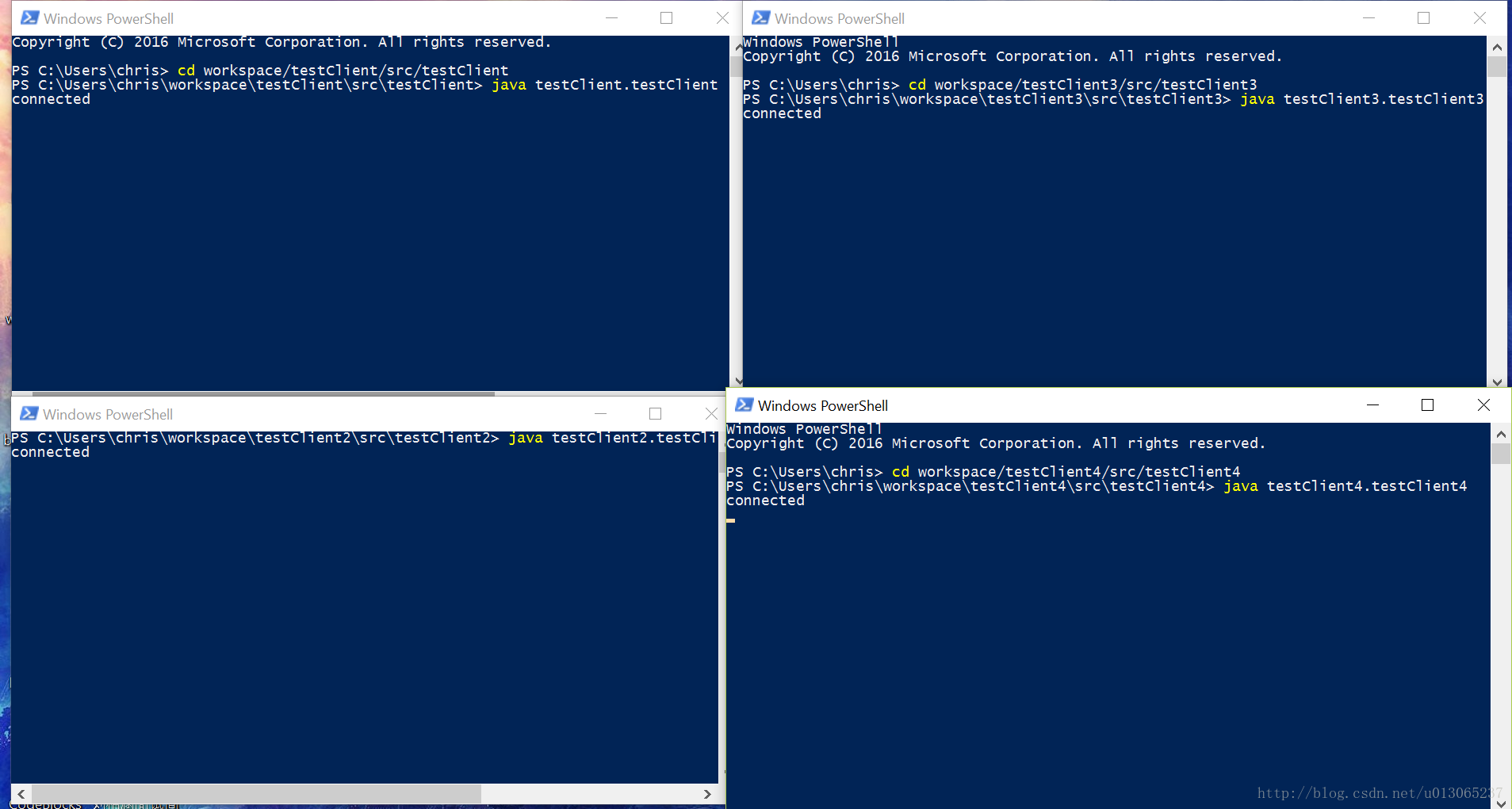
实现群聊效果:
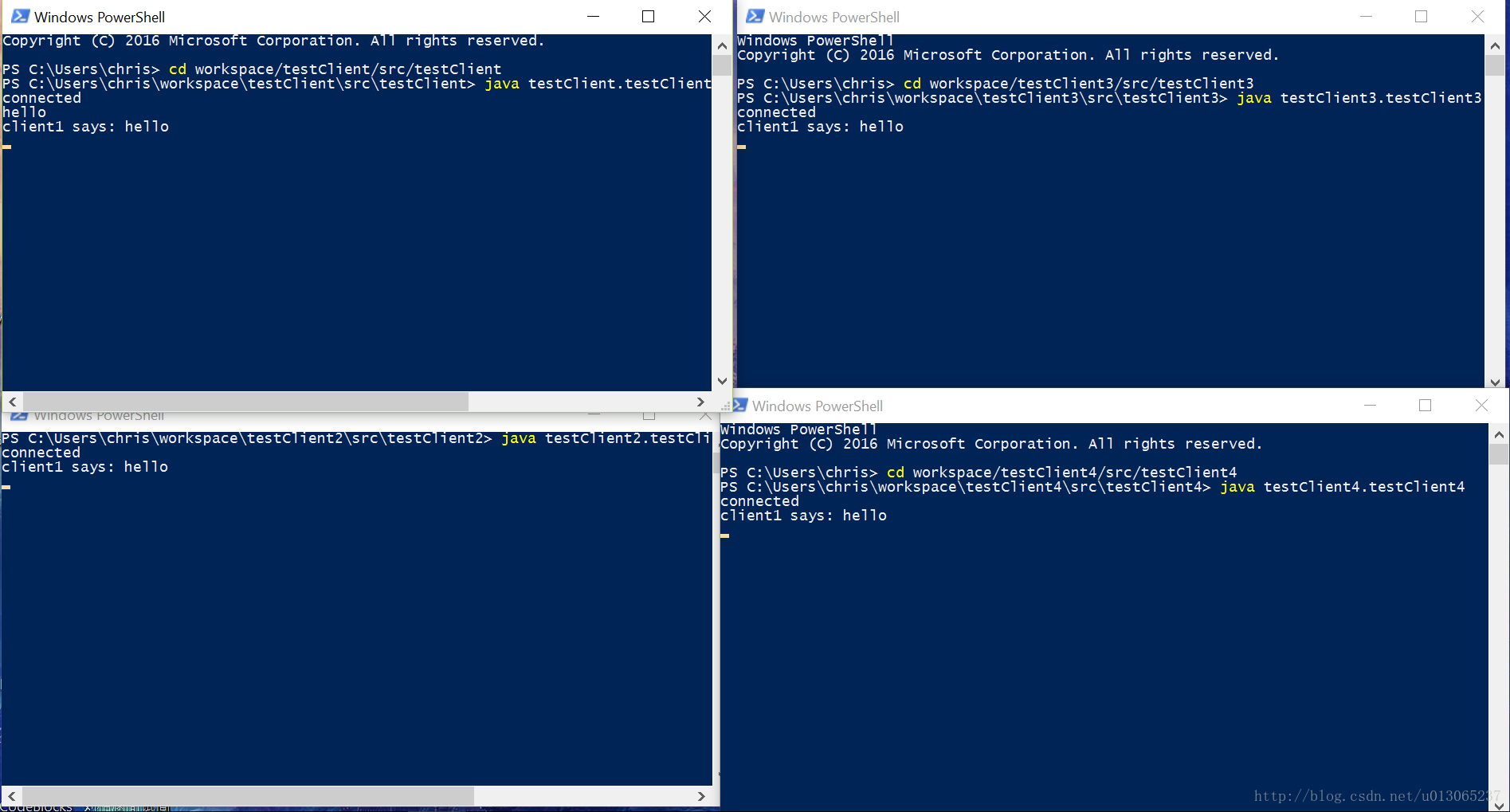
实现私聊效果:
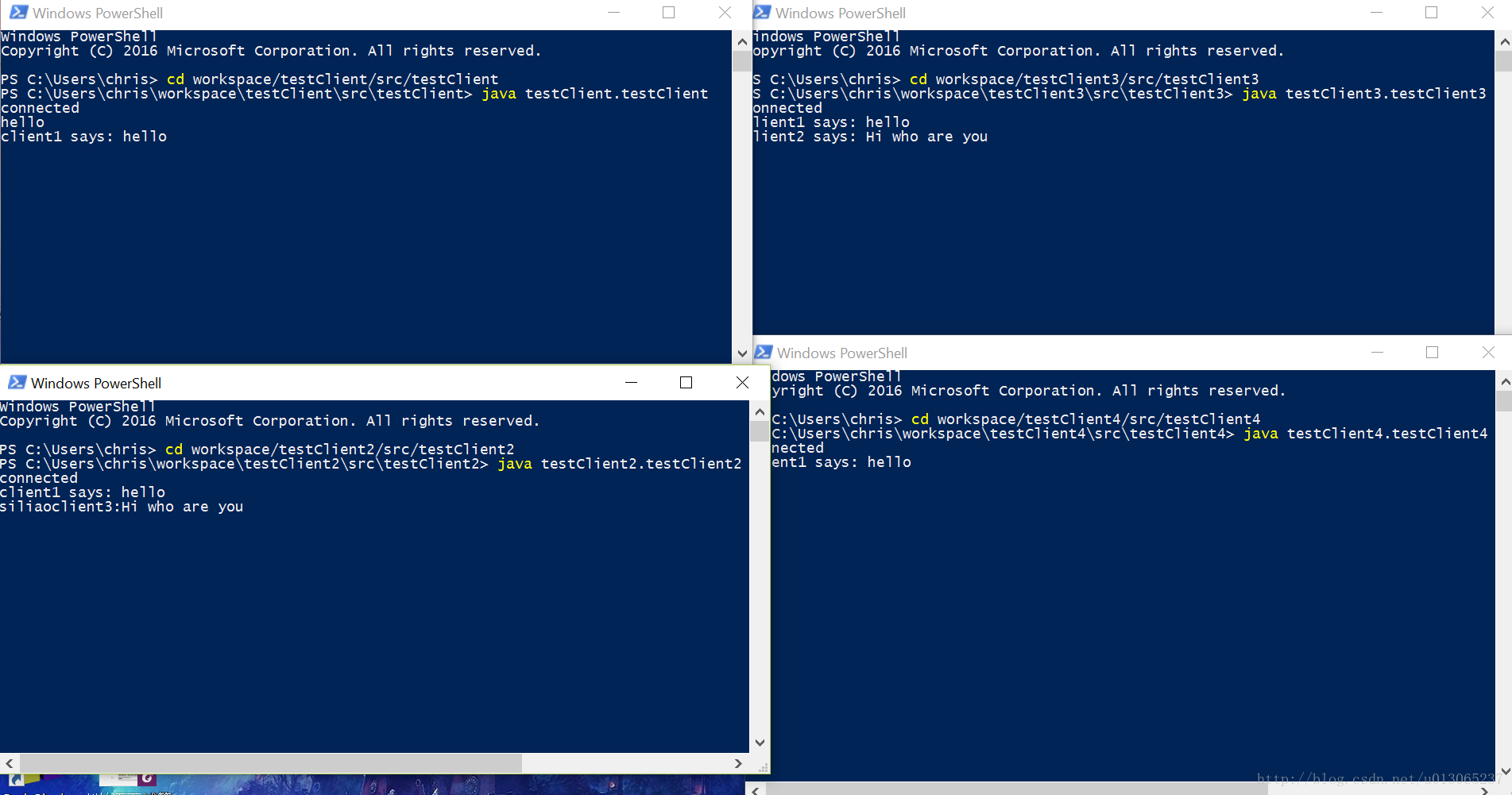
实现屏蔽效果:
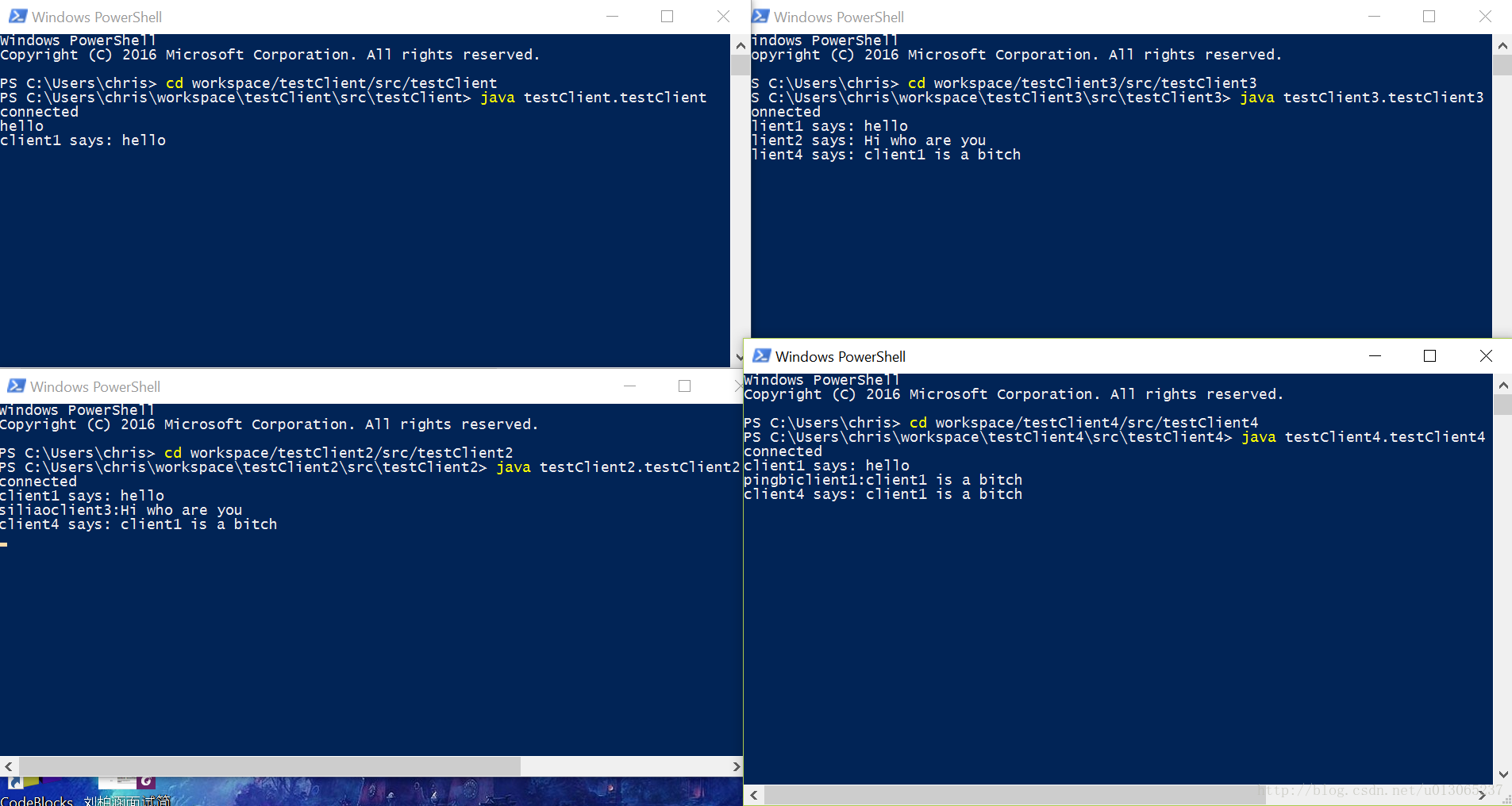
实现文件群发效果:
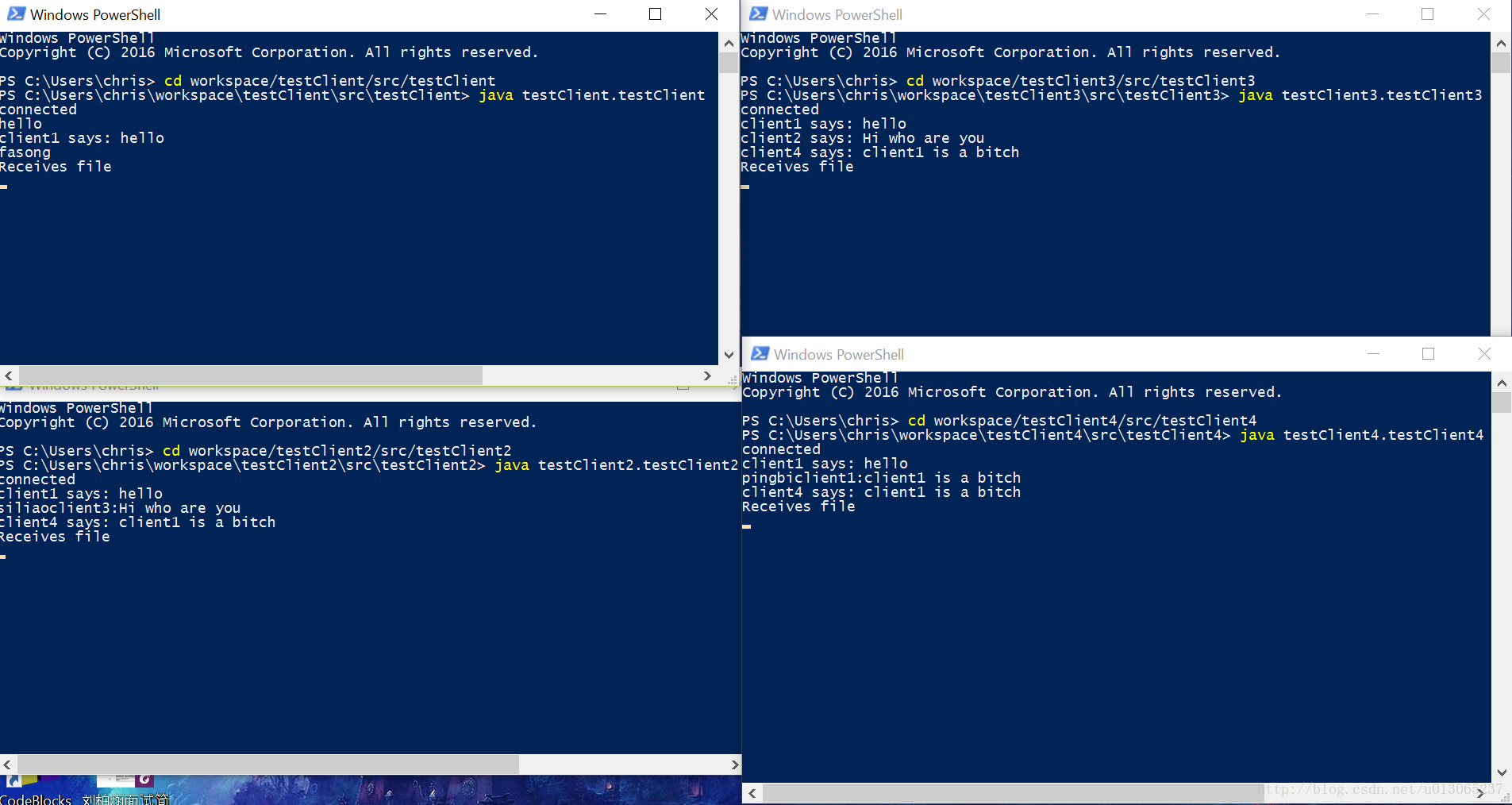
实现端对端文件传输:
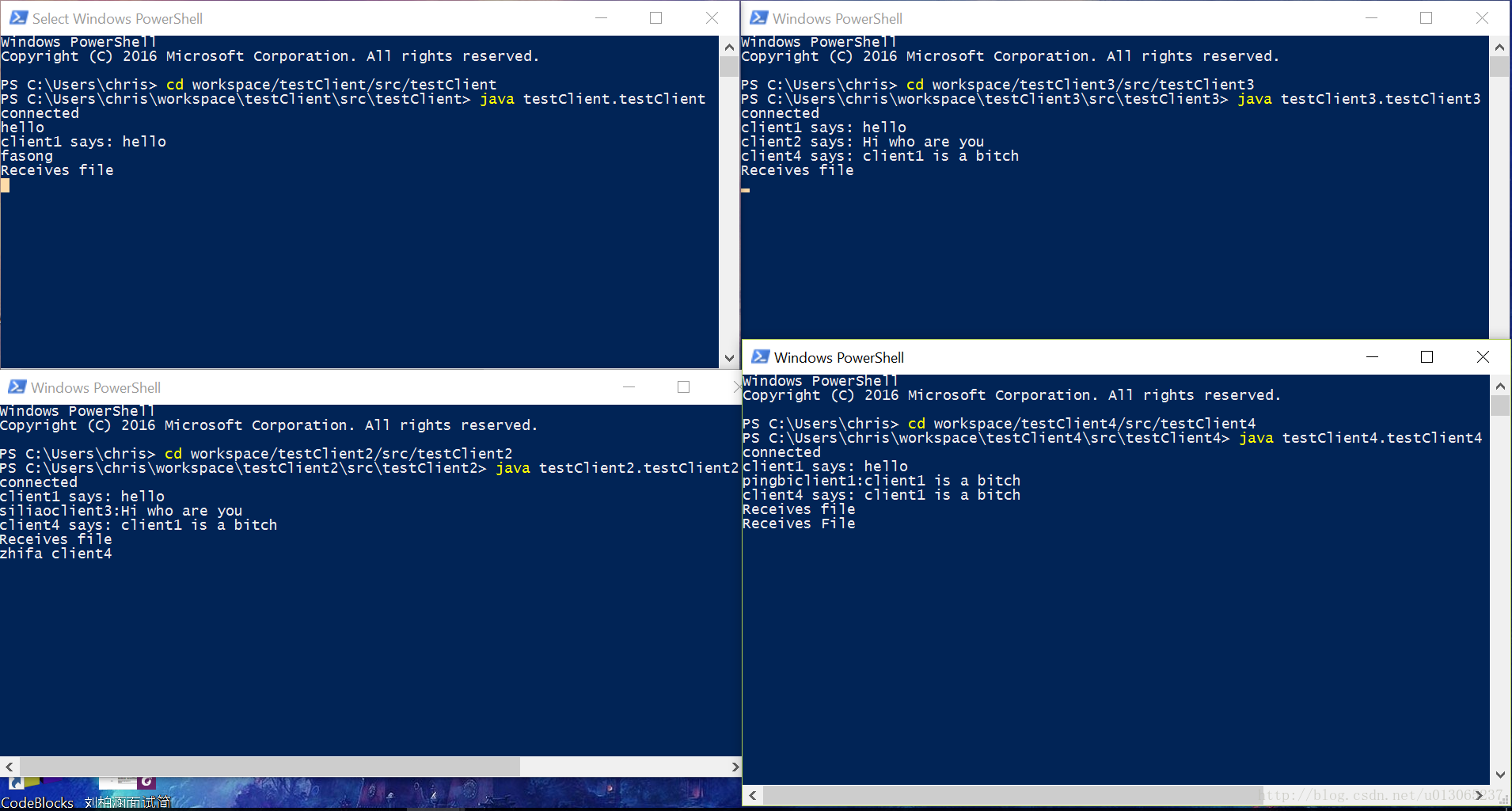
服务器工作过程:
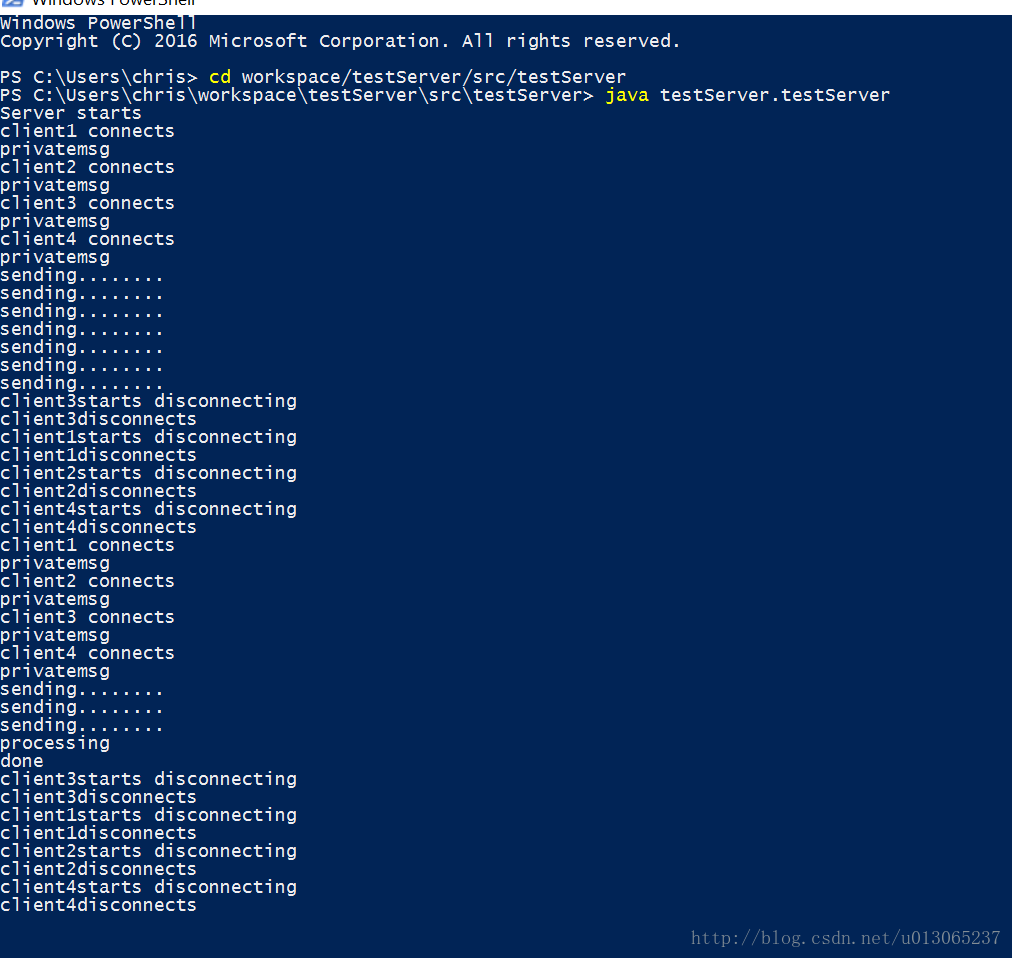
实现过程主要是利用Java里面的TCP与Socket套接字进行编程,在服务器建立一个Hash表用于存储每个客户端的名字以及IP地址。
群聊等传输功能都是在服务器端实现,现在把客户端的代码附在下面:
然后开启四个客户端:
实现群聊效果:
实现私聊效果:
实现屏蔽效果:
实现文件群发效果:
实现端对端文件传输:
服务器工作过程:
实现过程主要是利用Java里面的TCP与Socket套接字进行编程,在服务器建立一个Hash表用于存储每个客户端的名字以及IP地址。
群聊等传输功能都是在服务器端实现,现在把客户端的代码附在下面:
package testClient; import java.io.*; import java.net.*; public class testClient implements Runnable{ static Socket socket = null; static PrintWriter pw = null; BufferedReader bufferedReader; Client client; private static String string = ""; public static int m=0; public testClient(){ try { socket = new Socket(InetAddress.getLocalHost(),5678);// set socket System.out.println("connected"); pw = new PrintWriter(socket.getOutputStream());//set outputstream pw.println("client1"); pw.flush(); client = new Client(socket); client.start(); } catch (Exception e) { System.out.println("connect failed"); } } @Override public void run() { bufferedReader = new BufferedReader(new InputStreamReader(System.in)); while (true) { try { string = bufferedReader.readLine(); if(string.equals("end")){ testClient.Close(); break; } else if(!"".equals(string)){ pw.println(InetAddress.getLocalHost()+ ":" +string); pw.flush(); } } catch (IOException e) { } } } public static void sendFile(String filePath,Socket socket){ DataOutputStream dos=null; DataInputStream dis=null; try { File file=new File(filePath); dos=new DataOutputStream(socket.getOutputStream()); dis=new DataInputStream(new BufferedInputStream(new FileInputStream(filePath))); int buffferSize=1024; byte[]bufArray=new byte[buffferSize]; dos.writeUTF(file.getName()); dos.flush(); dos.writeLong((long) file.length()); dos.flush(); while (true) { int read = 0; if (dis!= null) { read = dis.read(bufArray); } if (read == -1) { break; } dos.write(bufArray, 0, read); } dos.flush(); } catch (FileNotFoundException e) { // TODO Auto-generated catch block e.printStackTrace(); } catch (IOException e) { // TODO Auto-generated catch block e.printStackTrace(); } finally { try { if (dos != null) dos.close(); } catch (IOException e) { } try { if (dis != null) dis.close(); } catch (IOException e) { } } } class Client extends Thread{//client thread Socket client; BufferedReader bufferedReader; PrintWriter printWriter; public Client (Socket socket) throws IOException{ this.client = socket; bufferedReader = new BufferedReader(new InputStreamReader(client.getInputStream()));//getinput printWriter = new PrintWriter(client.getOutputStream());//get output } public void run(){ String line = "" ; try { while(true){ if(string.equals("end")){ //end and break break; } else{ line = bufferedReader.readLine();//get input System.out.println(line);//print input } } } catch (UnknownHostException e) { e.printStackTrace(); } catch (IOException e) { System.out.println("Server disconnects"); } } } public static void Close() throws IOException{ pw.println(InetAddress.getLocalHost() + ":" +string); pw.flush(); socket = null; } public static void main(String args[]){ testClient myClient01 = new testClient(); myClient01.run(); } }
相关文章推荐
- java中进行socket编程实现tcp、udp协议总结
- Java网络编程,通过TCP,Socket实现多对一的局域网聊天室
- JAVA 通过 Socket 实现 TCP 编程
- JAVA 通过 Socket 实现 TCP 编程
- 网络编程_TCP_Socket通信_聊天室_私聊_构思_实现JAVA193-194
- Java通过Socket编程实现文件传输
- JAVA 通过 Socket 实现 TCP 编程
- java网络编程,通过TCP,Socket实现多对一的局域网聊天室 .
- Java网络编程实践和总结 --- 基于TCP的Socket编程之实现文件上传和下载服务
- java网络编程,通过TCP,Socket实现多对一的局域网聊天室
- java中进行socket编程实现tcp、udp协议总结
- java 实现 socket TCP 编程
- java网络编程,通过TCP,Socket实现多对一的局域网聊天室
- java基于TCP的socket编程简单实现[代码实践过]
- Java的Socket通信----通过 Socket 实现 TCP 编程之多线程demo(2)
- 4000 java基础_socket编程_TCP实现
- java TCP&UDP socket编程示例
- 实现了基于TCP的Java Socket编程实例代码
- JAVA 通过 Socket 实现 TCP 编程
- java网络编程,通过TCP,Socket实现多对一的局域网聊天室