C++实现一个日期类
2017-03-08 17:49
435 查看
函数实现部分:
头文件声明部分:
测试用例:
运行结果:
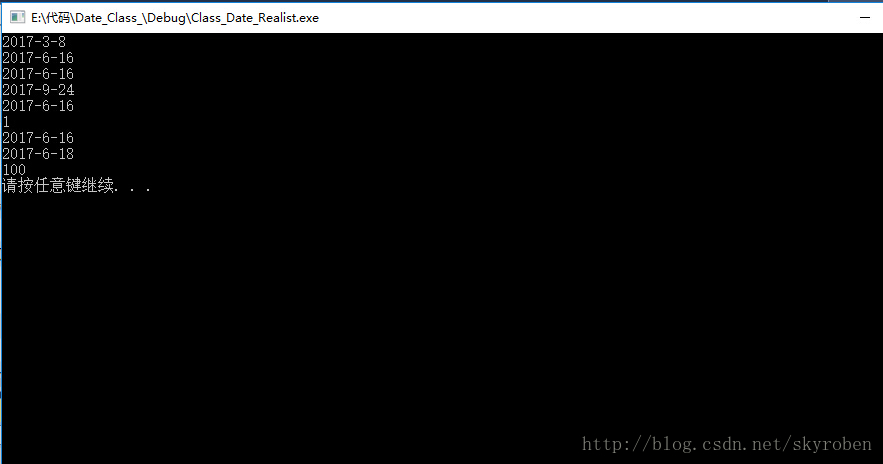
总结:
在实现日期类的时候要注意代码的复用+简洁,以及什么时候能够返回一个值的引用来使函数的效率更高(不能返回指向栈内存的引用和指针),这些都是需要注意的,以及函数的重载的理解(函数名可以相同,参数的类型不同或者参数的个数不同(参数列表不同),返回值可同可不同)。
#include "Date.h" void Date::display() { cout << _year << "-" << _month << "-" << _day << endl; } int GetMonthDay(int year, int month) { static int MonthDay[13] = { 0, 31, 29, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31 }; if (month == 2) { if (((year % 4 == 0) && (year % 100 != 0)) || (year % 400 == 0)) { return MonthDay[month] - 1; } } return MonthDay[month]; } bool Date::IsLegal() { if ((_year >= 0) && (_month > 0) && (_month < 13) && (_day>0) && (_day <= GetMonthDay(_year, _month))) { return true; } else { return false; } } Date Date:: operator+(int day) //不改变初始日期的值 { if (day < 0) { return *this - (-day); } Date tmp = *this; tmp._day += day; while (tmp._day > GetMonthDay(tmp._year, tmp._month)) { tmp._day = tmp._day - GetMonthDay(tmp._year, tmp._month); if (tmp._month == 12) { tmp._month = 1; tmp._year += 1; } else { tmp._month += 1; } } return tmp; } Date & Date::operator+=(int day) { *this = *this + day; //代码的复用 return *this; } Date Date::operator-(int day) //减去多少天,不改变初始日期的值 { if (day < 0) //d1-(-100) { return *this + (-day); } Date tmp = *this; tmp._day = tmp._day - day; while (tmp._day <= 0) { if (tmp._month == 1) { tmp._month = 12; tmp._year -= 1; } else { tmp._month -= 1; } tmp._day = tmp._day + GetMonthDay(tmp._year, tmp._month); } return tmp; } bool Date:: operator==(const Date&d) //比较日期大小 { if ((_year != d._year) || (_month != d._month) || (_day != d._day)) { return false; } return true; } bool Date:: operator!=(const Date&d) //比较日期大小 { return !((*this).operator==(d)); } Date & Date :: operator-=(int day) //减去多少天,改变初始日期的值 { *this = *this - day; return *this; } bool Date::operator> (const Date&d) //比较日期大小 { if ((this->_year>d._year) || ((this->_month>d._month)&&(this->_year==d._year)) ||((this->_day>d._day)&&(this->_month==d._month)&&(this->_year==d._year))) { return true; } return false; } Date Date:: operator++(int) //date++; { Date tmp = *this; *this += 1; return tmp; } Date & Date:: operator++() //date++; { *this += 1; return *this; } int Date::operator-(const Date&d) //两个日期相减相差多少天 { Date FutureDay; Date PastDay; int i = 0; if (*this > d) { FutureDay = *this; PastDay = d; } else { FutureDay = d; PastDay = *this; } while (FutureDay != PastDay) { PastDay += 1; i++; } return i; }
头文件声明部分:
#define _CRT_SECURE_NO_WARNINGS #ifndef __DATE_CLASS__ #define __DATE_CLASS__ #include <iostream> #include <windows.h> #include <assert.h> using namespace std; class Date { public: Date(int year=1995,int month=5 ,int day=6) :_year(year) , _month(month) , _day(day) { assert(IsLegal()); } void display(); //打印日期 Date operator+(int day); //加上多少天,不改变初始日期的值 Date & operator+=(int day); //加上多少天,改变初始日期的值 Date operator-(int day); //减去多少天,不改变初始日期的值 Date & operator-=(int day); //减去多少天,改变初始日期的值 bool operator>(const Date&d); //比较日期大小 bool operator==(const Date&d); //比较日期大小 bool operator!=(const Date&d); //比较日期大小 Date operator++(int); //date++; Date & operator++(); //++date; int operator-(const Date&d); //两个日期相减相差多少天 bool IsLegal(); //判断日期是否合法 private: int _year; int _month; int _day; };
测试用例:
#include "Date.h" int main() { Date d(2017,3,8); Date d1 = d + 100; d.display(); d1.display(); Date d2(2017, 3, 8); d2 += 100; d2.display(); Date d3 = d2 - (-100); d3.display(); Date d4 = d3 + (-100); d4.display(); int k=d3 > d4; cout << k << endl; Date d5 = d4; (d5++).display(); (++d5).display(); int a = d3 - d4; cout << a << endl; system("pause"); return 0; }
运行结果:
总结:
在实现日期类的时候要注意代码的复用+简洁,以及什么时候能够返回一个值的引用来使函数的效率更高(不能返回指向栈内存的引用和指针),这些都是需要注意的,以及函数的重载的理解(函数名可以相同,参数的类型不同或者参数的个数不同(参数列表不同),返回值可同可不同)。
相关文章推荐
- C++ 声明并实现一个日期类(运算符重载)
- 用c/c++混合编程方式为ios/android实现一个自绘日期选择控件(一)
- C++ 实现一个日期类
- C++ 实现一个日期类
- 【C++】模拟实现一个日期类,实现基本重载运算符等
- (android/swig实现)用c/c++混合编程方式为ios/android实现一个自绘日期选择控件(三)
- 用C++实现,输入一个日期,输出它是一年中的第几天。
- c++::实现一个日期类
- c++实现一个日期类
- C++实现一个日期类
- C++实现一个日期类
- (ios实现)用c/c++混合编程方式为ios/android实现一个自绘日期选择控件(二)
- 用C/C++实现一个日期类,重载运算符=,==,+,-,++,--,>,>=,<,<=等
- C++ 实现判断一个输入日期是星期几,是一年中的第几天
- 用C++实现,输入一个日期,输出它是一年中的第几天。
- 【C++基础】实现一个日期类
- C++实现的一个哈希表类
- 用C++的高级模版特性实现一个不需要IDL的RPC
- 用vbs实现按创建日期的顺序列出一个文件夹中的所有文件
- 用C++实现一个二叉树类