Spring事务管理源码分析
2017-03-08 13:55
633 查看
Spring事务管理方式
依据Spring.xsd文件可以发现,Spring提供了advice,annotation-driven,jta-transaction-manager3种事务管理方式。详情可查看相应版本xsd文件。这里参照的版本是3.2。我们也只分析advice方式的源码,期望以此为突破口了解Spring事务管理的原理。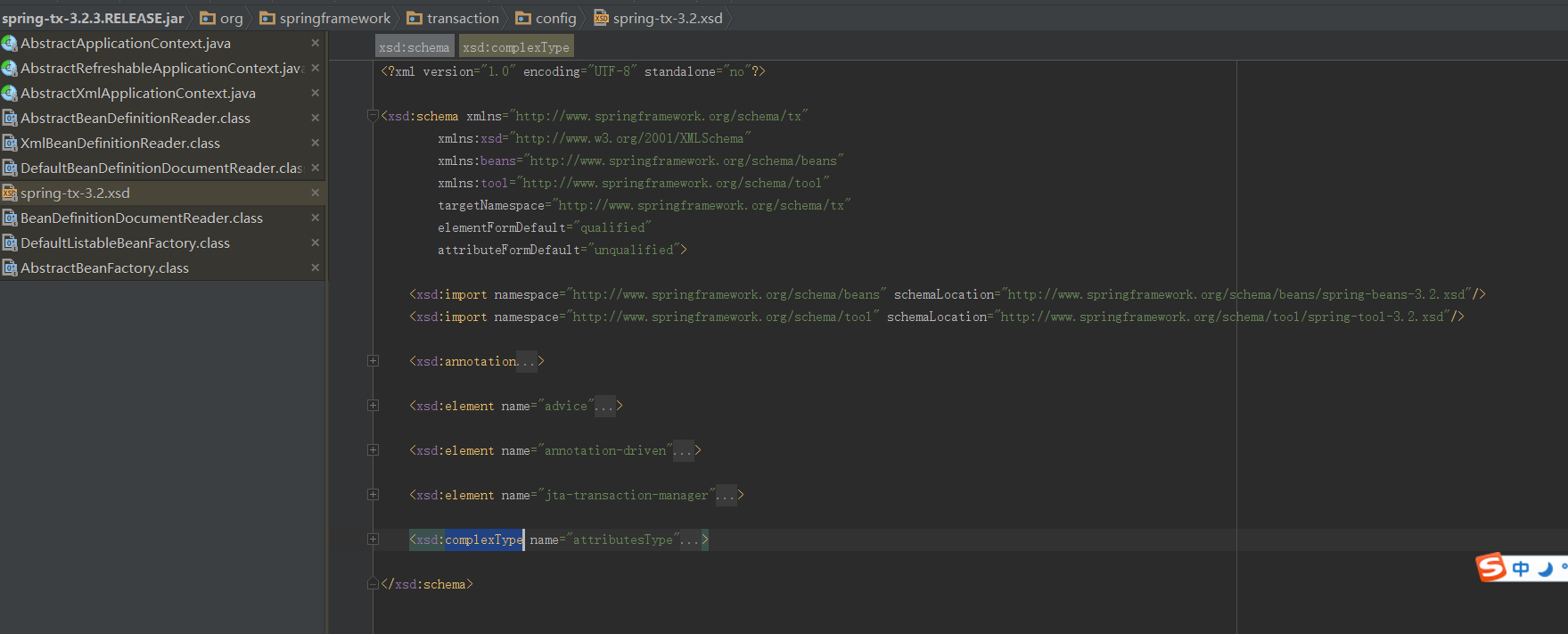
Advice事务管理
XSD文件
我们先来看下xsd文件的配置<xsd:element name="advice"> <xsd:complexType> <xsd:annotation> <xsd:documentation source="java:org.springframework.transaction.interceptor.TransactionInterceptor"><![CDATA[ Defines the transactional semantics of the AOP advice that is to be executed. That is, this advice element is where the transactional semantics of any number of methods are defined (where transactional semantics includes the propagation settings, the isolation level, the rollback rules, and suchlike). ]]></xsd:documentation> <xsd:appinfo> <tool:annotation> <tool:exports type="org.springframework.transaction.interceptor.TransactionInterceptor"/> </tool:annotation> </xsd:appinfo> </xsd:annotation> <xsd:complexContent> <xsd:extension base="beans:identifiedType"> <xsd:sequence> <xsd:element name="attributes" type="attributesType" minOccurs="0" maxOccurs="1"/> </xsd:sequence> <xsd:attribute name="transaction-manager" type="xsd:string" default="transactionManager"> <xsd:annotation> <xsd:documentation source="java:org.springframework.transaction.PlatformTransactionManager"><![CDATA[ The bean name of the PlatformTransactionManager that is to be used to drive transactions. This attribute is not required, and only needs to be specified explicitly if the bean name of the desired PlatformTransactionManager is not 'transactionManager'. ]]></xsd:documentation> <xsd:appinfo> <tool:annotation kind="ref"> <tool:expected-type type="org.springframework.transaction.PlatformTransactionManager"/> </tool:annotation> </xsd:appinfo> </xsd:annotation> </xsd:attribute> </xsd:extension> </xsd:complexContent> </xsd:complexType> </xsd:element>
文档中的注释大概语义如下:
定义AOP的advice的执行事务语义.也就是说,这个元素可以定义任意数量的事务语义(事务语义包括传播设置,隔离级别,回滚规则等等)
名为platformtransactionmanager的bean是该模式下用来 驱动事务的默认方式。 这个属性不是必选属性,只有在要求PlatformTransactionManager不是transactionManager的时候显性设置
由此可得,此方式下的默认advice方式的事务管理方式默认是PlatformTransactionManager(bean进行装载的这种自定义标签的时候,注册主配置的TransactionInterceptor事件)。
具体实现
这里看核心源码// // Source code recreated from a .class file by IntelliJ IDEA // (powered by Fernflower decompiler) // package org.springframework.transaction.interceptor; import java.io.IOException; import java.io.ObjectInputStream; import java.io.ObjectOutputStream; import java.io.Serializable; import java.util.Properties; import org.aopalliance.intercept.MethodInterceptor; import org.aopalliance.intercept.MethodInvocation; import org.springframework.aop.support.AopUtils; import org.springframework.beans.factory.BeanFactory; import org.springframework.transaction.PlatformTransactionManager; import org.springframework.transaction.interceptor.TransactionAspectSupport; import org.springframework.transaction.interceptor.TransactionAttributeSource; import org.springframework.transaction.interceptor.TransactionAspectSupport.InvocationCallback; public class TransactionInterceptor extends TransactionAspectSupport implements MethodInterceptor, Serializable { public TransactionInterceptor() { } //根据配置文件注入transactionManager,以及attribute public TransactionInterceptor(PlatformTransactionManager ptm, Properties attributes) { this.setTransactionManager(ptm); this.setTransactionAttributes(attributes); } //同上 public TransactionInterceptor(PlatformTransactionManager ptm, TransactionAttributeSource tas) { this.setTransactionManager(ptm); this.setTransactionAttributeSource(tas); } //执行 核心是invokewithinTransaction public Object invoke(final MethodInvocation invocation) throws Throwable { Class targetClass = invocation.getThis() != null?AopUtils.getTargetClass(invocation.getThis()):null; return this.invokeWithinTransaction(invocation.getMethod(), targetClass, new InvocationCallback() { public Object proceedWithInvocation() throws Throwable { return invocation.proceed(); } }); } private void writeObject(ObjectOutputStream oos) throws IOException { oos.defaultWriteObject(); oos.writeObject(this.getTransactionManagerBeanName()); oos.writeObject(this.getTransactionManager()); oos.writeObject(this.getTransactionAttributeSource()); oos.writeObject(this.getBeanFactory()); } private void readObject(ObjectInputStream ois) throws IOException, ClassNotFoundException { ois.defaultReadObject(); this.setTransactionManagerBeanName((String)ois.readObject()); this.setTransactionManager((PlatformTransactionManager)ois.readObject()); this.setTransactionAttributeSource((TransactionAttributeSource)ois.readObject()); this.setBeanFactory((BeanFactory)ois.readObject()); } }
TransactionAspectSupport的 invokeWithinTransaction方法
/* * Copyright 2002-2013 the original author or authors. * * Licensed under the Apache License, Version 2.0 (the "License"); * you may not use this file except in compliance with the License. * You may obtain a copy of the License at * * http://www.apache.org/licenses/LICENSE-2.0 * * Unless required by applicable law or agreed to in writing, software * distributed under the License is distributed on an "AS IS" BASIS, * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. * See the License for the specific language governing permissions and * limitations under the License. */ package org.springframework.transaction.interceptor; import org.apache.commons.logging.Log; import org.apache.commons.logging.LogFactory; import org.springframework.beans.factory.BeanFactory; import org.springframework.beans.factory.BeanFactoryAware; import org.springframework.beans.factory.InitializingBean; import org.springframework.beans.factory.annotation.BeanFactoryAnnotationUtils; import org.springframework.core.NamedThreadLocal; import org.springframework.transaction.NoTransactionException; import org.springframework.transaction.PlatformTransactionManager; import org.springframework.transaction.TransactionStatus; import org.springframework.transaction.TransactionSystemException; import org.springframework.transaction.support.CallbackPreferringPlatformTransactionManager; import org.springframework.transaction.support.TransactionCallback; import org.springframework.util.StringUtils; import java.lang.reflect.Method; import java.util.Properties; /** * Base class for transactional aspects, such as the {@link TransactionInterceptor} * or an AspectJ aspect. * * <p>This enables the underlying Spring transaction infrastructure to be used easily * to implement an aspect for any aspect system. * * <p>Subclasses are responsible for calling methods in this class in the correct order. * * <p>If no transaction name has been specified in the {@code TransactionAttribute}, * the exposed name will be the {@code fully-qualified class name + "." + method name} * (by default). * * <p>Uses the <b>Strategy</b> design pattern. A {@code PlatformTransactionManager} * implementation will perform the actual transaction management, and a * {@code TransactionAttributeSource} is used for determining transaction definitions. * * <p>A transaction aspect is serializable if its {@code PlatformTransactionManager} * and {@code TransactionAttributeSource} are serializable. * * @author Rod Johnson * @author Juergen Hoeller * @since 1.1 * @see #setTransactionManager * @see #setTransactionAttributes * @see #setTransactionAttributeSource */ public abstract class TransactionAspectSupport implements BeanFactoryAware, InitializingBean { // NOTE: This class must not implement Serializable because it serves as base // class for AspectJ aspects (which are not allowed to implement Serializable)! /** * Holder to support the {@code currentTransactionStatus()} method, * and to support communication between different cooperating advices * (e.g. before and after advice) if the aspect involves more than a * single method (as will be the case for around advice). */ private static final ThreadLocal<TransactionInfo> transactionInfoHolder = new NamedThreadLocal<TransactionInfo>("Current aspect-driven transaction"); /** * Subclasses can use this to return the current TransactionInfo. * Only subclasses that cannot handle all operations in one method, * such as an AspectJ aspect involving distinct before and after advice, * need to use this mechanism to get at the current TransactionInfo. * An around advice such as an AOP Alliance MethodInterceptor can hold a * reference to the TransactionInfo throughout the aspect method. * <p>A TransactionInfo will be returned even if no transaction was created. * The {@code TransactionInfo.hasTransaction()} method can be used to query this. * <p>To find out about specific transaction characteristics, consider using * TransactionSynchronizationManager's {@code isSynchronizationActive()} * and/or {@code isActualTransactionActive()} methods. * @return TransactionInfo bound to this thread, or {@code null} if none * @see TransactionInfo#hasTransaction() * @see org.springframework.transaction.support.TransactionSynchronizationManager#isSynchronizationActive() * @see org.springframework.transaction.support.TransactionSynchronizationManager#isActualTransactionActive() */ protected static TransactionInfo currentTransactionInfo() throws NoTransactionException { return transactionInfoHolder.get(); } /** * Return the transaction status of the current method invocation. * Mainly intended for code that wants to set the current transaction * rollback-only but not throw an application exception. * @throws NoTransactionException if the transaction info cannot be found, * because the method was invoked outside an AOP invocation context */ public static TransactionStatus currentTransactionStatus() throws NoTransactionException { TransactionInfo info = currentTransactionInfo(); if (info == null) { throw new NoTransactionException("No transaction aspect-managed TransactionStatus in scope"); } return currentTransactionInfo().transactionStatus; } protected final Log logger = LogFactory.getLog(getClass()); private String transactionManagerBeanName; private PlatformTransactionManager transactionManager; private TransactionAttributeSource transactionAttributeSource; private BeanFactory beanFactory; /** * Specify the name of the default transaction manager bean. */ public void setTransactionManagerBeanName(String transactionManagerBeanName) { this.transactionManagerBeanName = transactionManagerBeanName; } /** * Return the name of the default transaction manager bean. */ protected final String getTransactionManagerBeanName() { return this.transactionManagerBeanName; } /** * Specify the target transaction manager. */ public void setTransactionManager(PlatformTransactionManager transactionManager) { this.transactionManager = transactionManager; } /** * Return the transaction manager, if specified. */ public PlatformTransactionManager getTransactionManager() { return this.transactionManager; } /** * Set properties with method names as keys and transaction attribute * descriptors (parsed via TransactionAttributeEditor) as values: * e.g. key = "myMethod", value = "PROPAGATION_REQUIRED,readOnly". * <p>Note: Method names are always applied to the target class, * no matter if defined in an interface or the class itself. * <p>Internally, a NameMatchTransactionAttributeSource will be * created from the given properties. * @see #setTransactionAttributeSource * @see TransactionAttributeEditor * @see NameMatchTransactionAttributeSource */ public void setTransactionAttributes(Properties transactionAttributes) { NameMatchTransactionAttributeSource tas = new NameMatchTransactionAttributeSource(); tas.setProperties(transactionAttributes); this.transactionAttributeSource = tas; } /** * Set multiple transaction attribute sources which are used to find transaction * attributes. Will build a CompositeTransactionAttributeSource for the given sources. * @see CompositeTransactionAttributeSource * @see MethodMapTransactionAttributeSource * @see NameMatchTransactionAttributeSource * @see org.springframework.transaction.annotation.AnnotationTransactionAttributeSource */ public void setTransactionAttributeSources(TransactionAttributeSource[] transactionAttributeSources) { this.transactionAttributeSource = new CompositeTransactionAttributeSource(transactionAttributeSources); } /** * Set the transaction attribute source which is used to find transaction * attributes. If specifying a String property value, a PropertyEditor * will create a MethodMapTransactionAttributeSource from the value. * @see TransactionAttributeSourceEditor * @see MethodMapTransactionAttributeSource * @see NameMatchTransactionAttributeSource * @see org.springframework.transaction.annotation.AnnotationTransactionAttributeSource */ public void setTransactionAttributeSource(TransactionAttributeSource transactionAttributeSource) { this.transactionAttributeSource = transactionAttributeSource; } /** * Return the transaction attribute source. */ public TransactionAttributeSource getTransactionAttributeSource() { return this.transactionAttributeSource; } /** * Set the BeanFactory to use for retrieving PlatformTransactionManager beans. */ public void setBeanFactory(BeanFactory beanFactory) { this.beanFactory = beanFactory; } /** * Return the BeanFactory to use for retrieving PlatformTransactionManager beans. */ protected final BeanFactory getBeanFactory() { return this.beanFactory; } /** * Check that required properties were set. */ public void afterPropertiesSet() { if (this.transactionManager == null && this.beanFactory == null) { throw new IllegalStateException( "Setting the property 'transactionManager' or running in a ListableBeanFactory is required"); } if (this.transactionAttributeSource == null) { throw new IllegalStateException( "Either 'transactionAttributeSource' or 'transactionAttributes' is required: " + "If there are no transactional methods, then don't use a transaction aspect."); } } /** * General delegate for around-advice-based subclasses, delegating to several other template * methods on this class. Able to handle {@link CallbackPreferringPlatformTransactionManager} * as well as regular {@link PlatformTransactionManager} implementations. * @param method the Method being invoked * @param targetClass the target class that we're invoking the method on * @param invocation the callback to use for proceeding with the target invocation * @return the return value of the method, if any * @throws Throwable propagated from the target invocation */ protected Object invokeWithinTransaction(Method method, Class targetClass, final InvocationCallback invocation) throws Throwable { // If the transaction attribute is null, the method is non-transactional. final TransactionAttribute txAttr = getTransactionAttributeSource().getTransactionAttribute(method, targetClass); final PlatformTransactionManager tm = determineTransactionManager(txAttr); final String joinpointIdentification = methodIdentification(method, targetClass); //默认事务管理方式 advice参数不为空,且不是偏向事务管理实例 if (txAttr == null || !(tm instanceof CallbackPreferringPlatformTransactionManager)) { // Standard transaction demarcation with getTransaction and commit/rollback calls. TransactionInfo txInfo = createTransactionIfNecessary(tm, txAttr, joinpointIdentification); Object retVal = null; try { // This is an around advice: Invoke the next interceptor in the chain. // This will normally result in a target object being invoked. retVal = invocation.proceedWithInvocation(); } catch (Throwable ex) { // target invocation exception completeTransactionAfterThrowing(txInfo, ex); throw ex; } finally { cleanupTransactionInfo(txInfo); } //提交事务 commitTransactionAfterReturning(txInfo); return retVal; } else { // It's a CallbackPreferringPlatformTransactionManager: pass a TransactionCallback in. try { Object result = ((CallbackPreferringPlatformTransactionManager) tm).execute(txAttr, new TransactionCallback<Object>() { public Object doInTransaction(TransactionStatus status) { TransactionInfo txInfo = prepareTransactionInfo(tm, txAttr, joinpointIdentification, status); try { return invocation.proceedWithInvocation(); } catch (Throwable ex) { if (txAttr.rollbackOn(ex)) { // A RuntimeException: will lead to a rollback. if (ex instanceof RuntimeException) { throw (RuntimeException) ex; } else { throw new ThrowableHolderException(ex); } } else { // A normal return value: will lead to a commit. return new ThrowableHolder(ex); } } finally { cleanupTransactionInfo(txInfo); } } }); // Check result: It might indicate a Throwable to rethrow. if (result instanceof ThrowableHolder) { throw ((ThrowableHolder) result).getThrowable(); } else { return result; } } catch (ThrowableHolderException ex) { throw ex.getCause(); } } } /** * Determine the specific transaction manager to use for the given transaction. */ protected PlatformTransactionManager determineTransactionManager(TransactionAttribute txAttr) { if (this.transactionManager != null || this.beanFactory == null || txAttr == null) { return this.transactionManager; } String qualifier = txAttr.getQualifier(); if (StringUtils.hasLength(qualifier)) { return BeanFactoryAnnotationUtils.qualifiedBeanOfType(this.beanFactory, PlatformTransactionManager.class, qualifier); } else if (this.transactionManagerBeanName != null) { return this.beanFactory.getBean(this.transactionManagerBeanName, PlatformTransactionManager.class); } else { return this.beanFactory.getBean(PlatformTransactionManager.class); } } /** * Convenience method to return a String representation of this Method * for use in logging. Can be overridden in subclasses to provide a * different identifier for the given method. * @param method the method we're interested in * @param targetClass the class that the method is being invoked on * @return a String representation identifying this method * @see org.springframework.util.ClassUtils#getQualifiedMethodName */ protected String methodIdentification(Method method, Class targetClass) { String simpleMethodId = methodIdentification(method); if (simpleMethodId != null) { return simpleMethodId; } return (targetClass != null ? targetClass : method.getDeclaringClass()).getName() + "." + method.getName(); } /** * Convenience method to return a String representation of this Method * for use in logging. Can be overridden in subclasses to provide a * different identifier for the given method. * @param method the method we're interested in * @return a String representation identifying this method * @deprecated in favor of {@link #methodIdentification(Method, Class)} */ @Deprecated protected String methodIdentification(Method method) { return null; } /** * Create a transaction if necessary, based on the given method and class. * <p>Performs a default TransactionAttribute lookup for the given method. * @param method the method about to execute * @param targetClass the class that the method is being invoked on * @return a TransactionInfo object, whether or not a transaction was created. * The {@code hasTransaction()} method on TransactionInfo can be used to * tell if there was a transaction created. * @see #getTransactionAttributeSource() * @deprecated in favor of * {@link #createTransactionIfNecessary(PlatformTransactionManager, TransactionAttribute, String)} */ @Deprecated protected TransactionInfo createTransactionIfNecessary(Method method, Class targetClass) { // If the transaction attribute is null, the method is non-transactional. TransactionAttribute txAttr = getTransactionAttributeSource().getTransactionAttribute(method, targetClass); PlatformTransactionManager tm = determineTransactionManager(txAttr); return createTransactionIfNecessary(tm, txAttr, methodIdentification(method, targetClass)); } /** * Create a transaction if necessary based on the given TransactionAttribute. * <p>Allows callers to perform custom TransactionAttribute lookups through * the TransactionAttributeSource. * @param txAttr the TransactionAttribute (may be {@code null}) * @param joinpointIdentification the fully qualified method name * (used for monitoring and logging purposes) * @return a TransactionInfo object, whether or not a transaction was created. * The {@code hasTransaction()} method on TransactionInfo can be used to * tell if there was a transaction created. * @see #getTransactionAttributeSource() */ @SuppressWarnings("serial") protected TransactionInfo createTransactionIfNecessary( PlatformTransactionManager tm, TransactionAttribute txAttr, final String joinpointIdentification) { // If no name specified, apply method identification as transaction name. if (txAttr != null && txAttr.getName() == null) { txAttr = new DelegatingTransactionAttribute(txAttr) { @Override public String getName() { return joinpointIdentification; } }; } TransactionStatus status = null; if (txAttr != null) { if (tm != null) { status = tm.getTransaction(txAttr); } else { if (logger.isDebugEnabled()) { logger.debug("Skipping transactional joinpoint [" + joinpointIdentification + "] because no transaction manager has been configured"); } } } return prepareTransactionInfo(tm, txAttr, joinpointIdentification, status); } /** * Prepare a TransactionInfo for the given attribute and status object. * @param txAttr the TransactionAttribute (may be {@code null}) * @param joinpointIdentification the fully qualified method name * (used for monitoring and logging purposes) * @param status the TransactionStatus for the current transaction * @return the prepared TransactionInfo object */ protected TransactionInfo prepareTransactionInfo(PlatformTransactionManager tm, TransactionAttribute txAttr, String joinpointIdentification, TransactionStatus status) { TransactionInfo txInfo = new TransactionInfo(tm, txAttr, joinpointIdentification); if (txAttr != null) { // We need a transaction for this method if (logger.isTraceEnabled()) { logger.trace("Getting transaction for [" + txInfo.getJoinpointIdentification() + "]"); } // The transaction manager will flag an error if an incompatible tx already exists txInfo.newTransactionStatus(status); } else { // The TransactionInfo.hasTransaction() method will return // false. We created it only to preserve the integrity of // the ThreadLocal stack maintained in this class. if (logger.isTraceEnabled()) logger.trace("Don't need to create transaction for [" + joinpointIdentification + "]: This method isn't transactional."); } // We always bind the TransactionInfo to the thread, even if we didn't create // a new transaction here. This guarantees that the TransactionInfo stack // will be managed correctly even if no transaction was created by this aspect. txInfo.bindToThread(); return txInfo; } /** * Execute after successful completion of call, but not after an exception was handled. * Do nothing if we didn't create a transaction. * @param txInfo information about the current transaction */ protected void commitTransactionAfterReturning(TransactionInfo txInfo) { if (txInfo != null && txInfo.hasTransaction()) { if (logger.isTraceEnabled()) { logger.trace("Completing transaction for [" + txInfo.getJoinpointIdentification() + "]"); } txInfo.getTransactionManager().commit(txInfo.getTransactionStatus()); } } /** * Handle a throwable, completing the transaction. * We may commit or roll back, depending on the configuration. * @param txInfo information about the current transaction * @param ex throwable encountered */ protected void completeTransactionAfterThrowing(TransactionInfo txInfo, Throwable ex) { if (txInfo != null && txInfo.hasTransaction()) { if (logger.isTraceEnabled()) { logger.trace("Completing transaction for [" + txInfo.getJoinpointIdentification() + "] after exception: " + ex); } if (txInfo.transactionAttribute.rollbackOn(ex)) { try { txInfo.getTransactionManager().rollback(txInfo.getTransactionStatus()); } catch (TransactionSystemException ex2) { logger.error("Application exception overridden by rollback exception", ex); ex2.initApplicationException(ex); throw ex2; } catch (RuntimeException ex2) { logger.error("Application exception overridden by rollback exception", ex); throw ex2; } catch (Error err) { logger.error("Application exception overridden by rollback error", ex); throw err; } } else { // We don't roll back on this exception. // Will still roll back if TransactionStatus.isRollbackOnly() is true. try { txInfo.getTransactionManager().commit(txInfo.getTransactionStatus()); } catch (TransactionSystemException ex2) { logger.error("Application exception overridden by commit exception", ex); ex2.initApplicationException(ex); throw ex2; } catch (RuntimeException ex2) { logger.error("Application exception overridden by commit exception", ex); throw ex2; } catch (Error err) { logger.error("Application exception overridden by commit error", ex); throw err; } } } } /** * Reset the TransactionInfo ThreadLocal. * <p>Call this in all cases: exception or normal return! * @param txInfo information about the current transaction (may be {@code null}) */ protected void cleanupTransactionInfo(TransactionInfo txInfo) { if (txInfo != null) { txInfo.restoreThreadLocalStatus(); } } /** * Opaque object used to hold Transaction information. Subclasses * must pass it back to methods on this class, but not see its internals. */ protected final class TransactionInfo { private final PlatformTransactionManager transactionManager; private final TransactionAttribute transactionAttribute; private final String joinpointIdentification; private TransactionStatus transactionStatus; private TransactionInfo oldTransactionInfo; public TransactionInfo(PlatformTransactionManager transactionManager, TransactionAttribute transactionAttribute, String joinpointIdentification) { this.transactionManager = transactionManager; this.transactionAttribute = transactionAttribute; this.joinpointIdentification = joinpointIdentification; } public PlatformTransactionManager getTransactionManager() { return this.transactionManager; } public TransactionAttribute getTransactionAttribute() { return this.transactionAttribute; } /** * Return a String representation of this joinpoint (usually a Method call) * for use in logging. */ public String getJoinpointIdentification() { return this.joinpointIdentification; } public void newTransactionStatus(TransactionStatus status) { this.transactionStatus = status; } public TransactionStatus getTransactionStatus() { return this.transactionStatus; } /** * Return whether a transaction was created by this aspect, * or whether we just have a placeholder to keep ThreadLocal stack integrity. */ public boolean hasTransaction() { return (this.transactionStatus != null); } private void bindToThread() { // Expose current TransactionStatus, preserving any existing TransactionStatus // for restoration after this transaction is complete. this.oldTransactionInfo = transactionInfoHolder.get(); transactionInfoHolder.set(this); } private void restoreThreadLocalStatus() { // Use stack to restore old transaction TransactionInfo. // Will be null if none was set. transactionInfoHolder.set(this.oldTransactionInfo); } @Override public String toString() { return this.transactionAttribute.toString(); } } /** * Simple callback interface for proceeding with the target invocation. * Concrete interceptors/aspects adapt this to their invocation mechanism. */ protected interface InvocationCallback { Object proceedWithInvocation() throws Throwable; } /** * Internal holder class for a Throwable, used as a return value * from a TransactionCallback (to be subsequently unwrapped again). */ private static class ThrowableHolder { private final Throwable throwable; public ThrowableHolder(Throwable throwable) { this.throwable = throwable; } public final Throwable getThrowable() { return this.throwable; } } /** * Internal holder class for a Throwable, used as a RuntimeException to be * thrown from a TransactionCallback (and subsequently unwrapped again). */ @SuppressWarnings("serial") private static class ThrowableHolderException extends RuntimeException { public ThrowableHolderException(Throwable throwable) { super(throwable); } @Override public String toString() { return getCause().toString(); } } }
AbstractPlatformTransactionManager 事务提交核心代码
/** * This implementation of commit handles participating in existing * transactions and programmatic rollback requests. * Delegates to {@code isRollbackOnly}, {@code doCommit} * and {@code rollback}. * @see org.springframework.transaction.TransactionStatus#isRollbackOnly() * @see #doCommit * @see #rollback */ public final void commit(TransactionStatus status) throws TransactionException { if (status.isCompleted()) { throw new IllegalTransactionStateException( "Transaction is already completed - do not call commit or rollback more than once per transaction"); } DefaultTransactionStatus defStatus = (DefaultTransactionStatus) status; if (defStatus.isLocalRollbackOnly()) { if (defStatus.isDebug()) { logger.debug("Transactional code has requested rollback"); } processRollback(defStatus); return; } if (!shouldCommitOnGlobalRollbackOnly() && defStatus.isGlobalRollbackOnly()) { if (defStatus.isDebug()) { logger.debug("Global transaction is marked as rollback-only but transactional code requested commit"); } processRollback(defStatus); // Throw UnexpectedRollbackException only at outermost transaction boundary // or if explicitly asked to. if (status.isNewTransaction() || isFailEarlyOnGlobalRollbackOnly()) { throw new UnexpectedRollbackException( "Transaction rolled back because it has been marked as rollback-only"); } return; } processCommit(defStatus); }
AbstractPlatformTransactionManager。 processCommit
/** * Process an actual commit. * Rollback-only flags have already been checked and applied. * @param status object representing the transaction * @throws TransactionException in case of commit failure */ private void processCommit(DefaultTransactionStatus status) throws TransactionException { try { boolean beforeCompletionInvoked = false; try { prepareForCommit(status); triggerBeforeCommit(status); triggerBeforeCompletion(status); beforeCompletionInvoked = true; boolean globalRollbackOnly = false; if (status.isNewTransaction() || isFailEarlyOnGlobalRollbackOnly()) { globalRollbackOnly = status.isGlobalRollbackOnly(); } if (status.hasSavepoint()) { if (status.isDebug()) { logger.debug("Releasing transaction savepoint"); } status.releaseHeldSavepoint(); } else if (status.isNewTransaction()) { if (status.isDebug()) { logger.debug("Initiating transaction commit"); } doCommit(status); } // Throw UnexpectedRollbackException if we have a global rollback-only // marker but still didn't get a corresponding exception from commit. if (globalRollbackOnly) { throw new UnexpectedRollbackException( "Transaction silently rolled back because it has been marked as rollback-only"); } } catch (UnexpectedRollbackException ex) { // can only be caused by doCommit triggerAfterCompletion(status, TransactionSynchronization.STATUS_ROLLED_BACK); throw ex; } catch (TransactionException ex) { // can only be caused by doCommit if (isRollbackOnCommitFailure()) { doRollbackOnCommitException(status, ex); } else { triggerAfterCompletion(status, TransactionSynchronization.STATUS_UNKNOWN); } throw ex; } catch (RuntimeException ex) { if (!beforeCompletionInvoked) { triggerBeforeCompletion(status); } doRollbackOnCommitException(status, ex); throw ex; } catch (Error err) { if (!beforeCompletionInvoked) { triggerBeforeCompletion(status); } doRollbackOnCommitException(status, err); throw err; } // Trigger afterCommit callbacks, with an exception thrown there // propagated to callers but the transaction still considered as committed. try { triggerAfterCommit(status); } finally { triggerAfterCompletion(status, TransactionSynchronization.STATUS_COMMITTED); } } finally { cleanupAfterCompletion(status); } }
DataSourceTransactionManager. doCommit
@Override protected void doCommit(DefaultTransactionStatus status) { DataSourceTransactionObject txObject = (DataSourceTransactionObject) status.getTransaction(); Connection con = txObject.getConnectionHolder().getConnection(); if (status.isDebug()) { logger.debug("Committing JDBC transaction on Connection [" + con + "]"); } try { con.commit();//底层jdbc提交 } catch (SQLException ex) { throw new TransactionSystemException("Could not commit JDBC transaction", ex); } }
总结
spring的事务管理有3种(advice,annotation-driven,jta-transaction-manage),而通过对advice方式的源码进行分析,发现 TransactionAspectSupport的transactionInfoHolderthreadLoca线程中存储每条数据库操作线程的事务行为,并在相关方法中执行了一系列的安全事务策略(比如准备策略,偏向事务管理)等。相关文章推荐
- spring源码分析之——spring 事务管理实现方式 (不太清晰,不明白aop会看不懂)
- mybatis源码分析(8)-----事务(mybatis管理、spring管理)
- spring源码分析之——spring 事务管理实现方式
- 全面分析 Spring 的编程式事务管理及声明式事务管理
- 全面分析 Spring 的编程式事务管理及声明式事务管理
- Spring事务分析(2)--基于声明式的事务管理实现分析
- 全面分析 Spring 的编程式事务管理及声明式事务管理
- 全面分析 Spring 的编程式事务管理及声明式事务管理--转
- 全面分析 Spring 的编程式事务管理及声明式事务管理(转)
- 对Spring事务管理实现技术的分析
- 全面分析 Spring 的编程式事务管理及声明式事务管理
- 全面分析 Spring 的编程式事务管理及声明式事务管理
- 全面分析 Spring 的编程式事务管理及声明式事务管理
- 全面分析 Spring 的编程式事务管理及声明式事务管理
- 全面分析 Spring 的编程式事务管理及声明式事务管理
- 全面分析 Spring 的编程式事务管理及声明式事务管理(1)
- 全面分析 Spring 的编程式事务管理及声明式事务管理(转)
- 全面分析spring编程式事务与声明式事务管理
- 全面分析 Spring 的编程式事务管理及声明式事务管理
- 新手程序员笔记-Spring-全面分析Spring 的编程式事务管理及声明式事务管理【转】